删除Python输出中的空格有多种方法,包括使用字符串方法、正则表达式、格式化输出等。在这些方法中,使用字符串方法是最常见和简便的方式。
1. 使用字符串方法:strip()、lstrip()、rstrip()、replace()
2. 使用正则表达式:re.sub()
3. 使用格式化输出:格式化字符串和f-strings
其中,使用字符串方法是最常见和简便的方式。例如,strip()方法可以用来删除字符串两端的空格,而replace()方法可以用来删除字符串中的所有空格。具体的代码示例如下:
# 使用strip()方法删除字符串两端的空格
s = " Hello, World! "
print(s.strip()) # 输出:Hello, World!
使用replace()方法删除字符串中的所有空格
s = "H e l l o , W o r l d !"
print(s.replace(" ", "")) # 输出:Hello,World!
一、使用字符串方法删除空格
1. strip()、lstrip()和rstrip()
strip()、lstrip()和rstrip()都是用来删除字符串两端或单端空格的方法。
- strip():删除字符串开头和结尾的空格。
- lstrip():删除字符串开头的空格。
- rstrip():删除字符串结尾的空格。
示例代码:
s = " Hello, World! "
print(s.strip()) # 输出:Hello, World!
print(s.lstrip()) # 输出:Hello, World!
print(s.rstrip()) # 输出: Hello, World!
2. replace()
replace()方法可以用来替换字符串中的字符,可以用来删除字符串中的所有空格。
示例代码:
s = "H e l l o , W o r l d !"
print(s.replace(" ", "")) # 输出:Hello,World!
二、使用正则表达式删除空格
正则表达式是处理字符串的强大工具,可以用来匹配和替换字符串中的模式。Python中的re模块提供了正则表达式的支持。
1. 使用re.sub()删除空格
re.sub()函数可以用来替换字符串中的模式,可以用来删除字符串中的所有空格。
示例代码:
import re
s = "H e l l o , W o r l d !"
print(re.sub(r'\s+', '', s)) # 输出:Hello,World!
三、使用格式化输出删除空格
Python提供了多种字符串格式化方法,可以用来生成格式化的字符串输出。这些方法包括%格式化、str.format()和f-strings。
1. 使用%格式化删除空格
%格式化可以用来生成格式化的字符串输出,可以用来删除字符串中的空格。
示例代码:
s = "H e l l o , W o r l d !"
formatted_s = "%s" % s.replace(" ", "")
print(formatted_s) # 输出:Hello,World!
2. 使用str.format()删除空格
str.format()方法可以用来生成格式化的字符串输出,可以用来删除字符串中的空格。
示例代码:
s = "H e l l o , W o r l d !"
formatted_s = "{}".format(s.replace(" ", ""))
print(formatted_s) # 输出:Hello,World!
3. 使用f-strings删除空格
f-strings是Python 3.6引入的一种新的字符串格式化方法,可以用来生成格式化的字符串输出,可以用来删除字符串中的空格。
示例代码:
s = "H e l l o , W o r l d !"
formatted_s = f"{s.replace(' ', '')}"
print(formatted_s) # 输出:Hello,World!
四、删除列表或字典中的空格
有时候,我们需要删除列表或字典中的空格。可以使用列表推导式或字典推导式来实现。
1. 删除列表中的空格
示例代码:
list_with_spaces = [" Hello ", " World ", " ! "]
cleaned_list = [s.strip() for s in list_with_spaces]
print(cleaned_list) # 输出:['Hello', 'World', '!']
2. 删除字典中的空格
示例代码:
dict_with_spaces = {"key1": " value1 ", "key2": " value2 "}
cleaned_dict = {k: v.strip() for k, v in dict_with_spaces.items()}
print(cleaned_dict) # 输出:{'key1': 'value1', 'key2': 'value2'}
五、删除文件中的空格
有时候,我们需要删除文件中的空格,可以使用Python的文件操作功能来实现。
1. 删除文件中的所有空格
示例代码:
with open("input.txt", "r") as file:
content = file.read()
cleaned_content = content.replace(" ", "")
with open("output.txt", "w") as file:
file.write(cleaned_content)
2. 删除文件中每行开头和结尾的空格
示例代码:
with open("input.txt", "r") as file:
lines = file.readlines()
cleaned_lines = [line.strip() for line in lines]
with open("output.txt", "w") as file:
file.writelines(cleaned_lines)
六、删除字符串中的特定字符
有时候,我们可能不仅需要删除空格,还需要删除其他特定的字符。可以使用字符串方法或正则表达式来实现。
1. 使用字符串方法删除特定字符
示例代码:
s = "H#e#l#l#o#,##W#o#r#l#d#!"
cleaned_s = s.replace("#", "")
print(cleaned_s) # 输出:Hello,World!
2. 使用正则表达式删除特定字符
示例代码:
import re
s = "H#e#l#l#o#,##W#o#r#l#d#!"
cleaned_s = re.sub(r'#', '', s)
print(cleaned_s) # 输出:Hello,World!
七、删除字符串中的多余空格
有时候,字符串中可能有多个连续的空格,我们需要将其替换为单个空格。可以使用正则表达式来实现。
1. 使用正则表达式删除多余空格
示例代码:
import re
s = "Hello, World! How are you?"
cleaned_s = re.sub(r'\s+', ' ', s)
print(cleaned_s) # 输出:Hello, World! How are you?
八、删除字符串中的空行
有时候,字符串中可能包含空行,我们需要将其删除。可以使用字符串方法或正则表达式来实现。
1. 使用字符串方法删除空行
示例代码:
s = "Hello, World!\n\nHow are you?\n\n"
cleaned_s = "\n".join([line for line in s.split("\n") if line.strip() != ""])
print(cleaned_s) # 输出:Hello, World!\nHow are you?
2. 使用正则表达式删除空行
示例代码:
import re
s = "Hello, World!\n\nHow are you?\n\n"
cleaned_s = re.sub(r'\n\s*\n', '\n', s)
print(cleaned_s) # 输出:Hello, World!\nHow are you?
九、删除字符串中的特定模式
有时候,字符串中可能包含特定的模式,我们需要将其删除。可以使用正则表达式来实现。
1. 使用正则表达式删除特定模式
示例代码:
import re
s = "Hello, [World]! How are [you]?"
cleaned_s = re.sub(r'\[.*?\]', '', s)
print(cleaned_s) # 输出:Hello, ! How are ?
十、总结
删除Python输出中的空格有多种方法,包括使用字符串方法、正则表达式、格式化输出等。根据具体的需求,可以选择合适的方法来删除空格或特定字符。使用字符串方法是最常见和简便的方式,适用于大多数场景。而正则表达式则更为灵活和强大,适用于更复杂的字符串处理需求。
通过了解和掌握这些方法,可以更高效地处理字符串,删除不需要的空格或字符,从而生成更为整洁和规范的输出。无论是处理单个字符串、列表、字典,还是文件,都可以找到合适的解决方案来达到预期的效果。
相关问答FAQs:
如何在Python中去除字符串中的多余空格?
在Python中,可以使用strip()
方法来删除字符串开头和结尾的空格。对于字符串中间的多余空格,可以使用replace()
方法或正则表达式库re
来实现。例如,使用re.sub()
可以将多个空格替换为一个空格。
使用Python删除文件中每行的空格的方法是什么?
可以使用Python的文件读取功能,逐行读取文件并利用strip()
或replace()
方法去除每行的空格。处理完成后,可以将结果写入新的文件中,或者覆盖原文件。
在Python中,如何在打印输出时去除多余的空格?
可以通过使用字符串格式化方法或在print()
函数中应用strip()
或其他字符串处理方法,来确保输出内容中没有多余的空格。例如,使用print(my_string.strip())
可以确保只输出去除空格后的字符串。
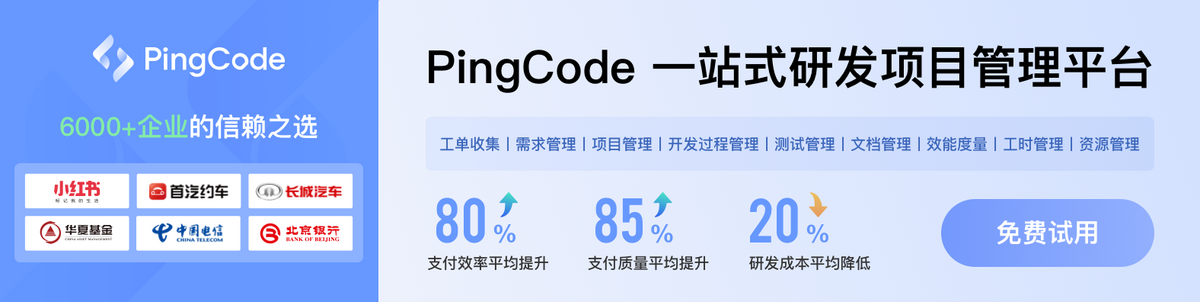