要使用Python提取邮件内容,可以使用Python的内置库email和imaplib、使用第三方库如IMAPClient和BeautifulSoup。
一、使用 IMAP 和 Email 模块
1.1、连接到邮件服务器
首先,你需要连接到电子邮件服务器。使用IMAP协议与邮件服务器通信。以下是一个示例代码,展示如何连接到Gmail服务器:
import imaplib
连接到Gmail的IMAP服务器
mail = imaplib.IMAP4_SSL('imap.gmail.com')
mail.login('your_email@gmail.com', 'your_password')
1.2、选择邮箱文件夹
选择你要操作的邮箱文件夹,例如收件箱:
mail.select('inbox')
1.3、搜索邮件
使用IMAP的搜索功能来查找特定的邮件。以下示例代码展示如何查找所有未读邮件:
status, messages = mail.search(None, 'UNSEEN')
1.4、获取邮件内容
获取邮件的唯一标识符后,可以通过ID获取邮件内容:
for num in messages[0].split():
status, data = mail.fetch(num, '(RFC822)')
raw_email = data[0][1]
print(raw_email)
1.5、解析邮件内容
使用email模块解析邮件内容:
import email
msg = email.message_from_bytes(raw_email)
for part in msg.walk():
if part.get_content_type() == "text/plain":
body = part.get_payload(decode=True)
print(body.decode())
二、使用 IMAPClient 和 BeautifulSoup
2.1、安装依赖库
首先,需要安装IMAPClient和BeautifulSoup:
pip install imapclient
pip install beautifulsoup4
2.2、连接到邮件服务器
使用IMAPClient库连接到邮件服务器:
from imapclient import IMAPClient
server = IMAPClient('imap.gmail.com', use_uid=True)
server.login('your_email@gmail.com', 'your_password')
2.3、选择邮箱文件夹
选择要操作的邮箱文件夹:
server.select_folder('INBOX')
2.4、搜索邮件
搜索特定条件的邮件,例如未读邮件:
messages = server.search(['UNSEEN'])
2.5、获取邮件内容
获取邮件的唯一标识符后,可以通过ID获取邮件内容:
response = server.fetch(messages, ['RFC822'])
2.6、解析邮件内容
使用email和BeautifulSoup解析邮件内容:
from bs4 import BeautifulSoup
import email
for msgid, data in response.items():
msg = email.message_from_bytes(data[b'RFC822'])
for part in msg.walk():
if part.get_content_type() == 'text/plain':
body = part.get_payload(decode=True)
print(body.decode())
elif part.get_content_type() == 'text/html':
html = part.get_payload(decode=True)
soup = BeautifulSoup(html, 'html.parser')
text = soup.get_text()
print(text)
三、处理附件
3.1、检查附件
在解析邮件内容时,可以检查是否有附件:
for part in msg.walk():
if part.get_content_disposition() is not None:
if 'attachment' in part.get_content_disposition():
# 处理附件
3.2、保存附件
将附件保存到本地:
import os
for part in msg.walk():
if part.get_content_disposition() is not None:
if 'attachment' in part.get_content_disposition():
filename = part.get_filename()
if filename:
filepath = os.path.join('/path/to/save/attachments', filename)
with open(filepath, 'wb') as f:
f.write(part.get_payload(decode=True))
四、处理邮件中的多部分内容
4.1、处理多部分邮件
有些邮件是多部分的,包含文本和HTML内容。可以分别处理每一部分:
for part in msg.walk():
content_type = part.get_content_type()
content_disposition = part.get_content_disposition()
if content_type == 'text/plain' and content_disposition is None:
# 处理纯文本部分
elif content_type == 'text/html' and content_disposition is None:
# 处理HTML部分
4.2、优先处理文本内容
在多部分邮件中,优先处理纯文本内容,如果没有纯文本内容,再处理HTML内容:
text_content = None
html_content = None
for part in msg.walk():
content_type = part.get_content_type()
if content_type == 'text/plain' and text_content is None:
text_content = part.get_payload(decode=True).decode()
elif content_type == 'text/html' and html_content is None:
html_content = part.get_payload(decode=True).decode()
if text_content:
print(text_content)
elif html_content:
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.get_text())
五、处理不同编码的邮件
5.1、检查邮件编码
有些邮件使用不同的字符编码,可以检查邮件的字符编码并进行相应处理:
for part in msg.walk():
charset = part.get_content_charset()
if charset:
body = part.get_payload(decode=True).decode(charset)
else:
body = part.get_payload(decode=True).decode()
print(body)
5.2、处理常见编码
常见编码包括UTF-8、ISO-8859-1等,可以根据邮件的编码进行相应的解码:
for part in msg.walk():
charset = part.get_content_charset()
if charset:
try:
body = part.get_payload(decode=True).decode(charset)
except (UnicodeDecodeError, LookupError):
body = part.get_payload(decode=True).decode('utf-8', errors='replace')
else:
body = part.get_payload(decode=True).decode()
print(body)
六、异常处理和错误处理
6.1、处理连接错误
在连接到邮件服务器时,可能会遇到网络问题或认证错误,需要进行异常处理:
import imaplib
try:
mail = imaplib.IMAP4_SSL('imap.gmail.com')
mail.login('your_email@gmail.com', 'your_password')
except imaplib.IMAP4.error as e:
print(f'Failed to connect or authenticate: {e}')
6.2、处理邮件解析错误
在解析邮件内容时,可能会遇到解析错误或邮件格式不标准的问题,需要进行异常处理:
import email
try:
msg = email.message_from_bytes(raw_email)
for part in msg.walk():
if part.get_content_type() == "text/plain":
body = part.get_payload(decode=True).decode()
print(body)
except Exception as e:
print(f'Failed to parse email: {e}')
6.3、处理附件下载错误
在下载和保存附件时,可能会遇到文件写入错误或网络中断等问题,需要进行异常处理:
import os
try:
for part in msg.walk():
if part.get_content_disposition() is not None:
if 'attachment' in part.get_content_disposition():
filename = part.get_filename()
if filename:
filepath = os.path.join('/path/to/save/attachments', filename)
with open(filepath, 'wb') as f:
f.write(part.get_payload(decode=True))
except Exception as e:
print(f'Failed to save attachment: {e}')
七、使用OAuth2进行认证
7.1、获取OAuth2令牌
对于一些邮件服务提供商如Gmail,可以使用OAuth2进行认证。首先,获取OAuth2令牌:
import google.auth
import google.auth.transport.requests
credentials, project = google.auth.default()
request = google.auth.transport.requests.Request()
credentials.refresh(request)
token = credentials.token
7.2、使用OAuth2令牌进行认证
使用获取的OAuth2令牌进行IMAP认证:
import imaplib
mail = imaplib.IMAP4_SSL('imap.gmail.com')
mail.authenticate('XOAUTH2', lambda x: f'user=your_email@gmail.com\1auth=Bearer {token}\1\1')
八、自动化脚本和定时任务
8.1、编写自动化脚本
将提取邮件内容的代码封装成一个自动化脚本,以便定期运行:
def extract_emails():
# 连接邮件服务器
mail = imaplib.IMAP4_SSL('imap.gmail.com')
mail.login('your_email@gmail.com', 'your_password')
# 选择邮箱文件夹
mail.select('inbox')
# 搜索邮件
status, messages = mail.search(None, 'UNSEEN')
# 获取邮件内容
for num in messages[0].split():
status, data = mail.fetch(num, '(RFC822)')
raw_email = data[0][1]
# 解析邮件内容
msg = email.message_from_bytes(raw_email)
for part in msg.walk():
if part.get_content_type() == "text/plain":
body = part.get_payload(decode=True)
print(body.decode())
if __name__ == '__main__':
extract_emails()
8.2、设置定时任务
使用操作系统的定时任务功能,如cron(在Unix系统上)或Task Scheduler(在Windows系统上)设置定时任务,定期运行自动化脚本:
在Unix系统上使用cron:
crontab -e
在crontab文件中添加以下行,每小时运行一次脚本:
0 * * * * /usr/bin/python3 /path/to/your_script.py
在Windows系统上使用Task Scheduler:
- 打开Task Scheduler。
- 创建一个新的任务。
- 设置触发器为定时触发。
- 设置操作为运行Python脚本。
通过设置定时任务,自动化脚本可以定期提取邮件内容,确保及时获取所需信息。
九、总结
通过使用Python的IMAP和email模块、IMAPClient和BeautifulSoup库,可以轻松提取邮件内容并进行解析。处理邮件中的多部分内容、不同编码的邮件、附件下载等问题时,可以结合实际需求进行相应处理。同时,编写自动化脚本并设置定时任务,可以实现邮件内容的定期提取,确保信息的及时获取。希望本文对您在使用Python提取邮件内容时有所帮助。
相关问答FAQs:
如何使用Python提取特定格式的邮件内容?
要提取特定格式的邮件内容,可以使用Python的内置库如imaplib
和email
。首先,通过imaplib
连接到邮件服务器并登录账户。接着,使用email
库解析邮件内容,筛选出所需的字段,例如发件人、主题和正文。可以通过正则表达式或字符串处理函数来提取特定的信息。
在提取邮件内容时,如何处理附件文件?
处理邮件附件可以通过email
库中的get_payload()
方法获取邮件的内容部分。如果邮件包含附件,可以检查其Content-Disposition
字段,并将其保存到本地文件系统。确保处理不同类型的附件(如PDF、图片等),并使用合适的库来读取或解析这些文件。
使用Python提取邮件内容需要注意哪些安全性问题?
在提取邮件内容时,确保遵循安全最佳实践。避免在代码中硬编码邮箱密码,考虑使用环境变量或配置文件来存储敏感信息。同时,使用SSL/TLS加密连接到邮件服务器,以保护数据传输的安全性。对于处理的邮件内容,确保遵循隐私法规,防止泄露用户信息。
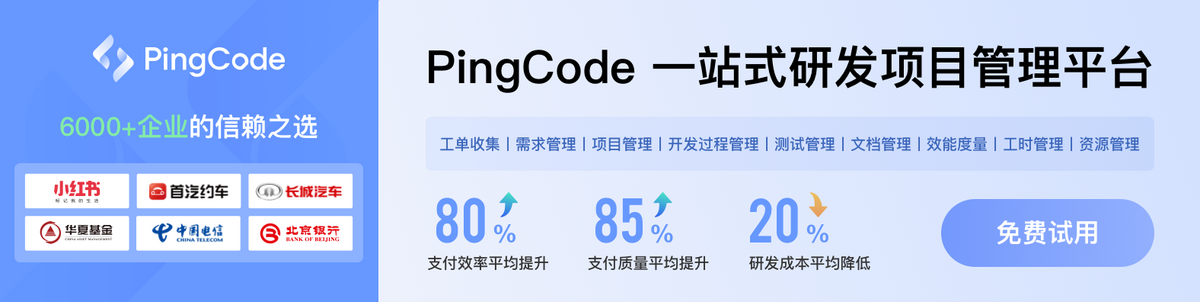