Python处理金额加的方式有:直接使用浮点数、使用Decimal类、使用自定义类。其中,推荐使用Decimal类,因为它可以避免浮点数计算中的精度问题,确保财务计算的准确性。
展开描述Decimal类的使用:Python的decimal
模块提供了一个Decimal
数据类型,用于十进制浮点数运算。相比于直接使用浮点数,Decimal
类可以精确地表示小数,并且在进行加法、减法、乘法和除法等运算时不会出现精度丢失的问题。这在处理金额计算时尤为重要,因为财务计算需要高度精确。
一、直接使用浮点数
直接使用浮点数是最简单的方法,但这种方法容易出现精度问题。例如:
amount1 = 100.10
amount2 = 200.20
total = amount1 + amount2
print(total) # 输出 300.30000000000007
如上所示,浮点数计算可能会出现额外的精度误差,不适合用于财务计算。
二、使用Decimal类
1、导入和基本使用
from decimal import Decimal
amount1 = Decimal('100.10')
amount2 = Decimal('200.20')
total = amount1 + amount2
print(total) # 输出 300.30
Decimal类可以通过字符串创建,避免了浮点数的精度问题。
2、设置精度和舍入方式
可以通过getcontext
方法设置精度和舍入方式:
from decimal import Decimal, getcontext, ROUND_HALF_UP
getcontext().prec = 2 # 设置精度为2
getcontext().rounding = ROUND_HALF_UP # 设置舍入方式为四舍五入
amount1 = Decimal('100.105')
amount2 = Decimal('200.205')
total = amount1 + amount2
print(total) # 输出 300.31
3、进行复杂的财务计算
Decimal类支持各种常见的财务计算方法,如加、减、乘、除等:
amount1 = Decimal('100.10')
amount2 = Decimal('200.20')
discount = Decimal('0.05') # 5% 折扣
加法
total = amount1 + amount2
print(total) # 输出 300.30
减法
difference = amount2 - amount1
print(difference) # 输出 100.10
乘法
discounted_price = total * (1 - discount)
print(discounted_price) # 输出 285.285
除法
average = total / Decimal('2')
print(average) # 输出 150.15
三、自定义类
如果需要更复杂的金额处理逻辑,可以自定义类。例如,实现一个Money类来封装金额处理:
class Money:
def __init__(self, amount):
self.amount = Decimal(amount)
def __add__(self, other):
return Money(self.amount + other.amount)
def __sub__(self, other):
return Money(self.amount - other.amount)
def __mul__(self, multiplier):
return Money(self.amount * Decimal(multiplier))
def __truediv__(self, divisor):
return Money(self.amount / Decimal(divisor))
def __str__(self):
return str(self.amount)
amount1 = Money('100.10')
amount2 = Money('200.20')
total = amount1 + amount2
print(total) # 输出 300.30
discounted_price = total * '0.95'
print(discounted_price) # 输出 285.285
通过自定义类,可以更灵活地处理金额操作,并且可以封装更多的业务逻辑和方法。
四、总结
在Python中处理金额加法时,推荐使用Decimal
类以避免浮点数的精度问题。这种方法不仅简单,而且可以确保财务计算的准确性。如果需要更复杂的处理逻辑,可以考虑自定义类来封装金额操作。无论选择哪种方法,都需要注意精度和舍入问题,以确保计算结果符合预期。
相关问答FAQs:
如何在Python中处理金额的加法计算?
在Python中处理金额的加法计算时,最常用的方法是使用Decimal模块。这个模块可以有效避免浮点数精度问题,确保计算结果的准确性。您可以通过以下示例代码实现金额相加:
from decimal import Decimal
amount1 = Decimal('10.50')
amount2 = Decimal('20.75')
total = amount1 + amount2
print(total) # 输出:31.25
使用Decimal模块进行金额计算有哪些优势?
Decimal模块提供了高精度的浮点运算,特别适合金融相关的计算。与传统的浮点数相比,Decimal可以精确表示小数,避免了在进行加减乘除时常见的舍入误差。此外,Decimal还支持自定义精度和舍入策略,使得在不同场景下的金额计算更加灵活。
如何在Python中格式化金额输出?
在Python中,可以使用字符串格式化来控制金额的输出格式。例如,可以将金额格式化为两位小数并加上货币符号。以下是一个简单的示例:
amount = Decimal('12345.6789')
formatted_amount = f"${amount:.2f}"
print(formatted_amount) # 输出:$12345.68
这种格式化方式不仅美观,还能确保金额的可读性,适用于报告和财务报表等场合。
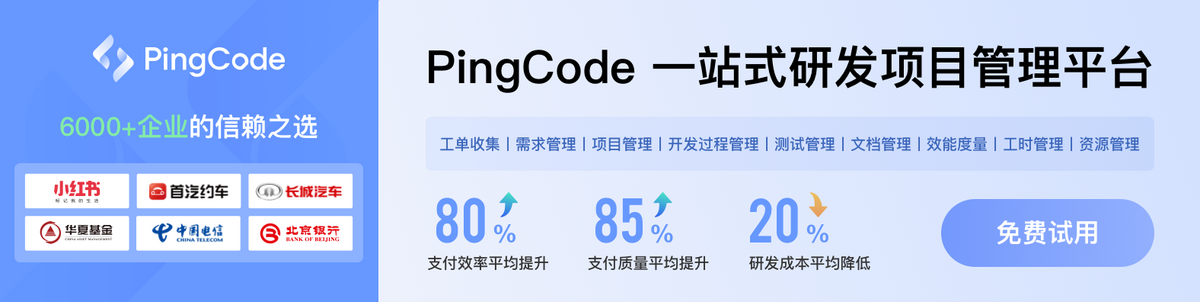