Python 可以通过编写程序实现生日祝福的发送、个性化的祝福内容生成、自动邮件发送等功能。主要方法包括:用Python生成个性化生日祝福、利用Python发送电子邮件、创建图形用户界面(GUI)来显示生日祝福等。 其中,利用Python发送电子邮件是一个非常实用且广泛应用的方法。通过Python的smtplib和email库,用户可以编写脚本自动发送个性化的生日祝福邮件。
一、用Python生成个性化生日祝福
Python可以通过编写简单的脚本来生成个性化的生日祝福。我们可以使用随机库来生成不同的祝福语,或者从预设的祝福语列表中随机选择一个。
import random
def generate_birthday_message(name):
messages = [
f"Happy Birthday, {name}! Wishing you a day filled with love and joy.",
f"Dear {name}, may your birthday be as wonderful and special as you are!",
f"{name}, happy birthday! Hope your day is full of fun and celebration."
]
return random.choice(messages)
name = "Alice"
print(generate_birthday_message(name))
二、利用Python发送电子邮件
利用Python发送电子邮件,我们通常使用smtplib和email库。这两个库可以帮助我们连接到邮件服务器并发送格式化的电子邮件。以下是一个基本的示例,展示如何发送生日祝福邮件。
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_birthday_email(to_email, subject, message):
from_email = "your_email@example.com"
from_password = "your_password"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(message, 'plain'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(from_email, from_password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
server.quit()
print("Email sent successfully")
except Exception as e:
print(f"Failed to send email: {e}")
name = "Alice"
to_email = "alice@example.com"
subject = "Happy Birthday!"
message = generate_birthday_message(name)
send_birthday_email(to_email, subject, message)
三、创建图形用户界面(GUI)来显示生日祝福
如果你希望创建一个更加互动的生日祝福体验,可以使用Python的Tkinter库来创建一个图形用户界面(GUI)。以下是一个简单的示例,展示如何创建一个窗口来显示生日祝福。
import tkinter as tk
from tkinter import messagebox
def show_birthday_message():
name = entry_name.get()
message = generate_birthday_message(name)
messagebox.showinfo("Birthday Message", message)
root = tk.Tk()
root.title("Birthday Wishes")
label_name = tk.Label(root, text="Enter the name:")
label_name.pack()
entry_name = tk.Entry(root)
entry_name.pack()
button_show = tk.Button(root, text="Show Birthday Message", command=show_birthday_message)
button_show.pack()
root.mainloop()
四、使用数据库存储生日信息
为了实现自动化的生日祝福发送,我们可以将生日信息存储在数据库中。以下示例使用SQLite数据库来存储和检索生日信息。
import sqlite3
def create_database():
conn = sqlite3.connect('birthdays.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS birthdays
(id INTEGER PRIMARY KEY, name TEXT, email TEXT, birthday DATE)''')
conn.commit()
conn.close()
def add_birthday(name, email, birthday):
conn = sqlite3.connect('birthdays.db')
c = conn.cursor()
c.execute("INSERT INTO birthdays (name, email, birthday) VALUES (?, ?, ?)", (name, email, birthday))
conn.commit()
conn.close()
def get_today_birthdays():
conn = sqlite3.connect('birthdays.db')
c = conn.cursor()
c.execute("SELECT name, email FROM birthdays WHERE strftime('%m-%d', birthday) = strftime('%m-%d', 'now')")
birthdays = c.fetchall()
conn.close()
return birthdays
create_database()
add_birthday("Alice", "alice@example.com", "1990-01-01")
today_birthdays = get_today_birthdays()
for name, email in today_birthdays:
message = generate_birthday_message(name)
send_birthday_email(email, "Happy Birthday!", message)
五、定时任务自动发送生日祝福
为了让程序每天自动检查并发送生日祝福,我们可以使用Python的定时任务库,例如schedule。
import schedule
import time
def job():
today_birthdays = get_today_birthdays()
for name, email in today_birthdays:
message = generate_birthday_message(name)
send_birthday_email(email, "Happy Birthday!", message)
schedule.every().day.at("09:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
六、使用模板生成个性化祝福邮件
我们可以使用Jinja2模板引擎来生成更加个性化和美观的祝福邮件内容。
from jinja2 import Template
def generate_birthday_message_with_template(name):
template = Template("""
<html>
<body>
<h1>Happy Birthday, {{ name }}!</h1>
<p>Wishing you a day filled with love and joy.</p>
</body>
</html>
""")
return template.render(name=name)
def send_birthday_email_with_template(to_email, subject, message):
from_email = "your_email@example.com"
from_password = "your_password"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(message, 'html'))
try:
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(from_email, from_password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
server.quit()
print("Email sent successfully")
except Exception as e:
print(f"Failed to send email: {e}")
name = "Alice"
to_email = "alice@example.com"
subject = "Happy Birthday!"
message = generate_birthday_message_with_template(name)
send_birthday_email_with_template(to_email, subject, message)
七、通过API发送生日祝福短信
除了发送电子邮件,我们还可以使用短信API来发送生日祝福短信。以下示例使用Twilio API来发送短信。
from twilio.rest import Client
def send_birthday_sms(to_phone, message):
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
try:
message = client.messages.create(
body=message,
from_='+1234567890',
to=to_phone
)
print("SMS sent successfully")
except Exception as e:
print(f"Failed to send SMS: {e}")
name = "Alice"
to_phone = "+1234567890"
message = generate_birthday_message(name)
send_birthday_sms(to_phone, message)
八、结合社交媒体API发送生日祝福
利用社交媒体API,我们可以在用户的社交媒体账号上发布生日祝福。以下是使用Facebook Graph API发布生日祝福的示例。
import requests
def post_facebook_birthday_message(access_token, user_id, message):
url = f"https://graph.facebook.com/{user_id}/feed"
payload = {
'message': message,
'access_token': access_token
}
try:
response = requests.post(url, data=payload)
if response.status_code == 200:
print("Post published successfully")
else:
print(f"Failed to publish post: {response.status_code}")
except Exception as e:
print(f"Error: {e}")
access_token = "your_facebook_access_token"
user_id = "user_facebook_id"
name = "Alice"
message = generate_birthday_message(name)
post_facebook_birthday_message(access_token, user_id, message)
九、使用语音合成技术发送生日祝福
Python还可以结合语音合成技术(如Google Text-to-Speech)发送语音生日祝福。
from gtts import gTTS
import os
def generate_birthday_message_audio(name):
message = generate_birthday_message(name)
tts = gTTS(text=message, lang='en')
audio_file = f"{name}_birthday_message.mp3"
tts.save(audio_file)
return audio_file
name = "Alice"
audio_file = generate_birthday_message_audio(name)
os.system(f"mpg321 {audio_file}")
十、结合云服务实现更复杂的生日祝福系统
为了实现更复杂的生日祝福系统,我们可以结合云服务(如AWS、Google Cloud等)来处理数据库、消息队列、邮件服务等。
import boto3
from botocore.exceptions import NoCredentialsError
def send_birthday_email_with_aws(to_email, subject, message):
client = boto3.client('ses', region_name='us-east-1')
try:
response = client.send_email(
Source='your_email@example.com',
Destination={
'ToAddresses': [to_email],
},
Message={
'Subject': {
'Data': subject,
},
'Body': {
'Text': {
'Data': message,
},
},
}
)
print("Email sent successfully")
except NoCredentialsError:
print("Credentials not available")
name = "Alice"
to_email = "alice@example.com"
subject = "Happy Birthday!"
message = generate_birthday_message(name)
send_birthday_email_with_aws(to_email, subject, message)
通过以上方法,我们可以利用Python实现各种形式的生日祝福,从简单的文本生成到复杂的自动化系统,满足不同场景的需求。希望这些示例能够帮助你更好地利用Python进行生日祝福的发送。
相关问答FAQs:
如何使用Python生成个性化的生日祝福?
使用Python生成个性化的生日祝福可以通过创建一个简单的程序来实现。你可以让用户输入他们的姓名和年龄,然后根据这些信息生成独特的祝福语。例如,可以使用字符串格式化将用户的姓名融入到祝福语中。结合随机祝福语库,程序可以随机选择祝福语,使每次的祝福都充满新意。
Python支持哪些库来创建生日祝福应用?
在Python中,有几个库可以帮助你创建生日祝福应用。tkinter
可以用来制作简单的图形用户界面,使用户输入信息更加友好。random
库可以用来随机选择祝福语,而datetime
库则可以用来计算用户的年龄或判断是否是生日,提升应用的智能化水平。
如何将生日祝福程序与社交媒体集成?
为了将生日祝福程序与社交媒体集成,你可以使用社交媒体的API,比如Twitter或Facebook API。通过这些接口,用户能够直接分享他们的生日祝福到社交媒体平台。需要注意的是,集成时必须遵循社交媒体的开发者政策,并确保用户的隐私和数据安全。
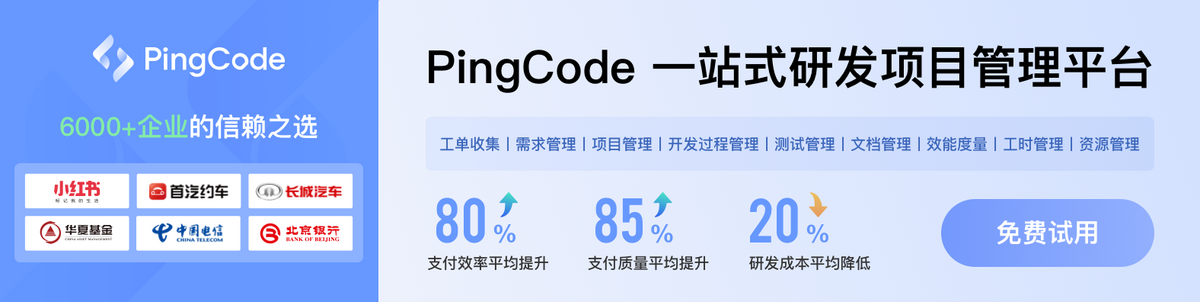