如何使用Python自动发邮件
使用Python自动发邮件需要使用smtplib库、MIME库、配置SMTP服务器。具体步骤包括:1. 导入所需库,2. 设置SMTP服务器及端口,3. 创建MIME邮件对象,4. 配置发件人、收件人、邮件主题及内容,5. 发送邮件。配置SMTP服务器是其中的关键步骤之一。接下来将详细介绍如何配置SMTP服务器以及完整的代码示例。
一、导入所需库
使用Python发送邮件需要导入smtplib
和email.mime
库。smtplib
库用于连接SMTP服务器并发送邮件,而email.mime
库用于创建邮件内容。
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
二、设置SMTP服务器及端口
不同的邮件服务提供商有不同的SMTP服务器地址和端口号。以下是常见的邮件服务提供商的SMTP服务器信息:
- Gmail: smtp.gmail.com, 端口号587
- Outlook: smtp-mail.outlook.com, 端口号587
- Yahoo: smtp.mail.yahoo.com, 端口号587
smtp_server = 'smtp.gmail.com'
smtp_port = 587
三、创建MIME邮件对象
创建一个MIME邮件对象,用于存储邮件内容及相关信息。
msg = MIMEMultipart()
msg['From'] = 'your_email@example.com'
msg['To'] = 'recipient_email@example.com'
msg['Subject'] = 'Your Subject Here'
四、配置发件人、收件人、邮件主题及内容
设置邮件的发件人、收件人、主题及正文内容。
body = 'This is the body of the email.'
msg.attach(MIMEText(body, 'plain'))
五、发送邮件
连接到SMTP服务器,使用发件人邮箱及密码进行登录,然后发送邮件。
try:
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用安全加密
server.login('your_email@example.com', 'your_password')
text = msg.as_string()
server.sendmail('your_email@example.com', 'recipient_email@example.com', text)
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
finally:
server.quit()
六、完整代码示例
以下是一个完整的Python自动发送邮件的代码示例:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
def send_email(subject, body, to_email):
smtp_server = 'smtp.gmail.com'
smtp_port = 587
from_email = 'your_email@example.com'
password = 'your_password'
# 创建MIME邮件对象
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
# 添加邮件正文
msg.attach(MIMEText(body, 'plain'))
try:
# 连接到SMTP服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用安全加密
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
finally:
server.quit()
调用函数发送邮件
send_email('Test Subject', 'This is the body of the test email.', 'recipient_email@example.com')
七、发送带附件的邮件
如果需要发送带附件的邮件,可以使用MIMEBase
类来添加附件。
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment(subject, body, to_email, file_path):
smtp_server = 'smtp.gmail.com'
smtp_port = 587
from_email = 'your_email@example.com'
password = 'your_password'
# 创建MIME邮件对象
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
# 添加邮件正文
msg.attach(MIMEText(body, 'plain'))
# 添加附件
filename = file_path.split('/')[-1]
attachment = open(file_path, 'rb')
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename= {filename}')
msg.attach(part)
try:
# 连接到SMTP服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用安全加密
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
finally:
server.quit()
调用函数发送带附件的邮件
send_email_with_attachment('Test Subject', 'This is the body of the test email.', 'recipient_email@example.com', 'path/to/your/file.txt')
八、发送HTML格式的邮件
发送HTML格式的邮件可以使用MIMEText
类,并将subtype
参数设置为html
。
def send_html_email(subject, html_content, to_email):
smtp_server = 'smtp.gmail.com'
smtp_port = 587
from_email = 'your_email@example.com'
password = 'your_password'
# 创建MIME邮件对象
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
# 添加HTML内容
msg.attach(MIMEText(html_content, 'html'))
try:
# 连接到SMTP服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用安全加密
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
print("HTML Email sent successfully!")
except Exception as e:
print(f"Failed to send HTML email: {e}")
finally:
server.quit()
调用函数发送HTML格式的邮件
html_content = """
<html>
<body>
<h1>This is an HTML Email</h1>
<p>This email contains <b>HTML</b> content.</p>
</body>
</html>
"""
send_html_email('HTML Email Subject', html_content, 'recipient_email@example.com')
九、使用SSL发送邮件
某些SMTP服务器可能要求使用SSL连接。可以使用SMTP_SSL
类来实现这一点。
def send_email_with_ssl(subject, body, to_email):
smtp_server = 'smtp.gmail.com'
smtp_port = 465 # SSL端口通常是465
from_email = 'your_email@example.com'
password = 'your_password'
# 创建MIME邮件对象
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
# 添加邮件正文
msg.attach(MIMEText(body, 'plain'))
try:
# 连接到SMTP服务器
server = smtplib.SMTP_SSL(smtp_server, smtp_port)
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
print("Email sent successfully with SSL!")
except Exception as e:
print(f"Failed to send email with SSL: {e}")
finally:
server.quit()
调用函数发送邮件
send_email_with_ssl('Test Subject', 'This is the body of the test email.', 'recipient_email@example.com')
十、处理发件人和收件人列表
在实际应用中,通常需要发送邮件给多个收件人,或者从多个发件人地址中选择一个。可以使用列表来处理这些情况。
def send_email_to_multiple_recipients(subject, body, to_emails):
smtp_server = 'smtp.gmail.com'
smtp_port = 587
from_email = 'your_email@example.com'
password = 'your_password'
# 创建MIME邮件对象
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = ', '.join(to_emails)
msg['Subject'] = subject
# 添加邮件正文
msg.attach(MIMEText(body, 'plain'))
try:
# 连接到SMTP服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用安全加密
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_emails, text)
print("Email sent successfully to multiple recipients!")
except Exception as e:
print(f"Failed to send email to multiple recipients: {e}")
finally:
server.quit()
调用函数发送邮件给多个收件人
send_email_to_multiple_recipients('Test Subject', 'This is the body of the test email.', ['recipient1@example.com', 'recipient2@example.com'])
十一、错误处理和日志记录
在实际应用中,为了提高代码的鲁棒性,通常需要添加错误处理和日志记录。
import logging
logging.basicConfig(level=logging.INFO, filename='email_log.log', filemode='a', format='%(asctime)s - %(levelname)s - %(message)s')
def send_email_with_logging(subject, body, to_email):
smtp_server = 'smtp.gmail.com'
smtp_port = 587
from_email = 'your_email@example.com'
password = 'your_password'
# 创建MIME邮件对象
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
# 添加邮件正文
msg.attach(MIMEText(body, 'plain'))
try:
# 连接到SMTP服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用安全加密
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
logging.info(f"Email sent successfully to {to_email}")
except Exception as e:
logging.error(f"Failed to send email to {to_email}: {e}")
finally:
server.quit()
调用函数发送邮件并记录日志
send_email_with_logging('Test Subject', 'This is the body of the test email.', 'recipient_email@example.com')
通过以上步骤,您可以使用Python自动发送邮件,无论是普通文本邮件、带附件的邮件、HTML格式的邮件、还是需要使用SSL发送的邮件。此外,处理多个收件人和发件人列表、添加错误处理和日志记录等进一步增强了代码的实用性和鲁棒性。希望这些内容能帮助您更好地理解和应用Python进行邮件自动化。
相关问答FAQs:
如何在Python中设置邮件服务器?
在Python中发送邮件通常需要设置一个邮件服务器。大部分情况下,您可以使用SMTP(简单邮件传输协议)来发送邮件。您可以使用内置的smtplib
库连接到邮件服务器,例如Gmail、Outlook等。确保您拥有正确的SMTP服务器地址、端口号以及用于身份验证的用户名和密码。
使用Python发送邮件时是否需要处理附件?
是的,您可以使用Python发送带附件的邮件。通过email
库中的MIME
模块,可以轻松地构建包含附件的电子邮件。您需要创建一个MIME
对象,将附件读入并附加到邮件正文中,从而确保接收者能够下载并查看文件。
如何确保邮件不会被标记为垃圾邮件?
为了减少邮件被标记为垃圾邮件的风险,建议您采取一些预防措施。首先,使用可靠的邮件服务器,并确保您的发件人地址看起来真实可信。使用明确的主题和内容,避免使用过于夸张的词汇,并确保提供合法的退订选项。此外,保持良好的邮件发送频率,避免一次性发送大量邮件。
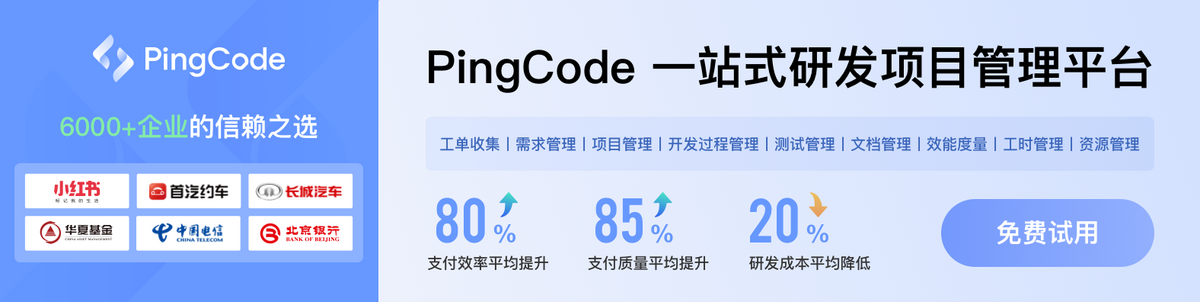