在Python中,多线程可以通过多种方式共享变量,使用全局变量、使用threading
模块中的Lock
对象、使用Queue
模块。其中,使用全局变量是最直接的方法,但需要注意线程安全问题。使用Lock
对象可以确保线程安全,但需要手动管理锁。使用Queue
模块则可以方便地在多线程间共享数据,并且内置了线程安全机制。
使用全局变量
全局变量是最简单的共享变量方式。只需要在多个线程之间访问同一个变量即可。然而,多线程访问同一个变量可能会导致竞争条件(race condition)问题,从而引发数据不一致的问题。
示例:
import threading
全局变量
shared_variable = 0
def increment():
global shared_variable
for _ in range(100000):
shared_variable += 1
threads = []
for i in range(10):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(shared_variable)
在上面的示例中,我们创建了10个线程,每个线程都对shared_variable
进行增量操作。由于没有使用锁,最终的结果可能不是预期的1000000。
使用threading
模块中的Lock
对象
为了确保线程安全,可以使用threading
模块中的Lock
对象。Lock
对象可以用来确保在同一时刻只有一个线程访问共享变量,从而避免竞争条件问题。
示例:
import threading
全局变量
shared_variable = 0
lock = threading.Lock()
def increment():
global shared_variable
for _ in range(100000):
lock.acquire()
shared_variable += 1
lock.release()
threads = []
for i in range(10):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(shared_variable)
在这个示例中,我们使用lock.acquire()
和lock.release()
来确保只有一个线程可以访问shared_variable
,从而避免了竞争条件问题。最终的结果将是预期的1000000。
使用Queue
模块
Queue
模块提供了一个线程安全的队列,可以方便地在多个线程之间共享数据。Queue
模块内部使用了锁和条件变量来确保线程安全。
示例:
import threading
import queue
创建队列
shared_queue = queue.Queue()
def producer():
for i in range(10):
shared_queue.put(i)
print(f"Produced {i}")
def consumer():
while not shared_queue.empty():
item = shared_queue.get()
print(f"Consumed {item}")
shared_queue.task_done()
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
producer_thread.join()
consumer_thread.join()
在这个示例中,我们创建了一个共享队列shared_queue
,并使用两个线程分别作为生产者和消费者。生产者线程将数据放入队列中,消费者线程从队列中取出数据。由于Queue
模块内部已经处理了线程安全问题,因此我们不需要手动管理锁。
多线程共享变量的注意事项
在多线程程序中共享变量时,需要注意以下几点:
- 线程安全:确保多个线程同时访问共享变量时不会引发数据不一致的问题。可以使用锁、队列或其他线程安全的数据结构来解决这个问题。
- 性能:锁的使用虽然可以确保线程安全,但也会引入额外的开销,从而影响程序性能。因此,需要在安全和性能之间找到平衡点。
- 死锁:在使用多个锁时,需要注意避免死锁问题。可以通过严格的锁顺序或使用其他同步原语(如条件变量)来避免死锁。
- 资源管理:确保在程序结束时正确释放所有资源,包括锁和队列。可以使用上下文管理器或确保在
finally
块中释放资源。
其他常见的线程同步机制
除了Lock
和Queue
,Python还提供了其他一些常见的线程同步机制,如RLock
、Semaphore
、Event
和Condition
等。这些同步机制可以帮助我们更灵活地处理多线程中的共享变量问题。
1. 使用RLock
RLock
(可重入锁)允许同一个线程多次获得同一个锁,而不会引发死锁问题。这在某些场景下非常有用。
示例:
import threading
shared_variable = 0
rlock = threading.RLock()
def increment():
global shared_variable
for _ in range(100000):
with rlock:
shared_variable += 1
threads = []
for i in range(10):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(shared_variable)
在这个示例中,我们使用with rlock
语句来确保线程安全。with
语句会自动获得和释放锁,从而简化了代码。
2. 使用Semaphore
Semaphore
(信号量)允许指定最多有多少个线程同时访问共享资源。这在需要控制并发线程数量时非常有用。
示例:
import threading
shared_variable = 0
semaphore = threading.Semaphore(5)
def increment():
global shared_variable
for _ in range(100000):
semaphore.acquire()
shared_variable += 1
semaphore.release()
threads = []
for i in range(10):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(shared_variable)
在这个示例中,我们使用semaphore.acquire()
和semaphore.release()
来控制最多有5个线程同时访问shared_variable
。
3. 使用Event
Event
对象允许一个线程等待另一个线程的通知。可以使用Event
对象来协调多个线程的执行顺序。
示例:
import threading
event = threading.Event()
def worker():
print("Worker waiting for event")
event.wait()
print("Worker received event")
thread = threading.Thread(target=worker)
thread.start()
print("Main thread setting event")
event.set()
thread.join()
在这个示例中,worker
线程会等待event
对象被设置。在主线程中,我们设置了event
对象,从而通知worker
线程继续执行。
4. 使用Condition
Condition
对象允许多个线程等待某个条件,并在条件满足时被唤醒。这在需要复杂的线程同步时非常有用。
示例:
import threading
condition = threading.Condition()
shared_variable = 0
def producer():
global shared_variable
with condition:
shared_variable += 1
print(f"Produced {shared_variable}")
condition.notify()
def consumer():
global shared_variable
with condition:
while shared_variable == 0:
condition.wait()
shared_variable -= 1
print(f"Consumed {shared_variable}")
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
consumer_thread.start()
producer_thread.start()
producer_thread.join()
consumer_thread.join()
在这个示例中,consumer
线程会等待condition
对象被通知。在producer
线程中,我们更新了shared_variable
并通知了condition
对象,从而唤醒了consumer
线程。
总结
在Python多线程中共享变量时,可以使用全局变量、threading
模块中的Lock
对象、Queue
模块以及其他同步机制(如RLock
、Semaphore
、Event
和Condition
)来确保线程安全。选择合适的同步机制可以有效地避免竞争条件、提高程序性能,并确保资源的正确管理。
通过理解和掌握这些同步机制,可以帮助我们编写更加健壮和高效的多线程程序。在实际开发中,需要根据具体的应用场景选择合适的同步机制,确保多线程程序的正确性和性能。
多线程共享变量的实际应用案例
为了更好地理解如何在实际应用中使用多线程共享变量,我们可以通过一些实际案例来演示这些同步机制的应用。
案例一:多线程下载器
假设我们需要编写一个多线程下载器,从多个URL中下载文件,并将下载进度和结果保存到共享变量中。我们可以使用Queue
模块来共享下载任务,并使用Lock
对象来确保下载进度的线程安全。
示例:
import threading
import queue
import requests
创建任务队列
task_queue = queue.Queue()
下载进度和结果
download_progress = 0
download_results = []
lock = threading.Lock()
def downloader():
global download_progress
while not task_queue.empty():
url = task_queue.get()
try:
response = requests.get(url)
lock.acquire()
download_progress += 1
download_results.append((url, response.status_code))
lock.release()
except Exception as e:
lock.acquire()
download_progress += 1
download_results.append((url, str(e)))
lock.release()
task_queue.task_done()
添加下载任务
urls = [
"https://example.com/file1",
"https://example.com/file2",
"https://example.com/file3",
]
for url in urls:
task_queue.put(url)
创建并启动下载线程
threads = []
for _ in range(3):
thread = threading.Thread(target=downloader)
threads.append(thread)
thread.start()
等待所有任务完成
for thread in threads:
thread.join()
输出下载结果
print(f"Download progress: {download_progress}/{len(urls)}")
print("Download results:")
for result in download_results:
print(result)
在这个示例中,我们创建了一个任务队列task_queue
,并将下载任务放入队列中。每个下载线程从队列中获取任务,进行下载,并更新下载进度和结果。通过使用lock
对象,我们确保了下载进度和结果的线程安全。
案例二:多线程爬虫
假设我们需要编写一个多线程爬虫,从多个起始URL开始爬取网页,并提取其中的链接进行进一步爬取。我们可以使用Queue
模块来共享爬取任务,并使用Lock
对象来确保共享数据的线程安全。
示例:
import threading
import queue
import requests
from bs4 import BeautifulSoup
创建任务队列
task_queue = queue.Queue()
爬取进度和结果
crawled_urls = set()
crawled_results = []
lock = threading.Lock()
def crawler():
while not task_queue.empty():
url = task_queue.get()
try:
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
links = soup.find_all("a", href=True)
lock.acquire()
crawled_urls.add(url)
crawled_results.append((url, response.status_code))
for link in links:
href = link["href"]
if href not in crawled_urls:
task_queue.put(href)
lock.release()
except Exception as e:
lock.acquire()
crawled_results.append((url, str(e)))
lock.release()
task_queue.task_done()
添加起始URL
start_urls = [
"https://example.com",
"https://example.org",
]
for url in start_urls:
task_queue.put(url)
创建并启动爬虫线程
threads = []
for _ in range(5):
thread = threading.Thread(target=crawler)
threads.append(thread)
thread.start()
等待所有任务完成
for thread in threads:
thread.join()
输出爬取结果
print(f"Crawled URLs: {len(crawled_urls)}")
print("Crawled results:")
for result in crawled_results:
print(result)
在这个示例中,我们创建了一个任务队列task_queue
,并将起始URL放入队列中。每个爬虫线程从队列中获取任务,进行爬取,并提取其中的链接进行进一步爬取。通过使用lock
对象,我们确保了爬取进度和结果的线程安全。
总结
通过实际案例,我们可以更好地理解如何在实际应用中使用多线程共享变量。无论是多线程下载器还是多线程爬虫,通过使用Queue
模块和Lock
对象,我们可以确保共享数据的线程安全,并实现高效的多线程程序。
在实际开发中,需要根据具体的应用场景选择合适的同步机制,确保多线程程序的正确性和性能。通过理解和掌握这些同步机制,可以帮助我们编写更加健壮和高效的多线程程序。
相关问答FAQs:
如何在Python多线程中实现变量的共享?
在Python中,实现多线程共享变量通常可以通过全局变量或者使用线程安全的结构(如队列或共享内存)来完成。全局变量可以在多个线程之间共享,但需要注意线程安全问题。为此,可以使用threading
模块中的锁(Lock)来确保对共享变量的访问是安全的。
在Python多线程中,如何保证共享变量的线程安全?
保证线程安全的常用方法是使用Lock
或RLock
。通过在访问共享变量之前获取锁,确保只有一个线程可以访问该变量,从而避免数据竞争和不一致的问题。此外,可以使用Condition
和Semaphore
等同步原语来控制对共享资源的访问。
Python多线程与多进程的区别是什么?
Python多线程适合I/O密集型任务,通过共享内存和变量的方式实现高效的任务处理,而多进程则适合CPU密集型任务,利用多核CPU的优势。在多线程中,线程之间的共享变量可以引发线程安全问题,而多进程则通过进程间通信(IPC)来实现数据共享,通常更为安全。选择使用哪种方式取决于具体的应用场景和性能需求。
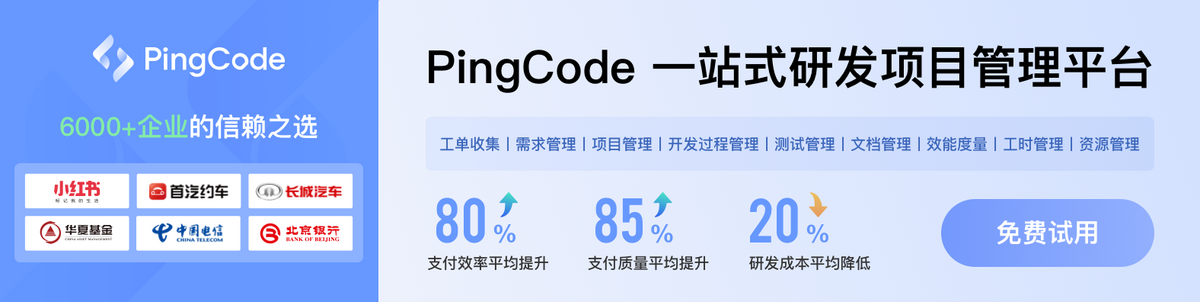