Python中同时判断多个条件可以使用'and'、'or'和'not'关键字。 这些关键字用来将多个条件结合在一起进行判断。例如,'and'表示两个条件都要满足,'or'表示任意一个条件满足即可,'not'表示条件取反。使用这些关键字可以简化代码逻辑并提高代码的可读性。 具体来说,'and'关键字非常常用,可以大大减少冗余代码。例如:
age = 25
income = 50000
if age > 18 and income > 30000:
print("Eligible for loan")
在这个例子中,只有当age
大于18并且income
大于30000时,才会输出“Eligible for loan”。下面将详细介绍这三个关键字及其使用场景。
一、AND关键字的使用
'and'关键字用于同时满足多个条件时。所有条件都必须为真,整个表达式才为真。否则,只要有一个条件为假,整个表达式就为假。
1. 基本用法
a = 10
b = 20
c = 30
if a < b and b < c:
print("Both conditions are True")
else:
print("One or both conditions are False")
在这个例子中,a < b
和b < c
两个条件都为真,所以会输出“Both conditions are True”。
2. 复杂条件
在实际开发中,判断条件往往会比较复杂,可能需要结合多个条件进行判断。此时,可以使用'and'关键字将多个条件组合在一起。
age = 30
income = 60000
credit_score = 700
if age > 18 and income > 40000 and credit_score > 650:
print("Eligible for premium loan")
else:
print("Not eligible for premium loan")
在这个例子中,只有当age
大于18,income
大于40000,并且credit_score
大于650时,才会输出“Eligible for premium loan”。
二、OR关键字的使用
'or'关键字用于满足任一条件即可。只要有一个条件为真,整个表达式就为真。只有当所有条件都为假时,整个表达式才为假。
1. 基本用法
a = 10
b = 20
c = 5
if a > b or c < b:
print("At least one condition is True")
else:
print("Both conditions are False")
在这个例子中,a > b
为假,但c < b
为真,所以会输出“At least one condition is True”。
2. 复杂条件
在实际开发中,判断条件可能会比较复杂,此时可以使用'or'关键字将多个条件组合在一起。
temperature = 35
humidity = 85
weather = "sunny"
if temperature > 30 or humidity > 80 or weather == "sunny":
print("It might be a hot day")
else:
print("The weather is moderate")
在这个例子中,只要temperature
大于30,或humidity
大于80,或weather
等于"sunny"中的任一条件为真,就会输出“It might be a hot day”。
三、NOT关键字的使用
'not'关键字用于取反。即如果条件为真,则整个表达式为假;如果条件为假,则整个表达式为真。
1. 基本用法
a = 10
b = 20
if not a > b:
print("a is not greater than b")
else:
print("a is greater than b")
在这个例子中,a > b
为假,取反后为真,所以会输出“a is not greater than b”。
2. 复杂条件
在实际开发中,判断条件可能会比较复杂,此时可以使用'not'关键字将条件取反。
age = 25
is_student = False
if not (age < 18 or is_student):
print("Eligible for full membership")
else:
print("Not eligible for full membership")
在这个例子中,如果age
小于18或is_student
为真,则整个条件为假,取反后为真,所以会输出“Eligible for full membership”。
四、AND、OR、NOT组合使用
在实际开发中,可能需要同时使用and
、or
和not
关键字来处理复杂的判断逻辑。
1. 组合使用
age = 22
income = 30000
is_student = True
if (age > 18 and income > 25000) or (is_student and income > 20000):
print("Eligible for special loan")
else:
print("Not eligible for special loan")
在这个例子中,只要满足age
大于18并且income
大于25000,或者is_student
为真并且income
大于20000中的任一条件,就会输出“Eligible for special loan”。
2. 使用括号明确优先级
当判断条件非常复杂时,可以使用括号来明确优先级,避免逻辑混淆。
age = 20
income = 15000
credit_score = 650
is_student = True
if (age > 18 and (income > 20000 or credit_score > 600)) and not is_student:
print("Eligible for standard loan")
else:
print("Not eligible for standard loan")
在这个例子中,使用了括号来明确条件的优先级,先判断age > 18
和(income > 20000 or credit_score > 600)
,然后再判断是否not is_student
。只有在所有条件都为真时,才会输出“Eligible for standard loan”。
五、实践中的应用
在实际开发中,合理使用and
、or
和not
关键字能够简化代码逻辑,提高代码的可读性和维护性。
1. 用户认证
在用户认证中,通常需要同时判断多个条件,例如用户名和密码是否正确,用户是否被冻结等。
username = "user1"
password = "password123"
is_active = True
if username == "user1" and password == "password123" and is_active:
print("Login successful")
else:
print("Login failed")
在这个例子中,只有当用户名、密码正确,并且用户未被冻结时,才会输出“Login successful”。
2. 数据验证
在数据验证中,通常需要判断多个条件,例如数据是否为空,数据类型是否正确等。
data = "example"
is_valid = True
is_not_empty = bool(data)
if is_valid and is_not_empty:
print("Data is valid and not empty")
else:
print("Data is invalid or empty")
在这个例子中,只有当数据有效并且不为空时,才会输出“Data is valid and not empty”。
3. 访问权限控制
在访问权限控制中,通常需要判断用户的角色和权限,例如用户是否为管理员,是否有访问权限等。
role = "admin"
has_permission = True
if role == "admin" or has_permission:
print("Access granted")
else:
print("Access denied")
在这个例子中,只要用户是管理员,或者有访问权限,就会输出“Access granted”。
六、注意事项
在使用and
、or
和not
关键字时,需要注意以下几点:
1. 优先级
在Python中,not
的优先级最高,其次是and
,最后是or
。在进行复杂判断时,可以使用括号来明确优先级,避免逻辑混淆。
condition1 = True
condition2 = False
condition3 = True
result = condition1 and not condition2 or condition3
print(result) # 输出: True
在这个例子中,由于not
的优先级最高,所以先对condition2
进行取反,然后再进行and
和or
的判断。
2. 短路求值
在Python中,and
和or
都具有短路求值的特性。即在判断条件时,如果前面的条件已经能够确定整个表达式的结果,则后面的条件将不会被判断。
def check1():
print("check1 called")
return True
def check2():
print("check2 called")
return False
result = check1() and check2() # 只会输出 "check1 called"
print(result) # 输出: False
在这个例子中,由于check1()
返回True
,所以会继续判断check2()
。但如果check1()
返回False
,则不会继续判断check2()
,因为整个表达式已经可以确定为False
。
3. 可读性
在编写代码时,尽量保持代码的简洁和可读性。如果判断条件过于复杂,可以考虑将其拆分成多个简单的条件,以提高代码的可读性。
age = 25
income = 40000
credit_score = 700
is_student = False
拆分成多个简单的条件
is_adult = age > 18
has_good_income = income > 30000
has_good_credit = credit_score > 650
is_not_student = not is_student
if is_adult and has_good_income and has_good_credit and is_not_student:
print("Eligible for special offer")
else:
print("Not eligible for special offer")
在这个例子中,将复杂的判断条件拆分成多个简单的条件,提高了代码的可读性。
七、示例应用场景
为了更好地理解如何同时判断多个条件,下面列举一些实际应用场景。
1. 表单验证
在表单验证中,通常需要同时判断多个条件,例如用户名、密码、邮箱等是否符合要求。
username = "user123"
password = "pass123"
email = "user@example.com"
is_valid_username = len(username) >= 5
is_valid_password = len(password) >= 6
is_valid_email = "@" in email and "." in email
if is_valid_username and is_valid_password and is_valid_email:
print("Form is valid")
else:
print("Form is invalid")
在这个例子中,只有当用户名、密码和邮箱都符合要求时,才会输出“Form is valid”。
2. 商品筛选
在电商平台上,通常需要根据多个条件筛选商品,例如价格、评分、库存等。
price = 100
rating = 4.5
in_stock = True
is_affordable = price <= 150
has_good_rating = rating >= 4.0
is_available = in_stock
if is_affordable and has_good_rating and is_available:
print("Product meets the criteria")
else:
print("Product does not meet the criteria")
在这个例子中,只有当商品价格合适、评分高并且有库存时,才会输出“Product meets the criteria”。
3. 动态权限控制
在某些应用中,可能需要根据多个条件动态控制用户的权限,例如用户角色、权限等级等。
role = "editor"
permission_level = 3
is_admin = role == "admin"
is_editor_with_high_permission = role == "editor" and permission_level > 2
if is_admin or is_editor_with_high_permission:
print("Access granted")
else:
print("Access denied")
在这个例子中,只要用户是管理员,或者是权限等级大于2的编辑,就会输出“Access granted”。
八、总结
通过上述内容,我们详细介绍了如何在Python中同时判断多个条件。主要使用的关键字是and
、or
和not
,它们可以结合使用来处理复杂的判断逻辑。 在实际开发中,合理使用这些关键字能够简化代码逻辑,提高代码的可读性和维护性。希望通过本文的介绍,能够帮助你更好地理解和掌握这部分内容,并在实际项目中灵活应用。
相关问答FAQs:
在Python中,如何使用逻辑运算符来判断多个条件?
在Python中,可以使用逻辑运算符and
、or
和not
来判断多个条件。and
运算符用于当所有条件都为真时返回真,or
运算符则是在任一条件为真时返回真,而not
运算符则用于反转条件的真值。例如,可以这样写:if condition1 and condition2:
来判断两个条件是否同时为真。
如何在Python中使用列表或字典来判断多个条件?
可以通过遍历列表或字典中的元素来判断多个条件。例如,使用any()
或all()
函数可以方便地检查条件。例如,if all(x > 0 for x in my_list):
可以检查列表中的所有元素是否都大于0,而if any(x > 0 for x in my_list):
则检查是否至少有一个元素大于0。
Python中有哪些常见的多条件判断实例?
在Python中,常见的多条件判断实例包括用户输入验证、数据过滤以及复杂的业务逻辑处理。例如,可以使用if
语句结合多个条件来验证用户输入是否符合特定格式或要求,或者在数据分析中筛选出符合特定条件的数据集。这些实例不仅提高了代码的可读性,还能增强程序的灵活性。
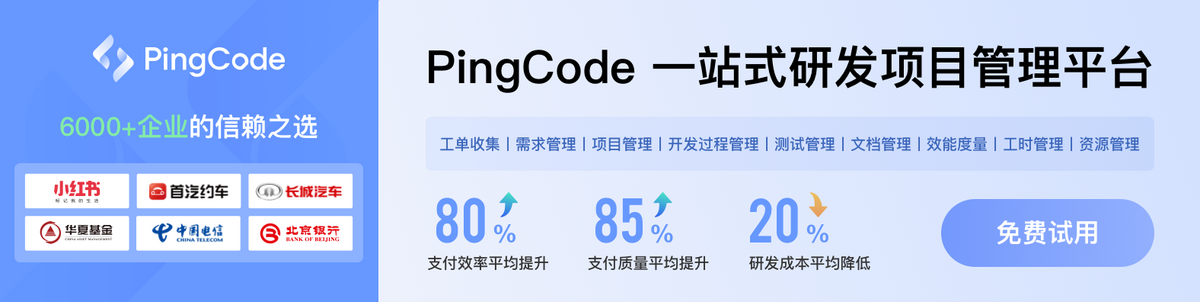