在Python中添加一个时钟箭头,可以通过多个方法实现。使用图形界面库(如Tkinter、PyQt或Pygame)、使用数学公式计算箭头位置、使用时间模块获取当前时间,这些都是常见的解决方案。以下将详细描述如何使用Tkinter库来创建一个时钟并添加箭头。
一、使用Tkinter库创建时钟
Tkinter是Python内置的图形界面库,使用它可以简单快速地创建图形界面程序。我们将使用Tkinter绘制一个时钟,并通过数学公式和时间模块来确定箭头的位置。
1、安装并导入Tkinter库
首先,确保你已经安装了Tkinter库。大多数Python安装包都自带Tkinter库,但如果你使用的是精简版的Python,可以通过以下命令安装:
pip install tk
在你的Python脚本中导入Tkinter库:
import tkinter as tk
import time
import math
2、创建基础时钟界面
创建一个Tkinter窗口,并在其中绘制一个圆形表示时钟的表盘:
root = tk.Tk()
root.title("Analog Clock")
canvas = tk.Canvas(root, width=400, height=400, bg="white")
canvas.pack()
绘制表盘
canvas.create_oval(50, 50, 350, 350, outline="black", width=2)
3、计算箭头位置
我们需要使用数学公式来计算箭头的位置。时钟上的时、分、秒针位置可以通过三角函数计算:
def update_clock():
canvas.delete("hands")
# 获取当前时间
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
# 计算时针位置
hour_angle = math.radians(360 * (hours / 12) - 90)
hour_x = 200 + 50 * math.cos(hour_angle)
hour_y = 200 + 50 * math.sin(hour_angle)
canvas.create_line(200, 200, hour_x, hour_y, fill="black", width=6, tags="hands")
# 计算分针位置
minute_angle = math.radians(360 * (minutes / 60) - 90)
minute_x = 200 + 70 * math.cos(minute_angle)
minute_y = 200 + 70 * math.sin(minute_angle)
canvas.create_line(200, 200, minute_x, minute_y, fill="black", width=4, tags="hands")
# 计算秒针位置
second_angle = math.radians(360 * (seconds / 60) - 90)
second_x = 200 + 90 * math.cos(second_angle)
second_y = 200 + 90 * math.sin(second_angle)
canvas.create_line(200, 200, second_x, second_y, fill="red", width=2, tags="hands")
root.after(1000, update_clock)
初始调用
update_clock()
root.mainloop()
二、详细解释箭头位置计算
1、时针位置计算
时针位置的计算是基于当前小时数。时针在表盘上以每小时30度的速度移动。为了将时针从12点开始向右移动,我们将角度减去90度(即math.radians(360 * (hours / 12) – 90))。
2、分针位置计算
分针位置的计算与时针类似,但分针每分钟移动6度。计算公式为math.radians(360 * (minutes / 60) – 90)。
3、秒针位置计算
秒针每秒钟移动6度。计算公式为math.radians(360 * (seconds / 60) – 90)。
三、优化代码并增强功能
1、添加数字刻度
在表盘上添加数字刻度可以让时钟更加直观。我们可以通过以下代码实现:
def draw_ticks():
for i in range(12):
angle = math.radians(360 * (i / 12) - 90)
x = 200 + 90 * math.cos(angle)
y = 200 + 90 * math.sin(angle)
canvas.create_text(x, y, text=str(i + 1), fill="black")
draw_ticks()
2、添加背景颜色和样式
为了让时钟更加美观,可以添加背景颜色和样式:
canvas.configure(bg="light blue")
3、增加动态更新效果
为了使箭头动态更新,我们需要在update_clock函数中调用root.after方法。这个方法会在指定的时间间隔后再次调用update_clock:
def update_clock():
canvas.delete("hands")
# 获取当前时间
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
# 计算时针位置
hour_angle = math.radians(360 * (hours / 12) - 90)
hour_x = 200 + 50 * math.cos(hour_angle)
hour_y = 200 + 50 * math.sin(hour_angle)
canvas.create_line(200, 200, hour_x, hour_y, fill="black", width=6, tags="hands")
# 计算分针位置
minute_angle = math.radians(360 * (minutes / 60) - 90)
minute_x = 200 + 70 * math.cos(minute_angle)
minute_y = 200 + 70 * math.sin(minute_angle)
canvas.create_line(200, 200, minute_x, minute_y, fill="black", width=4, tags="hands")
# 计算秒针位置
second_angle = math.radians(360 * (seconds / 60) - 90)
second_x = 200 + 90 * math.cos(second_angle)
second_y = 200 + 90 * math.sin(second_angle)
canvas.create_line(200, 200, second_x, second_y, fill="red", width=2, tags="hands")
root.after(1000, update_clock)
初始调用
update_clock()
root.mainloop()
四、总结
通过以上步骤,我们使用Tkinter库创建了一个简单的模拟时钟,并通过数学公式计算时、分、秒针的位置。使用Tkinter绘制表盘、通过数学公式计算箭头位置、使用时间模块获取当前时间,这些方法使得我们的时钟可以准确显示当前时间并且动态更新。这个项目不仅展示了Tkinter库的强大功能,还让我们更深入地理解了如何在Python中进行图形界面编程和时间处理。
五、进一步拓展
1、增加日期显示
我们可以在时钟界面上添加当前日期显示,使得时钟功能更加完善:
def update_date():
current_time = time.localtime()
date_str = time.strftime("%Y-%m-%d", current_time)
canvas.create_text(200, 370, text=date_str, fill="black", font=("Arial", 14), tags="date")
def update_clock():
canvas.delete("hands")
canvas.delete("date")
# 获取当前时间
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
# 计算时针位置
hour_angle = math.radians(360 * (hours / 12) - 90)
hour_x = 200 + 50 * math.cos(hour_angle)
hour_y = 200 + 50 * math.sin(hour_angle)
canvas.create_line(200, 200, hour_x, hour_y, fill="black", width=6, tags="hands")
# 计算分针位置
minute_angle = math.radians(360 * (minutes / 60) - 90)
minute_x = 200 + 70 * math.cos(minute_angle)
minute_y = 200 + 70 * math.sin(minute_angle)
canvas.create_line(200, 200, minute_x, minute_y, fill="black", width=4, tags="hands")
# 计算秒针位置
second_angle = math.radians(360 * (seconds / 60) - 90)
second_x = 200 + 90 * math.cos(second_angle)
second_y = 200 + 90 * math.sin(second_angle)
canvas.create_line(200, 200, second_x, second_y, fill="red", width=2, tags="hands")
# 更新日期
update_date()
root.after(1000, update_clock)
初始调用
update_clock()
root.mainloop()
2、添加定时功能
我们还可以为时钟添加定时功能,设置一个特定时间点,时钟到达该时间点时会触发一个事件:
alarm_time = "15:30"
def check_alarm():
current_time = time.strftime("%H:%M", time.localtime())
if current_time == alarm_time:
print("Time to take a break!")
# 可以添加更多的提醒功能,比如弹出窗口或者播放声音
def update_clock():
canvas.delete("hands")
canvas.delete("date")
# 获取当前时间
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
# 计算时针位置
hour_angle = math.radians(360 * (hours / 12) - 90)
hour_x = 200 + 50 * math.cos(hour_angle)
hour_y = 200 + 50 * math.sin(hour_angle)
canvas.create_line(200, 200, hour_x, hour_y, fill="black", width=6, tags="hands")
# 计算分针位置
minute_angle = math.radians(360 * (minutes / 60) - 90)
minute_x = 200 + 70 * math.cos(minute_angle)
minute_y = 200 + 70 * math.sin(minute_angle)
canvas.create_line(200, 200, minute_x, minute_y, fill="black", width=4, tags="hands")
# 计算秒针位置
second_angle = math.radians(360 * (seconds / 60) - 90)
second_x = 200 + 90 * math.cos(second_angle)
second_y = 200 + 90 * math.sin(second_angle)
canvas.create_line(200, 200, second_x, second_y, fill="red", width=2, tags="hands")
# 更新日期
update_date()
# 检查闹钟
check_alarm()
root.after(1000, update_clock)
初始调用
update_clock()
root.mainloop()
通过添加这些功能,我们的时钟不仅可以显示当前时间,还能显示日期并提供定时提醒功能。添加日期显示、增加定时功能、进一步美化界面,这些拓展使得我们的时钟项目更加实用和完善。
相关问答FAQs:
如何在Python中创建一个带有箭头的时钟?
在Python中,可以使用Tkinter和Matplotlib等库来创建一个带箭头的时钟。使用Matplotlib可以绘制时钟的指针,Tkinter则可以创建图形界面。通过设置时钟的时间和箭头的角度,可以实现动态效果。
有哪些Python库可以帮助我绘制时钟指针?
常用的库包括Matplotlib、Tkinter和Pygame。Matplotlib提供了强大的绘图功能,可以绘制时钟的各个部分并添加动态效果;Tkinter适合简单的GUI应用,能够直接在窗口中绘制;而Pygame则适合制作更复杂的游戏或动画效果。
如何在我的时钟中实现动态更新?
动态更新可以通过使用定时器或循环来实现。在Matplotlib中,可以使用FuncAnimation
来定期更新时钟指针的位置;在Tkinter中,可以使用after()
方法来设置每秒更新一次的功能。这些方法可以确保时钟的时间随着系统时间的变化而更新。
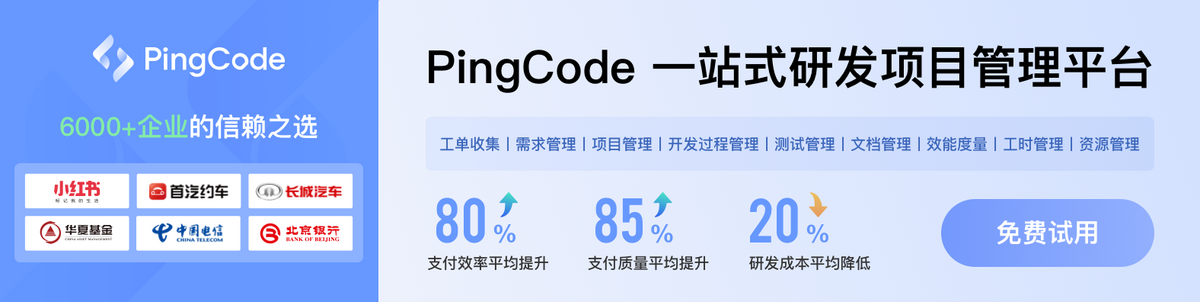