在Python中,可以通过多种方式获取指定文字内容,例如使用字符串方法、正则表达式、BeautifulSoup、lxml等。常用的方法包括字符串查找、正则表达式匹配、HTML解析等。本文将详细介绍这些方法及其使用场景。
一、字符串查找方法
字符串查找方法是最基本的方式之一,适用于简单的字符串匹配和提取。
1.1、使用 find
方法
find
方法用于查找子字符串在字符串中的位置。
text = "Hello, this is a sample text for Python string searching."
keyword = "sample"
position = text.find(keyword)
if position != -1:
print(f"Keyword '{keyword}' found at position {position}")
else:
print(f"Keyword '{keyword}' not found")
1.2、使用 split
方法
split
方法可以将字符串拆分成列表,从而提取指定内容。
text = "Hello, this is a sample text for Python string searching."
parts = text.split("sample")
if len(parts) > 1:
print(f"Content before keyword: {parts[0]}")
print(f"Content after keyword: {parts[1]}")
else:
print(f"Keyword 'sample' not found")
二、正则表达式方法
正则表达式是一种强大的文本处理工具,适用于复杂的字符串匹配和提取。
2.1、使用 re.search
方法
re.search
方法用于查找字符串中符合正则表达式的内容。
import re
text = "The price of the product is $25.99."
pattern = r"\$\d+\.\d{2}"
match = re.search(pattern, text)
if match:
print(f"Found price: {match.group()}")
else:
print("Price not found")
2.2、使用 re.findall
方法
re.findall
方法可以查找所有符合正则表达式的内容,并返回一个列表。
import re
text = "Contact us at support@example.com or sales@example.com."
pattern = r"\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b"
emails = re.findall(pattern, text)
print(f"Found emails: {emails}")
三、HTML解析方法
HTML解析方法适用于从HTML文档中提取内容,例如使用 BeautifulSoup 和 lxml。
3.1、使用 BeautifulSoup
BeautifulSoup 是一个用于解析HTML和XML文档的库。
from bs4 import BeautifulSoup
html = """
<html>
<head><title>Sample Page</title></head>
<body>
<p id="content">This is a sample paragraph.</p>
</body>
</html>
"""
soup = BeautifulSoup(html, 'html.parser')
content = soup.find(id="content").text
print(f"Extracted content: {content}")
3.2、使用 lxml
lxml 是一个用于解析和处理XML和HTML的库。
from lxml import etree
html = """
<html>
<head><title>Sample Page</title></head>
<body>
<p id="content">This is a sample paragraph.</p>
</body>
</html>
"""
tree = etree.HTML(html)
content = tree.xpath('//p[@id="content"]/text()')[0]
print(f"Extracted content: {content}")
四、使用第三方库
除了上述方法,还有许多第三方库可以帮助提取指定文字内容,例如 requests 和 selenium。
4.1、使用 requests 和 BeautifulSoup
requests 库用于获取网页内容,BeautifulSoup 用于解析网页。
import requests
from bs4 import BeautifulSoup
url = "http://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
content = soup.find('h1').text
print(f"Extracted content: {content}")
4.2、使用 selenium
selenium 是一个自动化测试工具,可以模拟浏览器操作。
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get("http://example.com")
element = driver.find_element(By.TAG_NAME, "h1")
content = element.text
print(f"Extracted content: {content}")
driver.quit()
五、综合应用场景
在实际应用中,往往需要将多种方法结合起来使用,以处理复杂的文本提取需求。
5.1、从网页中提取特定信息
假设需要从网页中提取所有文章标题和链接,可以结合使用 requests 和 BeautifulSoup。
import requests
from bs4 import BeautifulSoup
url = "http://example-blog.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
articles = soup.find_all('article')
for article in articles:
title = article.find('h2').text
link = article.find('a')['href']
print(f"Title: {title}, Link: {link}")
5.2、从日志文件中提取错误信息
假设需要从日志文件中提取所有错误信息,可以使用正则表达式。
import re
log_file = "system.log"
pattern = r"ERROR: (.*)"
with open(log_file, 'r') as file:
for line in file:
match = re.search(pattern, line)
if match:
print(f"Error message: {match.group(1)}")
六、处理不同编码格式的文本
在处理文本时,经常会遇到不同的编码格式,Python 提供了多种方法来处理这些情况。
6.1、读取不同编码格式的文件
使用 open
函数的 encoding
参数,可以指定文件的编码格式。
file_path = "example.txt"
encoding = "utf-8"
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
print(content)
6.2、处理网络请求中的编码格式
使用 requests 库时,可以通过 response.encoding
属性设置编码格式。
import requests
url = "http://example.com"
response = requests.get(url)
response.encoding = 'utf-8'
content = response.text
print(content)
七、文本预处理
在提取指定文字内容之前,通常需要对文本进行预处理,以提高提取的准确性。
7.1、去除多余的空格和换行符
可以使用 strip
方法去除字符串两端的空格和换行符。
text = " This is a sample text. \n"
cleaned_text = text.strip()
print(f"Cleaned text: '{cleaned_text}'")
7.2、转换大小写
可以使用 lower
或 upper
方法将字符串转换为小写或大写。
text = "This is a Sample Text."
lower_text = text.lower()
upper_text = text.upper()
print(f"Lowercase text: '{lower_text}'")
print(f"Uppercase text: '{upper_text}'")
八、处理多行文本
在处理多行文本时,可以使用 splitlines
方法将字符串按行拆分为列表。
8.1、逐行处理文本
text = "Line 1\nLine 2\nLine 3"
lines = text.splitlines()
for line in lines:
print(f"Processing line: {line}")
8.2、查找包含关键字的行
text = "Line 1: Error\nLine 2: Success\nLine 3: Error"
lines = text.splitlines()
keyword = "Error"
for line in lines:
if keyword in line:
print(f"Found keyword in line: {line}")
九、处理特殊字符
在处理文本时,经常会遇到特殊字符,可以使用正则表达式或字符串方法进行处理。
9.1、去除特殊字符
可以使用正则表达式去除字符串中的特殊字符。
import re
text = "Hello, this is a sample text! @#&*()"
cleaned_text = re.sub(r'[^\w\s]', '', text)
print(f"Cleaned text: '{cleaned_text}'")
9.2、替换特殊字符
可以使用 replace
方法替换字符串中的特殊字符。
text = "Hello, this is a sample text! @#&*()"
replaced_text = text.replace('@', 'at').replace('#', 'number')
print(f"Replaced text: '{replaced_text}'")
十、处理多种语言文本
在处理多种语言文本时,需要注意字符编码和文本方向。
10.1、处理Unicode字符
可以使用 unicodedata
模块处理Unicode字符。
import unicodedata
text = "Café"
normalized_text = unicodedata.normalize('NFC', text)
print(f"Normalized text: '{normalized_text}'")
10.2、处理RTL文本
在处理右到左(RTL)文本时,可以使用 bidi
模块。
from bidi.algorithm import get_display
text = "مرحبا بالعالم"
display_text = get_display(text)
print(f"Display text: '{display_text}'")
十一、处理大文件
在处理大文件时,可以逐行读取文件,以节省内存。
11.1、逐行读取文件
file_path = "large_file.txt"
with open(file_path, 'r') as file:
for line in file:
print(f"Processing line: {line.strip()}")
11.2、使用生成器处理大文件
可以使用生成器逐行处理大文件。
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
file_path = "large_file.txt"
for line in read_large_file(file_path):
print(f"Processing line: {line}")
十二、总结
在Python中获取指定文字内容的方法多种多样,包括字符串查找方法、正则表达式、HTML解析和使用第三方库等。每种方法都有其适用的场景和优缺点。在实际应用中,往往需要根据具体需求选择合适的方法,并结合文本预处理、编码处理、多行文本处理和特殊字符处理等技术,以提高文本提取的准确性和效率。通过掌握这些方法和技巧,可以更好地应对各种文本处理任务。
相关问答FAQs:
如何在Python中提取特定字符串的内容?
在Python中,可以使用字符串的内置方法,例如find()
、index()
或切片来提取特定字符串。使用正则表达式模块re
也是一种强大的方法,可以匹配复杂模式并提取所需内容。具体实现方式可以根据你的需求而定,简单的字符串查找适合使用内置方法,而复杂的模式匹配则建议使用re
模块。
在Python中使用正则表达式提取文本时需要注意哪些事项?
在使用正则表达式提取文本内容时,确保了解正则表达式的基本语法,包括字符类、量词和捕获组等。还要注意处理转义字符,以避免语法错误。调试正则表达式时,可以使用在线工具来测试和验证你的表达式,确保它能够正确提取所需的内容。
如何处理文本中的多个匹配项并提取所有相关内容?
使用re.findall()
方法可以轻松提取文本中所有匹配的内容。该方法返回一个列表,包含所有匹配的字符串。通过定义适当的正则表达式,可以获得所需的所有结果。如果需要提取匹配项的详细信息,可以使用re.finditer()
,该方法返回一个迭代器,允许你逐个访问匹配的对象并提取相关信息。
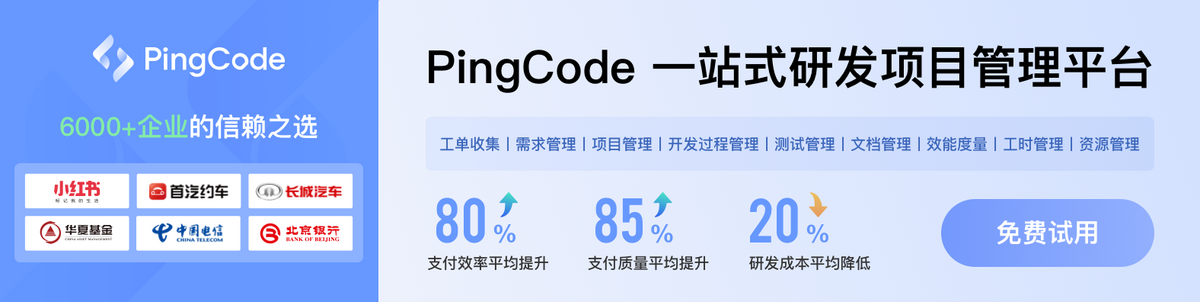