在Python中,改变方向可以通过多种方式实现,主要包括使用条件语句、使用循环语句、使用函数、面向对象编程等。这些方法可以帮助我们根据不同的需求和情境来改变代码的执行方向。其中,使用条件语句是最常见和基础的方法,通过if-else结构可以根据条件的不同来决定执行不同的代码块。
使用条件语句
条件语句是编程中最基础的控制结构之一,常见的有if
、elif
和else
。通过这些语句,可以根据条件的真假来控制程序的执行路径。
direction = "north"
if direction == "north":
print("You are heading north.")
elif direction == "south":
print("You are heading south.")
elif direction == "east":
print("You are heading east.")
elif direction == "west":
print("You are heading west.")
else:
print("Unknown direction.")
在上述代码中,程序根据变量direction
的值来决定打印不同的信息。
使用循环语句
循环语句也是一种重要的控制结构,可以重复执行某段代码,并根据条件改变方向。常见的循环语句有for
循环和while
循环。
directions = ["north", "east", "south", "west"]
for direction in directions:
if direction == "north":
print("You are heading north.")
elif direction == "south":
print("You are heading south.")
elif direction == "east":
print("You are heading east.")
elif direction == "west":
print("You are heading west.")
在这个例子中,程序会依次检查每一个方向,并根据条件输出相应的信息。
使用函数
通过定义函数,可以更好地组织代码,并根据需要调用不同的函数来改变执行方向。
def change_direction(direction):
if direction == "north":
return "You are heading north."
elif direction == "south":
return "You are heading south."
elif direction == "east":
return "You are heading east."
elif direction == "west":
return "You are heading west."
else:
return "Unknown direction."
print(change_direction("north"))
print(change_direction("west"))
在这个例子中,通过调用change_direction
函数,可以根据传入的参数来决定返回不同的字符串。
面向对象编程
通过面向对象编程,可以创建类和对象来更好地管理和改变方向。
class Navigator:
def __init__(self, direction):
self.direction = direction
def change_direction(self, new_direction):
self.direction = new_direction
def get_direction(self):
if self.direction == "north":
return "You are heading north."
elif self.direction == "south":
return "You are heading south."
elif self.direction == "east":
return "You are heading east."
elif self.direction == "west":
return "You are heading west."
else:
return "Unknown direction."
navigator = Navigator("north")
print(navigator.get_direction())
navigator.change_direction("west")
print(navigator.get_direction())
在这个例子中,通过创建Navigator
类,可以更灵活地管理方向的改变和获取。
其他方法
除了上述常见的方法外,还有一些其他的方法和技巧可以用来改变方向,如使用字典、使用状态机等。
directions_dict = {
"north": "You are heading north.",
"south": "You are heading south.",
"east": "You are heading east.",
"west": "You are heading west."
}
direction = "north"
print(directions_dict.get(direction, "Unknown direction."))
通过使用字典,可以更简洁地管理条件和对应的输出。
总结
在Python中,改变方向的方法多种多样,可以根据具体的需求选择合适的方法。使用条件语句、使用循环语句、使用函数、面向对象编程等都是常见且有效的方式。掌握这些方法,可以让你的代码更具灵活性和可读性。
相关问答FAQs:
如何在Python中控制对象的移动方向?
在Python中,可以通过修改对象的坐标或角度来控制其移动方向。通常,这涉及到对对象的x和y坐标进行加减操作,或者使用三角函数(如sin和cos)来计算新的位置。例如,如果你在一个游戏中想要让角色向右移动,可以增加其x坐标的值;如果想要让角色向上移动,则增加其y坐标的值。
在Python中是否有库可以方便地实现方向改变?
是的,Python有许多库可以帮助实现方向控制,例如Pygame和Turtle。Pygame提供了丰富的功能来处理游戏中的图形和运动,而Turtle则适合于教育和简单的图形绘制。这些库通常提供了简单的函数来改变方向和位置,使得开发过程更加高效。
如何在Python中实现方向的平滑过渡?
要实现方向的平滑过渡,可以使用插值算法或逐帧更新位置。例如,可以在每一帧中计算对象当前位置与目标位置之间的差值,并逐步调整位置,从而实现平滑移动。此外,使用动画库如Matplotlib或Pygame的时间控制功能,可以使方向变化更加自然。
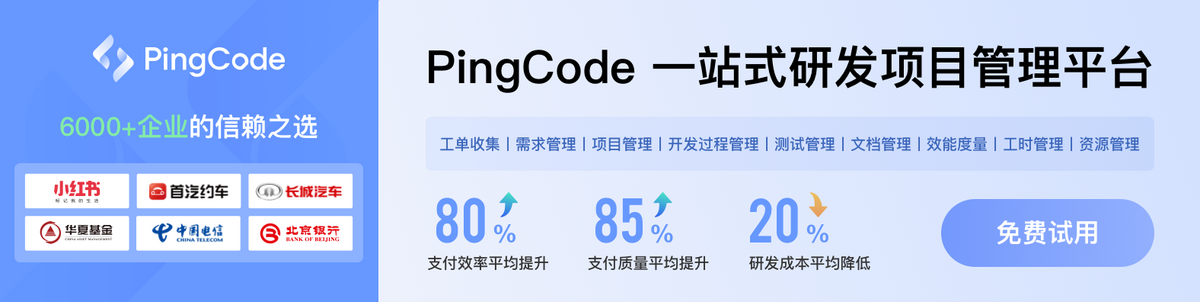