Python下载网上图片的常用方法有:使用requests库、使用urllib库、使用第三方库如BeautifulSoup和Selenium等。 其中,最常用和简便的方法是使用requests库,因为它易于使用,并且能够处理大多数情况。下面将详细描述如何使用requests库下载图片。
使用requests库下载图片时,首先需要导入requests库,然后通过requests.get方法获取图片的内容,最后将内容保存到本地文件中。下面是一个简单的示例代码:
import requests
url = 'https://example.com/image.jpg'
response = requests.get(url)
with open('image.jpg', 'wb') as file:
file.write(response.content)
在这个示例中,首先使用requests.get方法获取图片的内容,然后使用open方法以二进制写入模式打开一个本地文件,并将图片内容写入该文件中。
一、使用requests库下载图片
requests库是Python中非常流行的HTTP库,适用于处理网络请求。它简单易用,支持各种HTTP请求方法(如GET、POST等),并且能够处理Cookies、会话、SSL验证等复杂功能。
1、基本使用方法
使用requests库下载图片非常简单。下面的示例代码展示了如何通过requests库下载图片并保存到本地:
import requests
def download_image(url, filename):
response = requests.get(url)
if response.status_code == 200:
with open(filename, 'wb') as file:
file.write(response.content)
print(f"Image downloaded and saved as {filename}")
else:
print(f"Failed to retrieve image. Status code: {response.status_code}")
url = 'https://example.com/image.jpg'
filename = 'image.jpg'
download_image(url, filename)
在这个示例中,首先定义了一个函数download_image,该函数接收图片的URL和保存文件名作为参数。通过requests.get方法获取图片内容,并检查响应状态码是否为200(表示成功)。如果成功,则将图片内容写入本地文件中。
2、处理重定向和超时
在实际使用中,可能会遇到重定向和请求超时的问题。requests库提供了相关参数来处理这些问题:
import requests
def download_image(url, filename, timeout=10):
try:
response = requests.get(url, timeout=timeout)
if response.status_code == 200:
with open(filename, 'wb') as file:
file.write(response.content)
print(f"Image downloaded and saved as {filename}")
else:
print(f"Failed to retrieve image. Status code: {response.status_code}")
except requests.exceptions.Timeout:
print(f"Request timed out for URL: {url}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
url = 'https://example.com/image.jpg'
filename = 'image.jpg'
download_image(url, filename)
在这个示例中,增加了timeout参数来设置请求的超时时间,并使用try-except块捕获可能的异常(如超时异常和其他请求异常)。这样可以更好地处理请求失败的情况。
二、使用urllib库下载图片
urllib库是Python的标准库之一,包含了一系列用于处理URL的模块。与requests库相比,urllib库更底层一些,但也能完成下载图片的任务。
1、基本使用方法
使用urllib库下载图片同样非常简单。下面的示例代码展示了如何通过urllib库下载图片并保存到本地:
import urllib.request
def download_image(url, filename):
try:
urllib.request.urlretrieve(url, filename)
print(f"Image downloaded and saved as {filename}")
except urllib.error.URLError as e:
print(f"Failed to retrieve image. Error: {e}")
url = 'https://example.com/image.jpg'
filename = 'image.jpg'
download_image(url, filename)
在这个示例中,使用了urllib.request.urlretrieve方法直接下载图片并保存到本地文件中。如果下载失败,则会捕获并打印异常信息。
2、处理重定向和超时
与requests库类似,urllib库也能处理重定向和请求超时的问题。下面是一个处理重定向和超时的示例:
import urllib.request
import socket
def download_image(url, filename, timeout=10):
try:
response = urllib.request.urlopen(url, timeout=timeout)
with open(filename, 'wb') as file:
file.write(response.read())
print(f"Image downloaded and saved as {filename}")
except urllib.error.URLError as e:
print(f"Failed to retrieve image. Error: {e}")
except socket.timeout:
print(f"Request timed out for URL: {url}")
url = 'https://example.com/image.jpg'
filename = 'image.jpg'
download_image(url, filename)
在这个示例中,使用了urllib.request.urlopen方法获取图片内容,并设置了请求超时时间。通过捕获urllib.error.URLError和socket.timeout异常来处理请求失败的情况。
三、使用BeautifulSoup库下载图片
BeautifulSoup是一个用于解析HTML和XML文档的库,常用于网页抓取和数据提取。通过BeautifulSoup库,可以方便地提取网页中的图片URL,并使用requests或urllib库下载图片。
1、基本使用方法
首先,需要安装BeautifulSoup库和requests库:
pip install beautifulsoup4 requests
然后,使用BeautifulSoup库提取网页中的图片URL并下载图片:
import requests
from bs4 import BeautifulSoup
def download_images_from_page(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
img_tags = soup.find_all('img')
for img in img_tags:
img_url = img.get('src')
if img_url:
filename = img_url.split('/')[-1]
download_image(img_url, filename)
else:
print(f"Failed to retrieve page. Status code: {response.status_code}")
def download_image(url, filename):
response = requests.get(url)
if response.status_code == 200:
with open(filename, 'wb') as file:
file.write(response.content)
print(f"Image downloaded and saved as {filename}")
else:
print(f"Failed to retrieve image. Status code: {response.status_code}")
page_url = 'https://example.com'
download_images_from_page(page_url)
在这个示例中,首先通过requests.get方法获取网页内容,并使用BeautifulSoup解析HTML文档。然后,使用soup.find_all方法找到所有的img标签,并提取每个img标签的src属性(即图片URL)。最后,通过download_image函数下载图片并保存到本地。
2、处理相对路径和超时
在实际使用中,可能会遇到相对路径和请求超时的问题。下面的示例代码展示了如何处理这些问题:
import requests
from bs4 import BeautifulSoup
from urllib.parse import urljoin
def download_images_from_page(url, base_url=None, timeout=10):
try:
response = requests.get(url, timeout=timeout)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
img_tags = soup.find_all('img')
for img in img_tags:
img_url = img.get('src')
if img_url:
if base_url:
img_url = urljoin(base_url, img_url)
filename = img_url.split('/')[-1]
download_image(img_url, filename, timeout)
else:
print(f"Failed to retrieve page. Status code: {response.status_code}")
except requests.exceptions.Timeout:
print(f"Request timed out for URL: {url}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
def download_image(url, filename, timeout=10):
try:
response = requests.get(url, timeout=timeout)
if response.status_code == 200:
with open(filename, 'wb') as file:
file.write(response.content)
print(f"Image downloaded and saved as {filename}")
else:
print(f"Failed to retrieve image. Status code: {response.status_code}")
except requests.exceptions.Timeout:
print(f"Request timed out for URL: {url}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
page_url = 'https://example.com'
download_images_from_page(page_url, base_url=page_url)
在这个示例中,增加了base_url参数来处理相对路径问题,并使用urljoin函数将相对路径转换为绝对路径。同时,通过设置timeout参数和使用try-except块来处理请求超时和其他请求异常。
四、使用Selenium库下载图片
Selenium是一个用于Web应用程序测试的工具,但也常用于网页抓取。通过Selenium,可以模拟用户操作(如点击、滚动等)来获取动态加载的内容和图片。
1、基本使用方法
首先,需要安装Selenium库和相应的浏览器驱动程序(如ChromeDriver):
pip install selenium
然后,使用Selenium模拟用户操作并下载图片:
from selenium import webdriver
import requests
def download_images_from_page(url):
driver = webdriver.Chrome() # 使用Chrome浏览器
driver.get(url)
img_tags = driver.find_elements_by_tag_name('img')
for img in img_tags:
img_url = img.get_attribute('src')
if img_url:
filename = img_url.split('/')[-1]
download_image(img_url, filename)
driver.quit()
def download_image(url, filename):
response = requests.get(url)
if response.status_code == 200:
with open(filename, 'wb') as file:
file.write(response.content)
print(f"Image downloaded and saved as {filename}")
else:
print(f"Failed to retrieve image. Status code: {response.status_code}")
page_url = 'https://example.com'
download_images_from_page(page_url)
在这个示例中,使用Selenium的webdriver启动Chrome浏览器,并通过get方法打开指定的URL。然后,使用find_elements_by_tag_name方法找到所有的img标签,并提取每个img标签的src属性(即图片URL)。最后,通过download_image函数下载图片并保存到本地。
2、处理动态加载和超时
在实际使用中,可能会遇到动态加载和请求超时的问题。下面的示例代码展示了如何处理这些问题:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import requests
def download_images_from_page(url, timeout=10):
driver = webdriver.Chrome() # 使用Chrome浏览器
driver.get(url)
try:
# 等待页面加载完成
WebDriverWait(driver, timeout).until(
EC.presence_of_element_located((By.TAG_NAME, 'img'))
)
img_tags = driver.find_elements_by_tag_name('img')
for img in img_tags:
img_url = img.get_attribute('src')
if img_url:
filename = img_url.split('/')[-1]
download_image(img_url, filename, timeout)
except Exception as e:
print(f"An error occurred: {e}")
finally:
driver.quit()
def download_image(url, filename, timeout=10):
try:
response = requests.get(url, timeout=timeout)
if response.status_code == 200:
with open(filename, 'wb') as file:
file.write(response.content)
print(f"Image downloaded and saved as {filename}")
else:
print(f"Failed to retrieve image. Status code: {response.status_code}")
except requests.exceptions.Timeout:
print(f"Request timed out for URL: {url}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
page_url = 'https://example.com'
download_images_from_page(page_url)
在这个示例中,使用了WebDriverWait和expected_conditions来等待页面加载完成,确保所有图片都已经加载。在下载图片时,通过设置timeout参数和使用try-except块来处理请求超时和其他请求异常。
五、总结
通过上述介绍,可以看出Python提供了多种下载网上图片的方法,包括使用requests库、urllib库、BeautifulSoup库和Selenium库。每种方法都有其优缺点和适用场景。在实际使用中,可以根据具体需求选择合适的方法。
- requests库:简单易用,适用于处理大多数静态图片下载任务。
- urllib库:Python标准库,适用于不依赖第三方库的情况下下载图片。
- BeautifulSoup库:适用于从网页中提取图片URL并下载图片,尤其是需要处理复杂HTML结构的情况。
- Selenium库:适用于处理动态加载的图片和需要模拟用户操作的情况。
无论选择哪种方法,都需要注意处理请求失败、超时、重定向等问题,以确保下载任务能够顺利完成。希望通过本文的介绍,能够帮助大家更好地理解和使用Python下载网上图片。
相关问答FAQs:
如何使用Python下载特定网站上的图片?
要下载特定网站上的图片,可以使用Python的requests库和BeautifulSoup库。首先,使用requests库获取网页内容,然后利用BeautifulSoup解析HTML,找到图片的URL。接着,通过requests库下载图片并保存到本地。示例代码如下:
import requests
from bs4 import BeautifulSoup
url = '目标网址'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
for img in soup.find_all('img'):
img_url = img['src']
img_data = requests.get(img_url).content
with open('图片名称.jpg', 'wb') as handler:
handler.write(img_data)
在Python中如何处理下载的图片格式?
下载图片后,可能会面临不同的文件格式,例如JPEG、PNG或GIF。可以使用PIL(Pillow)库来处理这些图片格式。该库提供了一系列功能,如打开、修改和保存图片。示例代码如下:
from PIL import Image
image = Image.open('下载的图片.jpg')
image.show() # 显示图片
image.save('新图片.png') # 转换并保存为PNG格式
使用Python下载大量图片时应该注意哪些事项?
在下载大量图片时,需注意几个关键点。首先,确保遵循网站的使用协议,避免对其服务器造成过大负担。其次,可以设置下载间隔,避免被识别为爬虫。最后,务必检查图片的版权信息,以确保合法使用。使用time模块可以添加延时,例如:
import time
# 在下载每张图片后添加延时
time.sleep(1) # 延时1秒
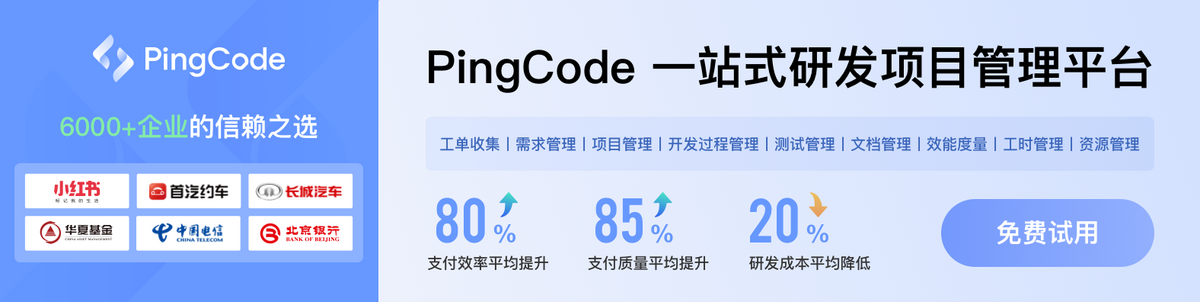