Python实现批量清理日志的方法有:使用os模块遍历目录、使用glob模块查找符合条件的文件、使用shutil模块删除文件、设置清理策略等。 其中,使用os模块遍历目录是最基础和最常用的方法之一。
一、使用os模块遍历目录
os模块提供了丰富的目录操作方法,例如os.listdir()可以列出指定目录下的所有文件和子目录,os.path.join()可以生成路径,os.remove()可以删除文件等。下面是一个示例代码,演示了如何使用os模块遍历目录并删除指定目录下的所有日志文件。
import os
def clean_logs(directory):
for filename in os.listdir(directory):
file_path = os.path.join(directory, filename)
try:
if os.path.isfile(file_path) and file_path.endswith('.log'):
os.remove(file_path)
print(f'Removed file: {file_path}')
except Exception as e:
print(f'Error while deleting file {file_path}. Reason: {e}')
使用示例
log_directory = '/path/to/logs'
clean_logs(log_directory)
二、使用glob模块查找符合条件的文件
glob模块可以使用通配符查找文件,特别适合用于查找符合特定命名模式的文件。下面是一个示例代码,演示了如何使用glob模块查找并删除指定目录下的所有日志文件。
import glob
import os
def clean_logs(directory):
for file_path in glob.glob(os.path.join(directory, '*.log')):
try:
os.remove(file_path)
print(f'Removed file: {file_path}')
except Exception as e:
print(f'Error while deleting file {file_path}. Reason: {e}')
使用示例
log_directory = '/path/to/logs'
clean_logs(log_directory)
三、使用shutil模块删除文件
shutil模块提供了高级的文件操作功能,例如shutil.rmtree()可以递归删除目录及其内容。下面是一个示例代码,演示了如何使用shutil模块递归删除指定目录及其所有内容。
import shutil
import os
def clean_logs(directory):
try:
shutil.rmtree(directory)
print(f'Removed directory and its contents: {directory}')
except Exception as e:
print(f'Error while deleting directory {directory}. Reason: {e}')
使用示例
log_directory = '/path/to/logs'
clean_logs(log_directory)
四、设置清理策略
在实际应用中,可能需要根据不同的清理策略(例如文件大小、文件创建时间、文件修改时间等)来清理日志文件。下面是一个示例代码,演示了如何根据文件修改时间清理指定目录下超过一定天数的日志文件。
import os
import time
def clean_logs(directory, days):
current_time = time.time()
for filename in os.listdir(directory):
file_path = os.path.join(directory, filename)
if os.path.isfile(file_path) and file_path.endswith('.log'):
file_mtime = os.path.getmtime(file_path)
if (current_time - file_mtime) // (24 * 3600) >= days:
try:
os.remove(file_path)
print(f'Removed file: {file_path}')
except Exception as e:
print(f'Error while deleting file {file_path}. Reason: {e}')
使用示例
log_directory = '/path/to/logs'
days_to_keep = 30 # 保留最近30天的日志
clean_logs(log_directory, days_to_keep)
五、结合多种方法进行综合处理
在实际项目中,可能需要结合多种方法进行综合处理,例如先使用glob模块查找符合条件的文件,再根据文件大小或修改时间等属性进行筛选,最后使用os或shutil模块删除文件。下面是一个示例代码,演示了如何结合glob和os模块清理超过一定大小的日志文件。
import glob
import os
def clean_logs(directory, max_size):
for file_path in glob.glob(os.path.join(directory, '*.log')):
try:
if os.path.getsize(file_path) > max_size:
os.remove(file_path)
print(f'Removed file: {file_path}')
except Exception as e:
print(f'Error while deleting file {file_path}. Reason: {e}')
使用示例
log_directory = '/path/to/logs'
max_file_size = 10 * 1024 * 1024 # 删除超过10MB的日志文件
clean_logs(log_directory, max_file_size)
六、日志清理脚本的自动化
为了方便管理和使用,通常会将日志清理脚本配置为定时任务(例如cron任务)自动执行。下面是一个示例代码,演示了如何使用Python的schedule
库配置一个每天凌晨3点执行的日志清理任务。
import schedule
import time
import os
def clean_logs(directory, days):
current_time = time.time()
for filename in os.listdir(directory):
file_path = os.path.join(directory, filename)
if os.path.isfile(file_path) and file_path.endswith('.log'):
file_mtime = os.path.getmtime(file_path)
if (current_time - file_mtime) // (24 * 3600) >= days:
try:
os.remove(file_path)
print(f'Removed file: {file_path}')
except Exception as e:
print(f'Error while deleting file {file_path}. Reason: {e}')
def job():
log_directory = '/path/to/logs'
days_to_keep = 30 # 保留最近30天的日志
clean_logs(log_directory, days_to_keep)
配置每天凌晨3点执行一次
schedule.every().day.at("03:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
七、提高日志清理效率
在处理大量日志文件时,可能需要提高日志清理的效率。可以考虑以下几种方法:
- 多线程或多进程处理:使用
concurrent.futures
模块或multiprocessing
模块实现并行处理,提高清理速度。 - 分批处理:将日志文件分成多个批次,每个批次处理一部分文件,减少单次处理的负担。
- 异步IO处理:使用
asyncio
模块实现异步IO处理,提高文件操作的效率。
下面是一个示例代码,演示了如何使用concurrent.futures
模块实现多线程日志清理。
import os
import concurrent.futures
def clean_log(file_path):
try:
os.remove(file_path)
print(f'Removed file: {file_path}')
except Exception as e:
print(f'Error while deleting file {file_path}. Reason: {e}')
def clean_logs(directory):
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = []
for filename in os.listdir(directory):
file_path = os.path.join(directory, filename)
if os.path.isfile(file_path) and file_path.endswith('.log'):
futures.append(executor.submit(clean_log, file_path))
for future in concurrent.futures.as_completed(futures):
future.result()
使用示例
log_directory = '/path/to/logs'
clean_logs(log_directory)
八、日志清理的最佳实践
在实际应用中,可以遵循以下最佳实践来管理和清理日志文件:
- 定期清理:根据日志产生的频率和重要性,定期清理日志文件,防止日志文件占用过多磁盘空间。
- 设置日志轮转:使用日志轮转工具(例如logrotate)自动管理日志文件的创建、归档和删除,减少手动清理的负担。
- 压缩归档:对于需要长期保存的日志文件,可以定期将其压缩归档,减少磁盘空间的占用。
- 监控和报警:配置监控工具(例如Prometheus、Nagios等)监控日志文件的大小和数量,及时发现异常情况并报警。
- 安全删除:对于敏感日志文件,使用安全删除工具(例如shred)彻底删除文件,防止数据恢复。
九、日志清理的案例分析
在实际项目中,不同的应用场景对日志清理有不同的需求。下面分析几个常见的案例。
案例一:Web服务器日志清理
Web服务器(例如Nginx、Apache)通常会产生大量访问日志和错误日志。为了保证服务器的稳定运行,需要定期清理日志文件。可以使用日志轮转工具(例如logrotate)结合自定义清理脚本,实现自动清理和归档。
案例二:数据库日志清理
数据库系统(例如MySQL、PostgreSQL)通常会产生大量的查询日志和错误日志。为了保证数据库的性能和数据完整性,需要定期清理日志文件。可以使用数据库自带的日志管理工具(例如MySQL的logrotate),结合自定义清理脚本,实现自动清理和归档。
案例三:应用程序日志清理
应用程序(例如Java、Python应用)通常会产生业务日志和错误日志。为了保证应用程序的稳定运行和日志的可追溯性,需要定期清理日志文件。可以使用日志轮转库(例如Python的logging库),结合自定义清理脚本,实现自动清理和归档。
十、日志清理的未来发展
随着云计算和大数据技术的发展,日志清理面临新的挑战和机遇。可以预见,未来日志清理将朝着以下几个方向发展:
- 智能化清理:结合机器学习和人工智能技术,实现日志清理的智能化和自动化,根据日志数据的特征自动调整清理策略。
- 分布式清理:随着分布式系统的普及,日志数据的分布式存储和处理成为趋势。未来日志清理将更加注重分布式环境下的高效清理。
- 实时清理:结合流处理技术,实现日志数据的实时清理和分析,提高日志管理的实时性和准确性。
- 安全清理:随着数据隐私和安全法规的加强,日志清理将更加注重数据的安全性和合规性,确保日志数据的安全删除和合规管理。
总之,Python提供了丰富的工具和方法,可以高效地实现批量清理日志。通过合理选择和组合这些工具和方法,可以满足不同应用场景的日志清理需求,保证系统的稳定运行和日志数据的可追溯性。
相关问答FAQs:
如何使用Python自动化清理日志文件的过程?
Python提供了多种库和方法来自动化日志文件的清理。例如,可以使用os
和glob
库来查找并删除特定目录下的旧日志文件。通过编写一个简单的脚本,可以设定清理的时间范围,例如删除超过30天的日志文件。此外,结合datetime
模块,可以方便地获取当前日期并与文件的最后修改时间进行比较,从而决定是否删除。
在清理日志时,有哪些最佳实践需要遵循?
清理日志时应注意几个最佳实践。首先,确保不删除正在使用的日志文件,以免影响系统运行。其次,建议在清理之前备份重要的日志数据,以防需要后续审查。还可以考虑使用压缩文件的方式存档旧日志,减小存储占用,同时保留必要的数据。最后,定期设置清理任务,可以通过cron作业或Windows任务调度器来自动执行。
是否可以通过Python实现日志清理的通知功能?
可以通过Python实现日志清理的通知功能。可以使用logging
模块记录清理操作的详细信息,并将其输出到控制台或另一个日志文件。此外,还可以利用smptlib
模块发送电子邮件通知,告知相关人员清理操作已完成并提供清理的详细记录。这样不仅提高了日志管理的透明度,还能有效提升团队的协作效率。
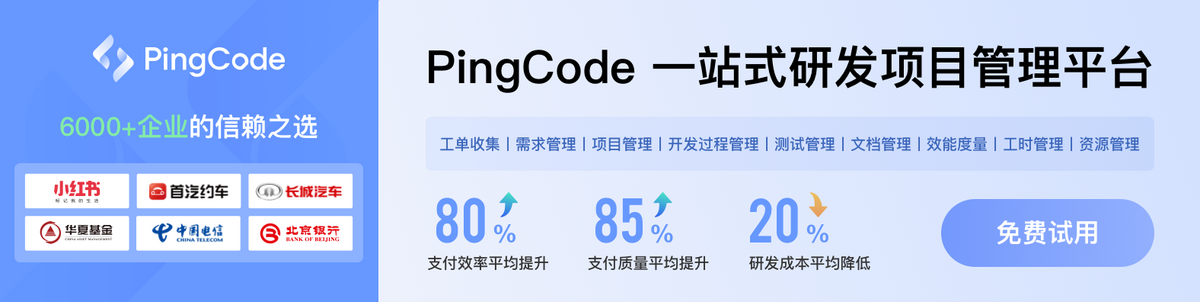