在Python中导入BeautifulSoup库,可以通过安装bs4包并在代码中进行导入。以下是具体步骤:安装bs4库、导入bs4库、使用BeautifulSoup对象解析HTML文档。 其中,安装bs4库是最基础的一步。
一、安装bs4库
在使用BeautifulSoup之前,我们首先需要安装bs4库。可以通过pip命令进行安装。在命令行界面输入以下命令:
pip install beautifulsoup4
这将从Python包索引(PyPI)下载并安装BeautifulSoup库及其依赖项。
二、导入bs4库
安装完成后,我们可以在Python代码中导入BeautifulSoup库。具体的导入方式如下:
from bs4 import BeautifulSoup
通过这行代码,我们成功将BeautifulSoup库导入到我们的Python项目中,接下来我们可以使用它来解析和处理HTML或XML文档。
三、使用BeautifulSoup对象解析HTML文档
导入BeautifulSoup库后,我们可以开始解析HTML文档。以下是一个简单的示例,展示如何使用BeautifulSoup解析一个HTML字符串:
from bs4 import BeautifulSoup
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.prettify())
在上面的代码中,我们首先导入了BeautifulSoup库,然后定义了一个HTML字符串html_doc
,接着使用BeautifulSoup
类创建了一个BeautifulSoup
对象soup
,并指定解析器为html.parser
。最后,我们使用soup.prettify()
方法打印了格式化后的HTML文档。
四、解析HTML内容
1、查找标签和获取内容
使用BeautifulSoup解析HTML文档后,我们可以使用各种方法来查找特定的标签和获取它们的内容。以下是几个常用的方法:
-
查找单个标签:使用
soup.tag
或soup.find()
方法可以查找第一个匹配的标签。例如,查找<title>
标签:title_tag = soup.title
print(title_tag)
print(title_tag.string)
上述代码将输出:
<title>The Dormouse's story</title>
The Dormouse's story
-
查找所有标签:使用
soup.find_all()
方法可以查找所有匹配的标签。例如,查找所有<a>
标签:a_tags = soup.find_all('a')
for tag in a_tags:
print(tag.get('href'))
上述代码将输出:
http://example.com/elsie
http://example.com/lacie
http://example.com/tillie
-
获取标签的属性:使用
tag['attribute']
方式可以获取标签的属性值。例如,获取第一个<a>
标签的href
属性:first_a_tag = soup.find('a')
print(first_a_tag['href'])
上述代码将输出:
http://example.com/elsie
2、使用CSS选择器
除了使用标签名查找标签外,我们还可以使用CSS选择器来查找标签。BeautifulSoup提供了soup.select()
方法来支持CSS选择器。例如:
# 查找所有class为'sister'的<a>标签
sister_tags = soup.select('a.sister')
for tag in sister_tags:
print(tag.get_text())
查找id为link1的标签
link1_tag = soup.select_one('#link1')
print(link1_tag.get_text())
上述代码将输出:
Elsie
Lacie
Tillie
Elsie
五、修改和操作HTML内容
除了解析和查找标签外,BeautifulSoup还允许我们修改和操作HTML文档的内容。
1、修改标签内容
我们可以直接修改标签的内容。例如,将<title>
标签的内容改为"New Title"
:
soup.title.string = "New Title"
print(soup.title)
上述代码将输出:
<title>New Title</title>
2、添加和删除标签
我们可以使用tag.append()
方法向标签中添加新内容,使用tag.decompose()
方法删除标签。例如:
# 添加新标签
new_tag = soup.new_tag('p')
new_tag.string = "New paragraph."
soup.body.append(new_tag)
print(soup.body)
删除标签
soup.p.decompose()
print(soup.body)
上述代码将输出:
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a class="sister" href="http://example.com/elsie" id="link1">Elsie</a>,
<a class="sister" href="http://example.com/lacie" id="link2">Lacie</a> and
<a class="sister" href="http://example.com/tillie" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
<p>New paragraph.</p>
</body>
<body>
<p class="story">Once upon a time there were three little sisters; and their names were
<a class="sister" href="http://example.com/elsie" id="link1">Elsie</a>,
<a class="sister" href="http://example.com/lacie" id="link2">Lacie</a> and
<a class="sister" href="http://example.com/tillie" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
<p>New paragraph.</p>
</body>
六、处理复杂HTML结构
在实际应用中,我们通常需要处理更复杂的HTML结构,以下是一些实用的示例:
1、解析嵌套标签
BeautifulSoup支持解析嵌套标签。例如,获取<p class="story">
标签内的所有<a>
标签:
story_p_tag = soup.find('p', class_='story')
a_tags_in_story = story_p_tag.find_all('a')
for tag in a_tags_in_story:
print(tag.get_text())
上述代码将输出:
Elsie
Lacie
Tillie
2、处理无效HTML
BeautifulSoup能够处理无效或格式不正确的HTML文档,并尝试修复这些错误。例如:
invalid_html = "<html><head><title>Invalid HTML</title></head><body><p>Paragraph without closing tag"
soup = BeautifulSoup(invalid_html, 'html.parser')
print(soup.prettify())
上述代码将输出:
<html>
<head>
<title>
Invalid HTML
</title>
</head>
<body>
<p>
Paragraph without closing tag
</p>
</body>
</html>
七、与其他库结合使用
BeautifulSoup可以与其他库结合使用,以实现更强大的功能。例如,与requests库结合使用,可以方便地从网络上获取HTML文档并进行解析:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
html_content = response.content
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify())
上述代码展示了如何使用requests库获取网页内容,并使用BeautifulSoup进行解析和处理。
八、总结
通过以上内容,我们学习了如何在Python中导入BeautifulSoup库,并使用它解析和处理HTML文档。具体步骤包括安装bs4库、导入BeautifulSoup库、使用BeautifulSoup对象解析HTML文档、查找和获取标签内容、使用CSS选择器、修改和操作HTML内容、处理复杂HTML结构、以及与其他库结合使用。
BeautifulSoup是一个功能强大且易于使用的HTML解析库,通过掌握这些基本操作,我们可以方便地解析和处理各种HTML文档,为我们的网页数据提取和处理工作带来极大的便利。
相关问答FAQs:
如何在Python中安装Beautiful Soup 4 (bs4)?
要在Python中使用Beautiful Soup 4,您首先需要安装它。可以通过使用pip命令来完成安装。在命令行或终端中输入以下命令:
pip install beautifulsoup4
确保您的Python环境已经正确设置,并且pip已安装。如果您使用的是Anaconda,可以在Anaconda Prompt中运行相同的命令。
使用Beautiful Soup 4时,通常需要导入哪些库?
在使用Beautiful Soup 4进行网页解析时,您通常需要导入bs4
模块以及requests
库。requests
库用于从网页获取内容,而bs4
则用于解析这些内容。以下是一个简单的示例:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
通过这种方式,您可以获取网页内容并进行解析。
在导入bs4时,如何处理ImportError?
如果在导入bs4时遇到ImportError,通常是因为没有正确安装该库。您可以通过以下步骤来解决这个问题:
- 确认是否使用了正确的Python环境,特别是在使用虚拟环境或Anaconda时。
- 使用pip重新安装bs4,确保安装过程中没有错误。
- 查看Python版本,确保所用版本与bs4兼容。
通过这些步骤,您应该能顺利导入bs4并开始使用。
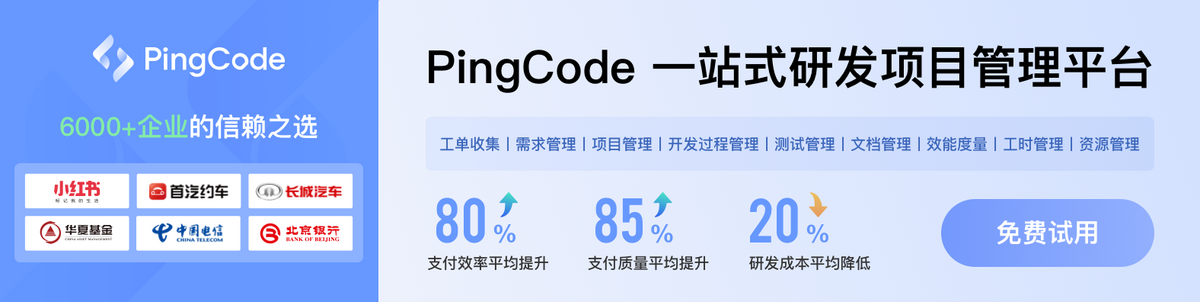