Python和C语言可以通过多种方式进行通信,包括使用Python的C扩展、通过Ctypes库调用C函数、使用Cython工具、通过文件或管道进行数据传输等。本文将详细介绍这些方法,其中使用Ctypes库调用C函数是最常用且易于上手的方法。
使用Ctypes库调用C函数的详细描述:Ctypes是Python内置的一个库,它允许Python代码调用C函数,加载共享库(DLLs或.so文件),并与C语言进行交互。通过Ctypes,我们可以直接调用C语言编写的函数并传递参数,获取返回值。具体步骤包括编写C语言代码并编译成共享库,然后在Python代码中使用Ctypes加载该共享库并调用其中的函数。
一、使用Python的C扩展
1、编写C语言代码
首先,我们需要编写C语言代码,并将其编译为共享库。以下是一个简单的C语言示例,包含一个求和函数:
// mymodule.c
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
2、编译为共享库
接下来,我们需要将上述C代码编译为共享库。在Linux系统上,可以使用以下命令:
gcc -shared -o mymodule.so -fPIC mymodule.c
在Windows系统上,可以使用以下命令:
gcc -shared -o mymodule.dll mymodule.c
3、在Python中调用C函数
在Python代码中,我们可以使用Ctypes库加载共享库并调用其中的函数:
import ctypes
加载共享库
mymodule = ctypes.CDLL('./mymodule.so') # Linux
mymodule = ctypes.CDLL('mymodule.dll') # Windows
调用add函数
result = mymodule.add(3, 5)
print(result) # 输出: 8
二、通过Ctypes库调用C函数
1、编写C语言代码
同样,我们首先需要编写C语言代码并编译为共享库。以下是一个示例,包含一个求平方的函数:
// square.c
#include <stdio.h>
double square(double num) {
return num * num;
}
2、编译为共享库
在Linux系统上,可以使用以下命令:
gcc -shared -o square.so -fPIC square.c
在Windows系统上,可以使用以下命令:
gcc -shared -o square.dll square.c
3、在Python中调用C函数
在Python代码中,我们可以使用Ctypes库加载共享库并调用其中的函数:
import ctypes
加载共享库
square_module = ctypes.CDLL('./square.so') # Linux
square_module = ctypes.CDLL('square.dll') # Windows
定义函数参数和返回值类型
square_module.square.argtypes = [ctypes.c_double]
square_module.square.restype = ctypes.c_double
调用square函数
result = square_module.square(4.0)
print(result) # 输出: 16.0
三、使用Cython工具
Cython是一种编译器,可以将Python代码转换为C代码,从而提高性能。我们可以使用Cython编写Python和C语言的混合代码,并生成共享库供Python调用。
1、编写Cython代码
首先,我们需要编写Cython代码,并保存为.pyx
文件。以下是一个示例,包含一个求和函数:
# mymodule.pyx
cdef int add(int a, int b):
return a + b
2、编写setup.py脚本
接下来,我们需要编写一个setup.py
脚本,用于编译Cython代码:
# setup.py
from setuptools import setup
from Cython.Build import cythonize
setup(
ext_modules=cythonize("mymodule.pyx")
)
3、编译Cython代码
在终端中运行以下命令编译Cython代码:
python setup.py build_ext --inplace
4、在Python中调用Cython函数
编译完成后,我们可以在Python代码中直接导入并调用Cython生成的模块:
import mymodule
调用add函数
result = mymodule.add(3, 5)
print(result) # 输出: 8
四、通过文件或管道进行数据传输
除了直接调用C函数外,Python和C语言还可以通过文件或管道进行数据传输。这种方法适用于需要传输大量数据或复杂数据结构的场景。
1、通过文件进行数据传输
我们可以让C程序将数据写入文件,然后在Python中读取文件内容。以下是一个示例:
编写C程序
// write_data.c
#include <stdio.h>
int main() {
FILE *file = fopen("data.txt", "w");
if (file == NULL) {
printf("Error opening file!\n");
return 1;
}
fprintf(file, "Hello from C!\n");
fclose(file);
return 0;
}
编译C程序:
gcc -o write_data write_data.c
编写Python代码
import os
运行C程序
os.system("./write_data")
读取文件内容
with open("data.txt", "r") as file:
data = file.read()
print(data) # 输出: Hello from C!
2、通过管道进行数据传输
我们可以使用Python的subprocess
模块创建子进程,并通过管道与C程序进行数据传输。以下是一个示例:
编写C程序
// echo.c
#include <stdio.h>
int main() {
char buffer[128];
while (fgets(buffer, sizeof(buffer), stdin)) {
printf("C received: %s", buffer);
}
return 0;
}
编译C程序:
gcc -o echo echo.c
编写Python代码
import subprocess
创建子进程
proc = subprocess.Popen("./echo", stdin=subprocess.PIPE, stdout=subprocess.PIPE, text=True)
通过管道发送数据
proc.stdin.write("Hello from Python!\n")
proc.stdin.flush()
读取子进程的输出
output = proc.stdout.readline()
print(output) # 输出: C received: Hello from Python!
以上介绍了Python和C语言通信的多种方法,包括使用Python的C扩展、通过Ctypes库调用C函数、使用Cython工具、通过文件或管道进行数据传输。根据实际需求,选择合适的方法可以有效地实现Python与C语言的通信。
相关问答FAQs:
如何在Python和C语言之间传递数据?
在Python和C语言之间传递数据可以通过多种方式实现,包括使用C扩展模块、ctypes库、或通过文件和网络通信等方法。C扩展模块允许你将C函数作为Python模块导入,ctypes库则提供了调用C共享库的能力。选择最佳的方法取决于应用的需求和复杂性。
使用Cython来实现Python与C的通信有什么优势?
Cython是一种编程语言,可以让你在Python中编写C扩展,提供了更高的性能。通过Cython,可以方便地调用C函数,并且可以在Python代码中使用C数据类型,这样可以提升计算效率,特别是在处理大量数据或需要高性能的计算时。
Python与C语言通信时常见的问题有哪些?
在进行Python与C语言的通信时,可能会遇到一些常见问题,包括数据类型不匹配、内存管理问题以及跨平台兼容性等。确保使用正确的数据类型转换,并小心内存的分配和释放,可以帮助避免这些问题。此外,使用工具和库(如ctypes或Cython)能够简化这一过程并减少错误发生的几率。
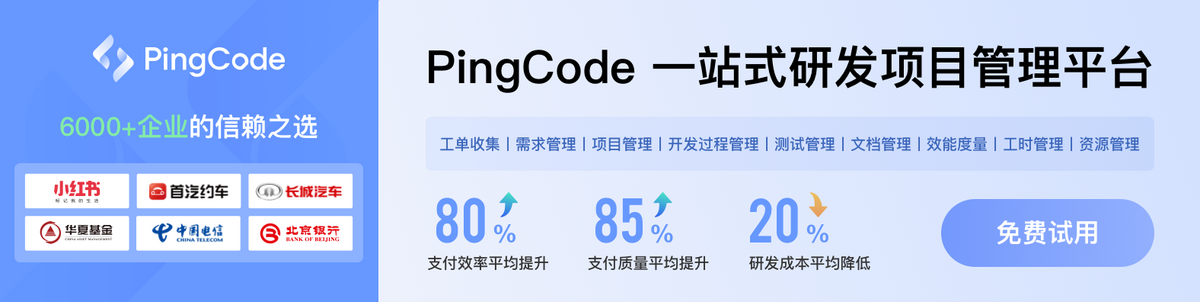