Python爬取软件数据的方法包括:使用requests库获取网页内容、解析HTML结构、模拟浏览器行为、处理动态数据。
其中,使用requests库获取网页内容是最基础也是最常用的方法之一。通过requests库,我们可以发送HTTP请求,获取网页的HTML内容,然后进一步解析和处理。
import requests
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print("Failed to retrieve the webpage")
这个简单的示例展示了如何使用requests库发送GET请求并获取网页内容。接下来我们会详细介绍其他方法。
一、使用requests库获取网页内容
requests库是Python中最常用的HTTP库之一,它简化了HTTP请求的操作。使用requests库,我们可以轻松地发送GET、POST请求,并处理响应。
1、发送GET请求
GET请求是最常见的HTTP请求,用于从服务器获取数据。requests库提供了方便的方法来发送GET请求。
import requests
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print("Failed to retrieve the webpage")
在这个示例中,我们使用requests.get()方法发送GET请求,并检查响应状态码是否为200(即请求成功)。如果成功,我们将获取到的HTML内容打印出来。
2、发送POST请求
除了GET请求,有时我们还需要发送POST请求,比如提交表单数据。requests库同样提供了方便的方法来发送POST请求。
import requests
url = "http://example.com"
data = {
"username": "user",
"password": "pass"
}
response = requests.post(url, data=data)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print("Failed to retrieve the webpage")
在这个示例中,我们使用requests.post()方法发送POST请求,并传递表单数据。与GET请求类似,我们检查响应状态码并处理响应内容。
二、解析HTML结构
获取到网页的HTML内容后,我们需要解析其中的数据。常用的HTML解析库有BeautifulSoup和lxml。
1、使用BeautifulSoup解析HTML
BeautifulSoup是一个功能强大的HTML解析库,它提供了简单的API来处理和导航HTML文档。下面是一个使用BeautifulSoup解析HTML的示例。
from bs4 import BeautifulSoup
import requests
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, "html.parser")
title = soup.title.string
print(f"Title: {title}")
else:
print("Failed to retrieve the webpage")
在这个示例中,我们使用BeautifulSoup将HTML内容解析为一个BeautifulSoup对象,然后通过导航HTML结构获取标题。
2、使用lxml解析HTML
lxml是另一个流行的HTML解析库,它在处理大规模HTML文档时表现出色。下面是一个使用lxml解析HTML的示例。
from lxml import etree
import requests
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
tree = etree.HTML(response.text)
title = tree.xpath("//title/text()")[0]
print(f"Title: {title}")
else:
print("Failed to retrieve the webpage")
在这个示例中,我们使用etree.HTML()方法将HTML内容解析为一个Element对象,然后使用XPath表达式获取标题。
三、模拟浏览器行为
有些网站使用JavaScript动态加载内容,直接获取HTML内容可能无法获取到我们需要的数据。在这种情况下,我们可以使用Selenium库来模拟浏览器行为。
1、使用Selenium模拟浏览器
Selenium是一个功能强大的Web自动化工具,它可以模拟用户在浏览器中的操作。下面是一个使用Selenium模拟浏览器的示例。
from selenium import webdriver
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
title = driver.title
print(f"Title: {title}")
driver.quit()
在这个示例中,我们使用Selenium启动一个Chrome浏览器,并访问指定的URL。然后我们获取页面标题并打印出来,最后关闭浏览器。
2、处理动态内容
有些网站使用JavaScript动态加载内容,我们可以使用Selenium等待页面加载完成后再获取数据。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
url = "http://example.com"
driver = webdriver.Chrome()
driver.get(url)
try:
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, "dynamic-element-id"))
)
print(f"Element text: {element.text}")
finally:
driver.quit()
在这个示例中,我们使用WebDriverWait等待特定元素加载完成,然后获取其文本内容。
四、处理动态数据
有些网站的数据通过API提供,我们可以直接调用API获取数据,而不需要解析HTML。
1、调用API获取数据
许多网站提供RESTful API接口,我们可以使用requests库直接调用这些API获取数据。
import requests
api_url = "http://example.com/api/data"
response = requests.get(api_url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print("Failed to retrieve the data")
在这个示例中,我们使用requests.get()方法调用API,并将响应内容解析为JSON格式。
2、处理分页数据
有些API返回的数据分页,我们需要处理分页数据。下面是一个处理分页数据的示例。
import requests
api_url = "http://example.com/api/data"
page = 1
while True:
response = requests.get(api_url, params={"page": page})
if response.status_code == 200:
data = response.json()
if not data:
break
for item in data:
print(item)
page += 1
else:
print("Failed to retrieve the data")
break
在这个示例中,我们通过循环处理分页数据,直到没有更多数据为止。
五、处理复杂的爬取需求
在实际项目中,我们可能需要处理更复杂的爬取需求,比如登录保护、数据处理和存储等。
1、处理登录保护
有些网站需要登录才能访问数据,我们可以使用requests库模拟登录。
import requests
login_url = "http://example.com/login"
data_url = "http://example.com/data"
login_data = {
"username": "user",
"password": "pass"
}
session = requests.Session()
response = session.post(login_url, data=login_data)
if response.status_code == 200:
response = session.get(data_url)
if response.status_code == 200:
print(response.text)
else:
print("Failed to retrieve the data")
else:
print("Failed to login")
在这个示例中,我们使用requests.Session()创建一个会话,并通过POST请求模拟登录,然后使用会话获取数据。
2、处理数据存储
获取到的数据通常需要存储到数据库或文件中,以便后续处理和分析。下面是一个将数据存储到SQLite数据库的示例。
import sqlite3
import requests
db = sqlite3.connect("data.db")
cursor = db.cursor()
cursor.execute("""
CREATE TABLE IF NOT EXISTS data (
id INTEGER PRIMARY KEY,
name TEXT,
value TEXT
)
""")
api_url = "http://example.com/api/data"
response = requests.get(api_url)
if response.status_code == 200:
data = response.json()
for item in data:
cursor.execute("INSERT INTO data (name, value) VALUES (?, ?)", (item["name"], item["value"]))
db.commit()
else:
print("Failed to retrieve the data")
db.close()
在这个示例中,我们使用sqlite3模块创建一个SQLite数据库,并将获取到的数据插入到数据库中。
3、处理数据清洗和分析
获取到的数据通常需要进行清洗和分析,以提取有用的信息。下面是一个简单的数据清洗和分析示例。
import pandas as pd
import requests
api_url = "http://example.com/api/data"
response = requests.get(api_url)
if response.status_code == 200:
data = response.json()
df = pd.DataFrame(data)
df.dropna(inplace=True)
summary = df.describe()
print(summary)
else:
print("Failed to retrieve the data")
在这个示例中,我们使用pandas库将数据加载到DataFrame中,并进行简单的数据清洗和分析。
六、爬虫的常见问题和解决方案
在实际操作中,爬虫会遇到各种问题,如反爬虫机制、IP封禁等。以下是一些常见问题及其解决方案。
1、应对反爬虫机制
许多网站会使用反爬虫机制来阻止爬虫访问。常见的反爬虫机制包括用户代理检测、IP封禁、验证码等。我们可以通过以下方法应对这些机制。
更改用户代理
通过更改用户代理,我们可以伪装成不同的浏览器,以绕过简单的反爬虫机制。
import requests
url = "http://example.com"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print(response.text)
else:
print("Failed to retrieve the webpage")
使用代理IP
通过使用代理IP,我们可以避免因频繁访问同一IP地址而被封禁。
import requests
url = "http://example.com"
proxies = {
"http": "http://proxy_ip:proxy_port",
"https": "https://proxy_ip:proxy_port"
}
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
print(response.text)
else:
print("Failed to retrieve the webpage")
处理验证码
对于需要验证码的网站,我们可以使用图像识别技术自动识别验证码,或手动输入验证码。
from PIL import Image
import pytesseract
import requests
url = "http://example.com"
captcha_url = "http://example.com/captcha"
captcha_image = requests.get(captcha_url).content
with open("captcha.jpg", "wb") as f:
f.write(captcha_image)
captcha_text = pytesseract.image_to_string(Image.open("captcha.jpg"))
data = {
"captcha": captcha_text
}
response = requests.post(url, data=data)
if response.status_code == 200:
print(response.text)
else:
print("Failed to retrieve the webpage")
2、避免IP封禁
频繁访问同一网站可能导致IP被封禁。我们可以通过以下方法避免IP封禁。
限制请求频率
通过限制请求频率,我们可以避免过于频繁地访问同一网站。
import requests
import time
url = "http://example.com"
for i in range(10):
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print("Failed to retrieve the webpage")
time.sleep(1) # 每次请求间隔1秒
使用多个代理IP
通过使用多个代理IP,我们可以分散请求,减少单个IP被封禁的风险。
import requests
import random
url = "http://example.com"
proxies_list = [
{"http": "http://proxy_ip1:proxy_port1", "https": "https://proxy_ip1:proxy_port1"},
{"http": "http://proxy_ip2:proxy_port2", "https": "https://proxy_ip2:proxy_port2"},
# 更多代理IP
]
for i in range(10):
proxies = random.choice(proxies_list)
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
print(response.text)
else:
print("Failed to retrieve the webpage")
七、爬虫的法律和道德问题
在进行爬虫操作时,我们需要遵守相关的法律和道德规范。以下是一些需要注意的问题。
1、尊重网站的robots.txt
许多网站会使用robots.txt文件来声明哪些页面可以被爬虫访问,哪些页面不能被爬虫访问。我们需要尊重这些声明。
import requests
from urllib.robotparser import RobotFileParser
url = "http://example.com"
robots_url = "http://example.com/robots.txt"
robots_parser = RobotFileParser()
robots_parser.set_url(robots_url)
robots_parser.read()
if robots_parser.can_fetch("*", url):
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print("Failed to retrieve the webpage")
else:
print("This page is disallowed by robots.txt")
2、遵守数据隐私和版权法规
在爬取数据时,我们需要遵守数据隐私和版权法规,确保不侵犯他人的隐私和知识产权。
不爬取敏感信息
我们应该避免爬取涉及个人隐私或敏感信息的数据。
遵守版权声明
对于受版权保护的数据,我们需要获得相关许可或遵守版权声明。
合理使用数据
我们应该合理使用爬取到的数据,不进行恶意利用。
八、爬虫项目的实际案例
通过一个实际的爬虫项目案例,我们可以更好地理解如何综合运用上述方法和技巧。
1、项目需求
假设我们需要爬取一个在线书店的网站,获取书籍的标题、作者、价格等信息,并存储到SQLite数据库中。
2、项目实现
创建数据库
首先,我们创建一个SQLite数据库来存储书籍信息。
import sqlite3
db = sqlite3.connect("books.db")
cursor = db.cursor()
cursor.execute("""
CREATE TABLE IF NOT EXISTS books (
id INTEGER PRIMARY KEY,
title TEXT,
author TEXT,
price REAL
)
""")
db.commit()
爬取书籍信息
接下来,我们使用requests和BeautifulSoup库爬取书籍信息。
import requests
from bs4 import BeautifulSoup
url = "http://example.com/books"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, "html.parser")
books = soup.find_all("div", class_="book")
for book in books:
title = book.find("h3").text
author = book.find("p", class_="author").text
price = float(book.find("p", class_="price").text.strip("$"))
cursor.execute("INSERT INTO books (title, author, price) VALUES (?, ?, ?)", (title, author, price))
db.commit()
else:
print("Failed to retrieve the webpage")
完整代码
import sqlite3
import requests
from bs4 import BeautifulSoup
创建数据库
db = sqlite3.connect("books.db")
cursor = db.cursor()
cursor.execute("""
CREATE TABLE IF NOT EXISTS books (
id INTEGER PRIMARY KEY,
title TEXT,
author TEXT,
price REAL
)
""")
db.commit()
爬取书籍信息
url = "http://example.com/books"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, "html.parser")
books = soup.find_all("div", class_="book")
for book in books:
title = book.find("h3").text
author = book.find("p", class_="author").text
price = float(book.find("p", class_="price").text.strip("$"))
cursor.execute("INSERT INTO books (title, author, price) VALUES (?, ?, ?)", (title, author, price))
db.commit()
else:
print("Failed to retrieve the webpage")
db.close()
这个案例展示了一个完整的爬虫项目,从创建数据库、爬取数据到存储数据的全过程。通过这个案例,我们可以更好地理解Python爬取软件数据的方法和技巧,并在实际项目中灵活应用。
相关问答FAQs:
如何选择合适的Python库来爬取软件数据?
在爬取软件数据时,选择合适的Python库至关重要。常用的库包括Requests、BeautifulSoup和Scrapy。Requests库用于发送HTTP请求,获取网页内容;BeautifulSoup则帮助解析HTML文档,提取所需信息;Scrapy是一个强大的爬虫框架,适合进行复杂的爬取任务。根据需求选择合适的工具,可以提高开发效率和爬取的准确性。
在爬取软件数据时,有哪些常见的反爬虫机制需要注意?
许多网站为防止数据被自动抓取,采取了反爬虫措施。这些措施包括IP限制、验证码、用户代理检测等。为了绕过这些限制,可以考虑使用代理IP池、随机设置用户代理以及增加请求间隔时间等策略,以降低被识别为爬虫的风险。
如何处理爬取到的数据,以便进行分析和存储?
一旦成功爬取数据,接下来需要对数据进行清洗和存储。可以使用Pandas库将数据转化为数据框格式,方便进行处理和分析。此外,数据可以存储在多种格式中,如CSV、JSON或数据库(如SQLite、MySQL),根据后续的使用需求选择合适的存储方式,将有助于数据的高效管理和后续分析。
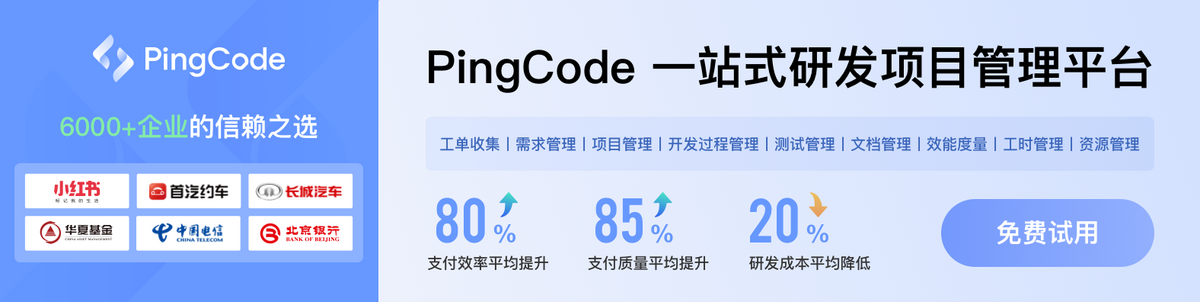