Python定义线性表的方法有很多种,常用的方法包括:使用列表、使用链表、使用numpy数组等。列表是最常见和最简单的方法,因为它在Python中是内置的数据结构,支持动态大小和各种常用操作。链表则更适合需要频繁插入和删除操作的场景。
在本节中,我们将详细介绍如何在Python中定义和操作线性表,涵盖列表和链表的定义、操作、以及一些高级用法。
一、使用列表定义线性表
1、列表的定义与基本操作
列表(List)是Python内置的一个数据类型,它是一种有序的集合,可以随时添加和删除其中的元素。
# 定义一个列表
linear_list = [1, 2, 3, 4, 5]
访问列表元素
print(linear_list[0]) # 输出: 1
添加元素
linear_list.append(6)
print(linear_list) # 输出: [1, 2, 3, 4, 5, 6]
删除元素
linear_list.remove(3)
print(linear_list) # 输出: [1, 2, 4, 5, 6]
插入元素
linear_list.insert(2, 3)
print(linear_list) # 输出: [1, 2, 3, 4, 5, 6]
列表长度
print(len(linear_list)) # 输出: 6
列表是动态数组,因此在实际使用中非常灵活,可以根据需要动态扩展。
2、列表的高级操作
除了基本操作,列表还支持切片、排序、反转等高级操作。
# 列表切片
sub_list = linear_list[1:4]
print(sub_list) # 输出: [2, 3, 4]
列表排序
linear_list.sort()
print(linear_list) # 输出: [1, 2, 3, 4, 5, 6]
列表反转
linear_list.reverse()
print(linear_list) # 输出: [6, 5, 4, 3, 2, 1]
这些操作使得列表在处理线性表时非常高效和方便。
二、使用链表定义线性表
1、链表的定义
链表(Linked List)是一种线性表,但不像列表那样存储在连续的内存位置。每个节点包含两个部分:数据部分和指向下一个节点的指针。
首先,我们需要定义一个节点类:
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
然后,定义一个链表类:
class LinkedList:
def __init__(self):
self.head = None
def is_empty(self):
return self.head is None
def append(self, data):
new_node = Node(data)
if self.is_empty():
self.head = new_node
return
last_node = self.head
while last_node.next:
last_node = last_node.next
last_node.next = new_node
def display(self):
current_node = self.head
while current_node:
print(current_node.data, end=" -> ")
current_node = current_node.next
print("None")
创建一个链表并添加一些元素
linked_list = LinkedList()
linked_list.append(1)
linked_list.append(2)
linked_list.append(3)
linked_list.display() # 输出: 1 -> 2 -> 3 -> None
2、链表的基本操作
链表支持基本操作,如插入、删除、查找等。
class LinkedList:
# 之前的代码...
def insert(self, data, position):
if position < 0:
raise ValueError("Position must be non-negative")
new_node = Node(data)
if position == 0:
new_node.next = self.head
self.head = new_node
return
current_node = self.head
current_position = 0
while current_node and current_position < position - 1:
current_node = current_node.next
current_position += 1
if not current_node:
raise IndexError("Position out of bounds")
new_node.next = current_node.next
current_node.next = new_node
def delete(self, position):
if self.is_empty():
raise IndexError("List is empty")
if position < 0:
raise ValueError("Position must be non-negative")
if position == 0:
self.head = self.head.next
return
current_node = self.head
current_position = 0
while current_node.next and current_position < position - 1:
current_node = current_node.next
current_position += 1
if not current_node.next:
raise IndexError("Position out of bounds")
current_node.next = current_node.next.next
创建一个链表并进行操作
linked_list = LinkedList()
linked_list.append(1)
linked_list.append(2)
linked_list.append(3)
linked_list.insert(4, 2)
linked_list.display() # 输出: 1 -> 2 -> 4 -> 3 -> None
linked_list.delete(1)
linked_list.display() # 输出: 1 -> 4 -> 3 -> None
三、使用NumPy数组定义线性表
1、NumPy数组的定义与基本操作
NumPy是一个用于科学计算的Python库,它提供了一个强大的N维数组对象。
import numpy as np
定义一个NumPy数组
linear_array = np.array([1, 2, 3, 4, 5])
访问数组元素
print(linear_array[0]) # 输出: 1
添加元素
linear_array = np.append(linear_array, 6)
print(linear_array) # 输出: [1 2 3 4 5 6]
删除元素
linear_array = np.delete(linear_array, 2)
print(linear_array) # 输出: [1 2 4 5 6]
插入元素
linear_array = np.insert(linear_array, 2, 3)
print(linear_array) # 输出: [1 2 3 4 5 6]
2、NumPy数组的高级操作
NumPy数组支持各种高级操作,如切片、矩阵运算、统计分析等。
# 数组切片
sub_array = linear_array[1:4]
print(sub_array) # 输出: [2 3 4]
数组排序
sorted_array = np.sort(linear_array)
print(sorted_array) # 输出: [1 2 3 4 5 6]
数组反转
reversed_array = linear_array[::-1]
print(reversed_array) # 输出: [6 5 4 3 2 1]
四、使用类封装线性表
1、封装线性表类
为了更好地管理线性表,我们可以将其封装到一个类中。
class LinearList:
def __init__(self):
self.elements = []
def add(self, element):
self.elements.append(element)
def remove(self, element):
self.elements.remove(element)
def insert(self, index, element):
self.elements.insert(index, element)
def display(self):
print(self.elements)
使用封装类
linear_list = LinearList()
linear_list.add(1)
linear_list.add(2)
linear_list.add(3)
linear_list.display() # 输出: [1, 2, 3]
linear_list.insert(1, 4)
linear_list.display() # 输出: [1, 4, 2, 3]
linear_list.remove(2)
linear_list.display() # 输出: [1, 4, 3]
2、封装链表类
我们也可以封装链表类,以便更好地管理链表。
class LinkedList:
def __init__(self):
self.head = None
def add(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
last_node = self.head
while last_node.next:
last_node = last_node.next
last_node.next = new_node
def remove(self, data):
if not self.head:
return
if self.head.data == data:
self.head = self.head.next
return
current_node = self.head
while current_node.next and current_node.next.data != data:
current_node = current_node.next
if not current_node.next:
return
current_node.next = current_node.next.next
def display(self):
current_node = self.head
while current_node:
print(current_node.data, end=" -> ")
current_node = current_node.next
print("None")
使用封装类
linked_list = LinkedList()
linked_list.add(1)
linked_list.add(2)
linked_list.add(3)
linked_list.display() # 输出: 1 -> 2 -> 3 -> None
linked_list.remove(2)
linked_list.display() # 输出: 1 -> 3 -> None
五、线性表的实际应用
1、线性表在数据分析中的应用
线性表在数据分析中非常常见,尤其是在处理数据集时。
import pandas as pd
创建一个数据框
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]}
df = pd.DataFrame(data)
访问数据
print(df['Name']) # 输出: 0 Alice
# 1 Bob
# 2 Charlie
# Name: Name, dtype: object
添加数据
df.loc[3] = ['David', 40]
print(df) # 输出:
# Name Age
# 0 Alice 25
# 1 Bob 30
# 2 Charlie 35
# 3 David 40
删除数据
df = df.drop(1)
print(df) # 输出:
# Name Age
# 0 Alice 25
# 2 Charlie 35
# 3 David 40
2、线性表在算法中的应用
线性表在算法中也非常常见,如排序算法、查找算法等。
# 冒泡排序算法
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
使用冒泡排序
arr = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(arr)
print("排序后的数组:", arr) # 输出: 排序后的数组: [11, 12, 22, 25, 34, 64, 90]
六、总结
通过上述内容,我们详细介绍了如何在Python中定义和操作线性表,包括使用列表、链表和NumPy数组的不同方法。每种方法都有其优点和适用场景,选择合适的方法可以提高程序的效率和可读性。
- 列表:适用于大多数通用场景,操作简单直观,适合频繁访问和少量插入删除操作。
- 链表:适用于需要频繁插入和删除操作的场景,但访问元素时效率较低。
- NumPy数组:适用于需要进行大量数值计算和矩阵操作的场景,提供了丰富的科学计算功能。
无论是哪种方法,理解和掌握线性表的定义和操作对于编写高效的Python程序都是非常重要的。
相关问答FAQs:
如何在Python中创建线性表的数据结构?
在Python中,可以使用列表(list)作为线性表的基本实现。列表是一种动态数组,允许存储不同类型的元素,并支持各种操作,如添加、删除和访问元素。此外,可以通过自定义类来实现更复杂的线性表结构,例如链表或双向链表。
线性表与其他数据结构相比有哪些优势?
线性表的主要优势在于其简单性和灵活性。它允许快速访问和操作元素,适合存储有序数据。与数组相比,列表在大小上更为灵活,不需要预先定义长度。与链表相比,列表在随机访问方面更快,但在频繁插入和删除操作时可能不如链表高效。
如何在Python中实现线性表的基本操作?
在Python中,可以使用内置的列表方法来实现线性表的基本操作。例如,使用append()
方法可以向线性表添加元素,使用remove()
方法可以删除指定元素,使用索引访问可以获取或修改特定位置的元素。此外,用户还可以编写自定义函数来实现更多特定功能,如排序或搜索。
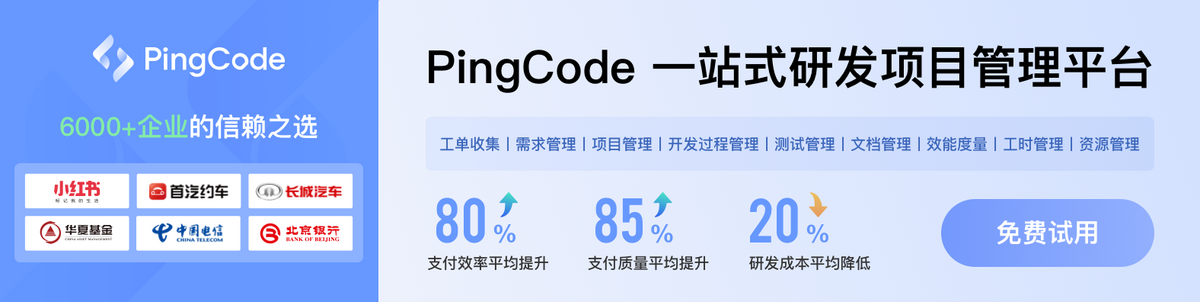