一、如何判断txt能否剪切
在Python中判断一个TXT文件是否可以剪切,需要检查文件的存在性、文件的读写权限、目标路径的可写性。其中,判断文件的存在性和读写权限是最基本的步骤,检查目标路径的可写性也是非常重要的一环。确保所有这些条件都满足后,才可以安全地执行剪切操作。接下来,我们详细描述一下如何判断文件是否存在。
判断文件是否存在:在Python中,可以使用os.path.exists()
函数来判断文件是否存在。这个函数会返回一个布尔值,如果文件存在返回True,否则返回False。通过这个函数,我们可以确定目标文件是否存在,从而决定是否可以继续进行剪切操作。
import os
def file_exists(file_path):
return os.path.exists(file_path)
如果文件存在,我们可以继续进行读写权限和目标路径的检查。
二、文件存在性检查
在进行任何文件操作之前,首先要确保文件存在。可以使用os.path.exists()
函数来完成这一检查。
import os
file_path = 'example.txt'
if os.path.exists(file_path):
print(f"The file {file_path} exists.")
else:
print(f"The file {file_path} does not exist.")
这个函数检查指定路径的文件是否存在,并返回一个布尔值。
三、文件读写权限检查
除了文件的存在性之外,还需要检查文件的读写权限。可以使用os.access()
函数来完成这一检查。
import os
file_path = 'example.txt'
if os.access(file_path, os.R_OK):
print(f"The file {file_path} is readable.")
else:
print(f"The file {file_path} is not readable.")
if os.access(file_path, os.W_OK):
print(f"The file {file_path} is writable.")
else:
print(f"The file {file_path} is not writable.")
这个函数检查文件是否具有读(os.R_OK
)和写(os.W_OK
)权限。
四、目标路径的可写性检查
在剪切操作中,目标路径的可写性也是一个重要的因素。可以使用os.access()
函数来检查目标路径的可写性。
import os
target_path = '/path/to/target/directory'
if os.access(target_path, os.W_OK):
print(f"The target path {target_path} is writable.")
else:
print(f"The target path {target_path} is not writable.")
这个函数检查目标路径是否具有写权限。
五、综合判断文件能否剪切
结合以上步骤,可以综合判断文件是否可以剪切。
import os
def can_cut_file(file_path, target_path):
if not os.path.exists(file_path):
print(f"The file {file_path} does not exist.")
return False
if not os.access(file_path, os.R_OK):
print(f"The file {file_path} is not readable.")
return False
if not os.access(file_path, os.W_OK):
print(f"The file {file_path} is not writable.")
return False
if not os.access(target_path, os.W_OK):
print(f"The target path {target_path} is not writable.")
return False
return True
file_path = 'example.txt'
target_path = '/path/to/target/directory'
if can_cut_file(file_path, target_path):
print(f"The file {file_path} can be cut to {target_path}.")
else:
print(f"The file {file_path} cannot be cut to {target_path}.")
六、文件剪切操作
在确定文件可以剪切之后,可以使用shutil.move()
函数来完成剪切操作。
import os
import shutil
def cut_file(file_path, target_path):
if can_cut_file(file_path, target_path):
shutil.move(file_path, target_path)
print(f"The file {file_path} has been cut to {target_path}.")
else:
print(f"The file {file_path} cannot be cut to {target_path}.")
file_path = 'example.txt'
target_path = '/path/to/target/directory'
cut_file(file_path, target_path)
七、错误处理
在实际操作中,可能会遇到各种意外情况,因此需要进行错误处理。例如,可以使用try-except
语句来捕获和处理文件操作中的异常。
import os
import shutil
def cut_file(file_path, target_path):
try:
if can_cut_file(file_path, target_path):
shutil.move(file_path, target_path)
print(f"The file {file_path} has been cut to {target_path}.")
else:
print(f"The file {file_path} cannot be cut to {target_path}.")
except Exception as e:
print(f"An error occurred while cutting the file: {e}")
file_path = 'example.txt'
target_path = '/path/to/target/directory'
cut_file(file_path, target_path)
八、日志记录
在实际应用中,记录日志是非常重要的。可以使用Python的logging
模块来记录文件操作的相关信息。
import os
import shutil
import logging
logging.basicConfig(filename='file_operations.log', level=logging.INFO)
def cut_file(file_path, target_path):
try:
if can_cut_file(file_path, target_path):
shutil.move(file_path, target_path)
logging.info(f"The file {file_path} has been cut to {target_path}.")
else:
logging.warning(f"The file {file_path} cannot be cut to {target_path}.")
except Exception as e:
logging.error(f"An error occurred while cutting the file: {e}")
file_path = 'example.txt'
target_path = '/path/to/target/directory'
cut_file(file_path, target_path)
九、文件操作的安全性
在进行文件操作时,安全性是一个非常重要的考虑因素。确保文件操作不会对系统造成破坏,尤其是在处理敏感文件时。
- 备份文件:在进行剪切等操作之前,可以先备份文件,以防止意外丢失。
import shutil
def backup_file(file_path, backup_path):
shutil.copy2(file_path, backup_path)
print(f"The file {file_path} has been backed up to {backup_path}.")
file_path = 'example.txt'
backup_path = 'example_backup.txt'
backup_file(file_path, backup_path)
-
权限检查:确保只有授权用户才能进行敏感文件操作,避免未经授权的访问和修改。
-
日志记录:记录所有文件操作,便于日后审计和追踪。
十、文件操作的效率
在处理大量文件时,效率是一个关键问题。可以考虑使用以下方法来提高文件操作的效率:
- 批量处理:一次性处理多个文件,减少重复操作。
import os
import shutil
def cut_files(file_paths, target_path):
for file_path in file_paths:
try:
if can_cut_file(file_path, target_path):
shutil.move(file_path, target_path)
print(f"The file {file_path} has been cut to {target_path}.")
else:
print(f"The file {file_path} cannot be cut to {target_path}.")
except Exception as e:
print(f"An error occurred while cutting the file {file_path}: {e}")
file_paths = ['example1.txt', 'example2.txt', 'example3.txt']
target_path = '/path/to/target/directory'
cut_files(file_paths, target_path)
- 多线程处理:使用多线程来并行处理多个文件,进一步提高效率。
import os
import shutil
import threading
def cut_file_thread(file_path, target_path):
try:
if can_cut_file(file_path, target_path):
shutil.move(file_path, target_path)
print(f"The file {file_path} has been cut to {target_path}.")
else:
print(f"The file {file_path} cannot be cut to {target_path}.")
except Exception as e:
print(f"An error occurred while cutting the file {file_path}: {e}")
file_paths = ['example1.txt', 'example2.txt', 'example3.txt']
target_path = '/path/to/target/directory'
threads = []
for file_path in file_paths:
thread = threading.Thread(target=cut_file_thread, args=(file_path, target_path))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
十一、总结
综上所述,判断一个TXT文件是否可以剪切,涉及到多个步骤和考虑因素。首先,需要确保文件的存在性和读写权限,其次检查目标路径的可写性。在确保所有条件都满足后,可以使用shutil.move()
函数来执行剪切操作。同时,注意进行错误处理和日志记录,确保文件操作的安全性和可追溯性。在处理大量文件时,可以考虑使用批量处理和多线程处理来提高操作效率。通过以上方法,可以有效地判断和执行TXT文件的剪切操作。
相关问答FAQs:
如何判断一个txt文件是否可以被剪切?
在Python中,可以通过检查文件的属性来判断一个txt文件是否可以被剪切。通常情况下,如果文件没有被其他程序占用,并且你对该文件有足够的权限(如读、写权限),那么它就可以被剪切。可以使用os
模块中的access()
函数来验证这些权限。
如何处理被占用的txt文件?
如果一个txt文件被其他程序占用,通常会导致剪切操作失败。你可以使用try...except
语句来捕捉异常并处理错误。确保在剪切文件之前,先检查是否有程序正在使用该文件。如果需要,可以提示用户关闭相关程序以释放文件。
在剪切txt文件时需要注意哪些事项?
在进行剪切操作时,确保目标位置有足够的空间来容纳文件,并且在剪切之前进行必要的备份,以防文件丢失或损坏。此外,考虑到文件名的唯一性,避免在目标位置出现同名文件。如果目标路径下已有同名文件,可以选择覆盖、重命名或提示用户进行选择。
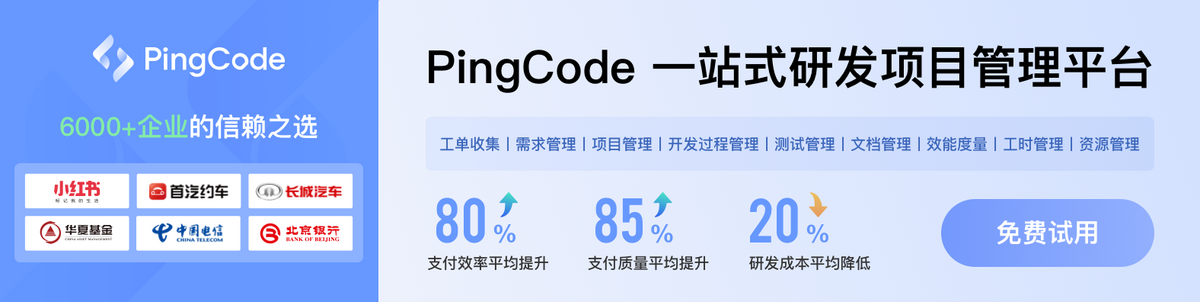