使用Python进行网络爬虫可以通过以下几个核心步骤:选择合适的库、解析网页内容、处理数据存储、处理反爬机制。其中,选择合适的库是一个关键步骤,详细介绍如下:
在Python中,用于网络爬虫的库主要有requests和BeautifulSoup。requests库用于发送HTTP请求,可以方便地获取网页的源代码,而BeautifulSoup库则用于解析和提取网页内容。通过这两个库的结合,可以有效地完成大部分的爬虫任务。此外,Scrapy也是一个强大的网络爬虫框架,适用于更复杂的爬虫项目。
一、选择合适的库
1. Requests库
Requests库是Python中最常用的HTTP库之一。它可以很方便地发送HTTP请求,并且处理复杂的HTTP请求和响应。使用Requests库,你可以简单地获取网页的HTML源代码。
import requests
response = requests.get('https://example.com')
print(response.text)
2. BeautifulSoup库
BeautifulSoup库是一个用于解析HTML和XML的库。它可以帮助你从网页中提取有用的信息,比如标题、图片、链接等。
from bs4 import BeautifulSoup
html = response.text
soup = BeautifulSoup(html, 'html.parser')
title = soup.find('title').text
print(title)
3. Scrapy框架
Scrapy是一个强大的网络爬虫框架,适用于更复杂的爬虫项目。Scrapy提供了完整的爬虫开发工具,包括下载器、中间件、管道等。
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
title = response.xpath('//title/text()').get()
print(title)
二、解析网页内容
1. 使用BeautifulSoup解析HTML
BeautifulSoup可以解析HTML和XML文件,并将其转换为一个可以方便操作的BeautifulSoup对象。你可以使用BeautifulSoup的各种方法来查找和提取你需要的信息。
from bs4 import BeautifulSoup
html = response.text
soup = BeautifulSoup(html, 'html.parser')
查找所有的链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
2. 使用XPath解析HTML
XPath是一种用于在XML文档中查找信息的语言。Scrapy框架支持使用XPath来解析网页内容。
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
links = response.xpath('//a/@href').getall()
for link in links:
print(link)
三、处理数据存储
在获取并解析网页内容后,下一步就是处理数据存储。你可以将数据保存到本地文件、数据库或其他存储系统中。
1. 保存到本地文件
你可以将爬取的数据保存到本地文件中,比如CSV、JSON等格式。
import csv
data = [{'title': 'Example 1', 'link': 'https://example.com/1'},
{'title': 'Example 2', 'link': 'https://example.com/2'}]
with open('data.csv', 'w', newline='') as csvfile:
fieldnames = ['title', 'link']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for item in data:
writer.writerow(item)
2. 保存到数据库
你可以将爬取的数据保存到数据库中,比如MySQL、MongoDB等。
import pymysql
connection = pymysql.connect(host='localhost',
user='user',
password='passwd',
database='db')
cursor = connection.cursor()
cursor.execute('INSERT INTO table (title, link) VALUES (%s, %s)', ('Example', 'https://example.com'))
connection.commit()
cursor.close()
connection.close()
四、处理反爬机制
很多网站都有反爬机制,防止用户过于频繁地访问。为了避免被封禁,可以采取以下几种方法:
1. 设置请求头
通过设置请求头,可以伪装成浏览器访问网页,避免被识别为爬虫。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get('https://example.com', headers=headers)
2. 设置延时
通过设置延时,可以避免过于频繁地访问网页,降低被封禁的风险。
import time
for url in urls:
response = requests.get(url)
time.sleep(1) # 延时1秒
3. 使用代理
通过使用代理,可以隐藏真实的IP地址,避免被封禁。
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get('https://example.com', proxies=proxies)
五、综合实例
结合上面的内容,我们可以实现一个简单的网络爬虫,爬取一个新闻网站的标题和链接,并保存到CSV文件中。
import requests
from bs4 import BeautifulSoup
import csv
import time
def get_html(url, headers=None):
response = requests.get(url, headers=headers)
return response.text
def parse_html(html):
soup = BeautifulSoup(html, 'html.parser')
articles = soup.find_all('article')
data = []
for article in articles:
title = article.find('h2').text
link = article.find('a').get('href')
data.append({'title': title, 'link': link})
return data
def save_to_csv(data, filename):
with open(filename, 'w', newline='') as csvfile:
fieldnames = ['title', 'link']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for item in data:
writer.writerow(item)
def main():
url = 'https://example.com/news'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
html = get_html(url, headers=headers)
data = parse_html(html)
save_to_csv(data, 'news.csv')
# 延时1秒,避免过于频繁地访问网页
time.sleep(1)
if __name__ == '__main__':
main()
这个综合实例展示了如何使用Requests库获取网页HTML源代码,使用BeautifulSoup解析网页内容,并将爬取的数据保存到CSV文件中。通过设置请求头和延时,可以有效地避免被识别为爬虫并降低被封禁的风险。
六、进阶内容
1. 异步爬虫
对于大规模的数据爬取,异步爬虫可以显著提高爬取效率。Python的aiohttp和asyncio库可以用于实现异步爬虫。
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
async with aiohttp.ClientSession() as session:
html = await fetch(session, 'https://example.com')
print(html)
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
2. 爬取动态网页
对于使用JavaScript加载内容的动态网页,传统的Requests和BeautifulSoup可能无法获取完整的网页内容。此时,可以使用Selenium或Playwright等工具来模拟浏览器行为。
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
html = driver.page_source
print(html)
driver.quit()
3. 分布式爬虫
对于更大规模的爬取任务,可以使用分布式爬虫架构,比如Scrapy-Redis。它可以实现分布式爬取,多个爬虫节点协同工作,提高爬取效率。
# Scrapy-Redis的使用示例
在settings.py中配置
SCHEDULER = "scrapy_redis.scheduler.Scheduler"
DUPEFILTER_CLASS = "scrapy_redis.dupefilter.RFPDupeFilter"
SCHEDULER_PERSIST = True
REDIS_URL = 'redis://localhost:6379'
在爬虫代码中
import scrapy
from scrapy_redis.spiders import RedisSpider
class ExampleSpider(RedisSpider):
name = 'example'
redis_key = 'example:start_urls'
def parse(self, response):
title = response.xpath('//title/text()').get()
yield {'title': title}
七、最佳实践
1. 合法性和伦理道德
在进行网络爬虫之前,请确保你有权访问并爬取目标网站的数据。遵守网站的robots.txt文件和相关法律法规,避免给网站带来负担和损害。
2. 代码组织
将爬虫代码组织成模块化、可重用的组件,可以提高代码的可维护性和可扩展性。使用面向对象编程(OOP)和设计模式,可以使代码更加清晰和易于理解。
3. 错误处理
在网络爬虫过程中,可能会遇到各种错误和异常情况,比如网络连接错误、解析错误等。需要编写健壮的错误处理机制,以确保爬虫能够稳定运行。
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
4. 数据清洗和预处理
在数据存储之前,进行必要的数据清洗和预处理,可以提高数据的质量和一致性。比如,去除重复数据、处理缺失值、格式化数据等。
5. 监控和日志
在大规模爬虫项目中,使用监控和日志记录,可以帮助你了解爬虫的运行状态,及时发现和解决问题。使用日志库(比如logging)记录关键操作和错误信息。
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
logger.info('Starting spider...')
logger.error('Error occurred: %s', error)
八、总结
通过以上内容,你可以了解到使用Python进行网络爬虫的基本方法和步骤。选择合适的库和框架,解析网页内容,处理数据存储,并应对反爬机制,是实现一个成功爬虫项目的关键。希望这些内容对你有所帮助,祝你在爬虫开发中取得成功。
相关问答FAQs:
如何开始学习Python网络爬虫的基础知识?
学习Python网络爬虫的第一步是掌握Python语言的基本语法和数据结构。了解HTTP协议、网页结构(如HTML、CSS)是非常重要的。可以通过在线课程、书籍或视频教程来获取这些知识。此外,熟悉一些爬虫相关的库如Requests和BeautifulSoup,将帮助你在实际操作中更加得心应手。
在进行网络爬虫时,如何处理网站的反爬虫措施?
许多网站会采取反爬虫措施来保护其数据。为了有效应对这些措施,可以采取一些策略。使用随机的用户代理(User-Agent)可以避免被识别为爬虫;设置适当的请求间隔来模仿人类用户的行为;还可以利用代理IP来隐藏真实IP地址。这些方法可以提高爬虫的成功率。
如何存储爬取到的数据以便后续分析?
爬取到的数据可以根据需求存储在多种格式中。常见的存储方式包括CSV文件、JSON文件和数据库(如SQLite、MySQL等)。选择合适的存储方式不仅可以方便后续的数据分析,还能提高数据的可访问性。根据数据的复杂性和使用频率,合理选择存储格式非常重要。
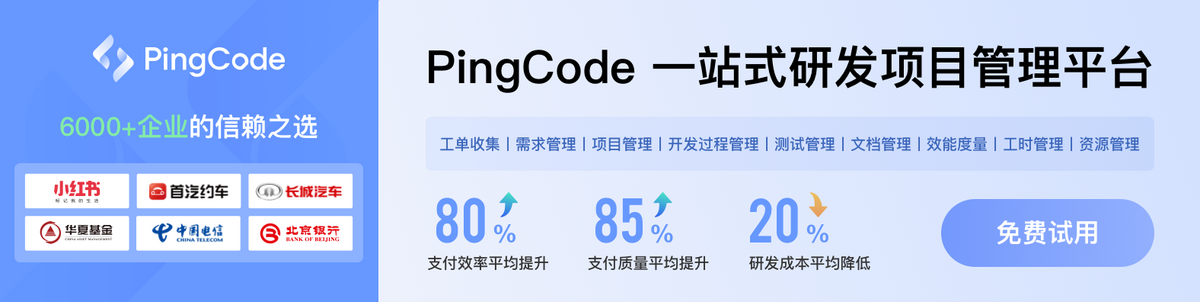