Python判断程序的书写主要依赖于条件语句,如if、elif和else。这些语句可以帮助程序在满足特定条件时执行不同的代码块。
示例如下:
- 使用if语句,判断一个变量是否满足某个条件。
- 使用elif语句,增加其他条件判断。
- 使用else语句,处理所有不满足前面条件的情况。
下面将详细描述如何编写一个Python判断程序,并探讨一些常见的应用场景和注意事项。
一、基础语法
1、if语句
if语句用于在条件为真时执行特定代码块。它的基本语法如下:
if condition:
# code to execute if condition is true
例子:
a = 10
if a > 5:
print("a is greater than 5")
在这个例子中,如果变量a的值大于5,程序将输出“a is greater than 5”。
2、elif语句
elif是“else if”的缩写,用于检查另一个条件。它的基本语法如下:
if condition1:
# code to execute if condition1 is true
elif condition2:
# code to execute if condition2 is true
例子:
a = 10
if a > 10:
print("a is greater than 10")
elif a == 10:
print("a is equal to 10")
在这个例子中,如果变量a的值等于10,程序将输出“a is equal to 10”。
3、else语句
else语句用于处理所有不满足前面条件的情况。它的基本语法如下:
if condition1:
# code to execute if condition1 is true
elif condition2:
# code to execute if condition2 is true
else:
# code to execute if neither condition1 nor condition2 is true
例子:
a = 10
if a > 10:
print("a is greater than 10")
elif a == 10:
print("a is equal to 10")
else:
print("a is less than 10")
在这个例子中,如果变量a的值既不大于10也不等于10,程序将输出“a is less than 10”。
二、逻辑运算符
在条件语句中,逻辑运算符可以用于组合多个条件。主要的逻辑运算符包括and、or和not。
1、and运算符
and运算符用于检查多个条件都为真时的情况。
if condition1 and condition2:
# code to execute if both condition1 and condition2 are true
例子:
a = 10
b = 20
if a > 5 and b > 15:
print("a is greater than 5 and b is greater than 15")
2、or运算符
or运算符用于检查至少一个条件为真时的情况。
if condition1 or condition2:
# code to execute if either condition1 or condition2 is true
例子:
a = 10
b = 5
if a > 5 or b > 15:
print("a is greater than 5 or b is greater than 15")
3、not运算符
not运算符用于取反一个条件。
if not condition:
# code to execute if condition is false
例子:
a = 10
if not a < 5:
print("a is not less than 5")
三、常见应用场景
1、判断用户输入
判断用户输入是Python判断程序的一个常见应用场景。以下是一个简单的例子:
user_input = input("Please enter a number: ")
if user_input.isdigit():
number = int(user_input)
if number > 0:
print("The number is positive")
elif number < 0:
print("The number is negative")
else:
print("The number is zero")
else:
print("That's not a valid number")
在这个例子中,程序首先检查用户输入是否为数字,然后根据输入的数字判断其正负。
2、判断列表或字典中的元素
Python判断程序还可以用于判断列表或字典中的元素。
例子:
my_list = [1, 2, 3, 4, 5]
my_dict = {'a': 1, 'b': 2, 'c': 3}
if 3 in my_list:
print("3 is in the list")
if 'b' in my_dict:
print("b is a key in the dictionary")
if 2 in my_dict.values():
print("2 is a value in the dictionary")
3、判断文件是否存在
在处理文件操作时,判断文件是否存在也是一个常见的应用场景。
例子:
import os
file_path = "example.txt"
if os.path.exists(file_path):
print("The file exists")
else:
print("The file does not exist")
四、嵌套条件语句
在某些情况下,你可能需要在一个条件语句中嵌套另一个条件语句。
例子:
a = 10
b = 5
if a > 0:
if b > 0:
print("Both a and b are positive")
else:
print("a is positive, but b is not")
else:
print("a is not positive")
在这个例子中,程序首先检查变量a是否为正,然后在a为正的情况下进一步检查变量b是否为正。
五、使用三元运算符
Python提供了一种简洁的方式来编写简单的条件语句,称为三元运算符。
例子:
a = 10
b = 5
result = "a is greater than b" if a > b else "a is not greater than b"
print(result)
在这个例子中,三元运算符用于根据条件选择一个值,并将结果赋值给变量result。
六、错误处理与异常捕获
在编写判断程序时,处理潜在的错误和异常也是非常重要的。
例子:
try:
user_input = input("Please enter a number: ")
number = int(user_input)
if number > 0:
print("The number is positive")
elif number < 0:
print("The number is negative")
else:
print("The number is zero")
except ValueError:
print("That's not a valid number")
在这个例子中,程序使用try-except块来捕获ValueError异常,以处理用户输入的无效数字。
七、优化与性能考虑
在编写判断程序时,优化和性能考虑也是非常重要的。在某些情况下,可以通过提前退出或者减少不必要的计算来提高程序的性能。
例子:
def is_prime(n):
if n <= 1:
return False
if n <= 3:
return True
if n % 2 == 0 or n % 3 == 0:
return False
i = 5
while i * i <= n:
if n % i == 0 or n % (i + 2) == 0:
return False
i += 6
return True
number = 29
if is_prime(number):
print(f"{number} is a prime number")
else:
print(f"{number} is not a prime number")
在这个例子中,is_prime函数使用了一些优化技巧,如提前退出和减少不必要的计算,以提高判断素数的效率。
八、总结
Python判断程序的书写主要依赖于if、elif和else语句,通过结合逻辑运算符、嵌套条件语句和三元运算符,可以实现复杂的判断逻辑。在实际应用中,判断用户输入、列表或字典中的元素、文件是否存在等都是常见的应用场景。此外,处理潜在的错误和异常、优化和性能考虑也是编写高效Python判断程序的重要方面。通过掌握这些技巧和实践经验,你可以编写出更加健壮和高效的Python判断程序。
相关问答FAQs:
如何在Python中实现条件判断?
在Python中,条件判断通常使用if
语句。基本的语法结构为:
if condition:
# 执行的代码
elif another_condition:
# 执行的代码
else:
# 执行的代码
通过这种方式,可以根据不同的条件执行不同的代码块。条件可以是任何返回布尔值(True或False)的表达式。
Python中可以使用哪些逻辑运算符进行判断?
在Python中,常用的逻辑运算符包括and
、or
和not
。这些运算符可以组合多个条件,以进行更复杂的判断。例如:
if condition1 and condition2:
# 两个条件都为真时执行的代码
这种灵活性使得程序能够根据不同的输入情况做出相应的处理。
在Python中如何处理嵌套判断?
嵌套判断是指在一个if
语句内部再包含另一个if
语句。这种方式可以处理更复杂的逻辑。例如:
if condition1:
if condition2:
# condition1和condition2都为真时执行的代码
else:
# condition1为真,但condition2为假时执行的代码
else:
# condition1为假时执行的代码
这种结构能够精确地控制程序的执行流程,适用于多层次的条件判断。
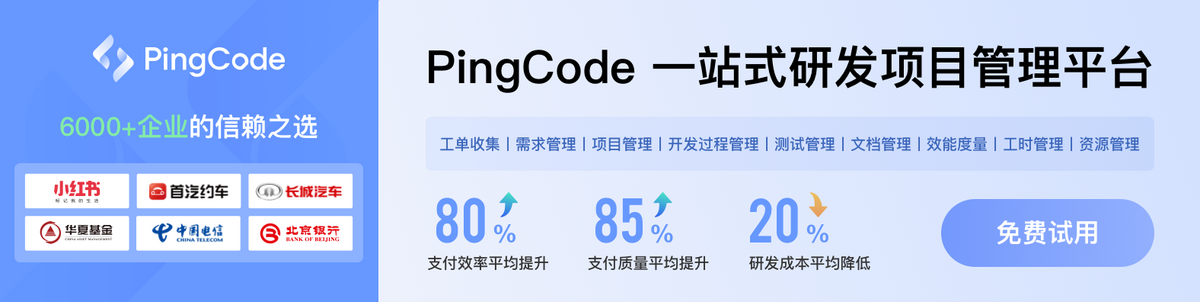