Python抓包解析天气的方法主要有:使用requests库发送HTTP请求、使用BeautifulSoup库解析HTML、使用第三方API、使用Selenium库模拟浏览器抓取数据。其中,使用requests库发送HTTP请求是比较常见且简单的方法。通过requests库,我们可以发送GET请求到天气网站的API接口,获取天气数据的JSON响应,然后解析这些数据。下面将详细讲解如何使用requests库抓包解析天气数据。
一、使用requests库发送HTTP请求
-
安装requests库
首先,我们需要安装requests库。如果你还没有安装,可以使用以下命令进行安装:
pip install requests
-
发送HTTP请求
使用requests库发送GET请求获取天气数据。以OpenWeatherMap的API为例:
import requests
api_key = 'your_api_key'
city = 'London'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
data = response.json()
print(data)
-
解析JSON响应
获取到的响应是一个JSON格式的数据,可以使用Python内置的json库进行解析:
import json
weather_data = json.loads(response.text)
print(weather_data)
二、使用BeautifulSoup解析HTML
有时候我们需要解析HTML页面来获取天气数据,这时可以使用BeautifulSoup库。以下是具体步骤:
-
安装BeautifulSoup库
pip install beautifulsoup4
-
发送HTTP请求并获取HTML内容
import requests
from bs4 import BeautifulSoup
url = 'http://example-weather-website.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
-
解析HTML并提取数据
weather = soup.find('div', class_='weather-info').text
print(weather)
三、使用第三方API
使用第三方API是获取天气数据的最简单方法之一。这些API通常会提供详细的文档,说明如何获取和解析数据。以下是具体步骤:
-
注册并获取API密钥
以OpenWeatherMap为例,首先需要注册一个账户并获取API密钥。
-
使用API获取数据
import requests
api_key = 'your_api_key'
city = 'London'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
data = response.json()
print(data)
-
解析数据
weather_data = response.json()
temperature = weather_data['main']['temp']
description = weather_data['weather'][0]['description']
print(f'Temperature: {temperature}')
print(f'Weather: {description}')
四、使用Selenium模拟浏览器抓取数据
有些网站的天气数据需要通过动态加载才能获取到,此时可以使用Selenium库来模拟浏览器抓取数据。
-
安装Selenium库
pip install selenium
-
安装浏览器驱动
例如,使用Chrome浏览器,需要下载ChromeDriver并将其路径添加到系统PATH中。
-
使用Selenium抓取数据
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example-weather-website.com')
weather_element = driver.find_element_by_class_name('weather-info')
weather = weather_element.text
print(weather)
driver.quit()
五、综合示例
下面是一个综合示例,展示如何使用requests库和BeautifulSoup库从一个实际的天气网站抓取并解析天气数据:
import requests
from bs4 import BeautifulSoup
def get_weather(city):
url = f'https://www.weather-website.com/cities/{city}'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
weather = soup.find('div', class_='weather-info').text
temperature = soup.find('span', class_='temp').text
description = soup.find('span', class_='desc').text
return {
'city': city,
'weather': weather,
'temperature': temperature,
'description': description
}
city = 'London'
weather_data = get_weather(city)
print(weather_data)
六、处理异常情况
在实际应用中,处理异常情况是非常重要的。我们需要考虑网络连接问题、API返回错误等情况。以下是一些处理异常的示例:
-
处理网络连接问题
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
except requests.exceptions.RequestException as e:
print(f'Error fetching data: {e}')
-
处理API返回错误
if response.status_code == 200:
data = response.json()
else:
print(f'Error: {response.status_code}')
-
处理JSON解析错误
try:
data = response.json()
except json.JSONDecodeError as e:
print(f'Error parsing JSON: {e}')
七、定时抓取天气数据
在实际应用中,我们可能需要定时抓取天气数据,以便实时更新天气信息。可以使用Python的调度库,比如schedule或APScheduler来实现定时任务。
-
安装schedule库
pip install schedule
-
编写定时任务
import schedule
import time
def job():
weather_data = get_weather('London')
print(weather_data)
schedule.every().hour.do(job)
while True:
schedule.run_pending()
time.sleep(1)
八、总结
通过以上方法,我们可以使用Python抓包解析天气数据。无论是使用requests库发送HTTP请求、使用BeautifulSoup解析HTML、使用第三方API,还是使用Selenium模拟浏览器抓取数据,都各有优缺点。根据具体需求选择合适的方法,可以有效地获取并解析天气数据。
掌握了这些方法后,你可以更灵活地处理各种天气数据抓取和解析的任务,为你的项目提供实时准确的天气信息。希望这篇文章能帮助你更好地理解和应用Python抓包解析天气数据的方法。
相关问答FAQs:
如何使用Python抓取天气数据的API?
抓取天气数据的一个有效方法是使用天气API,如OpenWeatherMap或Weather API。用户可以申请API密钥,然后通过Python的requests库发送GET请求,以获取JSON格式的天气数据。解析JSON数据后,可以提取所需的天气信息,如温度、湿度和风速等。确保在抓取之前阅读API文档,以了解请求格式和数据结构。
在抓取天气数据时需要注意哪些法律和伦理问题?
在抓取天气数据时,用户应确保遵循API提供商的使用条款。有些服务可能限制请求频率或要求在使用其数据时给予适当的归属。此外,避免使用爬虫技术直接从网页抓取数据,因为这可能违反服务条款,并导致法律问题。
如果抓包过程中遇到错误,应该如何调试?
在抓包过程中,如果遇到错误,可以通过查看响应状态码和返回的错误信息来进行调试。常见的HTTP状态码如404(未找到)或401(未授权)都可以指示问题所在。用户还可以通过使用Python的logging模块记录请求和响应,以便更好地分析问题。同时,检查网络连接和API密钥的有效性也是解决问题的关键步骤。
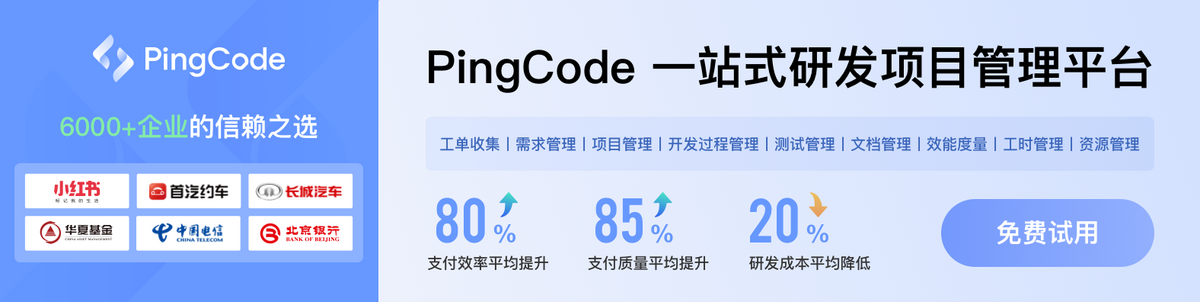