用Python统计单词数的方法包括使用字符串方法、使用正则表达式、使用collections.Counter模块、使用NLTK库等。以下是详细描述其中一种方法——使用字符串方法来统计单词数:
使用字符串方法统计单词数是最简单也是最基础的方法。首先,你需要读取文本内容,然后可以使用字符串的split()
方法将文本切割成单词列表,最后统计列表的长度即可得到单词的总数。下面是一个示例代码:
def count_words(text):
words = text.split()
return len(words)
text = "This is a sample text with several words."
print("Number of words:", count_words(text))
在上面的代码中,split()
方法会自动以空白字符(空格、换行、制表符等)为分隔符,将字符串分割成单词列表。然后使用len()
函数计算列表的长度,即为单词总数。
一、使用字符串方法统计单词数
1. 基本方法:split()方法
如前所述,split()
方法是最基础的字符串方法之一。它能够将字符串按照指定的分隔符(默认为空白字符)分割成多个部分,返回一个列表。通过计算这个列表的长度,我们可以轻松得到文本中的单词数。以下是一个更详细的示例:
def count_words(text):
words = text.split()
return len(words)
text = "Hello, world! Welcome to the world of Python."
word_count = count_words(text)
print(f"Number of words: {word_count}")
在这个示例中,split()
方法将字符串分割成了多个单词,并存储在列表words
中。len(words)
返回列表的长度,即文本中的单词数。
2. 处理标点符号
在实际应用中,标点符号会影响单词统计的准确性。为了提高准确性,我们需要移除标点符号。可以使用str.translate()
方法配合str.maketrans()
方法来移除标点符号:
import string
def count_words(text):
translator = str.maketrans('', '', string.punctuation)
text = text.translate(translator)
words = text.split()
return len(words)
text = "Hello, world! Welcome to the world of Python."
word_count = count_words(text)
print(f"Number of words: {word_count}")
在这个示例中,我们使用str.maketrans()
方法创建了一个翻译表,将所有标点符号映射为空字符。然后使用translate()
方法移除了文本中的所有标点符号,再使用split()
方法将文本分割成单词列表。
二、使用正则表达式统计单词数
1. 基本方法:re.findall()
正则表达式是一种强大的字符串处理工具,能够通过复杂的模式匹配来提取文本中的单词。Python的re
模块提供了正则表达式的支持。我们可以使用re.findall()
方法来查找所有的单词:
import re
def count_words(text):
words = re.findall(r'\b\w+\b', text)
return len(words)
text = "Hello, world! Welcome to the world of Python."
word_count = count_words(text)
print(f"Number of words: {word_count}")
在这个示例中,正则表达式\b\w+\b
匹配所有的单词边界和单词字符(字母、数字和下划线),re.findall()
方法返回所有匹配的单词列表。最后使用len()
函数计算列表的长度,即为单词总数。
2. 处理不同语言和字符集
正则表达式还可以处理不同的语言和字符集。通过使用Unicode字符集,我们可以匹配多种语言的单词。例如,匹配中文单词的正则表达式可以是:
import re
def count_words(text):
words = re.findall(r'[\u4e00-\u9fff]+', text)
return len(words)
text = "你好,世界!欢迎来到Python的世界。"
word_count = count_words(text)
print(f"Number of words: {word_count}")
在这个示例中,正则表达式[\u4e00-\u9fff]+
匹配所有的中文字符,re.findall()
方法返回所有匹配的中文单词列表。最后使用len()
函数计算列表的长度,即为单词总数。
三、使用collections.Counter模块统计单词数
1. 基本方法:collections.Counter
collections
模块中的Counter
类是一个方便的工具,可以用来统计单词的频率。我们可以结合split()
方法和Counter
类来统计单词数:
from collections import Counter
def count_words(text):
words = text.split()
word_count = Counter(words)
return word_count
text = "Hello, world! Welcome to the world of Python."
word_count = count_words(text)
print(f"Number of words: {sum(word_count.values())}")
print(f"Word frequencies: {word_count}")
在这个示例中,Counter(words)
创建了一个单词频率计数器,sum(word_count.values())
返回所有单词的总数,word_count
包含了每个单词的频率。
2. 处理标点符号和忽略大小写
为了提高统计的准确性,我们可以移除标点符号并将所有单词转换为小写字母:
import string
from collections import Counter
def count_words(text):
translator = str.maketrans('', '', string.punctuation)
text = text.translate(translator).lower()
words = text.split()
word_count = Counter(words)
return word_count
text = "Hello, world! Welcome to the world of Python."
word_count = count_words(text)
print(f"Number of words: {sum(word_count.values())}")
print(f"Word frequencies: {word_count}")
在这个示例中,我们移除了文本中的标点符号,并将所有单词转换为小写字母,以确保统计结果的准确性。
四、使用NLTK库统计单词数
1. 基本方法:NLTK库
NLTK(Natural Language Toolkit)是一个强大的自然语言处理库,提供了丰富的文本处理功能。我们可以使用NLTK库来统计单词数:
import nltk
from nltk.tokenize import word_tokenize
下载需要的数据
nltk.download('punkt')
def count_words(text):
words = word_tokenize(text)
return len(words)
text = "Hello, world! Welcome to the world of Python."
word_count = count_words(text)
print(f"Number of words: {word_count}")
在这个示例中,word_tokenize()
方法使用NLTK的分词器将文本分割成单词列表。len(words)
返回列表的长度,即为单词总数。
2. 处理标点符号和忽略大小写
NLTK库能够自动处理标点符号,但如果需要忽略大小写,可以手动将单词转换为小写字母:
import nltk
from nltk.tokenize import word_tokenize
下载需要的数据
nltk.download('punkt')
def count_words(text):
words = word_tokenize(text)
words = [word.lower() for word in words if word.isalnum()]
return len(words)
text = "Hello, world! Welcome to the world of Python."
word_count = count_words(text)
print(f"Number of words: {word_count}")
在这个示例中,我们使用列表推导式将所有单词转换为小写字母,并且只保留字母和数字的单词,以确保统计结果的准确性。
五、处理大文件和多线程处理
1. 处理大文件
对于大文件,逐行读取和处理文本可以显著提高效率。以下是逐行读取大文件并统计单词数的示例:
def count_words_in_file(file_path):
word_count = 0
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
words = line.split()
word_count += len(words)
return word_count
file_path = 'large_text_file.txt'
word_count = count_words_in_file(file_path)
print(f"Number of words in file: {word_count}")
在这个示例中,我们逐行读取文件内容,并使用split()
方法将每行文本分割成单词列表,逐步累加单词数。
2. 多线程处理
对于非常大的文件或需要处理多个文件的情况,可以考虑使用多线程来提高处理速度。以下是一个使用多线程统计多个文件单词数的示例:
import threading
def count_words_in_file(file_path):
word_count = 0
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
words = line.split()
word_count += len(words)
return word_count
def count_words_in_files(file_paths):
total_word_count = 0
threads = []
results = [0] * len(file_paths)
def worker(file_path, index):
results[index] = count_words_in_file(file_path)
for i, file_path in enumerate(file_paths):
thread = threading.Thread(target=worker, args=(file_path, i))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
total_word_count = sum(results)
return total_word_count
file_paths = ['file1.txt', 'file2.txt', 'file3.txt']
total_word_count = count_words_in_files(file_paths)
print(f"Total number of words in files: {total_word_count}")
在这个示例中,我们使用threading
模块创建多个线程,每个线程处理一个文件,并将结果存储在results
列表中。所有线程完成后,计算所有文件的总单词数。
六、总结
用Python统计单词数的方法有很多,主要包括使用字符串方法、使用正则表达式、使用collections.Counter模块、使用NLTK库等。每种方法都有其优缺点和适用场景:
- 字符串方法:简单易用,适合处理小规模文本。
- 正则表达式:灵活强大,适合处理复杂的文本模式。
- collections.Counter:方便统计单词频率,适合分析单词分布。
- NLTK库:功能强大,适合自然语言处理。
根据具体需求选择合适的方法,可以提高统计单词数的效率和准确性。在处理大文件或多个文件时,可以考虑逐行读取和多线程处理,以提高处理速度。
相关问答FAQs:
如何使用Python读取文本文件并统计单词数?
要读取文本文件并统计单词数,可以使用Python的内置函数和字符串方法。首先,使用open()
函数打开文件并读取内容,然后使用split()
方法将文本分割为单词,最后使用len()
函数计算单词的数量。以下是一个示例代码:
with open('yourfile.txt', 'r') as file:
text = file.read()
word_count = len(text.split())
print(f'The number of words is: {word_count}')
这个方法简单易懂,可以有效统计文本文件中的单词数量。
在Python中如何处理标点符号以确保准确的单词统计?
统计单词时,标点符号可能会影响结果。为了提高统计的准确性,可以使用re
模块的正则表达式来替换或去除标点符号。例如:
import re
with open('yourfile.txt', 'r') as file:
text = file.read()
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
word_count = len(text.split())
print(f'The number of words is: {word_count}')
通过这种方式,标点符号不会影响到单词的计数。
使用Python统计单词数时,有哪些常见的错误需要避免?
在进行单词统计时,常见的错误包括未考虑大小写的差异、未处理的空白字符以及标点符号的干扰。为确保统计的准确性,可以采取以下措施:
- 将文本转换为小写形式,以避免大小写导致的重复计数。
- 使用
strip()
方法去除单词前后的空白字符。 - 正确处理标点符号,确保它们不会与单词混淆。
通过遵循这些原则,可以提高统计的准确性。
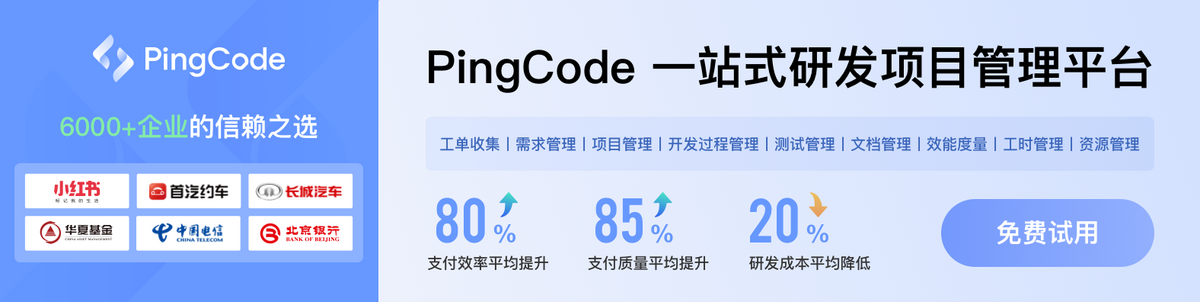