Python接口测试的方法包括使用Python的requests库、编写测试用例、使用pytest进行测试自动化、编写测试报告。下面将详细介绍如何实现其中的一点:使用requests库进行接口测试。
requests库是Python中一个非常常用的库,用于发送HTTP请求。通过requests库,开发者可以方便地模拟HTTP请求,获取服务器返回的响应,用于接口测试。下面是一个使用requests库进行接口测试的示例:
import requests
def test_get():
url = 'http://example.com/api/resource'
response = requests.get(url)
assert response.status_code == 200
assert 'expected_data' in response.json()
def test_post():
url = 'http://example.com/api/resource'
data = {
'key1': 'value1',
'key2': 'value2'
}
response = requests.post(url, json=data)
assert response.status_code == 201
assert response.json()['key1'] == 'value1'
在这个示例中,我们定义了两个测试函数:test_get
和test_post
。test_get
函数发送一个GET请求,检查响应状态码是否为200,并验证响应数据中是否包含预期数据。test_post
函数发送一个POST请求,检查响应状态码是否为201,并验证响应数据中的内容是否正确。
一、使用requests库进行接口测试
1. requests库的安装和基本用法
requests库需要通过pip进行安装,可以使用以下命令进行安装:
pip install requests
安装完成后,可以通过以下代码进行基本的HTTP请求操作:
import requests
GET请求
response = requests.get('http://example.com')
print(response.status_code)
print(response.text)
POST请求
data = {'key': 'value'}
response = requests.post('http://example.com', json=data)
print(response.status_code)
print(response.json())
2. 使用requests库发送不同类型的请求
requests库支持多种HTTP请求方法,包括GET、POST、PUT、DELETE等。下面是一些常见的请求方法的示例:
import requests
GET请求
response = requests.get('http://example.com/api/resource')
print(response.status_code)
print(response.json())
POST请求
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('http://example.com/api/resource', json=data)
print(response.status_code)
print(response.json())
PUT请求
data = {'key1': 'new_value1'}
response = requests.put('http://example.com/api/resource/1', json=data)
print(response.status_code)
print(response.json())
DELETE请求
response = requests.delete('http://example.com/api/resource/1')
print(response.status_code)
3. 处理请求的参数和头信息
在实际的接口测试中,常常需要在请求中添加参数和头信息。requests库支持通过params参数和headers参数来添加这些信息:
import requests
GET请求,添加查询参数
params = {'key1': 'value1', 'key2': 'value2'}
response = requests.get('http://example.com/api/resource', params=params)
print(response.status_code)
print(response.json())
POST请求,添加头信息
headers = {'Authorization': 'Bearer your_token'}
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('http://example.com/api/resource', json=data, headers=headers)
print(response.status_code)
print(response.json())
二、编写测试用例
编写测试用例是接口测试的核心步骤。一个好的测试用例应该覆盖接口的各个方面,包括正常情况、异常情况和边界情况。下面是一些编写测试用例的示例:
1. 正常情况的测试用例
import requests
def test_get_resource():
url = 'http://example.com/api/resource'
response = requests.get(url)
assert response.status_code == 200
assert 'expected_data' in response.json()
def test_create_resource():
url = 'http://example.com/api/resource'
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, json=data)
assert response.status_code == 201
assert response.json()['key1'] == 'value1'
2. 异常情况的测试用例
import requests
def test_get_resource_not_found():
url = 'http://example.com/api/resource/999'
response = requests.get(url)
assert response.status_code == 404
def test_create_resource_with_invalid_data():
url = 'http://example.com/api/resource'
data = {'key1': '', 'key2': 'value2'} # key1为空,数据无效
response = requests.post(url, json=data)
assert response.status_code == 400
3. 边界情况的测试用例
import requests
def test_get_resource_with_large_id():
url = 'http://example.com/api/resource/2147483647' # 最大的32位整数
response = requests.get(url)
assert response.status_code in [200, 404] # 根据实际情况判断
def test_create_resource_with_large_data():
url = 'http://example.com/api/resource'
data = {'key1': 'a' * 1000, 'key2': 'b' * 1000} # 大量数据
response = requests.post(url, json=data)
assert response.status_code == 201
三、使用pytest进行测试自动化
pytest是一个功能强大的Python测试框架,支持编写简单的单元测试和复杂的功能测试。通过pytest,可以方便地管理和运行测试用例,并生成测试报告。
1. 安装pytest
pytest可以通过pip进行安装:
pip install pytest
2. 编写pytest测试用例
pytest的测试用例可以直接使用函数定义,并通过assert语句进行断言。下面是一个简单的pytest测试用例示例:
import requests
def test_get_resource():
url = 'http://example.com/api/resource'
response = requests.get(url)
assert response.status_code == 200
assert 'expected_data' in response.json()
def test_create_resource():
url = 'http://example.com/api/resource'
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, json=data)
assert response.status_code == 201
assert response.json()['key1'] == 'value1'
3. 运行pytest测试用例
pytest测试用例可以通过以下命令运行:
pytest test_api.py
其中,test_api.py
是包含测试用例的文件名。运行后,pytest会自动执行所有以test_
开头的函数,并输出测试结果。
4. 生成测试报告
pytest支持生成多种格式的测试报告,包括文本、HTML、XML等。可以通过安装pytest-html插件生成HTML格式的测试报告:
pip install pytest-html
然后使用以下命令生成HTML报告:
pytest test_api.py --html=report.html
生成的report.html
文件包含详细的测试结果,可以在浏览器中查看。
四、编写测试报告
编写测试报告是接口测试的最后一步。一个好的测试报告应该包含测试用例的执行情况、测试结果和测试覆盖率等信息。下面是一些编写测试报告的示例:
1. 简单的文本报告
import requests
def test_get_resource():
url = 'http://example.com/api/resource'
response = requests.get(url)
assert response.status_code == 200
assert 'expected_data' in response.json()
print('test_get_resource: PASSED')
def test_create_resource():
url = 'http://example.com/api/resource'
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, json=data)
assert response.status_code == 201
assert response.json()['key1'] == 'value1'
print('test_create_resource: PASSED')
2. 使用pytest生成详细的报告
通过pytest生成详细的测试报告,可以包含更多的信息,如测试用例的执行时间、失败的详细信息等。可以使用pytest-html插件生成HTML格式的报告:
pip install pytest-html
然后使用以下命令生成HTML报告:
pytest test_api.py --html=report.html
生成的report.html
文件包含详细的测试结果,可以在浏览器中查看。
3. 使用Allure生成报告
Allure是一个功能强大的测试报告工具,支持生成详细的测试报告。可以通过安装pytest-allure-adaptor插件生成Allure格式的报告:
pip install allure-pytest
然后使用以下命令生成Allure报告:
pytest test_api.py --alluredir=allure-results
生成的allure-results
目录包含测试结果,可以通过以下命令生成HTML报告:
allure generate allure-results -o allure-report
生成的allure-report
目录包含HTML格式的报告,可以在浏览器中查看。
五、接口测试的最佳实践
1. 使用虚拟环境
在进行接口测试时,建议使用Python的虚拟环境(virtualenv)来管理依赖库。通过虚拟环境,可以避免依赖库版本冲突的问题。可以使用以下命令创建虚拟环境:
python -m venv myenv
source myenv/bin/activate # 在Linux或MacOS上
myenv\Scripts\activate # 在Windows上
2. 配置文件管理
在接口测试中,常常需要使用各种配置,如API的URL、请求的头信息等。可以使用配置文件来管理这些配置信息,便于在不同的环境中切换。可以使用Python的configparser模块来管理配置文件:
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
api_url = config['API']['url']
headers = {
'Authorization': f"Bearer {config['API']['token']}"
}
配置文件config.ini的示例:
[API]
url = http://example.com/api
token = your_token
3. 使用Mock服务
在进行接口测试时,可能会遇到外部服务不可用或不稳定的情况。可以使用Mock服务来模拟外部服务,保证测试的稳定性。可以使用unittest.mock模块来创建Mock对象:
from unittest.mock import patch
import requests
def test_get_resource():
url = 'http://example.com/api/resource'
mock_response = {
'status_code': 200,
'json': lambda: {'key': 'value'}
}
with patch('requests.get') as mock_get:
mock_get.return_value = mock_response
response = requests.get(url)
assert response.status_code == 200
assert response.json() == {'key': 'value'}
六、接口测试的高级技巧
1. 参数化测试
参数化测试可以提高测试用例的复用性,通过一次编写多次执行不同的数据集。pytest支持参数化测试,可以使用@pytest.mark.parametrize装饰器来实现:
import pytest
import requests
@pytest.mark.parametrize("resource_id, expected_status", [
(1, 200),
(999, 404),
(2147483647, 200)
])
def test_get_resource(resource_id, expected_status):
url = f'http://example.com/api/resource/{resource_id}'
response = requests.get(url)
assert response.status_code == expected_status
2. 使用Fixture
Fixture是pytest中的一种机制,用于在测试用例执行前后进行一些准备和清理工作。可以使用@pytest.fixture装饰器来定义Fixture:
import pytest
import requests
@pytest.fixture
def api_url():
return 'http://example.com/api/resource'
def test_get_resource(api_url):
response = requests.get(api_url)
assert response.status_code == 200
assert 'expected_data' in response.json()
3. 使用依赖注入
依赖注入是一种设计模式,可以通过依赖注入提高代码的可测试性和复用性。在接口测试中,可以通过依赖注入来模拟不同的依赖对象:
class APIClient:
def __init__(self, base_url, session=None):
self.base_url = base_url
self.session = session or requests.Session()
def get(self, endpoint):
url = f'{self.base_url}/{endpoint}'
response = self.session.get(url)
return response
def test_get_resource():
session = requests.Session()
client = APIClient('http://example.com/api', session=session)
response = client.get('resource')
assert response.status_code == 200
assert 'expected_data' in response.json()
七、接口测试的常见问题和解决方法
1. 接口返回的数据格式不一致
在接口测试中,可能会遇到接口返回的数据格式不一致的问题。可以通过统一的数据结构来解决这个问题:
import requests
def get_json(response):
try:
return response.json()
except ValueError:
return {}
def test_get_resource():
url = 'http://example.com/api/resource'
response = requests.get(url)
data = get_json(response)
assert response.status_code == 200
assert 'expected_data' in data
2. 接口的响应时间过长
在接口测试中,可能会遇到接口的响应时间过长的问题,可以通过设置请求超时时间来解决这个问题:
import requests
def test_get_resource():
url = 'http://example.com/api/resource'
response = requests.get(url, timeout=5) # 设置超时时间为5秒
assert response.status_code == 200
assert 'expected_data' in response.json()
3. 多环境测试
在实际项目中,接口测试可能需要在多个环境中执行,如开发环境、测试环境和生产环境。可以通过配置文件或环境变量来管理不同环境的配置信息:
import os
import requests
def get_base_url():
env = os.getenv('ENV', 'development')
if env == 'production':
return 'http://prod.example.com/api'
elif env == 'staging':
return 'http://staging.example.com/api'
else:
return 'http://dev.example.com/api'
def test_get_resource():
base_url = get_base_url()
url = f'{base_url}/resource'
response = requests.get(url)
assert response.status_code == 200
assert 'expected_data' in response.json()
通过以上方法,可以有效地解决接口测试中的常见问题,提高测试的稳定性和可靠性。
八、接口测试的工具和框架
1. Postman
Postman是一个非常流行的API测试工具,支持发送各种HTTP请求和验证响应数据。通过Postman,可以方便地进行接口测试和自动化测试。Postman还支持生成代码片段,可以将Postman中的测试用例导出为Python代码。
2. Swagger
Swagger是一个API文档生成工具,支持自动生成API文档和接口测试用例。通过Swagger,可以方便地查看和测试API接口。Swagger还支持生成代码,可以将Swagger中的API定义导出为Python代码。
3. Robot Framework
Robot Framework是一个功能强大的测试自动化框架,支持多种测试类型,包括接口测试、UI测试和性能测试。通过Robot Framework,可以方便地管理和执行测试用例,并生成详细的测试报告。
4. JMeter
JMeter是一个开源的性能测试工具,支持多种协议和请求类型。通过JMeter,可以进行接口的性能测试和压力测试,验证接口在高并发场景下的稳定性和性能表现。
通过以上工具和框架,可以提高接口测试的效率和质量,保证接口的稳定性和可靠性。
九、总结
通过本文的介绍,我们详细了解了Python接口测试的基本方法和高级技巧,包括使用requests库发送HTTP请求、编写测试用例、使用pytest进行测试自动化、编写测试报告以及接口测试的最佳实践和常见问题的解决方法。希望通过本文的介绍,能够帮助读者更好地进行接口测试,提高接口的稳定性和可靠性。
相关问答FAQs:
如何在Python中进行接口测试?
在Python中进行接口测试通常使用一些流行的库,比如requests
和unittest
。通过requests
库,你可以发送HTTP请求并验证响应数据,而unittest
则帮助你组织和运行测试用例。结合这两者,你可以轻松构建高效的接口测试。
接口测试中如何验证响应数据的正确性?
在接口测试中,验证响应数据的正确性可以通过检查HTTP状态码、响应时间、响应体内容等方面进行。可以使用assert
语句来验证这些条件是否符合预期,确保API返回的数据与文档中描述的一致。
Python接口测试需要哪些工具和库?
进行Python接口测试时,常用的工具和库包括requests
用于发送请求,unittest
或pytest
用于组织测试用例,以及jsonschema
用于验证JSON格式的响应数据。此外,使用mock
库可以模拟API的行为,帮助测试不同场景下的响应。
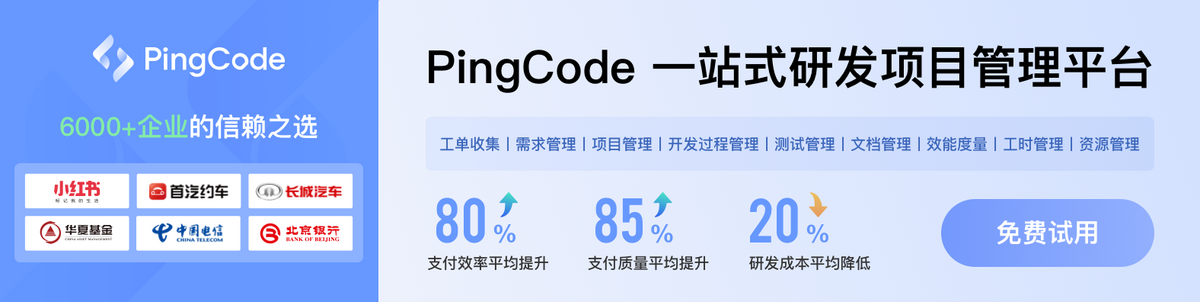