Python可以通过数据获取、数据清洗、数据分析、数据可视化等步骤来分析地产股。 其中,数据获取是基础,可以通过API或爬虫技术从金融网站获取股票数据;数据清洗是关键,包括处理缺失值、异常值等;数据分析可以使用Pandas等库进行统计分析,计算股票的收益率、波动率等指标;数据可视化则可以使用Matplotlib、Seaborn等库来展示分析结果。下面将详细描述数据获取的过程。
一、数据获取
获取地产股的数据是分析的第一步,通常可以通过以下几种方式:
1、使用金融数据API
金融数据API是获取股票数据的常见方式,例如Yahoo Finance、Alpha Vantage等提供了丰富的股票数据接口。使用这些API需要注册并获取API密钥,然后通过HTTP请求获取数据。例如,使用Alpha Vantage的Python库可以轻松获取股票数据:
import requests
api_key = 'your_api_key'
symbol = 'AAPL'
url = f'https://www.alphavantage.co/query?function=TIME_SERIES_DAILY&symbol={symbol}&apikey={api_key}'
response = requests.get(url)
data = response.json()
2、使用爬虫技术
如果需要获取自定义格式的数据或API不满足需求,可以使用爬虫技术从金融网站获取数据。常用的爬虫库有BeautifulSoup和Scrapy。以下是一个使用BeautifulSoup从Yahoo Finance获取股票数据的示例:
import requests
from bs4 import BeautifulSoup
url = 'https://finance.yahoo.com/quote/AAPL/history?p=AAPL'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
提取数据
table = soup.find('table', {'data-test': 'historical-prices'})
rows = table.find_all('tr')
for row in rows[1:]:
cols = row.find_all('td')
print([col.text for col in cols])
二、数据清洗
获取到的数据通常需要进行清洗,以确保数据的质量和一致性。数据清洗包括处理缺失值、异常值以及转换数据格式等。
1、处理缺失值
缺失值是数据分析中的常见问题,可以通过删除缺失值、填充缺失值等方法处理。例如,使用Pandas库可以方便地处理缺失值:
import pandas as pd
读取数据
df = pd.read_csv('stock_data.csv')
删除缺失值
df.dropna(inplace=True)
填充缺失值
df.fillna(method='ffill', inplace=True)
2、处理异常值
异常值是指偏离正常范围的数据点,可以通过统计分析方法检测和处理异常值。例如,使用Z-score方法检测异常值:
import numpy as np
计算Z-score
df['z_score'] = (df['Close'] - df['Close'].mean()) / df['Close'].std()
过滤异常值
df = df[np.abs(df['z_score']) < 3]
三、数据分析
数据分析是对清洗后的数据进行统计分析和建模,以揭示数据中的规律和趋势。
1、计算股票收益率
股票收益率是衡量股票表现的重要指标,可以通过计算每日收益率和累计收益率来分析股票的表现:
# 计算每日收益率
df['daily_return'] = df['Close'].pct_change()
计算累计收益率
df['cumulative_return'] = (1 + df['daily_return']).cumprod() - 1
2、计算波动率
波动率是衡量股票价格波动程度的指标,可以通过计算标准差来衡量波动率:
# 计算波动率
df['volatility'] = df['daily_return'].rolling(window=30).std() * np.sqrt(252)
四、数据可视化
数据可视化是展示数据分析结果的重要手段,可以通过绘制图表来直观展示股票的表现和趋势。
1、绘制股票价格走势
可以使用Matplotlib库绘制股票价格走势图:
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 6))
plt.plot(df['Date'], df['Close'], label='Close Price')
plt.title('Stock Price Trend')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
2、绘制收益率和波动率
可以分别绘制股票的收益率和波动率图表:
# 绘制收益率
plt.figure(figsize=(10, 6))
plt.plot(df['Date'], df['cumulative_return'], label='Cumulative Return')
plt.title('Cumulative Return')
plt.xlabel('Date')
plt.ylabel('Return')
plt.legend()
plt.show()
绘制波动率
plt.figure(figsize=(10, 6))
plt.plot(df['Date'], df['volatility'], label='Volatility')
plt.title('Volatility')
plt.xlabel('Date')
plt.ylabel('Volatility')
plt.legend()
plt.show()
五、数据建模与预测
数据建模与预测是高级数据分析的关键步骤,可以使用机器学习和统计建模方法对股票价格进行预测。
1、使用时间序列模型
时间序列模型是股票价格预测的常用方法,例如ARIMA模型:
from statsmodels.tsa.arima_model import ARIMA
拆分训练集和测试集
train, test = df['Close'][:int(0.8*len(df))], df['Close'][int(0.8*len(df)):]
训练ARIMA模型
model = ARIMA(train, order=(5, 1, 0))
model_fit = model.fit(disp=0)
预测
forecast, stderr, conf_int = model_fit.forecast(steps=len(test))
绘制预测结果
plt.figure(figsize=(10, 6))
plt.plot(test.index, test, label='Actual Price')
plt.plot(test.index, forecast, label='Forecasted Price')
plt.fill_between(test.index, conf_int[:, 0], conf_int[:, 1], color='k', alpha=0.1)
plt.title('Stock Price Prediction')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
2、使用机器学习模型
可以使用机器学习模型如随机森林、支持向量机等对股票价格进行预测:
from sklearn.ensemble import RandomForestRegressor
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
准备特征和目标
X = df[['Open', 'High', 'Low', 'Volume']]
y = df['Close']
拆分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
训练随机森林模型
model = RandomForestRegressor(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
预测
y_pred = model.predict(X_test)
计算误差
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
绘制预测结果
plt.figure(figsize=(10, 6))
plt.plot(y_test.index, y_test, label='Actual Price')
plt.plot(y_test.index, y_pred, label='Predicted Price')
plt.title('Stock Price Prediction')
plt.xlabel('Date')
plt.ylabel('Price')
plt.legend()
plt.show()
六、总结
通过以上步骤,可以使用Python分析地产股的数据,包括获取数据、清洗数据、分析数据、可视化数据以及进行数据建模与预测。每个步骤都可以使用不同的方法和工具,根据实际需求选择合适的方法。通过这种方式,可以全面分析地产股的表现和趋势,为投资决策提供科学依据。
相关问答FAQs:
1. 如何利用Python获取地产股的实时数据?
可以使用多种Python库来获取地产股的实时数据,比如pandas_datareader
和yfinance
。通过这些库,用户可以从Yahoo Finance、Google Finance等平台获取股票的历史和实时价格。只需安装相应库并编写简单的代码,即可实现数据的抓取和处理。
2. 在分析地产股时,Python能提供哪些数据可视化工具?
Python提供多种强大的数据可视化工具,如Matplotlib
、Seaborn
和Plotly
。这些库允许用户创建各种图表,如折线图、柱状图和热图,从而使地产股的趋势和波动更加直观。通过结合这些可视化工具,分析师可以轻松识别市场模式和潜在投资机会。
3. 如何使用Python进行地产股的基本面分析?
基本面分析涉及评估公司的财务健康状况。使用Python,可以通过pandas
库读取和处理财务报表数据,包括利润表、资产负债表和现金流量表。分析师可以计算各种财务比率,如市盈率(P/E)、市净率(P/B)和负债率等,以帮助判断地产股的投资价值。结合数据分析和可视化技术,可以更全面地理解公司的财务状况。
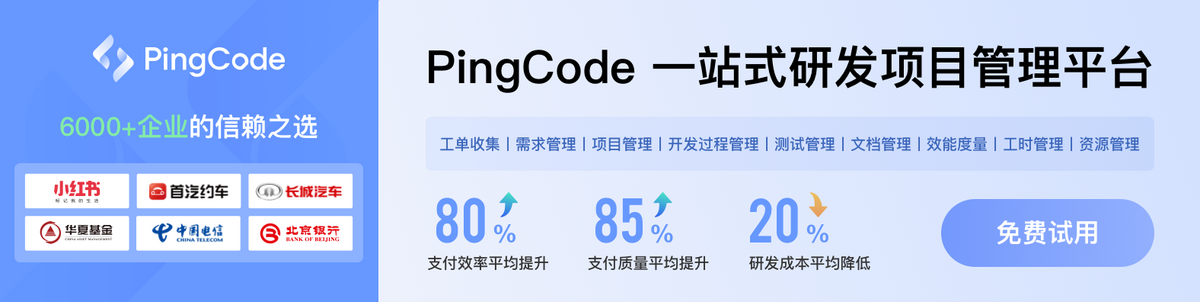