在Python中,比较对象的大小可以通过多种方式实现。使用内置比较运算符、实现特殊方法、使用functools模块的cmp_to_key函数、以及第三方库。下面我们将详细探讨这些方法中的一个,并展开讨论。
使用内置比较运算符
Python提供了一组内置的比较运算符,例如<
, <=
, >
, >=
, ==
, 和 !=
,这些运算符可以用于比较对象的大小。默认情况下,这些运算符只能用于比较内置类型(如数字、字符串、元组等)。要比较自定义对象,我们需要在类中实现相应的特殊方法,如__lt__
, __le__
, __gt__
, __ge__
, __eq__
和__ne__
。
详细描述:实现特殊方法
为了使自定义对象支持比较运算符,我们可以在类中实现相应的特殊方法。下面是一个示例,展示了如何在自定义类中实现这些方法:
class MyClass:
def __init__(self, value):
self.value = value
def __lt__(self, other):
return self.value < other.value
def __le__(self, other):
return self.value <= other.value
def __gt__(self, other):
return self.value > other.value
def __ge__(self, other):
return self.value >= other.value
def __eq__(self, other):
return self.value == other.value
def __ne__(self, other):
return self.value != other.value
示例用法
a = MyClass(10)
b = MyClass(20)
print(a < b) # 输出: True
print(a <= b) # 输出: True
print(a > b) # 输出: False
print(a >= b) # 输出: False
print(a == b) # 输出: False
print(a != b) # 输出: True
在上面的示例中,我们定义了一个MyClass
类,并在其中实现了__lt__
, __le__
, __gt__
, __ge__
, __eq__
和__ne__
方法。这些方法用于比较两个MyClass
对象的value
属性。通过实现这些方法,我们可以使用内置的比较运算符来比较自定义对象。
使用functools模块的cmp_to_key函数
在某些情况下,我们可能需要使用自定义的比较函数来比较对象。Python的functools
模块提供了一个cmp_to_key
函数,可以将自定义的比较函数转换为用于排序的键函数。以下是一个示例:
from functools import cmp_to_key
class MyClass:
def __init__(self, value):
self.value = value
def my_compare(x, y):
if x.value < y.value:
return -1
elif x.value > y.value:
return 1
else:
return 0
使用cmp_to_key将自定义的比较函数转换为键函数
key_func = cmp_to_key(my_compare)
a = MyClass(10)
b = MyClass(20)
c = MyClass(15)
my_list = [a, b, c]
使用sorted函数进行排序
sorted_list = sorted(my_list, key=key_func)
输出排序后的结果
for obj in sorted_list:
print(obj.value)
在上面的示例中,我们定义了一个MyClass
类和一个自定义的比较函数my_compare
。通过使用cmp_to_key
函数,我们将自定义的比较函数转换为键函数,并使用sorted
函数对对象列表进行排序。
使用第三方库
除了内置方法和functools模块外,还有一些第三方库可以帮助我们实现对象比较。例如,attr
库提供了简洁的方式来定义比较方法。以下是一个使用attr
库的示例:
import attr
@attr.s
class MyClass:
value = attr.ib()
def __lt__(self, other):
return self.value < other.value
def __le__(self, other):
return self.value <= other.value
def __gt__(self, other):
return self.value > other.value
def __ge__(self, other):
return self.value >= other.value
def __eq__(self, other):
return self.value == other.value
def __ne__(self, other):
return self.value != other.value
示例用法
a = MyClass(10)
b = MyClass(20)
print(a < b) # 输出: True
print(a <= b) # 输出: True
print(a > b) # 输出: False
print(a >= b) # 输出: False
print(a == b) # 输出: False
print(a != b) # 输出: True
在上面的示例中,我们使用attr
库定义了一个MyClass
类,并在其中实现了比较方法。这种方式简洁明了,可以大大减少代码量。
进一步探讨对象比较的细节
一、如何实现多重属性的比较
在某些情况下,我们可能需要根据多个属性来比较对象。例如,假设我们有一个包含name
和age
属性的类,我们希望首先比较name
,如果name
相同,则比较age
。以下是一个示例:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __lt__(self, other):
if self.name == other.name:
return self.age < other.age
return self.name < other.name
def __le__(self, other):
if self.name == other.name:
return self.age <= other.age
return self.name <= other.name
def __gt__(self, other):
if self.name == other.name:
return self.age > other.age
return self.name > other.name
def __ge__(self, other):
if self.name == other.name:
return self.age >= other.age
return self.name >= other.name
def __eq__(self, other):
return self.name == other.name and self.age == other.age
def __ne__(self, other):
return not self.__eq__(other)
示例用法
p1 = Person("Alice", 30)
p2 = Person("Bob", 25)
p3 = Person("Alice", 25)
print(p1 < p2) # 输出: True
print(p1 < p3) # 输出: False
print(p3 < p1) # 输出: True
在上面的示例中,我们定义了一个Person
类,并在其中实现了多重属性的比较方法。我们首先比较name
属性,如果name
相同,则比较age
属性。这种方式可以满足多重属性比较的需求。
二、如何处理不可比较的对象
在某些情况下,我们可能会遇到不可比较的对象。例如,比较两个不相关的类的实例。在这种情况下,我们可以在比较方法中抛出TypeError
异常。以下是一个示例:
class MyClass:
def __init__(self, value):
self.value = value
def __lt__(self, other):
if not isinstance(other, MyClass):
raise TypeError(f"Cannot compare MyClass with {type(other)}")
return self.value < other.value
def __le__(self, other):
if not isinstance(other, MyClass):
raise TypeError(f"Cannot compare MyClass with {type(other)}")
return self.value <= other.value
def __gt__(self, other):
if not isinstance(other, MyClass):
raise TypeError(f"Cannot compare MyClass with {type(other)}")
return self.value > other.value
def __ge__(self, other):
if not isinstance(other, MyClass):
raise TypeError(f"Cannot compare MyClass with {type(other)}")
return self.value >= other.value
def __eq__(self, other):
if not isinstance(other, MyClass):
raise TypeError(f"Cannot compare MyClass with {type(other)}")
return self.value == other.value
def __ne__(self, other):
if not isinstance(other, MyClass):
raise TypeError(f"Cannot compare MyClass with {type(other)}")
return self.value != other.value
示例用法
a = MyClass(10)
b = 20
try:
print(a < b) # 将抛出TypeError异常
except TypeError as e:
print(e)
在上面的示例中,我们在比较方法中检查other
是否为MyClass
的实例,如果不是,则抛出TypeError
异常。这种方式可以有效地处理不可比较的对象。
三、如何自定义对象的排序顺序
在某些情况下,我们可能希望自定义对象的排序顺序。例如,我们希望根据某个属性的降序来排序对象。以下是一个示例:
class MyClass:
def __init__(self, value):
self.value = value
def __lt__(self, other):
return self.value > other.value # 使用大于号来实现降序排序
def __le__(self, other):
return self.value >= other.value
def __gt__(self, other):
return self.value < other.value
def __ge__(self, other):
return self.value <= other.value
def __eq__(self, other):
return self.value == other.value
def __ne__(self, other):
return self.value != other.value
示例用法
a = MyClass(10)
b = MyClass(20)
c = MyClass(15)
my_list = [a, b, c]
使用sorted函数进行排序
sorted_list = sorted(my_list)
输出排序后的结果
for obj in sorted_list:
print(obj.value)
在上面的示例中,我们通过在__lt__
方法中使用大于号来实现降序排序。这种方式可以根据需要自定义对象的排序顺序。
结论
通过本文,我们详细探讨了Python中比较对象大小的多种方法,包括使用内置比较运算符、实现特殊方法、使用functools模块的cmp_to_key函数、以及第三方库。我们还进一步讨论了如何实现多重属性的比较、处理不可比较的对象以及自定义对象的排序顺序。
在实际应用中,根据具体需求选择合适的方法可以使代码更加简洁和高效。希望本文能为您在Python中比较对象大小提供有用的指导和参考。
相关问答FAQs:
如何在Python中比较自定义对象的大小?
在Python中,比较自定义对象的大小通常需要实现特定的方法。可以通过定义__lt__
(小于)、__le__
(小于等于)、__gt__
(大于)、__ge__
(大于等于)、__eq__
(等于)和__ne__
(不等于)等特殊方法来实现对象间的比较。这些方法允许你根据对象的属性来定义其大小关系。例如,若你有一个表示学生的类,可以根据学生的成绩来比较两个学生对象。
Python中如何使用内置函数进行对象比较?
内置的sorted()
函数和min()
、max()
等函数可以直接用于自定义对象的比较。在这些函数调用时,可以通过设置key
参数来指定比较的关键属性。例如,如果你有一个包含多个学生对象的列表,可以使用sorted(students, key=lambda s: s.score)
来根据学生的成绩进行排序。
是否可以比较不同类型的Python对象?
在Python中,不同类型的对象通常不能直接进行比较。如果尝试比较不兼容的类型,Python会抛出TypeError
异常。如果需要比较不同类型的对象,建议实现自定义的比较逻辑,或者使用适当的方法将对象转换为相同类型后再进行比较。
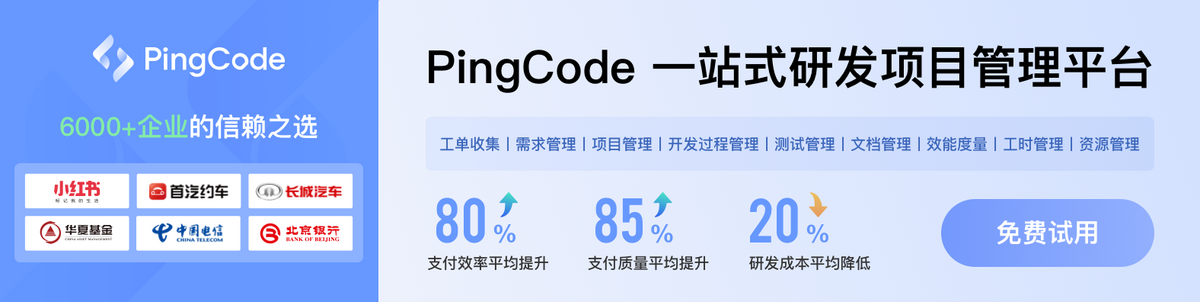