Python读取并调用函数的方式有多种,包括import模块、直接定义函数、调用内置函数等。 在实际应用中,最常见的方法是使用import语句导入模块,然后调用模块中的函数。此方法不仅能够提高代码的可重用性,还能使代码结构更加清晰。以下是详细描述其中一种方法:
使用import语句导入模块中的函数。在Python中,有很多预定义的模块包含许多有用的函数,我们可以通过import语句来导入这些模块,然后调用模块中的函数。例如,math模块包含了许多数学函数,如sqrt()、sin()等。使用math模块中的函数,可以大大简化复杂的数学运算。
导入模块的方式有几种:
import module_name
:导入整个模块。from module_name import function_name
:从模块中导入特定的函数。import module_name as alias
:为导入的模块指定别名。
具体示例:
假设我们需要计算一个数的平方根,可以使用math模块中的sqrt()函数:
import math
number = 25
square_root = math.sqrt(number)
print(f"The square root of {number} is {square_root}")
通过上述方法,我们不仅能简化代码,还能提高代码的可读性和可维护性。以下将详细介绍Python中如何读取并调用函数的其他方法。
一、定义并调用函数
在Python中,函数是一组语句的集合,用于执行特定任务。定义函数使用def
关键字,接着是函数名、参数列表和冒号。函数体缩进四个空格。
def greet(name):
"""This function greets the person passed in as a parameter"""
print(f"Hello, {name}!")
调用函数
greet('Alice')
1、函数定义
定义函数时,需要注意以下几点:
- 函数名应简洁、有意义,通常使用小写字母和下划线。
- 参数列表可以为空或包含多个参数。
- 函数体包含执行特定任务的代码块。
- 函数可以包含文档字符串,用于描述函数的功能。
def add_numbers(a, b):
"""This function returns the sum of two numbers"""
return a + b
2、函数调用
调用函数时,传递的参数个数和类型应与定义时一致。可以通过位置参数或关键字参数传递参数。
# 位置参数
result = add_numbers(5, 3)
print(result) # 输出:8
关键字参数
result = add_numbers(a=5, b=3)
print(result) # 输出:8
二、模块的导入与使用
Python模块是一组相关函数和变量的集合,通常包含在一个文件中。通过导入模块,可以重用代码,提高开发效率。
1、导入整个模块
使用import
语句导入模块,然后通过module_name.function_name
调用函数。
import math
调用math模块中的sqrt函数
result = math.sqrt(16)
print(result) # 输出:4.0
2、从模块中导入特定函数
使用from module_name import function_name
语句,可以只导入模块中的特定函数,直接使用函数名调用。
from math import sqrt
直接调用sqrt函数
result = sqrt(16)
print(result) # 输出:4.0
3、为模块指定别名
使用import module_name as alias
语句,可以为模块指定别名,简化调用。
import math as m
使用别名调用sqrt函数
result = m.sqrt(16)
print(result) # 输出:4.0
三、内置函数的使用
Python提供了许多内置函数,直接可用,无需导入。例如,print()
函数用于输出信息,len()
函数用于获取序列的长度。
# 使用print函数输出信息
print("Hello, World!")
使用len函数获取列表长度
numbers = [1, 2, 3, 4, 5]
length = len(numbers)
print(length) # 输出:5
四、高阶函数
高阶函数是指可以接收函数作为参数,或返回一个函数的函数。例如,map()
、filter()
和reduce()
都是高阶函数。
1、map()函数
map()
函数用于将指定函数应用于可迭代对象的每个元素,并返回一个迭代器。
# 定义一个函数
def square(x):
return x * x
使用map函数
numbers = [1, 2, 3, 4, 5]
squared_numbers = map(square, numbers)
print(list(squared_numbers)) # 输出:[1, 4, 9, 16, 25]
2、filter()函数
filter()
函数用于过滤可迭代对象的元素,返回一个迭代器。
# 定义一个函数
def is_even(x):
return x % 2 == 0
使用filter函数
numbers = [1, 2, 3, 4, 5]
even_numbers = filter(is_even, numbers)
print(list(even_numbers)) # 输出:[2, 4]
3、reduce()函数
reduce()
函数用于将可迭代对象的元素进行累积计算,返回单个值。需要从functools
模块导入。
from functools import reduce
定义一个函数
def add(x, y):
return x + y
使用reduce函数
numbers = [1, 2, 3, 4, 5]
sum_result = reduce(add, numbers)
print(sum_result) # 输出:15
五、匿名函数
匿名函数使用lambda
关键字定义,通常用于简单的、短小的函数。匿名函数没有名称,直接使用表达式计算结果。
# 定义一个匿名函数
square = lambda x: x * x
调用匿名函数
result = square(5)
print(result) # 输出:25
匿名函数常与高阶函数结合使用,例如map()
、filter()
等。
# 使用map函数和匿名函数
numbers = [1, 2, 3, 4, 5]
squared_numbers = map(lambda x: x * x, numbers)
print(list(squared_numbers)) # 输出:[1, 4, 9, 16, 25]
六、递归函数
递归函数是指在函数内部调用自身的函数。递归函数通常用于解决分治问题,如计算阶乘、斐波那契数列等。
1、计算阶乘
阶乘是指从1到n的所有整数的乘积,记作n!。使用递归函数计算阶乘如下:
def factorial(n):
"""This function returns the factorial of a number"""
if n == 0:
return 1
else:
return n * factorial(n - 1)
调用递归函数
result = factorial(5)
print(result) # 输出:120
2、计算斐波那契数列
斐波那契数列是指一个数列,其中每个数是前两个数之和。使用递归函数计算斐波那契数列如下:
def fibonacci(n):
"""This function returns the nth Fibonacci number"""
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
调用递归函数
result = fibonacci(6)
print(result) # 输出:8
七、函数装饰器
函数装饰器是用于修改函数行为的高级工具。装饰器是一个返回函数的函数,通常用于在函数前后添加额外逻辑。
def decorator(func):
"""This decorator prints a message before and after the function call"""
def wrapper(*args, kwargs):
print("Before function call")
result = func(*args, kwargs)
print("After function call")
return result
return wrapper
@decorator
def greet(name):
print(f"Hello, {name}!")
调用装饰后的函数
greet('Alice')
在上述示例中,@decorator
语法等效于greet = decorator(greet)
,装饰器在函数调用前后打印消息。
八、函数注解
函数注解是用于描述函数参数和返回值类型的元数据。使用注解可以提高代码的可读性和可维护性。
def add_numbers(a: int, b: int) -> int:
"""This function returns the sum of two integers"""
return a + b
调用带注解的函数
result = add_numbers(5, 3)
print(result) # 输出:8
注解不会影响函数的执行,仅作为文档信息存在。可以通过__annotations__
属性访问注解。
print(add_numbers.__annotations__)
输出:{'a': <class 'int'>, 'b': <class 'int'>, 'return': <class 'int'>}
九、参数解包
参数解包是指将可迭代对象的元素作为函数参数传递。使用*args
和kwargs
可以实现位置参数和关键字参数的解包。
1、位置参数解包
使用*args
解包位置参数,将可迭代对象的元素作为函数参数传递。
def add_numbers(*args):
"""This function returns the sum of all arguments"""
return sum(args)
调用函数时传递多个位置参数
result = add_numbers(1, 2, 3, 4, 5)
print(result) # 输出:15
2、关键字参数解包
使用kwargs
解包关键字参数,将字典的键值对作为函数参数传递。
def greet(kwargs):
"""This function greets the person with given keyword arguments"""
for key, value in kwargs.items():
print(f"{key} = {value}")
调用函数时传递多个关键字参数
greet(name='Alice', age=25)
输出:
name = Alice
age = 25
十、生成器函数
生成器函数是使用yield
关键字返回一个生成器对象的函数。生成器函数用于逐个生成值,而不是一次性返回所有值,节省内存。
def fibonacci(n):
"""This generator function yields the first n Fibonacci numbers"""
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
调用生成器函数
for num in fibonacci(10):
print(num)
输出:0 1 1 2 3 5 8 13 21 34
生成器函数在每次调用yield
时暂停执行,返回一个值。下一次调用生成器对象的__next__()
方法时,从暂停处继续执行。
十一、闭包
闭包是指在函数内部定义的函数,可以访问外部函数的局部变量。闭包用于实现数据封装和函数工厂。
def outer_func(x):
"""This function returns an inner function that adds x to its argument"""
def inner_func(y):
return x + y
return inner_func
调用外部函数,返回闭包
add_five = outer_func(5)
调用闭包函数
result = add_five(10)
print(result) # 输出:15
闭包通过保存外部函数的局部变量,实现数据的封装和共享。
十二、文档字符串
文档字符串是用于描述函数、模块、类和方法功能的字符串。文档字符串通常放在定义体的第一行,使用三重引号包围。
def add_numbers(a, b):
"""
This function returns the sum of two numbers.
Parameters:
a (int or float): The first number.
b (int or float): The second number.
Returns:
int or float: The sum of a and b.
"""
return a + b
访问函数的文档字符串
print(add_numbers.__doc__)
使用文档字符串可以提高代码的可读性和可维护性,便于他人理解函数的功能和使用方法。
十三、函数式编程
函数式编程是一种编程范式,强调函数的使用和组合。Python支持函数式编程的许多特性,如高阶函数、匿名函数和不可变数据。
1、不可变数据
不可变数据在创建后不能修改,如元组、字符串和frozenset。使用不可变数据可以避免副作用,提高代码的可靠性。
# 创建不可变元组
numbers = (1, 2, 3, 4, 5)
尝试修改元组会引发错误
numbers[0] = 0 # TypeError: 'tuple' object does not support item assignment
2、函数组合
函数组合是指将多个函数组合成一个新函数。可以使用lambda表达式和高阶函数实现函数组合。
# 定义两个简单函数
def multiply_by_two(x):
return x * 2
def add_three(x):
return x + 3
组合函数
def combined_func(x):
return add_three(multiply_by_two(x))
调用组合函数
result = combined_func(5)
print(result) # 输出:13
函数式编程强调函数的纯粹性,即函数不应有副作用,仅依赖输入参数,返回确定的输出。
十四、错误处理
错误处理是指在函数中处理可能出现的异常情况。使用try-except
语句可以捕获和处理异常,保证程序的正常运行。
def safe_divide(a, b):
"""This function safely divides two numbers and handles division by zero"""
try:
result = a / b
except ZeroDivisionError:
return "Error: Division by zero"
else:
return result
调用带错误处理的函数
print(safe_divide(10, 2)) # 输出:5.0
print(safe_divide(10, 0)) # 输出:Error: Division by zero
在上述示例中,try
块包含可能引发异常的代码,except
块处理异常情况,else
块在没有异常时执行。
十五、参数类型检查
参数类型检查是指在函数中验证参数的类型,确保函数接收到正确类型的参数。可以使用isinstance()
函数进行类型检查。
def add_numbers(a, b):
"""This function returns the sum of two numbers with type check"""
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both arguments must be int or float")
return a + b
调用带类型检查的函数
print(add_numbers(5, 3)) # 输出:8
print(add_numbers(5, '3')) # TypeError: Both arguments must be int or float
在上述示例中,函数在执行前检查参数类型,如果参数类型不正确,抛出TypeError
异常。
十六、变量作用域
变量作用域是指变量在程序中的可见范围。Python中有四种作用域:局部作用域、嵌套作用域、全局作用域和内置作用域。
1、局部作用域
局部作用域是指函数内部定义的变量,仅在函数内部可见。
def func():
x = 10 # 局部变量
print(x)
调用函数
func() # 输出:10
print(x) # NameError: name 'x' is not defined
2、嵌套作用域
嵌套作用域是指在嵌套函数中,内部函数可以访问外部函数的变量。
def outer_func():
x = 10 # 外部函数的局部变量
def inner_func():
print(x) # 访问外部函数的局部变量
inner_func()
调用外部函数
outer_func() # 输出:10
3、全局作用域
全局作用域是指在模块级别定义的变量,可以在整个模块中访问。
x = 10 # 全局变量
def func():
print(x) # 访问全局变量
调用函数
相关问答FAQs:
Python中如何导入其他模块以读取函数?
在Python中,您可以使用import
语句导入其他模块,从而读取和调用其中的函数。例如,如果您有一个名为my_module.py
的文件,其中定义了一个函数my_function()
,您可以通过以下方式导入并调用它:
import my_module
my_module.my_function()
确保模块在Python的搜索路径中,或者您可以使用sys.path.append()
将其添加到路径中。
如何在Python中读取和调用一个类中的方法?
在Python中,您可以通过实例化类来访问其方法。假设有一个类MyClass
,其中定义了一个方法my_method()
,您可以这样读取和调用它:
class MyClass:
def my_method(self):
print("Hello, World!")
my_instance = MyClass()
my_instance.my_method()
通过创建MyClass
的实例my_instance
,您就能够调用my_method()
方法。
Python是否支持动态读取和调用函数?
是的,Python支持动态读取和调用函数。您可以使用getattr()
函数来获取对象的属性或方法。例如,如果您有一个对象obj
,并且您想调用名为func_name
的函数,可以这样做:
func = getattr(obj, 'func_name')
func()
这种方法允许您在运行时决定要调用的函数,使得代码更具灵活性和可扩展性。
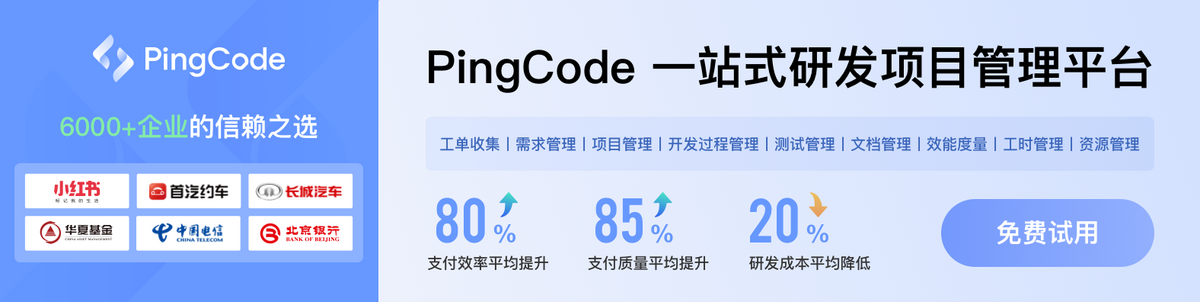