要在Python界面中控制PLC(可编程逻辑控制器),可以使用一些特定的库和工具。这些库和工具包括pyModbus、pycomm3、snap7等。通过使用这些库,您可以实现PLC的连接、读取和写入数据,完成对PLC的控制、简化与PLC通信的复杂性。以下是详细描述如何使用其中一个库snap7来控制PLC的步骤。
一、准备工作
在开始之前,确保您已经安装了snap7库。您可以使用以下命令来安装:
pip install python-snap7
此外,您还需要一个支持snap7的PLC设备,以及PLC的网络连接信息(如IP地址、端口等)。
二、snap7库简介
snap7是一个开源的S7协议库,支持与西门子S7系列PLC进行通信。通过snap7库,您可以实现与PLC的数据交换、控制PLC的状态、读取和写入PLC中的数据块等功能。
三、连接PLC
首先,我们需要创建一个连接到PLC的客户端对象,并连接到PLC。以下是实现连接的示例代码:
import snap7
from snap7.util import *
from snap7.types import *
def connect_to_plc(ip, rack, slot):
client = snap7.client.Client()
client.connect(ip, rack, slot)
return client
连接到PLC
plc_ip = '192.168.0.1'
rack = 0
slot = 1
client = connect_to_plc(plc_ip, rack, slot)
在上述代码中,我们使用了snap7.client.Client()创建了一个PLC客户端对象,并通过connect()方法连接到PLC。连接时需要提供PLC的IP地址、机架号和插槽号。
四、读取PLC数据
连接成功后,我们可以读取PLC中的数据。以下是一个读取PLC数据块的示例代码:
def read_data_block(client, db_number, start, size):
data = client.db_read(db_number, start, size)
return data
读取数据块
db_number = 1
start = 0
size = 4
data = read_data_block(client, db_number, start, size)
print("读取的数据:", data)
在上述代码中,db_read()方法用于读取指定数据块中的数据。需要提供数据块号(db_number)、起始地址(start)和读取的数据大小(size)。
五、写入PLC数据
除了读取数据,我们还可以向PLC中写入数据。以下是一个写入PLC数据块的示例代码:
def write_data_block(client, db_number, start, data):
client.db_write(db_number, start, data)
写入数据块
db_number = 1
start = 0
data = bytearray([0, 1, 2, 3])
write_data_block(client, db_number, start, data)
print("数据已写入")
在上述代码中,db_write()方法用于向指定数据块写入数据。需要提供数据块号(db_number)、起始地址(start)和要写入的数据(data)。
六、控制PLC状态
我们还可以控制PLC的运行状态,如启动和停止PLC。以下是控制PLC状态的示例代码:
def plc_start(client):
client.plc_hot_start()
def plc_stop(client):
client.plc_stop()
启动PLC
plc_start(client)
print("PLC已启动")
停止PLC
plc_stop(client)
print("PLC已停止")
在上述代码中,plc_hot_start()方法用于启动PLC,plc_stop()方法用于停止PLC。
七、关闭连接
完成对PLC的操作后,记得关闭与PLC的连接。以下是关闭连接的示例代码:
def disconnect_from_plc(client):
client.disconnect()
关闭连接
disconnect_from_plc(client)
print("连接已关闭")
八、错误处理
在与PLC通信的过程中,可能会遇到各种错误。为了提高程序的健壮性,我们需要对可能发生的错误进行处理。以下是一个包含错误处理的示例代码:
import snap7
from snap7.util import *
from snap7.types import *
def connect_to_plc(ip, rack, slot):
client = snap7.client.Client()
try:
client.connect(ip, rack, slot)
except snap7.snap7exceptions.Snap7Exception as e:
print("连接失败:", e)
return client
def read_data_block(client, db_number, start, size):
try:
data = client.db_read(db_number, start, size)
except snap7.snap7exceptions.Snap7Exception as e:
print("读取失败:", e)
data = None
return data
def write_data_block(client, db_number, start, data):
try:
client.db_write(db_number, start, data)
except snap7.snap7exceptions.Snap7Exception as e:
print("写入失败:", e)
def plc_start(client):
try:
client.plc_hot_start()
except snap7.snap7exceptions.Snap7Exception as e:
print("启动失败:", e)
def plc_stop(client):
try:
client.plc_stop()
except snap7.snap7exceptions.Snap7Exception as e:
print("停止失败:", e)
def disconnect_from_plc(client):
try:
client.disconnect()
except snap7.snap7exceptions.Snap7Exception as e:
print("断开连接失败:", e)
使用示例
plc_ip = '192.168.0.1'
rack = 0
slot = 1
client = connect_to_plc(plc_ip, rack, slot)
if client.get_connected():
data = read_data_block(client, 1, 0, 4)
if data is not None:
print("读取的数据:", data)
write_data_block(client, 1, 0, bytearray([0, 1, 2, 3]))
print("数据已写入")
plc_start(client)
print("PLC已启动")
plc_stop(client)
print("PLC已停止")
disconnect_from_plc(client)
print("连接已关闭")
else:
print("未能连接到PLC")
在上述代码中,我们对每个操作都添加了try-except块,以捕获可能的异常,并进行适当的处理。
九、总结
通过使用snap7库,您可以在Python界面中轻松地控制PLC,实现与PLC的数据交换和状态控制。在实际应用中,您可以根据具体需求,结合上述示例代码,编写自己的PLC控制程序。关键在于理解如何连接PLC、读取和写入数据、控制PLC状态,并处理可能的错误。通过不断实践和优化,您可以实现更加稳定和高效的PLC控制系统。
相关问答FAQs:
如何使用Python与PLC进行通信?
Python可以通过多种协议与PLC进行通信,例如Modbus、Ethernet/IP等。可以使用第三方库如pyModbus、pycomm3等,这些库提供了与不同类型PLC的接口。用户需根据所使用PLC的通信协议选择合适的库,并编写Python代码来读取和写入PLC的数据。
在Python中如何处理PLC的数据读取和写入?
在Python中处理PLC数据时,通常会使用特定的函数来进行数据的读取和写入。例如,使用pyModbus库时,可以使用read_holding_registers()
来读取寄存器数据,而使用write_register()
可以写入数据。确保在操作时注意数据类型的匹配及错误处理,以确保数据的准确性。
Python控制PLC的应用场景有哪些?
Python控制PLC的应用场景非常广泛,包括工业自动化、设备监控、数据采集和分析等。在生产线中,Python可以实现对PLC的实时监控,自动化设备的控制,以及将PLC的数据与云平台或数据库进行集成,以便进行更深入的数据分析和决策支持。
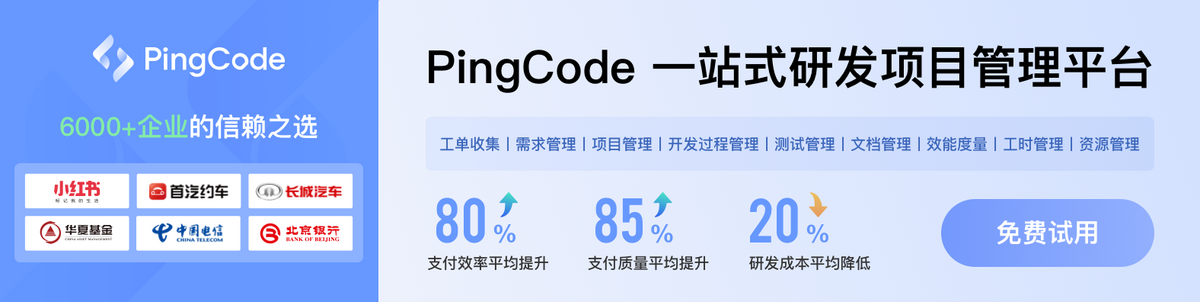