Python可以通过使用BeautifulSoup、Requests、Scrapy等爬虫库来实现自动爬取多页内容,关键在于找到页面的翻页逻辑、模拟请求获取数据、解析页面内容。例如,使用Requests库发送HTTP请求、BeautifulSoup解析HTML内容、Scrapy框架处理爬虫任务。其中,Scrapy框架提供了更为强大的功能,可以轻松处理复杂的多页爬取任务。接下来,我们将详细介绍如何使用这些工具实现自动爬取多页内容。
一、Requests和BeautifulSoup的基本使用
1、安装与基本操作
首先,安装Requests和BeautifulSoup库:
pip install requests
pip install beautifulsoup4
使用Requests库发送HTTP请求,获取网页内容:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
使用BeautifulSoup解析HTML内容:
soup = BeautifulSoup(html_content, 'html.parser')
2、获取分页链接
找到网页中用于翻页的链接,通常通过分析页面的HTML结构来找到翻页按钮的链接:
next_page_link = soup.find('a', {'class': 'next-page'})['href']
3、循环爬取多页内容
通过循环和条件判断,依次爬取每一页的内容:
while url:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 处理当前页内容
process_page(soup)
# 获取下一页的链接
next_page_tag = soup.find('a', {'class': 'next-page'})
if next_page_tag:
url = next_page_tag['href']
else:
url = None
二、使用Scrapy框架实现多页爬取
1、安装与基本操作
首先,安装Scrapy框架:
pip install scrapy
创建一个新的Scrapy项目:
scrapy startproject myproject
2、定义Item和Spider
在items.py
中定义要爬取的数据结构:
import scrapy
class MyprojectItem(scrapy.Item):
title = scrapy.Field()
link = scrapy.Field()
在spiders
目录下创建一个新的Spider:
import scrapy
from myproject.items import MyprojectItem
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['https://example.com']
def parse(self, response):
for article in response.css('article'):
item = MyprojectItem()
item['title'] = article.css('h2::text').get()
item['link'] = article.css('a::attr(href)').get()
yield item
next_page = response.css('a.next-page::attr(href)').get()
if next_page:
yield response.follow(next_page, self.parse)
3、运行爬虫
在项目根目录下运行爬虫:
scrapy crawl myspider
三、处理复杂的翻页逻辑
1、模拟表单提交
有些网站的翻页是通过表单提交实现的,可以使用Requests库模拟表单提交来实现翻页:
data = {
'page': 2,
'other_param': 'value'
}
response = requests.post(url, data=data)
2、处理AJAX请求
有些网站的翻页是通过AJAX请求实现的,可以使用Requests库发送AJAX请求获取数据:
headers = {
'X-Requested-With': 'XMLHttpRequest'
}
response = requests.get(url, headers=headers)
四、数据存储与处理
1、存储到CSV文件
使用Python内置的csv模块将数据存储到CSV文件中:
import csv
with open('data.csv', 'w', newline='') as csvfile:
fieldnames = ['title', 'link']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for item in items:
writer.writerow(item)
2、存储到数据库
使用SQLAlchemy库将数据存储到数据库中:
from sqlalchemy import create_engine, Column, String, Integer, Base
from sqlalchemy.orm import sessionmaker
engine = create_engine('sqlite:///data.db')
Base = declarative_base()
class Article(Base):
__tablename__ = 'articles'
id = Column(Integer, primary_key=True)
title = Column(String)
link = Column(String)
Base.metadata.create_all(engine)
Session = sessionmaker(bind=engine)
session = Session()
for item in items:
article = Article(title=item['title'], link=item['link'])
session.add(article)
session.commit()
五、处理动态加载页面
1、使用Selenium模拟浏览器操作
对于需要动态加载内容的页面,可以使用Selenium库来模拟浏览器操作:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
while True:
# 处理当前页内容
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
process_page(soup)
# 查找并点击下一页按钮
next_page_button = driver.find_element_by_class_name('next-page')
if next_page_button:
next_page_button.click()
else:
break
六、总结
通过使用Requests、BeautifulSoup、Scrapy和Selenium等工具,Python可以实现自动爬取多页内容。关键在于找到页面的翻页逻辑,模拟请求获取数据,并解析页面内容。对于复杂的翻页逻辑,可以通过模拟表单提交、发送AJAX请求、使用Selenium等方法来实现。最后,将爬取到的数据存储到CSV文件或数据库中,以便后续处理和分析。这些方法和工具使得Python在自动化爬取网页数据方面非常强大和灵活。
相关问答FAQs:
如何使用Python实现多页爬虫?
要实现多页爬虫,您可以使用requests
库获取网页内容,并结合BeautifulSoup
或lxml
解析网页。在处理多页时,需注意网站的分页结构,比如URL中可能包含页码参数。通过循环遍历页码,您可以顺利抓取每一页的数据。
在爬取多页时,如何避免被网站封禁?
为了避免被网站封禁,建议您在爬取时设置请求间隔,使用随机的时间间隔进行请求。此外,您可以使用代理IP来隐藏真实IP,并在请求头中添加一些常见的浏览器信息,模拟正常用户的访问行为。
我应该如何处理抓取到的数据?
抓取到的数据通常需要进行清洗和存储。您可以使用pandas
库将数据整理成表格格式,便于后续分析。同时,可以选择将数据保存到CSV文件、数据库或其他数据存储方案,以便后续使用和分析。
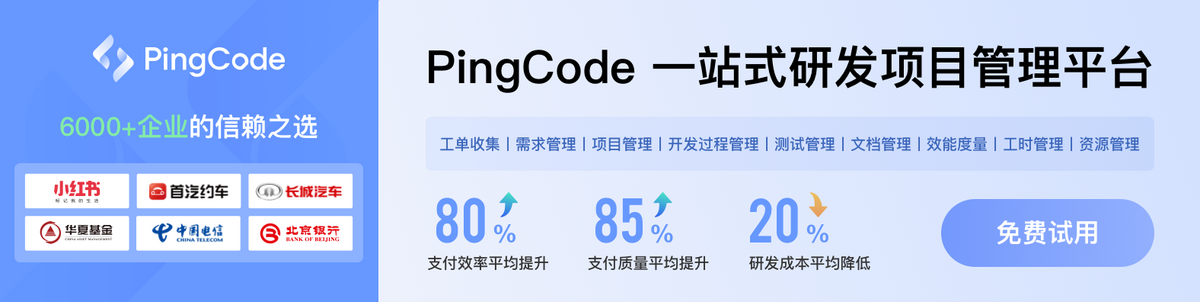