在Python中,字符串引用的方法有多种,包括单引号、双引号、三重引号、反斜杠转义字符等。其中,单引号和双引号是最常用的方式。三重引号用于多行字符串,反斜杠用于转义特殊字符。例如,使用双引号可以在字符串中包含单引号,而使用三重引号可以创建包含换行符的字符串。以下是详细介绍:
一、单引号和双引号
在Python中,字符串可以用单引号或者双引号引起来。两者的功能基本一致,可以互换使用。使用单引号和双引号的主要区别在于方便性。例如,当字符串中包含单引号时,使用双引号可以避免转义字符的使用,反之亦然。
# 使用单引号
string1 = 'Hello, World!'
print(string1)
使用双引号
string2 = "Hello, World!"
print(string2)
包含单引号的字符串
string3 = "It's a beautiful day."
print(string3)
包含双引号的字符串
string4 = 'He said, "Hello, World!"'
print(string4)
二、三重引号
三重引号(单引号或双引号的三重形式)用于定义多行字符串,或者包含单引号和双引号的复杂字符串。使用三重引号还可以保留字符串中的换行符和其他空白字符。
# 多行字符串
string5 = """This is a multi-line string.
It can span multiple lines.
It preserves the newline characters."""
print(string5)
包含单引号和双引号的字符串
string6 = '''He said, "It's a beautiful day."'''
print(string6)
三、转义字符
在字符串中使用反斜杠(\)作为转义字符,可以在字符串中包含特殊字符,例如换行符(\n)、制表符(\t)、反斜杠本身(\)等。
# 包含换行符的字符串
string7 = "This is a string with a newline.\nHere is the second line."
print(string7)
包含制表符的字符串
string8 = "This is a string with a tab.\tHere is the tab."
print(string8)
包含反斜杠的字符串
string9 = "This is a string with a backslash: \\"
print(string9)
四、原始字符串
原始字符串是在字符串前添加字母r或R,告诉Python不处理反斜杠作为转义字符。这对于正则表达式和Windows文件路径非常有用。
# 原始字符串
string10 = r"C:\Users\name\Documents\file.txt"
print(string10)
原始字符串中的正则表达式
import re
pattern = r"\b[A-Za-z]+\b"
text = "This is a sample text with several words."
matches = re.findall(pattern, text)
print(matches)
五、字符串格式化
字符串格式化可以通过多种方式实现,包括百分号(%)格式化、str.format()方法和f-字符串(格式化字符串字面值)。
# 百分号格式化
name = "John"
age = 30
string11 = "My name is %s and I am %d years old." % (name, age)
print(string11)
str.format()方法
string12 = "My name is {} and I am {} years old.".format(name, age)
print(string12)
f-字符串
string13 = f"My name is {name} and I am {age} years old."
print(string13)
六、字符串操作
Python提供了一系列内置方法用于操作字符串,例如连接、分割、替换、查找等。
1. 字符串连接
使用加号(+)可以将多个字符串连接在一起。
string14 = "Hello" + ", " + "World" + "!"
print(string14)
2. 字符串分割
使用split()方法可以将字符串按指定的分隔符拆分成一个列表。
string15 = "apple,banana,cherry"
fruits = string15.split(',')
print(fruits)
3. 字符串替换
使用replace()方法可以将字符串中的某些子串替换为新的子串。
string16 = "I like cats."
new_string = string16.replace("cats", "dogs")
print(new_string)
4. 字符串查找
使用find()方法可以查找子串在字符串中的位置,如果找不到返回-1。
string17 = "Hello, World!"
position = string17.find("World")
print(position)
5. 字符串大小写转换
Python提供了多种方法用于字符串的大小写转换,例如upper()、lower()、capitalize()、title()等。
string18 = "hello, world!"
print(string18.upper()) # 全部转换为大写
print(string18.lower()) # 全部转换为小写
print(string18.capitalize()) # 首字母大写
print(string18.title()) # 每个单词的首字母大写
七、字符串切片
字符串切片是一种强大的工具,用于从字符串中提取子字符串。切片使用[start:end:step]的格式,其中start是起始位置,end是结束位置,step是步长。
string19 = "Hello, World!"
print(string19[0:5]) # 输出 "Hello"
print(string19[7:12]) # 输出 "World"
print(string19[::2]) # 输出 "Hlo ol!"
print(string19[::-1]) # 输出 "!dlroW ,olleH" 反转字符串
八、字符串常用内置方法
Python内置了许多字符串方法,用于处理和操作字符串。以下是一些常用的字符串方法:
1. strip()方法
strip()方法用于去除字符串两端的空白字符(包括空格、换行符等)。
string20 = " Hello, World! "
print(string20.strip()) # 输出 "Hello, World!"
2. join()方法
join()方法用于将序列中的元素连接成一个新的字符串。
list_of_strings = ["apple", "banana", "cherry"]
string21 = ", ".join(list_of_strings)
print(string21) # 输出 "apple, banana, cherry"
3. count()方法
count()方法用于统计子串在字符串中出现的次数。
string22 = "Hello, World!"
count = string22.count("l")
print(count) # 输出 3
4. startswith()和endswith()方法
startswith()方法用于检查字符串是否以指定的子串开头,endswith()方法用于检查字符串是否以指定的子串结尾。
string23 = "Hello, World!"
print(string23.startswith("Hello")) # 输出 True
print(string23.endswith("World!")) # 输出 True
九、字符串编码和解码
在处理字符串时,有时需要在不同的编码之间转换。Python提供了encode()和decode()方法用于字符串的编码和解码。
# 编码
string24 = "Hello, World!"
encoded_string = string24.encode("utf-8")
print(encoded_string) # 输出 b'Hello, World!'
解码
decoded_string = encoded_string.decode("utf-8")
print(decoded_string) # 输出 "Hello, World!"
十、字符串比较
Python中的字符串可以直接用比较运算符(例如==、!=、<、>、<=、>=)进行比较。比较时,Python会逐个字符进行比较,直至得出结果。
string25 = "apple"
string26 = "banana"
print(string25 == string26) # 输出 False
print(string25 != string26) # 输出 True
print(string25 < string26) # 输出 True(按字典顺序比较)
print(string25 > string26) # 输出 False
十一、字符串的不可变性
Python中的字符串是不可变的,这意味着一旦创建,字符串的内容就不能被改变。任何对字符串的修改都会生成一个新的字符串对象。
string27 = "Hello"
尝试修改字符串(会导致错误)
string27[0] = "h"
使用拼接创建新字符串
string28 = "h" + string27[1:]
print(string28) # 输出 "hello"
十二、字符串的常见应用
字符串在Python中有着广泛的应用,以下是一些常见的字符串应用场景:
1. 处理文件路径
在处理文件路径时,字符串操作非常有用。使用os.path模块可以方便地进行路径操作。
import os
拼接文件路径
base_path = "/home/user"
file_name = "document.txt"
full_path = os.path.join(base_path, file_name)
print(full_path) # 输出 "/home/user/document.txt"
获取文件名和扩展名
file_path = "/home/user/document.txt"
file_name, file_extension = os.path.splitext(file_path)
print(file_name) # 输出 "/home/user/document"
print(file_extension) # 输出 ".txt"
2. 处理URL
在处理URL时,字符串操作也非常重要。使用urllib.parse模块可以方便地解析和构建URL。
from urllib.parse import urlparse, urlunparse, parse_qs, urlencode
解析URL
url = "https://www.example.com/path?name=John&age=30"
parsed_url = urlparse(url)
print(parsed_url.scheme) # 输出 "https"
print(parsed_url.netloc) # 输出 "www.example.com"
print(parsed_url.path) # 输出 "/path"
print(parsed_url.query) # 输出 "name=John&age=30"
构建URL
query_params = {"name": "John", "age": 30}
query_string = urlencode(query_params)
base_url = "https://www.example.com/path"
full_url = f"{base_url}?{query_string}"
print(full_url) # 输出 "https://www.example.com/path?name=John&age=30"
3. 处理JSON数据
在处理JSON数据时,字符串操作也是必不可少的。使用json模块可以方便地解析和生成JSON数据。
import json
解析JSON字符串
json_string = '{"name": "John", "age": 30, "city": "New York"}'
data = json.loads(json_string)
print(data) # 输出 {'name': 'John', 'age': 30, 'city': 'New York'}
生成JSON字符串
data = {"name": "John", "age": 30, "city": "New York"}
json_string = json.dumps(data)
print(json_string) # 输出 '{"name": "John", "age": 30, "city": "New York"}'
十三、正则表达式
正则表达式是一种强大的字符串匹配和操作工具。Python提供了re模块用于正则表达式操作。
import re
匹配单词
pattern = r"\b[A-Za-z]+\b"
text = "This is a sample text with several words."
matches = re.findall(pattern, text)
print(matches) # 输出 ['This', 'is', 'a', 'sample', 'text', 'with', 'several', 'words']
替换字符串
pattern = r"\d+"
text = "There are 123 apples and 456 oranges."
new_text = re.sub(pattern, "#", text)
print(new_text) # 输出 "There are # apples and # oranges."
十四、字符串与列表的转换
字符串和列表之间的转换是常见的操作。使用split()方法可以将字符串转换为列表,使用join()方法可以将列表转换为字符串。
# 字符串转列表
string29 = "apple,banana,cherry"
fruits = string29.split(',')
print(fruits) # 输出 ['apple', 'banana', 'cherry']
列表转字符串
fruits = ["apple", "banana", "cherry"]
string30 = ", ".join(fruits)
print(string30) # 输出 "apple, banana, cherry"
十五、字符串的长度
使用len()函数可以获取字符串的长度。
string31 = "Hello, World!"
length = len(string31)
print(length) # 输出 13
十六、字符串的遍历
可以使用for循环遍历字符串中的每一个字符。
string32 = "Hello"
for char in string32:
print(char)
十七、字符串的比较与排序
可以使用sorted()函数对字符串进行排序,或者使用字符串比较运算符对字符串进行比较。
# 字符串排序
string33 = "banana"
sorted_string = ''.join(sorted(string33))
print(sorted_string) # 输出 "aaabnn"
字符串比较
string34 = "apple"
string35 = "banana"
print(string34 < string35) # 输出 True
十八、字符串的查找与匹配
使用find()方法和index()方法可以在字符串中查找子串的位置。使用startswith()和endswith()方法可以检查字符串是否以指定的子串开头或结尾。
# 查找子串位置
string36 = "Hello, World!"
position = string36.find("World")
print(position) # 输出 7
检查字符串开头和结尾
string37 = "Hello, World!"
print(string37.startswith("Hello")) # 输出 True
print(string37.endswith("World!")) # 输出 True
十九、字符串的格式化
字符串格式化可以通过百分号(%)格式化、str.format()方法和f-字符串(格式化字符串字面值)实现。
# 百分号格式化
name = "John"
age = 30
string38 = "My name is %s and I am %d years old." % (name, age)
print(string38)
str.format()方法
string39 = "My name is {} and I am {} years old.".format(name, age)
print(string39)
f-字符串
string40 = f"My name is {name} and I am {age} years old."
print(string40)
二十、字符串编码与解码
在处理不同编码的字符串时,使用encode()和decode()方法进行编码和解码。
# 编码
string41 = "Hello, World!"
encoded_string = string41.encode("utf-8")
print(encoded_string) # 输出 b'Hello, World!'
解码
decoded_string = encoded_string.decode("utf-8")
print(decoded_string) # 输出 "Hello, World!"
通过以上内容的介绍,相信大家对Python字符串的引用和操作有了全面的了解。Python字符串是一个强大且灵活的数据类型,可以满足各种字符串处理需求。无论是简单的字符串连接,还是复杂的正则表达式匹配,Python都提供了丰富的内置方法和模块来帮助我们高效地处理字符串。
相关问答FAQs:
如何在Python中创建字符串?
在Python中,可以使用单引号(')或双引号(")来创建字符串。例如,str1 = 'Hello, World!'
或 str2 = "Hello, World!"
都是有效的字符串定义方式。
在Python字符串中如何插入变量的值?
可以使用f-string、str.format()方法或者百分号(%)格式化来插入变量的值。例如,使用f-string可以这样写:name = "Alice"; greeting = f"Hello, {name}!"
。这样,greeting
就会包含"Hello, Alice!"
。
Python字符串支持哪些常用操作?
Python字符串支持多种操作,包括连接(使用+
)、重复(使用*
)、切片(例如str[0:5]
获取字符串的前五个字符)以及查找(如str.find('a')
查找字符'a'的位置)。此外,还有许多内置方法可以用于字符串处理,如upper()
、lower()
、replace()
等。
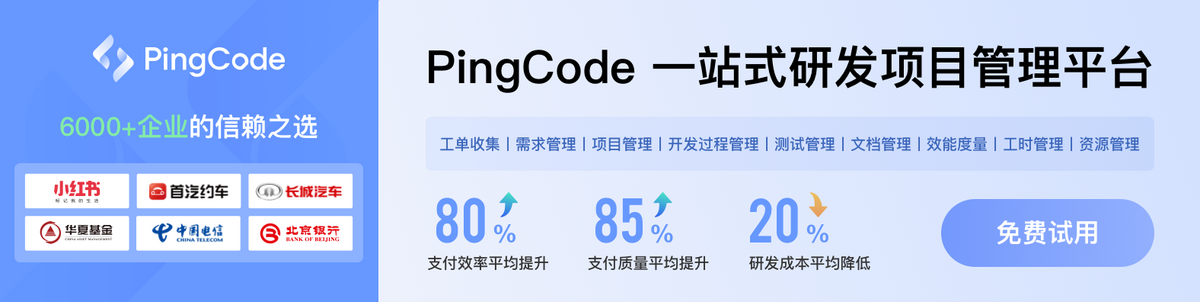