Python可以通过QQ的API接口、使用第三方库、通过Web Scraping等方式来发送和获取QQ信息。其中,使用QQ的API接口是一种最为直接和稳定的方法,因为它是由腾讯官方提供的,安全性和可靠性更高。本文将详细介绍如何通过API接口发送和获取QQ信息。
一、使用QQ的API接口
1、获取QQ API接口权限
要使用QQ的API接口,首先需要申请API权限。可以通过腾讯开放平台(open.qq.com)进行申请。在申请过程中,需要创建一个应用,并获取应用的AppID和AppKey。
2、使用OAuth2.0进行授权
QQ的API接口使用OAuth2.0授权机制,用户需要授权你的应用才能获取其信息。以下是一个简单的授权流程:
- 引导用户到QQ的授权页面,用户登录并授权后,会重定向到你设置的回调URL,并带上授权码(Authorization Code)。
- 使用授权码请求Access Token。
- 使用Access Token请求用户信息。
代码示例:
import requests
Step 1: Get Authorization Code
def get_authorization_code(client_id, redirect_uri):
authorization_url = f"https://graph.qq.com/oauth2.0/authorize?response_type=code&client_id={client_id}&redirect_uri={redirect_uri}"
print(f"Please go to this URL and authorize the app: {authorization_url}")
Step 2: Exchange Authorization Code for Access Token
def get_access_token(client_id, client_secret, authorization_code, redirect_uri):
token_url = "https://graph.qq.com/oauth2.0/token"
params = {
"grant_type": "authorization_code",
"client_id": client_id,
"client_secret": client_secret,
"code": authorization_code,
"redirect_uri": redirect_uri
}
response = requests.get(token_url, params=params)
return response.json()
Step 3: Get User Info using Access Token
def get_user_info(access_token, openid):
user_info_url = "https://graph.qq.com/user/get_user_info"
params = {
"access_token": access_token,
"oauth_consumer_key": client_id,
"openid": openid
}
response = requests.get(user_info_url, params=params)
return response.json()
Usage Example
client_id = "your_app_id"
client_secret = "your_app_secret"
redirect_uri = "your_redirect_uri"
authorization_code = "obtained_authorization_code"
access_token_response = get_access_token(client_id, client_secret, authorization_code, redirect_uri)
access_token = access_token_response["access_token"]
openid = access_token_response["openid"]
user_info = get_user_info(access_token, openid)
print(user_info)
二、使用第三方库
1、介绍第三方库
一些第三方库可以简化与QQ API的交互。常见的第三方库包括qqbot
等。这些库封装了大部分API调用,使得开发者可以更容易地进行消息发送和获取。
2、安装和使用qqbot库
首先需要安装qqbot
库:
pip install qqbot
然后,使用以下代码进行消息发送和获取:
from qqbot import QQBot, Message
Initialize the bot
bot = QQBot()
Login with your QQ account
bot.login("your_qq_number", "your_password")
Send a message
def send_message(target_qq_number, message):
bot.send(Message(target_qq_number, message))
Get messages
def get_messages():
messages = bot.get_messages()
for message in messages:
print(message.content)
Usage Example
target_qq_number = "target_qq_number"
message = "Hello, this is a test message."
send_message(target_qq_number, message)
get_messages()
三、通过Web Scraping
1、介绍Web Scraping
Web Scraping是一种通过HTTP请求获取网页数据并解析的技术。虽然这种方法不如API接口稳定和安全,但在某些场景下依然有效。
2、使用Selenium进行Web Scraping
Selenium是一个强大的Web Scraping工具,可以模拟用户操作网页。以下是一个使用Selenium登录QQ并获取信息的示例:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
Initialize the WebDriver
driver = webdriver.Chrome()
Open QQ login page
driver.get("https://qzone.qq.com/")
Switch to login iframe
driver.switch_to.frame("login_frame")
Click on account login
driver.find_element_by_id("switcher_plogin").click()
Enter your QQ number and password
qq_number = "your_qq_number"
password = "your_password"
driver.find_element_by_id("u").send_keys(qq_number)
driver.find_element_by_id("p").send_keys(password)
driver.find_element_by_id("login_button").click()
Wait for login to complete
time.sleep(5)
Switch to main frame
driver.switch_to.default_content()
driver.switch_to.frame("app_canvas_frame")
Get user info (example: friends list)
friends_list = driver.find_elements_by_class_name("qz_friend_name")
for friend in friends_list:
print(friend.text)
Close the WebDriver
driver.quit()
四、常见问题和解决方案
1、授权失败
授权失败可能是由于应用的AppID和AppKey配置错误,或者用户未授权。确保在腾讯开放平台正确配置应用,并引导用户完成授权。
2、获取Access Token失败
获取Access Token失败可能是由于授权码已过期或无效。确保在获取授权码后尽快请求Access Token。
3、API调用频率限制
QQ API接口对调用频率有限制,如果频繁调用接口可能会被限制。建议在代码中加入适当的延迟和重试机制。
4、Web Scraping被检测和封禁
使用Web Scraping获取信息时,可能会被网站检测并封禁。可以通过设置User-Agent、加入延迟等方式降低被检测的概率。
五、总结
通过本文的介绍,我们了解了如何通过QQ的API接口、使用第三方库以及通过Web Scraping等方式来发送和获取QQ信息。推荐使用QQ的API接口进行开发,因为它是官方提供的,安全性和可靠性更高。在开发过程中,遇到问题可以参考本文提供的解决方案。希望本文对你有所帮助,祝你开发顺利!
参考文献
- 腾讯开放平台:https://open.qq.com
- qqbot库文档:https://qqbot.readthedocs.io/en/latest/
- Selenium文档:https://selenium-python.readthedocs.io/
相关问答FAQs:
如何使用Python发送QQ信息?
要使用Python发送QQ信息,可以利用第三方库,如requests
或pyqq
,结合QQ的API接口来实现。首先,您需要注册一个QQ账号并获取相应的API权限。接着,您可以通过编写Python脚本,利用HTTP请求向QQ服务器发送消息。具体步骤包括设置请求头、构造消息体,并进行身份验证。
Python发送QQ信息需要哪些库和工具?
在使用Python发送QQ信息时,推荐使用requests
库来处理HTTP请求。此外,可能需要使用json
库来处理消息数据的格式。确保您的Python环境中已安装这些库,可以通过pip install requests
命令进行安装。
如何在Python中获取QQ消息?
获取QQ消息可以通过使用QQ的API接口进行。您需要先获取到相应的API密钥和用户身份验证信息。通过发送GET请求到QQ的消息接口,可以获取到消息数据。解析返回的JSON格式数据后,您就可以在Python中处理和展示这些信息。
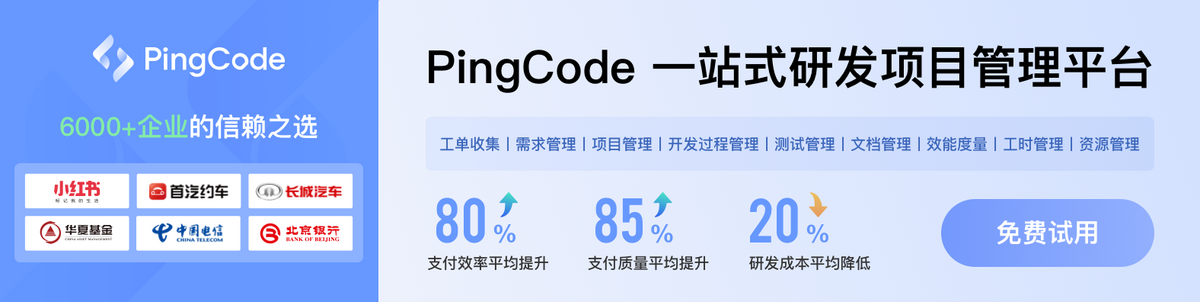