在Python中编写文档注释是编写高质量代码的重要部分。Python的文档注释通常使用docstring(文档字符串)来编写,这些字符串可以为模块、类、方法和函数提供说明。docstring的主要目的是提高代码的可读性和可维护性。以下是一些写文档注释的关键点:
-
使用三重引号:
在Python中,docstring使用三个引号(单引号或双引号)来包围注释内容。推荐使用双引号。
def function_name(parameters):
"""This is a docstring example.
Args:
parameters (type): Description of the parameters.
Returns:
return_type: Description of the return value.
"""
pass
-
首行简要描述:
每个docstring的第一行应该是简要描述函数、方法或类的功能。这一行应该尽量简洁,通常不超过一行。
-
详细描述:
如果需要更详细的说明,可以在首行之后添加一个空行,然后继续写详细说明。这可以包括函数的功能、参数说明、返回值、抛出的异常等信息。
-
遵循PEP 257标准:
PEP 257是Python的文档字符串约定,建议遵循这些约定来确保文档注释的一致性和可读性。
接下来,我们将详细介绍如何编写文档注释,并提供一些示例来展示最佳实践。
一、函数的文档注释
编写函数的文档注释需要包含函数的目的、参数和返回值等信息。以下是一个示例:
def add(a, b):
"""
Add two numbers and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
在这个示例中,docstring的第一行简要说明了函数的目的,即“Add two numbers and return the result”。接下来的部分详细描述了参数(a
和b
)以及返回值的类型和意义。
二、类的文档注释
类的文档注释应该描述类的用途和使用方法。以下是一个示例:
class Calculator:
"""
A simple calculator class to perform basic arithmetic operations.
Methods:
add(a, b): Adds two numbers.
subtract(a, b): Subtracts the second number from the first.
multiply(a, b): Multiplies two numbers.
divide(a, b): Divides the first number by the second.
"""
def add(self, a, b):
"""
Add two numbers and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
def subtract(self, a, b):
"""
Subtract the second number from the first and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The result of the subtraction.
"""
return a - b
def multiply(self, a, b):
"""
Multiply two numbers and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The product of the two numbers.
"""
return a * b
def divide(self, a, b):
"""
Divide the first number by the second and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
float: The quotient of the division.
Raises:
ValueError: If the second number (b) is zero.
"""
if b == 0:
raise ValueError("Cannot divide by zero.")
return a / b
在这个示例中,类Calculator
的docstring简要描述了类的用途,并列出了类中定义的方法。每个方法都有自己的docstring,详细说明了参数、返回值和可能抛出的异常。
三、模块的文档注释
模块的文档注释通常放在模块文件的顶部,用于说明模块的用途和功能。以下是一个示例:
"""
calculator.py
This module provides a simple calculator class to perform basic arithmetic operations.
Classes:
Calculator: A class for performing arithmetic operations.
"""
class Calculator:
"""
A simple calculator class to perform basic arithmetic operations.
Methods:
add(a, b): Adds two numbers.
subtract(a, b): Subtracts the second number from the first.
multiply(a, b): Multiplies two numbers.
divide(a, b): Divides the first number by the second.
"""
def add(self, a, b):
"""
Add two numbers and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
def subtract(self, a, b):
"""
Subtract the second number from the first and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The result of the subtraction.
"""
return a - b
def multiply(self, a, b):
"""
Multiply two numbers and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The product of the two numbers.
"""
return a * b
def divide(self, a, b):
"""
Divide the first number by the second and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
float: The quotient of the division.
Raises:
ValueError: If the second number (b) is zero.
"""
if b == 0:
raise ValueError("Cannot divide by zero.")
return a / b
在这个示例中,模块calculator.py
的docstring位于文件顶部,简要描述了模块的用途和功能。类Calculator
和其方法的docstring则提供了更详细的信息。
四、特殊格式的文档注释
在某些情况下,您可能需要使用特定格式的文档注释,以便与自动化文档生成工具(如Sphinx)兼容。这些工具可以从docstring中提取信息并生成HTML或PDF格式的文档。
以下是使用reStructuredText格式编写的文档注释示例:
def add(a, b):
"""
Add two numbers and return the result.
:param a: The first number.
:type a: int
:param b: The second number.
:type b: int
:return: The sum of the two numbers.
:rtype: int
"""
return a + b
在这个示例中,使用了reStructuredText格式的标签(如:param
和:return
)来描述参数和返回值的类型和意义。这种格式可以被Sphinx等工具识别并用于生成文档。
五、文档注释的最佳实践
编写高质量的文档注释需要遵循一些最佳实践,以确保注释的清晰和一致。以下是一些建议:
-
保持简洁:
docstring的首行应该尽量简短,描述函数、类或模块的主要功能。详细说明可以放在后面。
-
使用一致的格式:
选择一种格式(如Google风格或reStructuredText风格)并在整个项目中保持一致。这有助于提高文档的可读性和可维护性。
-
描述参数和返回值:
对每个参数和返回值进行详细描述,包括它们的类型和意义。如果函数可能抛出异常,也应在docstring中说明。
-
定期更新:
随着代码的变化,确保及时更新文档注释,以保持其准确性和相关性。
-
使用自动化工具:
利用自动化工具(如Sphinx)生成文档,并定期检查生成的文档,确保其内容准确和完整。
六、综合示例
以下是一个综合示例,展示了如何为模块、类和函数编写高质量的文档注释:
"""
math_operations.py
This module provides classes and functions for performing various mathematical operations.
Classes:
Calculator: A class for performing basic arithmetic operations.
AdvancedCalculator: A class for performing advanced mathematical operations.
Functions:
factorial(n): Calculate the factorial of a number.
"""
class Calculator:
"""
A simple calculator class to perform basic arithmetic operations.
Methods:
add(a, b): Adds two numbers.
subtract(a, b): Subtracts the second number from the first.
multiply(a, b): Multiplies two numbers.
divide(a, b): Divides the first number by the second.
"""
def add(self, a, b):
"""
Add two numbers and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of the two numbers.
"""
return a + b
def subtract(self, a, b):
"""
Subtract the second number from the first and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The result of the subtraction.
"""
return a - b
def multiply(self, a, b):
"""
Multiply two numbers and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The product of the two numbers.
"""
return a * b
def divide(self, a, b):
"""
Divide the first number by the second and return the result.
Args:
a (int): The first number.
b (int): The second number.
Returns:
float: The quotient of the division.
Raises:
ValueError: If the second number (b) is zero.
"""
if b == 0:
raise ValueError("Cannot divide by zero.")
return a / b
class AdvancedCalculator:
"""
A calculator class to perform advanced mathematical operations.
Methods:
power(base, exponent): Calculate the power of a number.
square_root(x): Calculate the square root of a number.
"""
def power(self, base, exponent):
"""
Calculate the power of a number.
Args:
base (float): The base number.
exponent (float): The exponent.
Returns:
float: The result of base raised to the power of exponent.
"""
return base exponent
def square_root(self, x):
"""
Calculate the square root of a number.
Args:
x (float): The number to calculate the square root of.
Returns:
float: The square root of the number.
Raises:
ValueError: If x is negative.
"""
if x < 0:
raise ValueError("Cannot calculate the square root of a negative number.")
return x 0.5
def factorial(n):
"""
Calculate the factorial of a number.
Args:
n (int): The number to calculate the factorial of.
Returns:
int: The factorial of the number.
Raises:
ValueError: If n is negative.
"""
if n < 0:
raise ValueError("Cannot calculate the factorial of a negative number.")
if n == 0:
return 1
result = 1
for i in range(1, n + 1):
result *= i
return result
在这个综合示例中,我们为模块math_operations.py
、类Calculator
和AdvancedCalculator
以及函数factorial
编写了详细的文档注释。每个docstring都遵循一致的格式,描述了参数、返回值和可能抛出的异常。
通过遵循这些最佳实践和示例,您可以编写出清晰、详细且一致的文档注释,从而提高代码的可读性和可维护性。记住,好的文档注释不仅对其他开发人员有帮助,也有助于您自己在未来回顾代码时快速理解其功能和用途。
相关问答FAQs:
文档注释在Python中的重要性是什么?
文档注释是Python代码中的重要组成部分,它提供了对代码功能的清晰描述,帮助开发者理解代码的目的和用法。使用文档注释,可以使代码更加易于维护和共享。文档注释通常用于模块、类和函数的开头,能够自动生成文档,提升代码的可读性和可用性。
如何在Python中撰写有效的文档注释?
撰写有效的文档注释时,建议遵循一些基本原则:明确简洁地描述函数的作用、参数及其类型、返回值及其类型,必要时提供示例。可以使用标准的文档字符串格式,比如Google风格或reStructuredText风格,确保注释的一致性和清晰度。这将帮助其他开发者快速理解代码的功能。
有哪些工具可以帮助生成Python文档注释?
有多种工具可用于生成和管理Python文档注释。例如,Sphinx是一个流行的文档生成工具,它可以从代码中的文档注释自动生成HTML或PDF文档。此外,PyDoc是Python自带的一个模块,可以用于查看模块的文档字符串。使用这些工具,可以大大提高文档的生成效率和专业性。
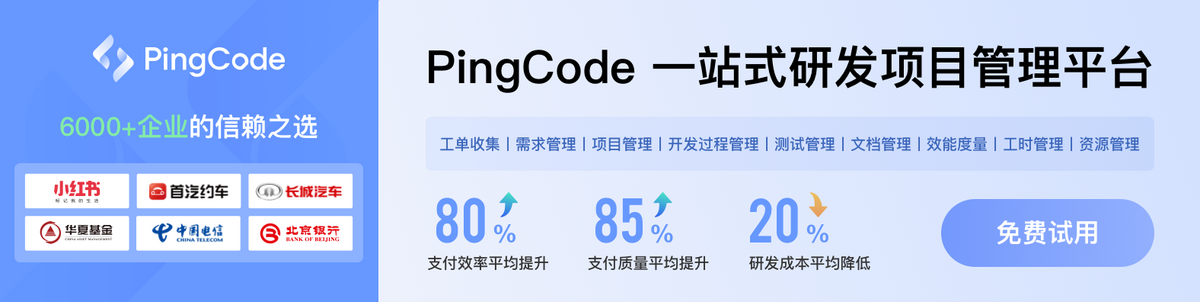