要在Python中移动折线图,有几种方法可以实现,主要包括更改数据坐标、使用动画库、调整图形位置。其中,更改数据坐标是最为常用和直接的方法。通过更改折线图的坐标数据,可以使折线图在图形中移动。下面详细描述如何实现这一点。
更改数据坐标
更改数据坐标是通过修改绘图数据中的 x 和 y 值来实现的。我们以 matplotlib
库为例,展示如何移动折线图。以下是具体步骤:
- 导入必要的库:首先导入
matplotlib
和numpy
库,用于绘制图形和生成数据。 - 生成数据:创建一个简单的折线图数据集。
- 绘制初始图形:用
matplotlib
生成初始折线图。 - 更新数据:通过改变数据的 x 和 y 坐标来移动折线图。
- 重绘图形:更新图形以展示新的数据位置。
import matplotlib.pyplot as plt
import numpy as np
生成初始数据
x = np.linspace(0, 10, 100)
y = np.sin(x)
创建初始图形
fig, ax = plt.subplots()
line, = ax.plot(x, y)
函数:更新数据并重绘图形
def update_line(new_x, new_y):
line.set_xdata(new_x)
line.set_ydata(new_y)
ax.relim()
ax.autoscale_view()
plt.draw()
移动折线图
new_x = x + 2 # 将 x 坐标整体右移2个单位
new_y = y # y 坐标保持不变
update_line(new_x, new_y)
plt.show()
使用动画库
除了直接更改数据坐标外,使用 matplotlib.animation
库可以创建动画效果,使折线图看起来像是动态移动的。
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
生成初始数据
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, ax = plt.subplots()
line, = ax.plot(x, y)
更新函数
def update(frame):
new_x = x + frame * 0.1 # 每帧移动 0.1 个单位
line.set_xdata(new_x)
ax.relim()
ax.autoscale_view()
return line,
ani = animation.FuncAnimation(fig, update, frames=np.arange(0, 20), interval=50, blit=True)
plt.show()
一、更改数据坐标
更改数据坐标是最为常见的方法,通过直接修改折线图的数据点坐标,来实现图形的移动。这种方法简单直接,并且不需要额外的库支持。
1、生成初始数据
首先,我们需要生成一些初始数据,作为绘制折线图的基础。常用的方法是使用 numpy
库生成等间隔的数据点。
import numpy as np
x = np.linspace(0, 10, 100) # 生成0到10之间的100个等间隔数据点
y = np.sin(x) # 使用正弦函数生成y值
2、绘制初始图形
使用 matplotlib
库来绘制初始的折线图。
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
line, = ax.plot(x, y)
plt.show()
3、更新数据并重绘图形
通过更改数据的 x 和 y 坐标,并调用 set_xdata
和 set_ydata
方法来更新图形。
def update_line(new_x, new_y):
line.set_xdata(new_x)
line.set_ydata(new_y)
ax.relim()
ax.autoscale_view()
plt.draw()
4、移动折线图
接下来,我们可以通过改变 x 和 y 的值来移动折线图。例如,将 x 坐标整体右移2个单位。
new_x = x + 2
new_y = y
update_line(new_x, new_y)
二、使用动画库
使用 matplotlib.animation
库可以创建动态效果,使折线图看起来像是连续移动的。这种方法适用于需要动态展示数据变化的场景。
1、导入动画库
首先,需要导入 matplotlib.animation
库。
import matplotlib.animation as animation
2、定义更新函数
定义一个更新函数,用于在每一帧中更新数据。
def update(frame):
new_x = x + frame * 0.1 # 每帧移动 0.1 个单位
line.set_xdata(new_x)
ax.relim()
ax.autoscale_view()
return line,
3、创建动画
使用 FuncAnimation
方法创建动画,并设置帧数和间隔时间。
ani = animation.FuncAnimation(fig, update, frames=np.arange(0, 20), interval=50, blit=True)
plt.show()
三、调整图形位置
调整图形位置的另一种方法是通过修改图形的布局参数,使整个图形在绘图区内移动。这种方法适用于需要整体平移图形的场景。
1、设置图形布局参数
使用 matplotlib
中的 subplots_adjust
方法可以调整图形的布局参数。
fig.subplots_adjust(left=0.2, right=0.8, top=0.8, bottom=0.2)
2、动态调整布局参数
通过动态调整布局参数,可以实现图形的移动效果。
import matplotlib.animation as animation
def update(frame):
fig.subplots_adjust(left=0.2 + frame * 0.01, right=0.8 + frame * 0.01)
plt.draw()
ani = animation.FuncAnimation(fig, update, frames=np.arange(0, 20), interval=50)
plt.show()
四、总结
在Python中,移动折线图的方法多种多样,可以根据具体需求选择合适的方法。更改数据坐标是最为常用和直接的方法,通过修改绘图数据中的 x 和 y 值,可以实现图形的移动。使用动画库可以创建动态效果,使折线图看起来像是连续移动的,非常适用于需要动态展示数据变化的场景。调整图形位置则是通过修改图形的布局参数,使整个图形在绘图区内移动,适用于需要整体平移图形的场景。无论采用哪种方法,都可以通过 matplotlib
库实现灵活的图形移动效果。
相关问答FAQs:
如何使用Python创建和移动折线图?
在Python中,可以使用Matplotlib库来创建折线图。通过调整图形的坐标轴和数据点,可以实现折线图的移动。使用plt.plot()
函数绘制折线图,然后通过plt.xlim()
和plt.ylim()
函数来设置坐标轴的范围,从而移动图形的位置。
在Python中,折线图的动态更新是如何实现的?
动态更新折线图可以通过使用Matplotlib的动画模块FuncAnimation
实现。该模块允许在图形中实时更新数据,从而使折线图看起来像是在移动。通过不断更新数据点并调用plt.draw()
,可以实现这种动态效果。
除了Matplotlib,还有哪些Python库可以用于移动折线图?
除了Matplotlib,Plotly和Seaborn也是常用的可视化库。Plotly提供了交互式图形功能,用户可以通过拖动和缩放图形来移动折线图。而Seaborn则在数据可视化上提供了更为美观的默认样式,虽然它的移动功能相对较少,但也可以结合Matplotlib实现图形的自定义移动。
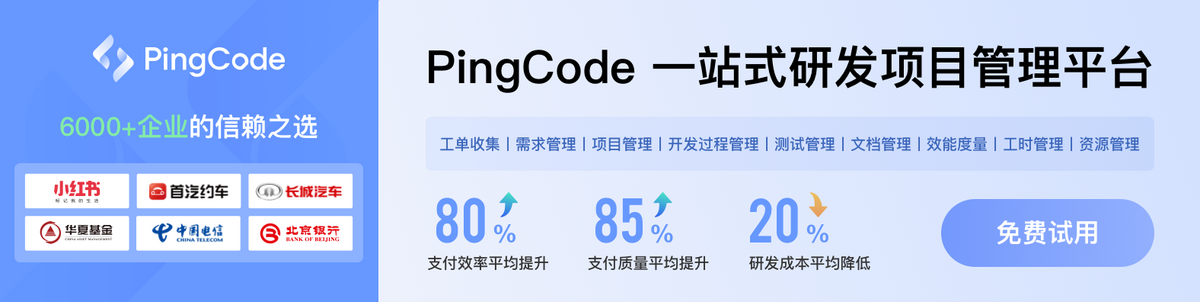