要在命令行中压缩文件或目录,可以使用Python的内置库 zipfile
和 shutil
。通过这两种方式,你可以轻松地在命令行中进行压缩操作。 下面将详细介绍如何使用这些方法,其中 zipfile
是用于创建和读取 .zip
文件,而 shutil
是用于创建和管理归档文件。我们将详细展开如何使用 zipfile
来压缩文件。
使用 zipfile
压缩文件
zipfile
是 Python 标准库中的一个模块,用于创建、读取、写入、添加和列出 ZIP 文件的内容。以下是使用 zipfile
压缩文件和目录的详细步骤:
1. 导入所需模块
首先,需要导入 zipfile
模块和其他一些辅助模块:
import zipfile
import os
2. 定义压缩函数
我们需要定义一个函数来压缩文件或目录。这个函数将接受文件或目录的路径和目标 ZIP 文件的路径:
def zip_files(source, destination):
with zipfile.ZipFile(destination, 'w') as zipf:
if os.path.isdir(source):
for root, dirs, files in os.walk(source):
for file in files:
zipf.write(os.path.join(root, file),
os.path.relpath(os.path.join(root, file),
os.path.join(source, '..')))
else:
zipf.write(source, os.path.basename(source))
3. 调用压缩函数
通过调用 zip_files
函数,可以将文件或目录压缩到指定的 ZIP 文件中:
source_path = 'path/to/source'
destination_path = 'path/to/destination.zip'
zip_files(source_path, destination_path)
使用 shutil
压缩文件
shutil
模块提供了更高层次的文件操作,包括复制、移动、删除和压缩等。使用 shutil
模块进行压缩的步骤如下:
1. 导入所需模块
同样,首先需要导入 shutil
模块:
import shutil
2. 定义压缩函数
可以定义一个函数来调用 shutil.make_archive
,它将源目录压缩为指定格式的归档文件:
def compress_with_shutil(source, destination, archive_format='zip'):
shutil.make_archive(destination, archive_format, source)
3. 调用压缩函数
通过调用 compress_with_shutil
函数,可以将目录压缩为指定格式的归档文件:
source_path = 'path/to/source'
destination_path = 'path/to/destination'
compress_with_shutil(source_path, destination_path)
一、压缩命令行参数解析
为了使压缩脚本更具通用性,可以使用 argparse
模块来解析命令行参数。这样,用户可以在命令行中指定源路径和目标路径。
1. 导入 argparse
模块
首先,需要导入 argparse
模块:
import argparse
2. 定义命令行参数解析器
通过定义命令行参数解析器,可以解析用户输入的参数:
def parse_args():
parser = argparse.ArgumentParser(description='Compress files and directories.')
parser.add_argument('source', help='Source file or directory to compress')
parser.add_argument('destination', help='Destination archive file path')
parser.add_argument('--method', choices=['zipfile', 'shutil'], default='zipfile',
help='Method to use for compression')
return parser.parse_args()
3. 使用解析器解析参数并调用压缩函数
通过调用解析器解析命令行参数,并根据选择的压缩方法调用相应的压缩函数:
if __name__ == '__main__':
args = parse_args()
if args.method == 'zipfile':
zip_files(args.source, args.destination)
elif args.method == 'shutil':
compress_with_shutil(args.source, args.destination)
二、处理错误和异常
在实际使用过程中,可能会遇到各种错误和异常情况,例如文件不存在、权限不足等。为了使脚本更加健壮,可以添加错误和异常处理。
1. 添加异常处理
在压缩函数中添加异常处理,以捕获和处理可能的异常:
def zip_files(source, destination):
try:
with zipfile.ZipFile(destination, 'w') as zipf:
if os.path.isdir(source):
for root, dirs, files in os.walk(source):
for file in files:
zipf.write(os.path.join(root, file),
os.path.relpath(os.path.join(root, file),
os.path.join(source, '..')))
else:
zipf.write(source, os.path.basename(source))
except Exception as e:
print(f'Error compressing {source}: {e}')
2. 使用异常处理调用压缩函数
在 __main__
部分中调用压缩函数时,也可以添加异常处理:
if __name__ == '__main__':
args = parse_args()
try:
if args.method == 'zipfile':
zip_files(args.source, args.destination)
elif args.method == 'shutil':
compress_with_shutil(args.source, args.destination)
except Exception as e:
print(f'Error: {e}')
三、支持更多压缩格式
除了 ZIP 格式,还可以支持其他压缩格式,例如 tar
, gztar
, bztar
等。通过修改 compress_with_shutil
函数,可以支持更多的压缩格式。
1. 修改 compress_with_shutil
函数
在 compress_with_shutil
函数中添加对更多压缩格式的支持:
def compress_with_shutil(source, destination, archive_format='zip'):
try:
shutil.make_archive(destination, archive_format, source)
except Exception as e:
print(f'Error compressing {source}: {e}')
2. 修改命令行参数解析器
在命令行参数解析器中添加对更多压缩格式的支持:
def parse_args():
parser = argparse.ArgumentParser(description='Compress files and directories.')
parser.add_argument('source', help='Source file or directory to compress')
parser.add_argument('destination', help='Destination archive file path')
parser.add_argument('--method', choices=['zipfile', 'shutil'], default='zipfile',
help='Method to use for compression')
parser.add_argument('--format', choices=['zip', 'tar', 'gztar', 'bztar'], default='zip',
help='Archive format to use with shutil')
return parser.parse_args()
3. 使用解析器解析参数并调用压缩函数
在 __main__
部分中调用压缩函数时,传递用户选择的压缩格式:
if __name__ == '__main__':
args = parse_args()
try:
if args.method == 'zipfile':
zip_files(args.source, args.destination)
elif args.method == 'shutil':
compress_with_shutil(args.source, args.destination, args.format)
except Exception as e:
print(f'Error: {e}')
四、提高压缩效率
在压缩大文件或目录时,压缩效率可能会成为一个瓶颈。可以通过调整压缩级别和使用多线程等方式提高压缩效率。
1. 调整压缩级别
在使用 zipfile
模块时,可以通过调整压缩级别提高压缩效率:
def zip_files(source, destination, compression=zipfile.ZIP_DEFLATED):
try:
with zipfile.ZipFile(destination, 'w', compression=compression) as zipf:
if os.path.isdir(source):
for root, dirs, files in os.walk(source):
for file in files:
zipf.write(os.path.join(root, file),
os.path.relpath(os.path.join(root, file),
os.path.join(source, '..')))
else:
zipf.write(source, os.path.basename(source))
except Exception as e:
print(f'Error compressing {source}: {e}')
2. 使用多线程进行压缩
为了提高压缩效率,还可以使用多线程进行并行压缩。可以使用 concurrent.futures
模块来实现多线程压缩:
import concurrent.futures
def zip_files_concurrently(source, destination):
try:
with zipfile.ZipFile(destination, 'w') as zipf:
if os.path.isdir(source):
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = []
for root, dirs, files in os.walk(source):
for file in files:
futures.append(executor.submit(zipf.write, os.path.join(root, file),
os.path.relpath(os.path.join(root, file),
os.path.join(source, '..'))))
for future in concurrent.futures.as_completed(futures):
future.result()
else:
zipf.write(source, os.path.basename(source))
except Exception as e:
print(f'Error compressing {source}: {e}')
3. 调用多线程压缩函数
在 __main__
部分中调用多线程压缩函数:
if __name__ == '__main__':
args = parse_args()
try:
if args.method == 'zipfile':
zip_files_concurrently(args.source, args.destination)
elif args.method == 'shutil':
compress_with_shutil(args.source, args.destination, args.format)
except Exception as e:
print(f'Error: {e}')
五、生成压缩报告
在压缩过程中,生成压缩报告可以帮助用户了解压缩的进展和结果。可以通过记录压缩的文件和压缩率来生成压缩报告。
1. 修改压缩函数生成报告
在压缩函数中记录压缩的文件和压缩率,并生成压缩报告:
def zip_files_with_report(source, destination, compression=zipfile.ZIP_DEFLATED):
try:
with zipfile.ZipFile(destination, 'w', compression=compression) as zipf:
report = []
if os.path.isdir(source):
for root, dirs, files in os.walk(source):
for file in files:
file_path = os.path.join(root, file)
zipf.write(file_path, os.path.relpath(file_path, os.path.join(source, '..')))
report.append(file_path)
else:
zipf.write(source, os.path.basename(source))
report.append(source)
generate_report(report, destination)
except Exception as e:
print(f'Error compressing {source}: {e}')
2. 定义生成报告的函数
定义一个函数来生成压缩报告:
def generate_report(files, destination):
report_path = f'{destination}.report.txt'
with open(report_path, 'w') as report_file:
report_file.write('Compressed Files:\n')
for file in files:
report_file.write(f'{file}\n')
report_file.write(f'\nTotal files compressed: {len(files)}\n')
print(f'Compression report saved to {report_path}')
3. 调用生成报告的压缩函数
在 __main__
部分中调用生成报告的压缩函数:
if __name__ == '__main__':
args = parse_args()
try:
if args.method == 'zipfile':
zip_files_with_report(args.source, args.destination)
elif args.method == 'shutil':
compress_with_shutil(args.source, args.destination, args.format)
except Exception as e:
print(f'Error: {e}')
六、总结
通过以上步骤,我们详细介绍了如何使用 zipfile
和 shutil
模块在命令行中压缩文件和目录,并且通过增加命令行参数解析、异常处理、多线程压缩和生成压缩报告等功能,使脚本更加健壮和高效。希望这篇文章能帮助你更好地理解和使用 Python 进行文件压缩操作。
相关问答FAQs:
如何在Python中使用命令行压缩文件?
在Python中,可以使用zipfile
模块来压缩文件。通过命令行,你可以编写一个简单的Python脚本来指定要压缩的文件或文件夹,并将其打包成zip格式。只需确保在命令行中调用该脚本,并传入相应的文件路径即可。
是否可以使用Python的其他库进行文件压缩?
是的,除了zipfile
,Python还有其他库可以用于压缩文件,比如tarfile
和gzip
。tarfile
适用于创建.tar或.tar.gz格式的压缩包,而gzip
则主要用于压缩单个文件。根据需求选择合适的库,可以获得更好的压缩效果。
如何在命令行中运行Python脚本以执行压缩操作?
要在命令行中运行Python脚本,首先确保你的系统已安装Python。接着,打开终端,导航到包含脚本的目录,输入python script_name.py
(将script_name.py
替换为你的脚本名称)并加上相关参数,如文件路径,按回车执行。确保脚本中正确处理了命令行参数,以便压缩所需的文件或文件夹。
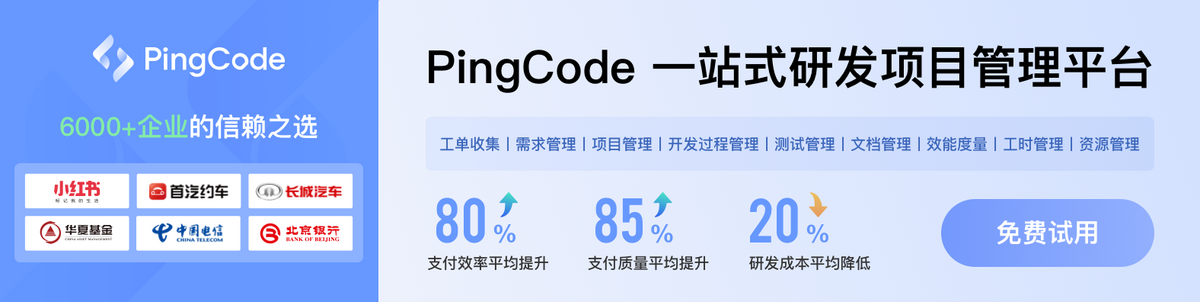