Python获取文件数据的方法有多种,包括使用内置函数open()、使用第三方库如pandas、利用文件路径和模式进行操作。在这三种方法中,open()函数是最基础且最常用的。下面将详细介绍如何利用open()函数获取文件数据。
一、使用open()函数读取文件
open()函数是Python内置的文件操作函数,可以用于读取、写入和追加文件数据。
1、读取文本文件
要读取文本文件,可以使用以下步骤:
- 打开文件
- 读取文件内容
- 关闭文件
# 打开文件并指定读取模式
file = open('example.txt', 'r')
读取文件内容
file_content = file.read()
打印文件内容
print(file_content)
关闭文件
file.close()
2、按行读取文件
有时我们需要逐行读取文件,可以使用readline()
或readlines()
方法:
# 使用readline逐行读取
file = open('example.txt', 'r')
line = file.readline()
while line:
print(line.strip())
line = file.readline()
file.close()
# 使用readlines读取所有行
file = open('example.txt', 'r')
lines = file.readlines()
for line in lines:
print(line.strip())
file.close()
二、使用with语句读取文件
使用with语句可以自动管理文件资源,确保文件在操作完成后自动关闭,避免忘记关闭文件导致的资源泄露。
with open('example.txt', 'r') as file:
file_content = file.read()
print(file_content)
1、按行读取文件
使用with语句逐行读取文件:
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
三、读取CSV文件
CSV文件是常见的数据格式,Python标准库中的csv模块可以方便地读取和写入CSV文件。
import csv
读取CSV文件
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
1、使用DictReader读取CSV文件
DictReader将CSV文件的每一行转换为一个字典,键为列名,值为对应的单元格内容。
import csv
with open('example.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
print(row)
四、读取JSON文件
JSON文件是一种常用的数据交换格式,Python标准库中的json模块可以方便地解析和生成JSON数据。
import json
读取JSON文件
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
五、使用pandas读取文件数据
pandas是Python中强大的数据处理库,可以方便地读取和处理多种格式的数据文件,如CSV、Excel、JSON等。
import pandas as pd
读取CSV文件
df = pd.read_csv('example.csv')
print(df)
读取Excel文件
df = pd.read_excel('example.xlsx')
print(df)
读取JSON文件
df = pd.read_json('example.json')
print(df)
1、处理数据
利用pandas可以方便地对数据进行处理,如筛选、排序、统计等。
# 筛选数据
filtered_df = df[df['column_name'] > 10]
print(filtered_df)
排序数据
sorted_df = df.sort_values(by='column_name')
print(sorted_df)
统计数据
mean_value = df['column_name'].mean()
print(mean_value)
六、读取二进制文件
对于二进制文件,可以使用open()函数并指定'b'模式。
# 读取二进制文件
with open('example.bin', 'rb') as file:
binary_data = file.read()
print(binary_data)
七、使用pathlib读取文件
pathlib模块提供了面向对象的文件和路径操作方式,简化了文件操作。
from pathlib import Path
读取文本文件
file_path = Path('example.txt')
file_content = file_path.read_text()
print(file_content)
读取二进制文件
binary_data = file_path.read_bytes()
print(binary_data)
八、异常处理
在文件操作过程中,可能会遇到文件不存在、权限不足等异常情况,应该使用异常处理机制来捕获和处理这些异常。
try:
with open('example.txt', 'r') as file:
file_content = file.read()
print(file_content)
except FileNotFoundError:
print('文件未找到')
except PermissionError:
print('权限不足')
except Exception as e:
print(f'发生错误:{e}')
九、写入文件
除了读取文件,Python还可以写入文件。
1、写入文本文件
# 写入文本文件
with open('example.txt', 'w') as file:
file.write('Hello, world!')
2、追加文本文件
# 追加文本文件
with open('example.txt', 'a') as file:
file.write('\nHello, again!')
3、写入CSV文件
import csv
写入CSV文件
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age'])
writer.writerow(['Alice', 25])
writer.writerow(['Bob', 30])
4、写入JSON文件
import json
data = {
'name': 'Alice',
'age': 25
}
写入JSON文件
with open('example.json', 'w') as file:
json.dump(data, file)
十、文件模式详解
在使用open()函数时,可以指定不同的模式来控制文件的操作方式。
1、常用模式
- 'r': 读取模式(默认)
- 'w': 写入模式(会覆盖文件)
- 'a': 追加模式
- 'b': 二进制模式
- 't': 文本模式(默认)
2、组合模式
- 'rb': 以二进制读取
- 'wb': 以二进制写入
- 'ab': 以二进制追加
- 'rt': 以文本读取(默认)
- 'wt': 以文本写入
- 'at': 以文本追加
十一、文件路径操作
在文件操作过程中,处理文件路径是常见的需求。
1、获取文件路径
import os
获取当前工作目录
current_dir = os.getcwd()
print(current_dir)
获取文件的绝对路径
file_path = os.path.abspath('example.txt')
print(file_path)
2、路径拼接
import os
拼接路径
file_path = os.path.join(current_dir, 'example.txt')
print(file_path)
3、检查文件是否存在
import os
检查文件是否存在
file_exists = os.path.exists('example.txt')
print(file_exists)
十二、批量读取文件
有时需要一次性读取多个文件,可以使用循环来实现。
import os
获取目录下所有文件
files = os.listdir('example_dir')
for file_name in files:
file_path = os.path.join('example_dir', file_name)
with open(file_path, 'r') as file:
file_content = file.read()
print(file_content)
十三、读取大文件
对于大文件,可以使用生成器或逐行读取的方式,避免一次性加载全部内容造成内存溢出。
# 使用生成器读取大文件
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
for line in read_large_file('large_file.txt'):
print(line.strip())
十四、总结
通过以上内容,我们详细介绍了Python中获取文件数据的多种方法,包括使用open()函数、with语句、pandas库、pathlib模块等。此外,我们还介绍了异常处理、文件路径操作、批量读取文件和读取大文件等高级技巧。这些方法和技巧可以帮助我们在日常编程中更加高效地处理文件数据。
相关问答FAQs:
如何使用Python读取文本文件中的数据?
Python提供了简单易用的文件操作功能,可以通过内置的open()
函数来读取文本文件。使用with
语句可以确保文件在使用后自动关闭。示例代码如下:
with open('文件名.txt', 'r', encoding='utf-8') as file:
data = file.read()
print(data)
这种方法可以有效地读取文件的全部内容,适合较小的文件。
Python是否支持读取CSV文件的数据?
确实支持,Python的csv
模块可以方便地处理CSV文件。你可以使用csv.reader()
函数来逐行读取CSV文件,示例代码如下:
import csv
with open('文件名.csv', 'r', encoding='utf-8') as file:
reader = csv.reader(file)
for row in reader:
print(row)
这种方式可以让你轻松处理CSV格式的数据。
如何从Excel文件中提取数据?
可以使用pandas
库来读取Excel文件。pandas
提供了read_excel()
函数,能够读取Excel文件并将其转化为DataFrame格式。示例代码如下:
import pandas as pd
data = pd.read_excel('文件名.xlsx')
print(data)
这种方法适合处理结构化数据,并且支持多种数据格式的处理。
如何读取JSON文件中的数据?
Python的json
模块可以轻松地读取JSON文件。使用json.load()
函数可以将JSON文件内容解析为Python对象,示例代码如下:
import json
with open('文件名.json', 'r', encoding='utf-8') as file:
data = json.load(file)
print(data)
这种方式适合处理复杂的数据结构,能够方便地进行数据分析与操作。
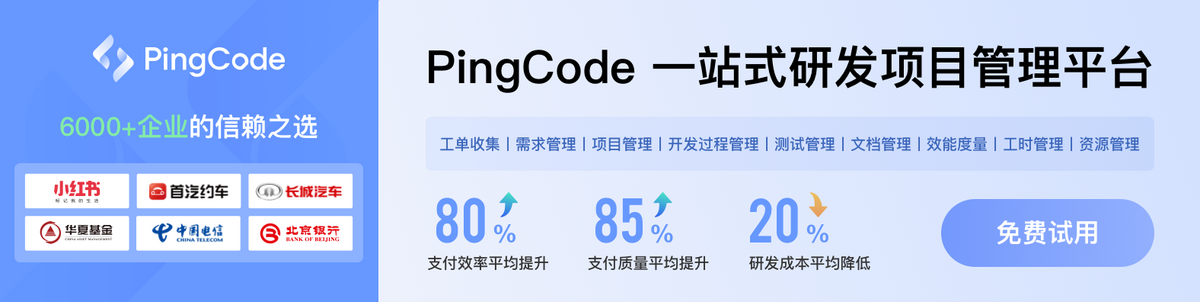