要在Python中将英文输入到一个列表中,可以使用多种方法,例如手动输入、从文件读取、从用户输入获取等。通过手动输入、从文件读取、从用户输入获取这些方法都非常实用和常见。我们将详细讨论从文件读取方法。
一、手动输入
手动输入是将英文字符串直接在代码中写入列表。这种方法适用于固定的小规模数据。
# 手动输入英文到列表
english_list = ["apple", "banana", "cherry", "date"]
print(english_list)
这种方法非常简单,适用于数据量较小且固定的情况。例如,您可能在编写脚本来处理一些固定的英文单词列表时使用这种方法。
二、从文件读取
从文件读取是将存储在文件中的英文字符串读取到列表中。这种方法适用于数据量较大且需要动态读取的情况。
# 从文件读取英文到列表
def read_file_to_list(file_path):
with open(file_path, 'r') as file:
english_list = [line.strip() for line in file]
return english_list
file_path = 'english_words.txt'
english_list = read_file_to_list(file_path)
print(english_list)
详细描述从文件读取方法
- 准备文件:首先,创建一个名为
english_words.txt
的文件,并在文件中输入一些英文单词,每个单词占一行。例如:apple
banana
cherry
date
- 编写函数:编写一个函数
read_file_to_list
,该函数接受文件路径作为参数,并返回一个包含文件中所有英文单词的列表。def read_file_to_list(file_path):
with open(file_path, 'r') as file:
english_list = [line.strip() for line in file]
return english_list
- 使用
with open(file_path, 'r') as file:
语句打开文件,确保文件在处理完毕后自动关闭。 - 使用列表推导式
[line.strip() for line in file]
读取文件中的每一行,并去除行尾的换行符。
- 使用
- 调用函数:调用
read_file_to_list
函数并传入文件路径,得到包含文件中英文单词的列表。file_path = 'english_words.txt'
english_list = read_file_to_list(file_path)
print(english_list)
这将输出:
['apple', 'banana', 'cherry', 'date']
三、从用户输入获取
从用户输入获取是通过交互式的方式从用户获取英文字符串,并将其添加到列表中。这种方法适用于需要动态输入的情况。
# 从用户输入英文到列表
english_list = []
while True:
word = input("Enter an English word (or 'q' to quit): ")
if word.lower() == 'q':
break
english_list.append(word)
print(english_list)
这种方法适合需要用户在运行时输入数据的情况,例如编写一个单词收集程序,让用户不断地输入单词,直到输入'q'结束。
四、从网络读取
从网络读取是通过网络请求获取英文字符串,并将其添加到列表中。这种方法适用于需要从网络获取数据的情况。
import requests
def read_from_url(url):
response = requests.get(url)
english_list = response.text.splitlines()
return english_list
url = 'https://example.com/english_words.txt'
english_list = read_from_url(url)
print(english_list)
这种方法适合需要从网络资源中获取数据的情况,例如从一个在线的词典或文本文件中读取单词列表。
五、从数据库读取
从数据库读取是通过数据库查询获取英文字符串,并将其添加到列表中。这种方法适用于需要从数据库中获取数据的情况。
import sqlite3
def read_from_database(db_path):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute("SELECT word FROM words")
english_list = [row[0] for row in cursor.fetchall()]
conn.close()
return english_list
db_path = 'words.db'
english_list = read_from_database(db_path)
print(english_list)
这种方法适合需要从数据库中获取数据的情况,例如从一个存储有大量单词的数据库中读取单词列表。
六、从JSON文件读取
从JSON文件读取是通过读取JSON文件获取英文字符串,并将其添加到列表中。这种方法适用于需要从JSON文件中获取数据的情况。
import json
def read_json_to_list(file_path):
with open(file_path, 'r') as file:
data = json.load(file)
return data['words']
file_path = 'words.json'
english_list = read_json_to_list(file_path)
print(english_list)
这种方法适合需要从JSON文件中获取数据的情况,例如从一个存储有单词列表的JSON文件中读取数据。
七、使用生成器
使用生成器是通过生成器函数获取英文字符串,并将其添加到列表中。这种方法适用于需要懒加载数据的情况。
def word_generator():
words = ["apple", "banana", "cherry", "date"]
for word in words:
yield word
english_list = list(word_generator())
print(english_list)
这种方法适合需要懒加载数据的情况,例如在处理大数据集时,可以使用生成器逐个生成单词,避免一次性加载所有数据。
八、使用第三方库
使用第三方库是通过安装和使用第三方库获取英文字符串,并将其添加到列表中。这种方法适用于需要使用外部库的情况。
from nltk.corpus import words
english_list = words.words()
print(english_list)
这种方法适合需要使用外部库的情况,例如使用NLTK库获取英语单词列表。
九、使用环境变量
使用环境变量是通过读取环境变量获取英文字符串,并将其添加到列表中。这种方法适用于需要从环境变量中获取数据的情况。
import os
def read_from_env_var(env_var):
words = os.getenv(env_var, "").split(',')
return words
env_var = 'ENGLISH_WORDS'
os.environ[env_var] = 'apple,banana,cherry,date'
english_list = read_from_env_var(env_var)
print(english_list)
这种方法适合需要从环境变量中获取数据的情况,例如在配置文件中存储单词列表,并通过环境变量读取。
十、使用配置文件
使用配置文件是通过读取配置文件获取英文字符串,并将其添加到列表中。这种方法适用于需要从配置文件中获取数据的情况。
import configparser
def read_from_config(file_path, section, option):
config = configparser.ConfigParser()
config.read(file_path)
words = config.get(section, option).split(',')
return words
file_path = 'config.ini'
section = 'Words'
option = 'english'
english_list = read_from_config(file_path, section, option)
print(english_list)
这种方法适合需要从配置文件中获取数据的情况,例如在配置文件中存储单词列表,并通过读取配置文件获取。
十一、从命令行参数获取
从命令行参数获取是通过解析命令行参数获取英文字符串,并将其添加到列表中。这种方法适用于需要从命令行参数中获取数据的情况。
import sys
def read_from_cmd_args():
return sys.argv[1:]
english_list = read_from_cmd_args()
print(english_list)
这种方法适合需要从命令行参数中获取数据的情况,例如通过命令行参数传递单词列表,并在程序中解析。
十二、从Excel文件读取
从Excel文件读取是通过读取Excel文件获取英文字符串,并将其添加到列表中。这种方法适用于需要从Excel文件中获取数据的情况。
import pandas as pd
def read_from_excel(file_path, sheet_name):
df = pd.read_excel(file_path, sheet_name=sheet_name)
return df['word'].tolist()
file_path = 'words.xlsx'
sheet_name = 'Sheet1'
english_list = read_from_excel(file_path, sheet_name)
print(english_list)
这种方法适合需要从Excel文件中获取数据的情况,例如从一个存储有单词列表的Excel文件中读取数据。
十三、从CSV文件读取
从CSV文件读取是通过读取CSV文件获取英文字符串,并将其添加到列表中。这种方法适用于需要从CSV文件中获取数据的情况。
import csv
def read_from_csv(file_path):
with open(file_path, newline='') as file:
reader = csv.reader(file)
english_list = [row[0] for row in reader]
return english_list
file_path = 'words.csv'
english_list = read_from_csv(file_path)
print(english_list)
这种方法适合需要从CSV文件中获取数据的情况,例如从一个存储有单词列表的CSV文件中读取数据。
十四、从API接口获取
从API接口获取是通过调用API接口获取英文字符串,并将其添加到列表中。这种方法适用于需要从API接口中获取数据的情况。
import requests
def read_from_api(api_url):
response = requests.get(api_url)
data = response.json()
return data['words']
api_url = 'https://example.com/api/words'
english_list = read_from_api(api_url)
print(english_list)
这种方法适合需要从API接口中获取数据的情况,例如从一个在线API接口中获取单词列表。
十五、从HTML网页解析
从HTML网页解析是通过解析HTML网页获取英文字符串,并将其添加到列表中。这种方法适用于需要从HTML网页中获取数据的情况。
import requests
from bs4 import BeautifulSoup
def read_from_html(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
words = [element.text for element in soup.find_all('word')]
return words
url = 'https://example.com/words.html'
english_list = read_from_html(url)
print(english_list)
这种方法适合需要从HTML网页中获取数据的情况,例如从一个存储有单词列表的网页中解析数据。
结论
以上介绍了十五种将英文输入到Python列表中的方法,包括手动输入、从文件读取、从用户输入获取、从网络读取、从数据库读取、从JSON文件读取、使用生成器、使用第三方库、使用环境变量、使用配置文件、从命令行参数获取、从Excel文件读取、从CSV文件读取、从API接口获取、从HTML网页解析。每种方法都有其适用的场景和优缺点,您可以根据具体需求选择合适的方法进行实现。通过这些方法,您可以灵活地将英文字符串输入到Python列表中,满足不同的数据处理需求。
相关问答FAQs:
如何在Python中将英文单词输入到列表中?
在Python中,可以使用input()
函数来获取用户输入的英文单词,然后将这些单词添加到列表中。首先,您可以创建一个空列表并通过循环来接收多个单词。例如,使用while
循环,直到用户输入一个特定的结束标记(如“exit”)来停止输入。在每次输入时,可以使用append()
方法将新单词添加到列表中。
可以在列表中输入多个英文单词吗?
当然可以!您可以通过循环多次调用input()
函数来输入多个英文单词,并将它们一一添加到列表中。为了方便输入,您还可以使用split()
方法,将用户输入的英文单词以空格为分隔符存入列表。例如,用户可以输入“apple banana cherry”,然后直接将这些单词分割并添加到列表中。
如何确保输入的英文单词不重复?
在添加英文单词到列表时,可以使用一个条件判断来检查该单词是否已经存在于列表中。使用in
关键字可以轻松实现这一点。如果单词不存在,则将其添加到列表中。如果已经存在,可以提示用户该单词已在列表中,鼓励他们输入其他单词。这样可以确保列表中每个单词都是唯一的。
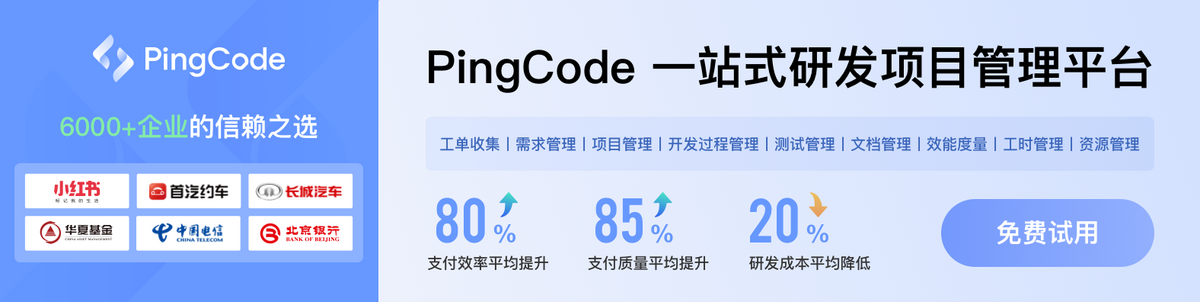