要用Python爬虫搜索资料,主要有以下几个步骤:选择合适的爬虫库、模拟浏览器请求、解析网页内容、处理反爬机制。在这些步骤中,选择合适的爬虫库是最重要的一步,因为它决定了你整个爬虫项目的开发效率和成功率。
选择合适的爬虫库是爬虫项目的关键一步。Python有许多优秀的爬虫库,如requests
、BeautifulSoup
、Scrapy
等。对于初学者来说,requests
和BeautifulSoup
是最常用的组合,它们简单易用且功能强大。requests
用于发送HTTP请求,而BeautifulSoup
用于解析HTML内容,提取所需信息。对于更复杂的爬虫项目,Scrapy
是一个更专业的选择,它提供了更多高级功能,如分布式爬取、自动处理反爬机制等。
一、选择合适的爬虫库
选择合适的爬虫库是成功开发爬虫项目的基础。不同的爬虫库有不同的优缺点,适用于不同的场景。
1、Requests库
requests
库是一个简单易用的HTTP库,它可以用于发送HTTP请求,获取网页内容。下面是一个简单的例子,展示如何使用requests
库发送一个GET请求:
import requests
response = requests.get('https://www.example.com')
print(response.text)
2、BeautifulSoup库
BeautifulSoup
库是一个用于解析HTML和XML的库,它可以将复杂的HTML文档转换成一个易于处理的树形结构。下面是一个简单的例子,展示如何使用BeautifulSoup
库解析一个HTML文档:
from bs4 import BeautifulSoup
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.prettify())
3、Scrapy库
Scrapy
是一个强大的爬虫框架,它提供了丰富的功能,如分布式爬取、自动处理反爬机制等。下面是一个简单的例子,展示如何使用Scrapy
库创建一个爬虫项目:
import scrapy
class QuotesSpider(scrapy.Spider):
name = 'quotes'
start_urls = [
'http://quotes.toscrape.com/page/1/',
]
def parse(self, response):
for quote in response.css('div.quote'):
yield {
'text': quote.css('span.text::text').get(),
'author': quote.css('span small.author::text').get(),
'tags': quote.css('div.tags a.tag::text').getall(),
}
next_page = response.css('li.next a::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
二、模拟浏览器请求
在爬虫项目中,模拟浏览器请求是非常重要的一步。很多网站会检测请求的来源,如果发现请求不是来自浏览器,可能会拒绝响应或者返回错误信息。为了避免这种情况,我们可以在请求中添加一些浏览器请求头信息,使请求看起来像是来自浏览器。
1、添加User-Agent
User-Agent是一个HTTP头字段,用于标识客户端的类型。我们可以在请求中添加一个User-Agent字段,使请求看起来像是来自某个浏览器。下面是一个使用requests
库添加User-Agent字段的例子:
import requests
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36',
}
response = requests.get('https://www.example.com', headers=headers)
print(response.text)
2、处理Cookies
有些网站会使用Cookies来跟踪用户的行为,如果没有正确处理Cookies,可能会导致请求被拒绝。我们可以使用requests
库的Session
对象来处理Cookies。下面是一个使用Session
对象处理Cookies的例子:
import requests
session = requests.Session()
response = session.get('https://www.example.com')
print(response.cookies)
使用会话对象发送请求,自动处理Cookies
response = session.get('https://www.example.com/another-page')
print(response.text)
三、解析网页内容
在获取网页内容后,下一步是解析网页内容,提取所需的信息。我们可以使用BeautifulSoup
库或者lxml
库来解析HTML文档。
1、使用BeautifulSoup解析HTML
BeautifulSoup
库可以将HTML文档转换成一个易于处理的树形结构,然后我们可以使用各种方法来查找和提取所需的信息。下面是一个使用BeautifulSoup
库解析HTML文档的例子:
from bs4 import BeautifulSoup
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
查找所有<a>标签
links = soup.find_all('a')
for link in links:
print(link.get('href'))
查找带有class属性的<p>标签
story_paragraph = soup.find('p', class_='story')
print(story_paragraph.text)
2、使用lxml解析HTML
lxml
库是一个高性能的HTML和XML解析库,它提供了丰富的功能和更高的解析速度。下面是一个使用lxml
库解析HTML文档的例子:
from lxml import etree
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
tree = etree.HTML(html_doc)
查找所有<a>标签
links = tree.xpath('//a')
for link in links:
print(link.get('href'))
查找带有class属性的<p>标签
story_paragraph = tree.xpath('//p[@class="story"]')[0]
print(story_paragraph.text)
四、处理反爬机制
在爬虫项目中,处理反爬机制是一个重要的问题。很多网站会使用各种反爬机制来防止爬虫抓取数据,如IP封禁、验证码、人机验证等。为了绕过这些反爬机制,我们可以使用一些技巧和工具。
1、使用代理IP
使用代理IP是绕过IP封禁的一种常用方法。我们可以使用一些免费的或者付费的代理IP服务,将请求通过代理IP发送,从而避免被封禁。下面是一个使用requests
库和代理IP发送请求的例子:
import requests
proxies = {
'http': 'http://123.456.789.012:8080',
'https': 'http://123.456.789.012:8080',
}
response = requests.get('https://www.example.com', proxies=proxies)
print(response.text)
2、处理验证码
处理验证码是一个比较复杂的问题,通常需要使用一些图像识别技术或者第三方验证码识别服务。下面是一个使用pytesseract
库识别验证码的例子:
from PIL import Image
import pytesseract
打开验证码图片
image = Image.open('captcha.png')
使用pytesseract识别验证码
captcha_text = pytesseract.image_to_string(image)
print(captcha_text)
3、模拟用户行为
模拟用户行为是绕过人机验证的一种方法,如模拟鼠标移动、点击、滚动等操作。我们可以使用一些自动化测试工具,如Selenium
,来模拟用户行为。下面是一个使用Selenium
模拟用户行为的例子:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
启动浏览器
driver = webdriver.Chrome()
打开网页
driver.get('https://www.example.com')
模拟鼠标滚动
driver.execute_script('window.scrollTo(0, document.body.scrollHeight);')
time.sleep(2)
模拟点击
element = driver.find_element(By.XPATH, '//button[@id="submit-button"]')
element.click()
time.sleep(2)
关闭浏览器
driver.quit()
五、数据存储
在成功抓取到数据后,下一步是将数据存储到合适的地方,以便后续分析和处理。我们可以将数据存储到文件、数据库或者云存储中。
1、存储到文件
将数据存储到文件是最简单的一种方法,我们可以使用Python的内置文件操作函数,将数据写入文本文件、CSV文件或者JSON文件。下面是一个将数据存储到CSV文件的例子:
import csv
data = [
['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles'],
['Charlie', 35, 'Chicago'],
]
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
2、存储到数据库
将数据存储到数据库是一种更专业的方法,我们可以使用关系数据库(如MySQL、PostgreSQL)或者NoSQL数据库(如MongoDB)来存储数据。下面是一个将数据存储到MySQL数据库的例子:
import mysql.connector
连接到MySQL数据库
conn = mysql.connector.connect(
host='localhost',
user='root',
password='password',
database='test_db'
)
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255),
age INT,
city VARCHAR(255)
)
''')
插入数据
data = [
('Alice', 30, 'New York'),
('Bob', 25, 'Los Angeles'),
('Charlie', 35, 'Chicago'),
]
cursor.executemany('INSERT INTO users (name, age, city) VALUES (%s, %s, %s)', data)
提交事务
conn.commit()
关闭连接
cursor.close()
conn.close()
3、存储到云存储
将数据存储到云存储是一种方便的方法,我们可以使用一些云存储服务,如AWS S3、Google Cloud Storage、Azure Blob Storage等,将数据存储到云端。下面是一个将数据存储到AWS S3的例子:
import boto3
创建S3客户端
s3 = boto3.client('s3')
上传文件到S3
s3.upload_file('data.csv', 'my-bucket', 'data.csv')
六、数据清洗和处理
在成功抓取到数据并存储后,下一步是对数据进行清洗和处理,以便后续分析和使用。数据清洗和处理的步骤包括去重、填充缺失值、数据转换等。
1、去重
去重是数据清洗的一步,目的是去除重复的数据记录。我们可以使用Pandas库来进行数据去重。下面是一个使用Pandas库进行数据去重的例子:
import pandas as pd
data = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'Alice'],
'age': [30, 25, 35, 30],
'city': ['New York', 'Los Angeles', 'Chicago', 'New York']
})
去重
data = data.drop_duplicates()
print(data)
2、填充缺失值
填充缺失值是数据清洗的一步,目的是填补数据中的空缺值。我们可以使用Pandas库来填充缺失值。下面是一个使用Pandas库填充缺失值的例子:
import pandas as pd
data = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', None],
'age': [30, 25, 35, None],
'city': ['New York', 'Los Angeles', 'Chicago', None]
})
填充缺失值
data = data.fillna({
'name': 'Unknown',
'age': data['age'].mean(),
'city': 'Unknown'
})
print(data)
3、数据转换
数据转换是数据处理的一步,目的是将数据转换成合适的格式。我们可以使用Pandas库来进行数据转换。下面是一个使用Pandas库进行数据转换的例子:
import pandas as pd
data = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [30, 25, 35],
'city': ['New York', 'Los Angeles', 'Chicago']
})
数据转换:将年龄转换成字符串
data['age'] = data['age'].astype(str)
print(data)
七、数据分析和可视化
在成功清洗和处理数据后,下一步是对数据进行分析和可视化,以便从数据中获取有价值的信息。我们可以使用Pandas库进行数据分析,使用Matplotlib和Seaborn库进行数据可视化。
1、数据分析
数据分析是从数据中提取有价值的信息的过程。我们可以使用Pandas库进行数据分析,如计算统计量、分组聚合等。下面是一个使用Pandas库进行数据分析的例子:
import pandas as pd
data = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'Dave'],
'age': [30, 25, 35, 40],
'city': ['New York', 'Los Angeles', 'Chicago', 'New York']
})
计算年龄的平均值
mean_age = data['age'].mean()
print(f'平均年龄: {mean_age}')
按城市分组,计算每个城市的平均年龄
grouped_data = data.groupby('city')['age'].mean()
print(grouped_data)
2、数据可视化
数据可视化是将数据转换成图表的过程,以便更直观地展示数据。我们可以使用Matplotlib和Seaborn库进行数据可视化。下面是一个使用Matplotlib和Seaborn库进行数据可视化的例子:
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
data = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'Dave'],
'age': [30, 25, 35, 40],
'city': ['New York', 'Los Angeles', 'Chicago',
相关问答FAQs:
如何选择适合的Python库进行爬虫开发?
在进行Python爬虫开发时,选择合适的库至关重要。常用的库包括Requests和BeautifulSoup,前者用于发送HTTP请求和获取网页数据,后者则用于解析HTML和XML文档。此外,Scrapy是一个功能强大的框架,适合进行大规模的数据抓取。选择时需要考虑项目的复杂性、数据量及对性能的要求。
在爬虫开发过程中,如何处理反爬虫机制?
很多网站会实施反爬虫措施来保护其数据。为了应对这些机制,可以采取多种方法,比如设置请求头以模拟浏览器行为、使用代理IP以避免IP被封禁、以及通过控制请求频率来减少被识别的风险。此外,使用浏览器自动化工具如Selenium也可以有效绕过某些反爬虫技术。
如何存储爬取到的数据?
在爬取数据后,存储形式的选择非常重要。常见的存储方式包括将数据保存为CSV文件、JSON文件,或直接存入数据库如SQLite、MySQL和MongoDB等。选择存储方式时,需考虑数据的规模、后续的处理需求以及访问频率,以确保数据能够高效管理和使用。
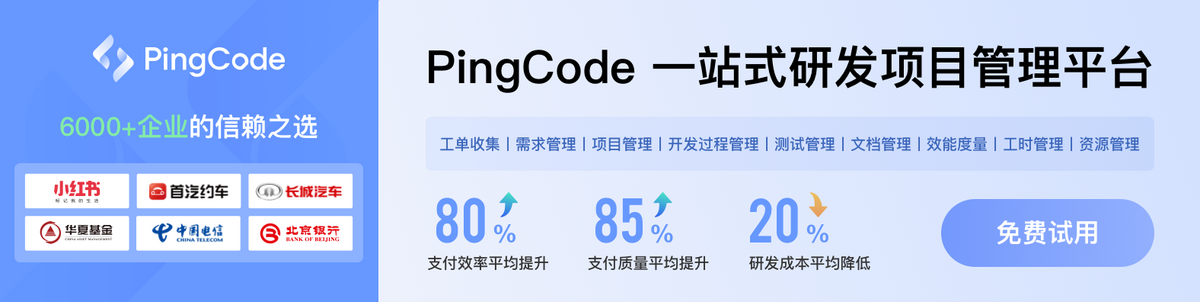