货币转换Python程序的运行方法包括:获取汇率数据、编写货币转换函数、处理用户输入、输出转换结果。以下是详细的介绍。
一、获取汇率数据
货币转换程序的核心在于准确的汇率数据。汇率数据可以通过多种方式获取,例如使用在线API服务。常见的汇率API服务有ExchangeRate-API、Open Exchange Rates、Fixer等。这些服务提供实时的汇率数据,通常需要注册获取API密钥。
import requests
def get_exchange_rate(api_key, base_currency, target_currency):
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/{base_currency}"
response = requests.get(url)
data = response.json()
if response.status_code == 200:
return data['conversion_rates'][target_currency]
else:
raise Exception("Error in fetching exchange rate data.")
二、编写货币转换函数
有了汇率数据后,我们需要编写一个函数来执行货币转换。此函数接收用户输入的金额、基础货币和目标货币,并返回转换后的金额。
def convert_currency(amount, exchange_rate):
return amount * exchange_rate
三、处理用户输入
为了使程序能够与用户交互,我们需要处理用户输入。这可以通过命令行输入或图形用户界面(GUI)来实现。以下是一个简单的命令行输入示例。
def get_user_input():
amount = float(input("Enter the amount to convert: "))
base_currency = input("Enter the base currency (e.g., USD): ")
target_currency = input("Enter the target currency (e.g., EUR): ")
return amount, base_currency, target_currency
四、输出转换结果
最后一步是将转换结果输出给用户。我们可以将上述所有步骤整合在一起形成一个完整的货币转换程序。
def main():
api_key = "your_api_key_here"
# 获取用户输入
amount, base_currency, target_currency = get_user_input()
# 获取汇率数据
try:
exchange_rate = get_exchange_rate(api_key, base_currency, target_currency)
# 进行货币转换
converted_amount = convert_currency(amount, exchange_rate)
# 输出结果
print(f"{amount} {base_currency} is equal to {converted_amount:.2f} {target_currency}")
except Exception as e:
print(f"Error: {e}")
if __name__ == "__main__":
main()
五、扩展功能
1、处理多种输入格式
为了增强用户体验,可以处理多种输入格式。例如,用户可能会输入"USD"或"usd"。我们可以将用户输入转换为大写或小写格式来处理这一问题。
def get_user_input():
amount = float(input("Enter the amount to convert: "))
base_currency = input("Enter the base currency (e.g., USD): ").upper()
target_currency = input("Enter the target currency (e.g., EUR): ").upper()
return amount, base_currency, target_currency
2、处理异常情况
为了提高程序的健壮性,我们应该处理各种可能的异常情况。例如,用户输入的货币代码可能无效,API请求可能失败,输入的金额可能不是数字等。
def get_user_input():
try:
amount = float(input("Enter the amount to convert: "))
except ValueError:
print("Invalid amount. Please enter a numeric value.")
return get_user_input()
base_currency = input("Enter the base currency (e.g., USD): ").upper()
target_currency = input("Enter the target currency (e.g., EUR): ").upper()
return amount, base_currency, target_currency
六、图形用户界面(GUI)
对于更友好的用户体验,可以使用图形用户界面来实现货币转换。Python有许多GUI库,如Tkinter、PyQt等。以下是使用Tkinter实现的简单GUI示例。
import tkinter as tk
from tkinter import messagebox
def get_exchange_rate(api_key, base_currency, target_currency):
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/{base_currency}"
response = requests.get(url)
data = response.json()
if response.status_code == 200:
return data['conversion_rates'][target_currency]
else:
raise Exception("Error in fetching exchange rate data.")
def convert_currency(amount, exchange_rate):
return amount * exchange_rate
def on_convert():
try:
amount = float(amount_entry.get())
base_currency = base_currency_entry.get().upper()
target_currency = target_currency_entry.get().upper()
exchange_rate = get_exchange_rate(api_key, base_currency, target_currency)
converted_amount = convert_currency(amount, exchange_rate)
result_label.config(text=f"{amount} {base_currency} is equal to {converted_amount:.2f} {target_currency}")
except Exception as e:
messagebox.showerror("Error", str(e))
api_key = "your_api_key_here"
root = tk.Tk()
root.title("Currency Converter")
tk.Label(root, text="Amount:").grid(row=0, column=0)
amount_entry = tk.Entry(root)
amount_entry.grid(row=0, column=1)
tk.Label(root, text="Base Currency:").grid(row=1, column=0)
base_currency_entry = tk.Entry(root)
base_currency_entry.grid(row=1, column=1)
tk.Label(root, text="Target Currency:").grid(row=2, column=0)
target_currency_entry = tk.Entry(root)
target_currency_entry.grid(row=2, column=1)
convert_button = tk.Button(root, text="Convert", command=on_convert)
convert_button.grid(row=3, column=0, columnspan=2)
result_label = tk.Label(root, text="")
result_label.grid(row=4, column=0, columnspan=2)
root.mainloop()
七、总结
货币转换程序的基本功能包括获取汇率数据、编写货币转换函数、处理用户输入和输出转换结果。通过扩展功能和使用图形用户界面,可以提高用户体验和程序的健壮性。希望这篇文章能帮助你更好地理解和实现货币转换Python程序。
相关问答FAQs:
如何使用Python进行货币转换?
要使用Python进行货币转换,您需要了解基本的Python编程知识,并安装一些必要的库,比如requests
用于获取实时汇率数据,或者使用forex-python
库来简化转换过程。通过编写一个简单的脚本,您可以输入要转换的金额和货币类型,并获得转换后的结果。
我可以使用哪些API来获取实时汇率?
有多种API可以为您提供实时汇率信息。例如,ExchangeRate-API、Open Exchange Rates和Fixer.io都是非常流行的选择。您需要注册并获取API密钥,然后在您的Python程序中使用requests
库发送请求以获取所需的汇率数据。
如何处理汇率波动对我的转换结果的影响?
汇率波动是货币转换中不可避免的因素。为了减少这种波动的影响,您可以定期更新汇率数据,或者在程序中添加一个选项,以允许用户选择固定汇率或最新汇率进行转换。此外,保持对市场动态的关注,可以帮助您在进行大额转换时做出更明智的决策。
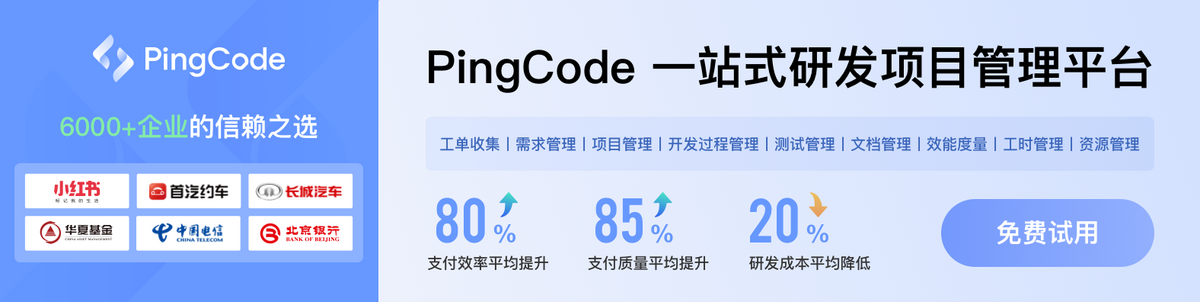