Python查看有多少行的方法有很多,例如使用文件读取、使用内置模块、使用Pandas库等。以下是几种常见的方法:
- 使用基本的文件读取方法;
- 使用内置模块如
os
或pathlib
; - 使用Pandas库读取数据文件并获取行数。
其中,使用基本的文件读取方法是最常用且简单的方法。下面详细介绍这种方法。
打开文件并逐行读取内容,然后使用一个计数器来统计行数。例如:
def count_lines(filepath):
with open(filepath, 'r') as file:
count = sum(1 for line in file)
return count
file_path = 'example.txt'
print(f'The file has {count_lines(file_path)} lines.')
这种方法的优点是简单明了,适用于大多数情况。下面将详细介绍其他方法及其应用场景。
一、使用基本的文件读取方法
1、逐行读取文件
Python提供了多种读取文件的方法,最常见的是使用open()
函数逐行读取文件的内容,然后使用计数器来统计行数。以下是一个示例:
def count_lines(filepath):
count = 0
with open(filepath, 'r') as file:
for line in file:
count += 1
return count
file_path = 'example.txt'
print(f'The file has {count_lines(file_path)} lines.')
这个方法很直观,适用于几乎所有文本文件。with open()
语句确保文件在读取完成后自动关闭。
2、使用内置函数sum
和生成器表达式
另一种简洁的方法是使用内置函数sum
和生成器表达式来统计行数:
def count_lines(filepath):
with open(filepath, 'r') as file:
return sum(1 for line in file)
file_path = 'example.txt'
print(f'The file has {count_lines(file_path)} lines.')
这种方法利用生成器表达式,使代码更加简洁且高效。
二、使用内置模块
1、使用os
模块
os
模块提供了操作系统相关的功能,可以用于查看文件的元数据,包括行数。虽然os
模块没有直接统计行数的函数,但我们可以结合os
模块和文件读取方法来实现:
import os
def count_lines(filepath):
with open(filepath, 'r') as file:
return sum(1 for line in file)
file_path = 'example.txt'
if os.path.exists(file_path):
print(f'The file has {count_lines(file_path)} lines.')
else:
print(f'The file {file_path} does not exist.')
2、使用pathlib
模块
pathlib
模块是Python 3.4引入的一个面向对象的文件系统路径操作库。与os
模块类似,pathlib
也没有直接统计行数的函数,但我们可以结合文件读取方法来实现:
from pathlib import Path
def count_lines(filepath):
with filepath.open('r') as file:
return sum(1 for line in file)
file_path = Path('example.txt')
if file_path.exists():
print(f'The file has {count_lines(file_path)} lines.')
else:
print(f'The file {file_path} does not exist.')
三、使用Pandas库
Pandas是一个强大的数据分析库,常用于处理结构化数据。如果你的文件是CSV、Excel等格式,使用Pandas会非常方便。
1、读取CSV文件
import pandas as pd
def count_lines(filepath):
df = pd.read_csv(filepath)
return len(df)
file_path = 'example.csv'
print(f'The file has {count_lines(file_path)} lines.')
2、读取Excel文件
import pandas as pd
def count_lines(filepath):
df = pd.read_excel(filepath)
return len(df)
file_path = 'example.xlsx'
print(f'The file has {count_lines(file_path)} lines.')
Pandas不仅可以方便地读取文件,还可以执行各种数据处理和分析操作,非常适合处理复杂的数据文件。
四、使用第三方库
除了Pandas,Python还有很多第三方库可以用于统计文件行数。例如fileinput
库,专为处理多个输入文件而设计。
1、使用fileinput
库
fileinput
库可以处理多个输入文件,并支持逐行读取,非常适合统计行数:
import fileinput
def count_lines(filepath):
count = 0
with fileinput.input(files=filepath) as file:
for line in file:
count += 1
return count
file_path = 'example.txt'
print(f'The file has {count_lines(file_path)} lines.')
2、使用wc
命令(Unix系统)
在Unix系统中,你也可以使用wc
命令来统计文件行数,然后通过Python的subprocess
模块调用:
import subprocess
def count_lines(filepath):
result = subprocess.run(['wc', '-l', filepath], stdout=subprocess.PIPE)
return int(result.stdout.split()[0])
file_path = 'example.txt'
print(f'The file has {count_lines(file_path)} lines.')
五、处理大文件
对于非常大的文件,逐行读取可能会消耗大量内存。这时可以采用分块读取的方法,以减少内存占用。
1、分块读取文件
def count_lines(filepath, chunk_size=1024*1024):
count = 0
with open(filepath, 'r') as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
count += chunk.count('\n')
return count
file_path = 'large_example.txt'
print(f'The file has {count_lines(file_path)} lines.')
这种方法适用于内存有限的情况,通过分块读取文件内容,可以有效减少内存占用。
2、使用mmap
模块
mmap
模块提供了对内存映射文件的支持,可以高效处理大文件:
import mmap
def count_lines(filepath):
with open(filepath, 'r+') as file:
mmapped_file = mmap.mmap(file.fileno(), 0)
count = 0
while mmapped_file.readline():
count += 1
mmapped_file.close()
return count
file_path = 'large_example.txt'
print(f'The file has {count_lines(file_path)} lines.')
mmap
模块通过将文件内容映射到内存中,提供了高效的文件读取方式,适合处理非常大的文件。
六、处理特殊格式文件
有时我们需要处理特定格式的文件,如JSON、XML等。这些文件的行数统计方法与普通文本文件有所不同。
1、处理JSON文件
对于JSON文件,可以使用json
模块解析文件内容,然后统计元素的数量:
import json
def count_lines(filepath):
with open(filepath, 'r') as file:
data = json.load(file)
return len(data)
file_path = 'example.json'
print(f'The file has {count_lines(file_path)} elements.')
2、处理XML文件
对于XML文件,可以使用xml.etree.ElementTree
模块解析文件内容,然后统计元素的数量:
import xml.etree.ElementTree as ET
def count_lines(filepath):
tree = ET.parse(filepath)
root = tree.getroot()
return len(root)
file_path = 'example.xml'
print(f'The file has {count_lines(file_path)} elements.')
这种方法适用于结构化的JSON和XML文件,通过解析文件内容,可以准确统计元素的数量。
七、总结
本文介绍了多种统计文件行数的方法,包括基本的文件读取方法、使用内置模块、使用Pandas库、第三方库、处理大文件和特殊格式文件等。每种方法都有其适用的场景和优缺点。
1、基本文件读取方法
适用于大多数情况,代码简单明了。
2、内置模块
结合os
或pathlib
模块,可以实现更多文件操作功能。
3、Pandas库
适用于处理结构化数据文件,如CSV、Excel等。
4、第三方库
如fileinput
库,适用于处理多个输入文件。
5、处理大文件
采用分块读取或mmap
模块,可以有效减少内存占用。
6、特殊格式文件
对于JSON、XML等格式文件,需要使用相应的解析模块。
选择适合的方法,可以更高效地完成文件行数统计任务。希望本文能为你提供有用的参考和帮助。
相关问答FAQs:
如何在Python中读取文件并计算行数?
您可以使用Python的内置函数来打开文件并逐行读取,从而计算文件的总行数。示例代码如下:
with open('your_file.txt', 'r') as file:
line_count = sum(1 for line in file)
print(f"总行数为: {line_count}")
这种方法高效且简洁,适用于大多数文本文件。
是否可以使用Python的库来计算行数?
是的,您可以使用pandas
库来轻松计算文件的行数。只需读取文件并使用len()
函数即可:
import pandas as pd
df = pd.read_csv('your_file.csv')
print(f"总行数为: {len(df)}")
这种方法特别适合处理CSV文件,也能够处理更复杂的数据。
对于大型文件,有哪些优化计算行数的方法?
在处理大型文件时,您可以选择逐块读取文件,以减少内存消耗。使用file.readlines()
方法可以读取特定数量的行,示例代码如下:
def count_lines(filename):
with open(filename, 'r') as file:
count = 0
while True:
lines = file.readlines(1024 * 1024) # 每次读取1MB
if not lines:
break
count += len(lines)
return count
print(f"总行数为: {count_lines('your_large_file.txt')}")
这种方法有效地处理大文件,避免内存溢出问题。
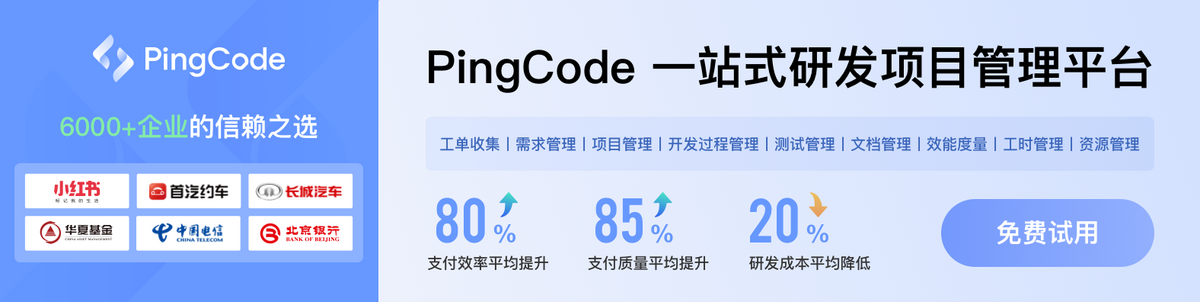