Python中可以使用多种方法来格式化数据,包括字符串格式化、f字符串、格式化方法和外部库。这些方法各有优缺点,适用于不同的应用场景。本文将详细介绍这些方法,并提供示例代码。
一、字符串格式化
字符串格式化是最早期的格式化方法,使用百分号(%)操作符来格式化字符串。它类似于C语言的printf函数。
1、基本用法
name = "John"
age = 25
formatted_string = "Name: %s, Age: %d" % (name, age)
print(formatted_string) # 输出:Name: John, Age: 25
2、格式化日期和时间
你可以使用时间模块来格式化日期和时间:
import time
current_time = time.localtime()
formatted_time = "Current time: %02d:%02d:%02d" % (current_time.tm_hour, current_time.tm_min, current_time.tm_sec)
print(formatted_time) # 输出:Current time: 14:30:15
二、使用format()方法
format()方法是Python 3引入的一种格式化字符串的方法,比百分号操作符更强大和灵活。
1、基本用法
name = "John"
age = 25
formatted_string = "Name: {}, Age: {}".format(name, age)
print(formatted_string) # 输出:Name: John, Age: 25
2、指定位置
你可以指定位置来格式化字符串:
formatted_string = "Name: {0}, Age: {1}".format(name, age)
print(formatted_string) # 输出:Name: John, Age: 25
3、命名参数
你还可以使用命名参数来格式化字符串:
formatted_string = "Name: {name}, Age: {age}".format(name=name, age=age)
print(formatted_string) # 输出:Name: John, Age: 25
三、使用f字符串(f-strings)
f字符串是Python 3.6引入的一种格式化字符串的方法,使用前缀f或F,并在字符串中使用花括号{}来包含表达式。
1、基本用法
name = "John"
age = 25
formatted_string = f"Name: {name}, Age: {age}"
print(formatted_string) # 输出:Name: John, Age: 25
2、表达式求值
你可以在f字符串中直接求值表达式:
a = 5
b = 3
formatted_string = f"Sum: {a + b}"
print(formatted_string) # 输出:Sum: 8
3、格式化数字
你可以使用格式说明符来格式化数字:
pi = 3.141592653589793
formatted_string = f"Pi: {pi:.2f}"
print(formatted_string) # 输出:Pi: 3.14
四、使用外部库
在某些复杂的格式化需求下,使用外部库可能是更好的选择,比如Pandas、NumPy等。
1、Pandas格式化数据
Pandas是一个强大的数据处理和分析库,特别适用于处理表格数据。
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter'],
'Age': [28, 24, 35]}
df = pd.DataFrame(data)
格式化数据
formatted_df = df.style.format({'Age': '{:.1f}'})
print(formatted_df)
2、NumPy格式化数据
NumPy是一个用于科学计算的库,特别适用于处理数组和矩阵。
import numpy as np
data = np.array([3.141592653589793, 2.718281828459045, 1.618033988749895])
formatted_data = np.round(data, 2)
print(formatted_data) # 输出:[3.14 2.72 1.62]
五、格式化日期和时间
Python中有多个库可以用于格式化日期和时间,最常用的是datetime模块。
1、使用datetime模块
from datetime import datetime
now = datetime.now()
formatted_time = now.strftime("%Y-%m-%d %H:%M:%S")
print(formatted_time) # 输出:2023-10-23 14:30:15
2、使用arrow库
arrow是一个第三方库,简化了日期和时间的处理。
import arrow
now = arrow.now()
formatted_time = now.format('YYYY-MM-DD HH:mm:ss')
print(formatted_time) # 输出:2023-10-23 14:30:15
六、格式化JSON数据
JSON是一种常用的数据交换格式,Python内置了json模块来处理JSON数据。
1、格式化输出JSON
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
formatted_json = json.dumps(data, indent=4)
print(formatted_json)
2、从文件读取和格式化JSON
with open('data.json', 'r') as file:
data = json.load(file)
formatted_json = json.dumps(data, indent=4)
print(formatted_json)
七、格式化CSV数据
CSV是一种常见的文本数据格式,Python内置了csv模块来处理CSV数据。
1、读取和格式化CSV
import csv
with open('data.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(', '.join(row))
2、写入CSV
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age', 'City'])
writer.writerow(['John', 30, 'New York'])
八、格式化XML数据
XML是一种常用的标记语言,Python可以使用xml.etree.ElementTree模块来处理XML数据。
1、解析和格式化XML
import xml.etree.ElementTree as ET
tree = ET.parse('data.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
2、生成和格式化XML
root = ET.Element("root")
doc = ET.SubElement(root, "doc")
ET.SubElement(doc, "field1", name="Name").text = "John"
ET.SubElement(doc, "field2", name="Age").text = "30"
tree = ET.ElementTree(root)
tree.write("output.xml", xml_declaration=True, encoding='utf-8')
九、格式化SQL数据
SQL是结构化查询语言,用于操作关系型数据库。Python可以使用sqlite3模块来处理SQL数据。
1、读取和格式化SQL数据
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute("SELECT * FROM users")
rows = cursor.fetchall()
for row in rows:
print(row)
2、写入SQL数据
cursor.execute("INSERT INTO users (name, age) VALUES (?, ?)", ('John', 30))
conn.commit()
十、格式化二进制数据
二进制数据的格式化和处理在某些应用场景下是非常重要的,Python可以使用struct模块来处理二进制数据。
1、打包和解包二进制数据
import struct
打包数据
packed_data = struct.pack('iif', 1, 2, 3.14)
print(packed_data)
解包数据
unpacked_data = struct.unpack('iif', packed_data)
print(unpacked_data) # 输出:(1, 2, 3.14)
十一、格式化HTML数据
HTML是网页的标记语言,Python可以使用BeautifulSoup库来处理HTML数据。
1、解析和格式化HTML
from bs4 import BeautifulSoup
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time...</p>
</body></html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
formatted_html = soup.prettify()
print(formatted_html)
十二、格式化Markdown数据
Markdown是一种轻量级标记语言,Python可以使用markdown库来处理Markdown数据。
1、Markdown转换为HTML
import markdown
md_text = """
This is a heading
This is some text with <strong>bold</strong> and *italic* formatting.
"""
html = markdown.markdown(md_text)
print(html)
十三、格式化日志数据
日志数据在应用程序的调试和监控中非常重要,Python可以使用logging模块来处理日志数据。
1、基本用法
import logging
logging.basicConfig(level=logging.INFO)
logging.info("This is an info message")
2、格式化日志输出
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s'
)
logging.info("This is an info message")
十四、格式化Excel数据
Excel是常见的电子表格软件,Python可以使用openpyxl和pandas库来处理Excel数据。
1、使用openpyxl读取和格式化Excel
from openpyxl import load_workbook
wb = load_workbook('data.xlsx')
ws = wb.active
for row in ws.iter_rows(values_only=True):
print(row)
2、使用pandas读取和格式化Excel
import pandas as pd
df = pd.read_excel('data.xlsx')
print(df)
十五、格式化YAML数据
YAML是一种易读的配置文件格式,Python可以使用PyYAML库来处理YAML数据。
1、读取和格式化YAML
import yaml
with open('data.yaml', 'r') as file:
data = yaml.safe_load(file)
print(data)
2、写入YAML
with open('output.yaml', 'w') as file:
yaml.safe_dump(data, file)
十六、格式化二进制大数据
处理大数据时,二进制格式如Parquet和Avro非常常见。Python可以使用PyArrow库来处理这些数据。
1、读取和格式化Parquet
import pyarrow.parquet as pq
table = pq.read_table('data.parquet')
df = table.to_pandas()
print(df)
2、写入Parquet
pq.write_table(table, 'output.parquet')
十七、格式化protobuf数据
Protocol Buffers(protobuf)是Google开发的一种高效的二进制序列化格式。Python可以使用protobuf库来处理这些数据。
1、定义protobuf消息
syntax = "proto3";
message Person {
string name = 1;
int32 age = 2;
}
2、使用protobuf库
from person_pb2 import Person
序列化
person = Person(name="John", age=30)
binary_data = person.SerializeToString()
反序列化
new_person = Person()
new_person.ParseFromString(binary_data)
print(new_person)
十八、总结
Python提供了丰富的库和方法来格式化各种类型的数据。选择合适的方法和库不仅可以提高代码的可读性,还可以提高程序的性能和可维护性。了解和掌握这些技术,将极大地提升你的编程效率和数据处理能力。
相关问答FAQs:
如何在Python中格式化字符串?
在Python中,可以使用多种方法来格式化字符串,包括f-字符串、str.format()
方法和百分号(%)格式化。f-字符串是Python 3.6及以上版本推荐的方式,语法简洁直观。例如,使用f-字符串可以这样写:name = "Alice"; age = 30; formatted_string = f"My name is {name} and I am {age} years old."
。这种方法不仅使代码更易读,还可以直接在字符串中嵌入变量。
Python中如何处理浮点数格式化?
处理浮点数格式化时,可以使用format()
方法或f-字符串来指定小数位数。例如,value = 3.14159; formatted_value = f"{value:.2f}"
会将浮点数格式化为两位小数。使用这种方式可以确保在输出中获得一致的数值表现,特别在财务或科学计算中非常重要。
怎样在Python中格式化日期和时间?
在Python中,可以使用datetime
模块来格式化日期和时间。通过strftime()
方法,可以将日期对象转换为字符串格式。比如,from datetime import datetime; now = datetime.now(); formatted_date = now.strftime("%Y-%m-%d %H:%M:%S")
会输出当前时间为"年-月-日 时:分:秒"的格式。这种灵活的格式化能力使得日期和时间的处理更为便捷。
在Python中如何格式化输出列表或字典?
如果希望对列表或字典进行格式化输出,可以使用循环结合f-字符串或json
模块。对于字典,使用json.dumps()
可以将其格式化为JSON字符串,便于阅读。例如,import json; my_dict = {"name": "Alice", "age": 30}; formatted_dict = json.dumps(my_dict, indent=4)
会以美化的方式输出字典内容。对于列表,可以遍历并格式化每个元素,以提升输出的可读性。
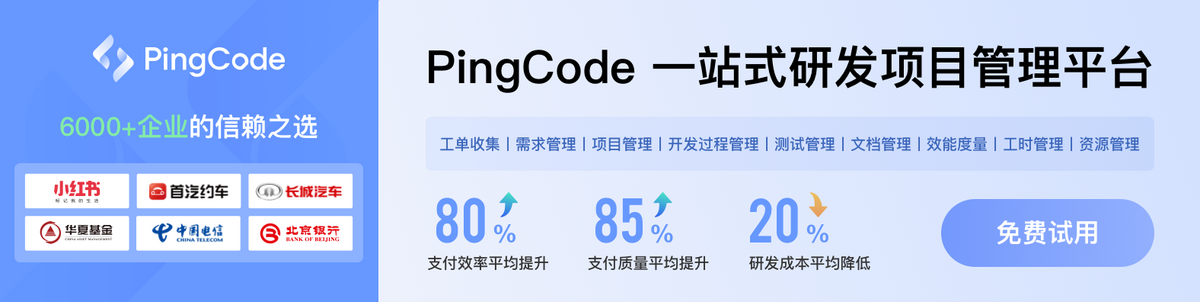