要用Python判断字符类型,可以通过字符串方法、正则表达式、Unicode分类等方式来实现。其中,字符串方法如str.isdigit()
、str.isalpha()
、str.isalnum()
等非常方便、正则表达式能够进行复杂的模式匹配、Unicode分类则可以用于处理国际化字符。具体选择哪种方法取决于具体的应用场景。例如,使用str.isdigit()
方法可以简单判断一个字符串是否只包含数字字符。
一、字符串方法
字符串方法是Python内置的功能,能够非常方便地判断字符串的某些属性。
1.1、str.isdigit()
str.isdigit()
方法用于判断字符串是否只包含数字字符。它返回一个布尔值,如果字符串只包含数字字符(包括全角字符),则返回True,否则返回False。
num_str = "12345"
print(num_str.isdigit()) # 输出: True
non_num_str = "12345abc"
print(non_num_str.isdigit()) # 输出: False
1.2、str.isalpha()
str.isalpha()
方法用于判断字符串是否只包含字母字符。它返回一个布尔值,如果字符串只包含字母字符,则返回True,否则返回False。
alpha_str = "abcdef"
print(alpha_str.isalpha()) # 输出: True
non_alpha_str = "abc123"
print(non_alpha_str.isalpha()) # 输出: False
1.3、str.isalnum()
str.isalnum()
方法用于判断字符串是否只包含字母和数字字符。它返回一个布尔值,如果字符串只包含字母和数字字符,则返回True,否则返回False。
alnum_str = "abc123"
print(alnum_str.isalnum()) # 输出: True
non_alnum_str = "abc123!"
print(non_alnum_str.isalnum()) # 输出: False
二、正则表达式
正则表达式(Regular Expression,简称Regex)是处理字符串匹配的强大工具。Python提供了re
模块来支持正则表达式。
2.1、数字字符匹配
可以使用正则表达式来匹配字符串是否只包含数字字符。
import re
num_pattern = re.compile(r'^\d+$')
num_str = "12345"
print(bool(num_pattern.match(num_str))) # 输出: True
non_num_str = "12345abc"
print(bool(num_pattern.match(non_num_str))) # 输出: False
2.2、字母字符匹配
同样可以使用正则表达式来匹配字符串是否只包含字母字符。
alpha_pattern = re.compile(r'^[a-zA-Z]+$')
alpha_str = "abcdef"
print(bool(alpha_pattern.match(alpha_str))) # 输出: True
non_alpha_str = "abc123"
print(bool(alpha_pattern.match(non_alpha_str))) # 输出: False
2.3、字母和数字字符匹配
也可以使用正则表达式来匹配字符串是否只包含字母和数字字符。
alnum_pattern = re.compile(r'^[a-zA-Z0-9]+$')
alnum_str = "abc123"
print(bool(alnum_pattern.match(alnum_str))) # 输出: True
non_alnum_str = "abc123!"
print(bool(alnum_pattern.match(non_alnum_str))) # 输出: False
三、Unicode分类
对于需要处理国际化字符的场景,可以使用unicodedata
模块来判断字符类型。unicodedata
模块提供了对Unicode字符属性的访问。
3.1、判断是否为数字字符
使用unicodedata
模块可以判断一个字符是否为数字字符。
import unicodedata
def is_digit(char):
return unicodedata.category(char).startswith('N')
num_char = '3'
print(is_digit(num_char)) # 输出: True
non_num_char = 'a'
print(is_digit(non_num_char)) # 输出: False
3.2、判断是否为字母字符
使用unicodedata
模块可以判断一个字符是否为字母字符。
def is_alpha(char):
return unicodedata.category(char).startswith('L')
alpha_char = 'a'
print(is_alpha(alpha_char)) # 输出: True
non_alpha_char = '1'
print(is_alpha(non_alpha_char)) # 输出: False
四、综合应用
在实际应用中,可能需要综合多种方法来判断字符串的类型。例如,判断一个字符串是否包含某种类型的字符,同时不包含其他类型的字符。
4.1、判断字符串是否只包含字母和数字字符
可以结合字符串方法和正则表达式来实现更复杂的判断逻辑。
import re
def is_alnum_str(s):
alnum_pattern = re.compile(r'^[a-zA-Z0-9]+$')
return bool(alnum_pattern.match(s))
alnum_str = "abc123"
print(is_alnum_str(alnum_str)) # 输出: True
non_alnum_str = "abc123!"
print(is_alnum_str(non_alnum_str)) # 输出: False
4.2、判断字符串是否只包含特定类型的Unicode字符
可以结合unicodedata
模块来实现更复杂的判断逻辑。
import unicodedata
def is_specific_unicode_str(s, category_prefix):
for char in s:
if not unicodedata.category(char).startswith(category_prefix):
return False
return True
判断字符串是否只包含字母字符
alpha_str = "abcdef"
print(is_specific_unicode_str(alpha_str, 'L')) # 输出: True
判断字符串是否只包含数字字符
num_str = "12345"
print(is_specific_unicode_str(num_str, 'N')) # 输出: True
判断字符串是否只包含字母和数字字符
alnum_str = "abc123"
print(is_specific_unicode_str(alnum_str, 'L') or is_specific_unicode_str(alnum_str, 'N')) # 输出: False
五、实战案例
通过上述方法,我们可以处理各种字符类型判断的需求。下面是一些实战案例,帮助更好地理解这些方法的应用。
5.1、验证用户输入的电话号码
电话号码通常只包含数字字符,有时还包含加号、空格或短横线。可以使用正则表达式来验证用户输入的电话号码是否符合规范。
import re
def is_valid_phone_number(phone_number):
phone_pattern = re.compile(r'^\+?\d[\d\s-]*$')
return bool(phone_pattern.match(phone_number))
phone_number = "+1 234-567-890"
print(is_valid_phone_number(phone_number)) # 输出: True
invalid_phone_number = "+1 234-567-890a"
print(is_valid_phone_number(invalid_phone_number)) # 输出: False
5.2、验证用户输入的邮箱地址
邮箱地址通常包含字母、数字、点号和特殊字符。可以使用正则表达式来验证用户输入的邮箱地址是否符合规范。
import re
def is_valid_email(email):
email_pattern = re.compile(r'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$')
return bool(email_pattern.match(email))
email = "user@example.com"
print(is_valid_email(email)) # 输出: True
invalid_email = "user@example"
print(is_valid_email(invalid_email)) # 输出: False
5.3、验证用户输入的密码
密码通常要求包含字母、数字和特殊字符,可以结合正则表达式和字符串方法来验证用户输入的密码是否符合规范。
import re
def is_valid_password(password):
if len(password) < 8:
return False
has_upper = any(char.isupper() for char in password)
has_lower = any(char.islower() for char in password)
has_digit = any(char.isdigit() for char in password)
has_special = any(char in "!@#$%^&*()-_=+[]{}|;:'\",.<>?/`~" for char in password)
return has_upper and has_lower and has_digit and has_special
password = "Passw0rd!"
print(is_valid_password(password)) # 输出: True
invalid_password = "password"
print(is_valid_password(invalid_password)) # 输出: False
六、总结
Python提供了多种方法来判断字符类型,包括字符串方法、正则表达式和Unicode分类。不同的方法有不同的适用场景,可以根据具体需求选择合适的方法。字符串方法适用于简单的判断,正则表达式适用于复杂的模式匹配,Unicode分类适用于处理国际化字符。综合应用这些方法,可以实现对字符类型的灵活判断和验证。通过实战案例,我们可以更好地理解和应用这些方法,解决实际问题。
相关问答FAQs:
如何在Python中判断一个字符是否为字母?
在Python中,可以使用字符串方法isalpha()
来判断字符是否为字母。该方法会返回布尔值,若字符是字母则返回True
,否则返回False
。例如,'A'.isalpha()
返回True
,而'1'.isalpha()
返回False
。
可以用什么方法判断字符是否为数字?
要判断字符是否为数字,可以使用字符串方法isdigit()
。该方法同样返回布尔值,若字符为数字则返回True
,否则返回False
。例如,'5'.isdigit()
返回True
,而'a'.isdigit()
返回False
。
如何判断字符是否为特殊字符或空白字符?
可以使用isspace()
方法来判断一个字符是否为空白字符(如空格、制表符等)。对于特殊字符的判断,可以结合使用string
模块中的punctuation
属性,使用in
运算符检查字符是否存在于该属性中。示例代码为:char in string.punctuation
,若字符为特殊字符则返回True
。
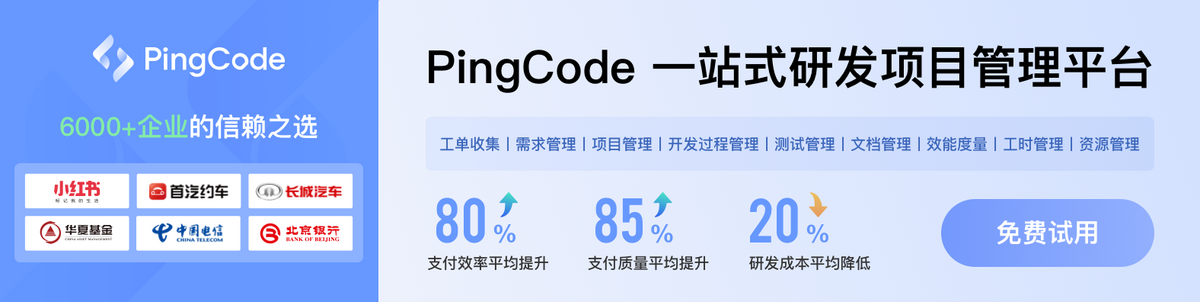