使用Python下载文章有多种方法,主要包括利用HTTP请求、解析HTML内容、利用API等。首先,你可以使用requests库发送HTTP请求获取网页内容,然后使用BeautifulSoup库解析HTML内容提取文章。其次,如果网站提供API,可以直接调用API获取文章内容。这些方法都需要确保遵守相关网站的使用政策和版权规定。
一、使用requests和BeautifulSoup下载文章
使用requests和BeautifulSoup库是下载文章的常见方法。requests库用于发送HTTP请求获取网页内容,BeautifulSoup库用于解析HTML内容并提取所需的文章部分。
1、安装必要的库
首先,确保安装了requests和BeautifulSoup库:
pip install requests
pip install beautifulsoup4
2、发送HTTP请求并解析HTML
使用requests库发送HTTP请求获取网页内容:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/article'
response = requests.get(url)
if response.status_code == 200:
page_content = response.text
else:
print(f"Failed to retrieve the page. Status code: {response.status_code}")
3、解析HTML内容提取文章
使用BeautifulSoup库解析HTML内容并提取文章部分:
soup = BeautifulSoup(page_content, 'html.parser')
article = soup.find('div', class_='article-content')
if article:
print(article.get_text())
else:
print("Article content not found")
二、使用API下载文章
有些网站提供API,可以直接调用API获取文章内容。这种方法通常更简单和可靠。
1、查找API文档
首先,查找目标网站的API文档,了解如何调用API获取文章内容。例如,某些新闻网站或博客平台可能提供API来获取文章内容。
2、发送API请求
使用requests库发送API请求:
import requests
api_url = 'https://api.example.com/articles'
params = {
'article_id': '12345'
}
response = requests.get(api_url, params=params)
if response.status_code == 200:
article_data = response.json()
print(article_data['content'])
else:
print(f"Failed to retrieve the article. Status code: {response.status_code}")
三、处理动态加载的网页
有些网页内容是通过JavaScript动态加载的,requests库无法直接获取这些内容。可以使用Selenium库模拟浏览器行为,获取动态加载的内容。
1、安装Selenium库和浏览器驱动
首先,安装Selenium库并下载相应的浏览器驱动(例如ChromeDriver):
pip install selenium
2、使用Selenium获取动态内容
使用Selenium库模拟浏览器行为,获取动态加载的内容:
from selenium import webdriver
from selenium.webdriver.common.by import By
url = 'https://example.com/dynamic-article'
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
article_element = driver.find_element(By.CLASS_NAME, 'article-content')
print(article_element.text)
driver.quit()
四、处理不同格式的文章
文章内容可能以不同的格式呈现,如纯文本、HTML、Markdown等。需要根据具体情况进行处理。
1、处理纯文本文章
对于纯文本文章,可以直接读取和保存内容:
article_text = article.get_text()
with open('article.txt', 'w', encoding='utf-8') as file:
file.write(article_text)
2、处理HTML格式的文章
对于HTML格式的文章,可以使用BeautifulSoup进一步解析和处理:
article_html = article.prettify()
with open('article.html', 'w', encoding='utf-8') as file:
file.write(article_html)
3、处理Markdown格式的文章
对于Markdown格式的文章,可以使用markdown库进行转换和处理:
import markdown
article_markdown = markdown.markdown(article_html)
with open('article.md', 'w', encoding='utf-8') as file:
file.write(article_markdown)
五、处理分页文章
有些文章内容可能分布在多个分页上,需要处理分页逻辑。
1、解析分页链接
首先,解析分页链接,获取所有分页的URL:
pagination_links = soup.find_all('a', class_='pagination-link')
page_urls = [link['href'] for link in pagination_links]
2、循环获取每一分页内容
循环获取每一分页内容,并合并到一起:
full_article = ''
for page_url in page_urls:
response = requests.get(page_url)
if response.status_code == 200:
page_soup = BeautifulSoup(response.text, 'html.parser')
page_article = page_soup.find('div', class_='article-content')
if page_article:
full_article += page_article.get_text()
with open('full_article.txt', 'w', encoding='utf-8') as file:
file.write(full_article)
六、处理带有图片的文章
有些文章中包含图片,需要同时下载图片并保存到本地。
1、解析图片链接
首先,解析文章中的图片链接:
images = article.find_all('img')
image_urls = [img['src'] for img in images]
2、下载并保存图片
循环下载每一图片,并保存到本地:
import os
os.makedirs('images', exist_ok=True)
for i, image_url in enumerate(image_urls):
image_response = requests.get(image_url)
if image_response.status_code == 200:
with open(f'images/image_{i}.jpg', 'wb') as file:
file.write(image_response.content)
七、处理需要登录的网站
有些网站需要登录才能访问文章内容,可以使用requests库的会话(session)功能处理登录。
1、发送登录请求
首先,发送登录请求,获取会话:
login_url = 'https://example.com/login'
credentials = {
'username': 'your_username',
'password': 'your_password'
}
session = requests.Session()
login_response = session.post(login_url, data=credentials)
if login_response.status_code == 200:
print("Login successful")
else:
print(f"Login failed. Status code: {login_response.status_code}")
2、使用会话获取文章内容
使用会话获取文章内容:
article_url = 'https://example.com/article'
article_response = session.get(article_url)
if article_response.status_code == 200:
article_content = article_response.text
article_soup = BeautifulSoup(article_content, 'html.parser')
article = article_soup.find('div', class_='article-content')
if article:
print(article.get_text())
else:
print("Article content not found")
else:
print(f"Failed to retrieve the article. Status code: {article_response.status_code}")
八、处理防爬虫机制
有些网站使用各种防爬虫机制,需要采取一些措施绕过这些机制。
1、模拟浏览器请求头
模拟浏览器请求头,有助于绕过简单的防爬虫机制:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get(url, headers=headers)
2、使用代理
使用代理可以隐藏真实的IP地址,绕过某些IP限制:
proxies = {
'http': 'http://your_proxy:port',
'https': 'http://your_proxy:port'
}
response = requests.get(url, headers=headers, proxies=proxies)
3、处理CAPTCHA
对于需要处理CAPTCHA的网站,可以使用第三方服务解决CAPTCHA,或手动处理CAPTCHA:
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver.get('https://example.com')
captcha_element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, 'captcha'))
)
这里可以手动处理CAPTCHA,或使用第三方服务自动解决CAPTCHA
九、保存文章内容到数据库
可以将下载的文章内容保存到数据库中,便于后续查询和分析。
1、安装数据库驱动
首先,安装相应的数据库驱动,例如MySQL:
pip install mysql-connector-python
2、连接数据库并保存文章内容
连接数据库并保存文章内容:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
cursor = connection.cursor()
article_title = 'Sample Article'
article_content = article.get_text()
cursor.execute(
"INSERT INTO articles (title, content) VALUES (%s, %s)",
(article_title, article_content)
)
connection.commit()
cursor.close()
connection.close()
十、总结
通过以上方法,可以使用Python下载各种类型的文章内容,并进行相应的处理和保存。在实际应用中,需要根据具体情况选择合适的方法,并确保遵守相关网站的使用政策和版权规定。使用requests和BeautifulSoup库是最常见的方法,处理动态加载内容时可以使用Selenium库,处理需要登录的网站时可以使用会话功能,处理防爬虫机制时可以模拟浏览器请求头或使用代理。此外,还可以将下载的文章内容保存到数据库中,便于后续查询和分析。
相关问答FAQs:
如何使用Python自动下载特定网站上的文章?
使用Python下载特定网站上的文章,通常可以使用库如Requests和BeautifulSoup。首先,使用Requests库发送HTTP请求以获取网页内容。接着,利用BeautifulSoup解析HTML,并提取所需的文章内容。最后,将提取的内容保存为文本文件或其他格式。确保遵守网站的爬虫规则和使用条款。
在下载文章时如何处理反爬虫机制?
许多网站会采取反爬虫措施来阻止自动化脚本访问其内容。为了有效下载文章,可以尝试设置请求头,模拟真实用户的浏览器行为。此外,使用随机的请求间隔和代理IP,可以进一步降低被识别为爬虫的风险。同时,遵循robots.txt文件中的爬虫规则也是非常重要的。
怎样将下载的文章格式化为PDF或其他文件格式?
下载的文章可以使用Python的第三方库如ReportLab或PDFKit进行格式化。将提取到的文本内容转换为PDF文件时,可以自定义布局、字体和样式。除了PDF,还可以使用Markdown或HTML格式化文章,便于后续的处理和发布。确保在转换过程中保持文章的原始结构和可读性。
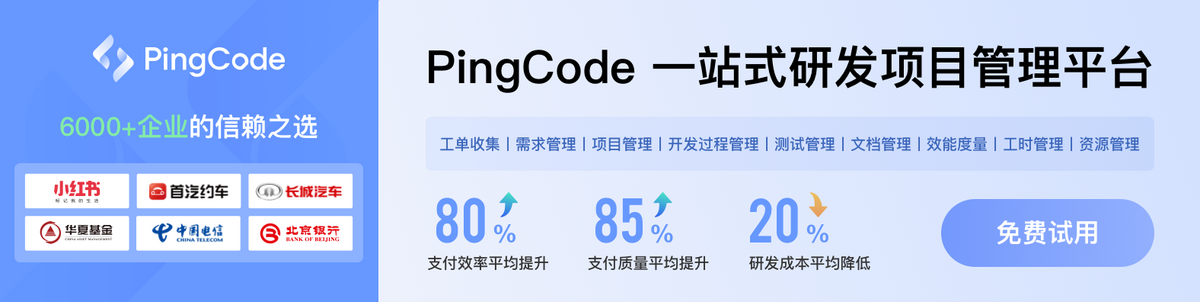