Python应声虫的设置包括:安装所需的库、创建一个应声虫类、定义应声虫的行为、运行应声虫。其中,安装所需的库是最重要的一步,因为它是实现应声虫功能的基础。具体方法如下:
首先,我们需要安装必要的库,例如pyttsx3
(用于语音合成)和speech_recognition
(用于语音识别)。这些库可以通过pip命令安装:
pip install pyttsx3
pip install SpeechRecognition
接下来,我们可以创建一个应声虫类,并在其中定义应声虫的行为。下面是一个简单的示例代码,用于实现基本的语音识别和语音合成功能:
import pyttsx3
import speech_recognition as sr
class EchoBot:
def __init__(self):
self.recognizer = sr.Recognizer()
self.engine = pyttsx3.init()
def listen(self):
with sr.Microphone() as source:
print("Listening...")
audio = self.recognizer.listen(source)
return audio
def recognize_speech(self, audio):
try:
text = self.recognizer.recognize_google(audio)
print(f"Recognized: {text}")
return text
except sr.UnknownValueError:
print("Could not understand audio")
return ""
except sr.RequestError:
print("Could not request results")
return ""
def speak(self, text):
self.engine.say(text)
self.engine.runAndWait()
def run(self):
while True:
audio = self.listen()
text = self.recognize_speech(audio)
if text:
self.speak(text)
if __name__ == "__main__":
bot = EchoBot()
bot.run()
在这个示例中,EchoBot
类使用pyttsx3
进行语音合成,使用speech_recognition
进行语音识别。在run
方法中,机器人会不断地监听用户的语音,识别语音内容,并将其通过语音合成器重复出来。
一、安装与配置
在开始编写应声虫程序之前,我们需要确保安装并配置好所需的库。以下是详细步骤:
1、安装Pyttsx3库
pyttsx3
是一个Python库,用于将文本转换为语音。它支持多个TTS(Text-to-Speech)引擎,并且可以在离线环境中运行。安装此库非常简单,只需运行以下命令:
pip install pyttsx3
安装完成后,我们可以进行简单的测试,确保其正常工作:
import pyttsx3
engine = pyttsx3.init()
engine.say("Hello, world!")
engine.runAndWait()
这段代码会初始化语音合成引擎,并让其朗读“Hello, world!”。
2、安装SpeechRecognition库
SpeechRecognition
是一个Python库,用于将语音转换为文本。它支持多个语音识别引擎,例如Google Web Speech API、IBM Watson等。安装此库同样简单,只需运行以下命令:
pip install SpeechRecognition
安装完成后,我们可以进行简单的测试,确保其正常工作:
import speech_recognition as sr
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Say something!")
audio = recognizer.listen(source)
try:
text = recognizer.recognize_google(audio)
print(f"You said: {text}")
except sr.UnknownValueError:
print("Google Speech Recognition could not understand audio")
except sr.RequestError as e:
print(f"Could not request results from Google Speech Recognition service; {e}")
这段代码会初始化语音识别器,并让其监听麦克风输入,随后使用Google Web Speech API将语音转换为文本。
二、创建应声虫类
完成了库的安装和配置后,我们可以开始创建应声虫类。应声虫类主要包含以下几个部分:初始化方法、监听方法、语音识别方法、语音合成方法和运行方法。
1、初始化方法
在初始化方法中,我们需要实例化语音识别器和语音合成引擎,并进行必要的配置:
class EchoBot:
def __init__(self):
self.recognizer = sr.Recognizer()
self.engine = pyttsx3.init()
self.configure_engine()
def configure_engine(self):
rate = self.engine.getProperty('rate')
self.engine.setProperty('rate', rate - 50)
volume = self.engine.getProperty('volume')
self.engine.setProperty('volume', volume + 0.25)
在configure_engine
方法中,我们调整了语音合成引擎的语速和音量,以便更适合应声虫的使用场景。
2、监听方法
监听方法用于捕获用户的语音输入。我们使用SpeechRecognition
库中的Microphone
类来实现这一功能:
def listen(self):
with sr.Microphone() as source:
print("Listening...")
audio = self.recognizer.listen(source)
return audio
在这个方法中,我们使用Microphone
类捕获音频数据,并将其返回。
3、语音识别方法
语音识别方法用于将捕获的音频数据转换为文本。我们使用SpeechRecognition
库中的recognize_google
方法来实现这一功能:
def recognize_speech(self, audio):
try:
text = self.recognizer.recognize_google(audio)
print(f"Recognized: {text}")
return text
except sr.UnknownValueError:
print("Could not understand audio")
return ""
except sr.RequestError:
print("Could not request results")
return ""
在这个方法中,我们处理了两种常见的异常情况:无法理解音频和请求失败。
4、语音合成方法
语音合成方法用于将文本转换为语音。我们使用pyttsx3
库中的say
和runAndWait
方法来实现这一功能:
def speak(self, text):
self.engine.say(text)
self.engine.runAndWait()
在这个方法中,我们调用语音合成引擎,将文本转换为语音并播放。
5、运行方法
运行方法用于启动应声虫,并实现其主要逻辑。我们在这个方法中不断地监听用户的语音输入,识别语音内容,并将其通过语音合成器重复出来:
def run(self):
while True:
audio = self.listen()
text = self.recognize_speech(audio)
if text:
self.speak(text)
三、语音识别与合成优化
在实际应用中,我们可能需要对语音识别和合成进行进一步优化,以提高应声虫的性能和用户体验。
1、提高语音识别准确性
为了提高语音识别的准确性,我们可以使用recognizer.adjust_for_ambient_noise
方法来适应环境噪音:
def listen(self):
with sr.Microphone() as source:
self.recognizer.adjust_for_ambient_noise(source)
print("Listening...")
audio = self.recognizer.listen(source)
return audio
在这个方法中,我们在开始监听之前调用adjust_for_ambient_noise
方法,以适应当前环境的噪音,从而提高语音识别的准确性。
2、调整语音合成参数
为了使语音合成更加自然和清晰,我们可以调整语音合成引擎的音调和声音属性:
def configure_engine(self):
rate = self.engine.getProperty('rate')
self.engine.setProperty('rate', rate - 50)
volume = self.engine.getProperty('volume')
self.engine.setProperty('volume', volume + 0.25)
voices = self.engine.getProperty('voices')
self.engine.setProperty('voice', voices[1].id) # Select a different voice
在这个方法中,我们选择了一个不同的声音,以提供更自然的语音合成效果。
四、添加更多功能
为了使应声虫更加实用和有趣,我们可以添加更多功能,例如对特定命令的响应、播放音乐、查询天气等。
1、响应特定命令
我们可以添加一个方法,用于识别特定命令并做出相应的响应:
def handle_command(self, command):
if "hello" in command:
self.speak("Hello! How can I help you?")
elif "weather" in command:
self.speak("The weather is sunny today.")
elif "play music" in command:
self.speak("Playing your favorite music.")
else:
self.speak("I'm sorry, I don't understand that command.")
在这个方法中,我们根据识别到的命令执行相应的操作。
2、集成到运行方法中
我们需要将处理命令的方法集成到运行方法中,以便在识别到特定命令时做出响应:
def run(self):
while True:
audio = self.listen()
text = self.recognize_speech(audio)
if text:
self.handle_command(text)
通过这种方式,我们可以使应声虫具备更多的功能和更高的实用性。
五、错误处理与调试
在开发应声虫的过程中,我们可能会遇到各种错误和问题。为了确保程序的稳定性和可靠性,我们需要进行充分的错误处理与调试。
1、捕获并处理异常
在各个方法中,我们需要捕获并处理可能出现的异常,以避免程序崩溃:
def recognize_speech(self, audio):
try:
text = self.recognizer.recognize_google(audio)
print(f"Recognized: {text}")
return text
except sr.UnknownValueError:
print("Could not understand audio")
return ""
except sr.RequestError:
print("Could not request results")
return ""
通过这种方式,我们可以确保程序在遇到错误时能够正常运行。
2、调试信息输出
为了方便调试,我们可以在各个方法中添加调试信息输出,以便了解程序的运行状态:
def listen(self):
with sr.Microphone() as source:
self.recognizer.adjust_for_ambient_noise(source)
print("Listening...")
audio = self.recognizer.listen(source)
return audio
通过这种方式,我们可以更容易地发现和解决问题。
六、扩展与集成
应声虫可以作为一个独立的应用程序,也可以集成到其他系统中,以提供语音交互功能。以下是一些可能的扩展与集成方向:
1、与智能家居系统集成
应声虫可以与智能家居系统集成,以提供语音控制功能。例如,我们可以使用智能家居平台的API,实现对灯光、温度、门锁等设备的语音控制:
def handle_command(self, command):
if "turn on the light" in command:
self.control_smart_home("light", "on")
elif "turn off the light" in command:
self.control_smart_home("light", "off")
elif "set temperature to 22 degrees" in command:
self.control_smart_home("thermostat", 22)
else:
self.speak("I'm sorry, I don't understand that command.")
def control_smart_home(self, device, action):
# Call the smart home platform's API to control the device
pass
通过这种方式,我们可以实现对智能家居设备的语音控制。
2、与聊天机器人集成
应声虫可以与聊天机器人集成,以提供更丰富的对话功能。例如,我们可以使用聊天机器人的API,实现对用户问题的智能回答:
import requests
def handle_command(self, command):
response = self.query_chatbot(command)
self.speak(response)
def query_chatbot(self, query):
api_url = "https://api.chatbot.com/query"
response = requests.post(api_url, json={"query": query})
return response.json().get("response")
通过这种方式,我们可以实现与用户的智能对话。
七、总结与展望
通过本文的介绍,我们了解了如何设置和实现一个基本的Python应声虫。我们从安装和配置必要的库开始,逐步创建应声虫类,并实现其主要功能。同时,我们还探讨了如何优化语音识别和合成,添加更多功能,以及进行错误处理与调试。最后,我们提出了应声虫的扩展与集成方向,以期在未来的应用中发挥更大的作用。
应声虫作为一个有趣且实用的语音交互工具,可以广泛应用于智能家居、聊天机器人、语音助手等领域。随着技术的发展和进步,我们可以期待应声虫在未来具有更高的性能和更广泛的应用场景。通过不断地探索和实践,我们相信应声虫将为我们的生活带来更多的便利和乐趣。
相关问答FAQs:
如何在Python中安装应声虫库?
要在Python中安装应声虫(pyttsx3)库,首先需要确保您的计算机上已经安装了Python。然后,您可以使用pip命令在终端或命令提示符中输入以下命令:pip install pyttsx3
。这样就可以轻松下载并安装该库。
应声虫支持哪些音频输出设备?
应声虫库通常支持多种音频输出设备,包括计算机内置音频系统、外部扬声器和耳机。具体支持的设备可能会根据您的操作系统和驱动程序的不同而有所变化。您可以通过调整系统的音频设置来选择希望使用的输出设备。
如何调整应声虫的语音速度和音调?
在使用应声虫库时,您可以通过设置语音属性来调整语音的速度和音调。可以使用以下代码示例来实现:
import pyttsx3
engine = pyttsx3.init()
engine.setProperty('rate', 150) # 设置语速
engine.setProperty('volume', 0.9) # 设置音量
engine.say("你好,欢迎使用应声虫!")
engine.runAndWait()
通过修改rate
和volume
的值,可以实现不同的语音效果。
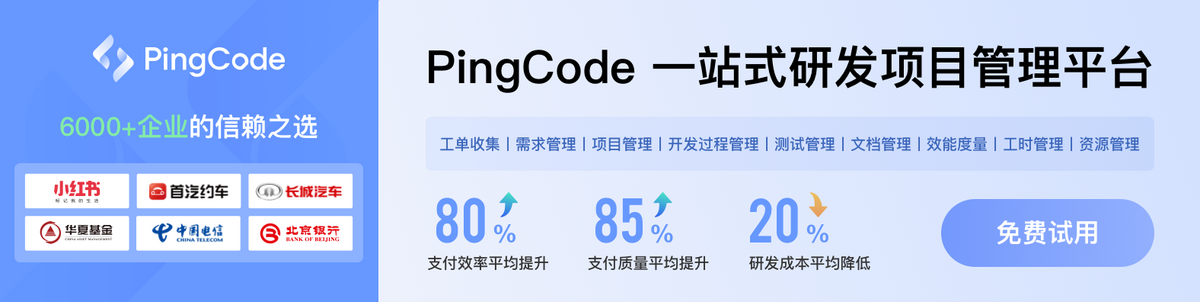