如何用Python提取句子主干,可以通过依存句法分析、词性标注、自然语言处理库等方法实现。 其中,依存句法分析是一种常用且有效的技术,通过解析句子的依存关系,可以提取句子的主干成分,如主语、谓语和宾语。 依存句法分析 是一种自然语言处理技术,它根据句子中的词语之间的依存关系,构建一个依存树,从中识别出句子的主干结构。下面将详细介绍如何用Python实现依存句法分析来提取句子主干。
一、安装和导入必要的库
在进行依存句法分析之前,我们需要安装一些必要的库,如spacy
、nltk
等。spacy
是一个强大的自然语言处理库,支持多种语言的依存句法分析。
pip install spacy
pip install nltk
python -m spacy download en_core_web_sm
安装好这些库后,我们可以在代码中导入它们:
import spacy
import nltk
from nltk.tokenize import sent_tokenize
二、加载语言模型
spacy
提供了多种语言模型,我们需要加载一个适用于我们分析的模型。这里以英文为例,加载 en_core_web_sm
模型:
nlp = spacy.load("en_core_web_sm")
三、进行句子分词
在进行句子主干提取之前,我们需要先对文本进行分句处理。可以使用 nltk
库中的 sent_tokenize
方法对文本进行分句:
text = "This is the first sentence. Here is another one."
sentences = sent_tokenize(text)
四、进行依存句法分析
对每个句子进行依存句法分析,提取出句子的主干成分。我们可以使用 spacy
的 doc
对象进行解析:
for sentence in sentences:
doc = nlp(sentence)
for token in doc:
# 打印词语及其依存关系
print(f"{token.text} ({token.dep_}) -> {token.head.text}")
五、提取句子主干
通过解析 spacy
的 doc
对象,我们可以提取出句子的主干成分。一般来说,句子的主干包括主语、谓语和宾语。我们可以根据依存关系来提取这些成分:
def extract_subject_verb_object(sentence):
doc = nlp(sentence)
subject = None
verb = None
obj = None
for token in doc:
if "subj" in token.dep_:
subject = token.text
if "VERB" in token.pos_:
verb = token.text
if "dobj" in token.dep_:
obj = token.text
return subject, verb, obj
for sentence in sentences:
subject, verb, obj = extract_subject_verb_object(sentence)
print(f"Subject: {subject}, Verb: {verb}, Object: {obj}")
六、进一步优化
在实际应用中,句子结构可能会更加复杂,可能包含从句、修饰语等成分。我们可以进一步优化提取算法,处理这些复杂情况。
1、处理复合句
对于包含多个子句的复合句,我们可以递归地对每个子句进行依存句法分析,提取出每个子句的主干成分。
def extract_main_clauses(doc):
main_clauses = []
for sent in doc.sents:
main_clauses.append(sent)
return main_clauses
text = "The cat, which was hungry, chased the mouse."
doc = nlp(text)
main_clauses = extract_main_clauses(doc)
for clause in main_clauses:
subject, verb, obj = extract_subject_verb_object(clause.text)
print(f"Clause: {clause.text}, Subject: {subject}, Verb: {verb}, Object: {obj}")
2、处理修饰语
句子中的修饰语(如形容词、副词)虽然不是句子的主干成分,但它们对句子的意义有重要影响。我们可以在提取主干成分的同时,记录下修饰语。
def extract_subject_verb_object_with_modifiers(sentence):
doc = nlp(sentence)
subject = None
verb = None
obj = None
modifiers = []
for token in doc:
if "subj" in token.dep_:
subject = token.text
if "VERB" in token.pos_:
verb = token.text
if "dobj" in token.dep_:
obj = token.text
if token.dep_ in ["amod", "advmod"]:
modifiers.append(token.text)
return subject, verb, obj, modifiers
for sentence in sentences:
subject, verb, obj, modifiers = extract_subject_verb_object_with_modifiers(sentence)
print(f"Subject: {subject}, Verb: {verb}, Object: {obj}, Modifiers: {modifiers}")
3、处理被动语态
在被动语态的句子中,主语和宾语的位置可能会发生变化。我们需要根据依存关系,正确识别出主语和宾语。
def extract_subject_verb_object_passive(sentence):
doc = nlp(sentence)
subject = None
verb = None
obj = None
for token in doc:
if "nsubjpass" in token.dep_: # 被动语态的主语
subject = token.text
if "VERB" in token.pos_:
verb = token.text
if "pobj" in token.dep_: # 被动语态的宾语
obj = token.text
return subject, verb, obj
for sentence in sentences:
subject, verb, obj = extract_subject_verb_object_passive(sentence)
print(f"Subject: {subject}, Verb: {verb}, Object: {obj}")
七、综合应用
通过以上方法,我们可以构建一个综合的句子主干提取器,能够处理多种复杂情况,并提取出句子的主干成分。
def extract_sentence_core(sentence):
doc = nlp(sentence)
subject = None
verb = None
obj = None
modifiers = []
for token in doc:
if "subj" in token.dep_ or "nsubjpass" in token.dep_:
subject = token.text
if "VERB" in token.pos_:
verb = token.text
if "dobj" in token.dep_ or "pobj" in token.dep_:
obj = token.text
if token.dep_ in ["amod", "advmod"]:
modifiers.append(token.text)
return subject, verb, obj, modifiers
text = "The big cat, which was very hungry, quickly chased the little mouse."
sentences = sent_tokenize(text)
for sentence in sentences:
subject, verb, obj, modifiers = extract_sentence_core(sentence)
print(f"Subject: {subject}, Verb: {verb}, Object: {obj}, Modifiers: {modifiers}")
通过这些步骤,我们可以用Python实现对句子的主干提取。这个过程不仅涉及到依存句法分析,还需要综合考虑句子的结构、修饰语和语态等因素。通过不断优化和调整算法,我们可以提取出更加准确和全面的句子主干。
相关问答FAQs:
提取句子主干的步骤是什么?
提取句子主干通常涉及几个步骤。首先,需要对句子进行分词和词性标注,以识别句子中的名词、动词、形容词等成分。接着,可以利用句法分析工具(如spaCy或NLTK)来构建句子的语法树,从而找出主语和谓语等主要成分。最后,通过分析句法树,可以提取出句子的主干部分,通常包括主语和谓语。
使用哪些Python库可以有效提取句子主干?
在Python中,有多个库可以帮助提取句子主干。spaCy是一个功能强大的自然语言处理库,支持高效的句法分析和词性标注。NLTK也是一个流行的库,提供了多种文本处理工具。此外,TextBlob和StanfordNLP等库也可以用于句子结构的分析和主干提取,用户可以根据自己的需求选择合适的工具。
提取句子主干的应用场景有哪些?
句子主干提取在多个领域中都具有重要应用。例如,在信息检索系统中,通过提取主干可以帮助改善搜索结果的相关性。在文本摘要生成方面,主干提取能够有效减少文本冗余,保留关键信息。此外,在自然语言理解和对话系统中,提取主干有助于实现更高效的语义理解和交互。
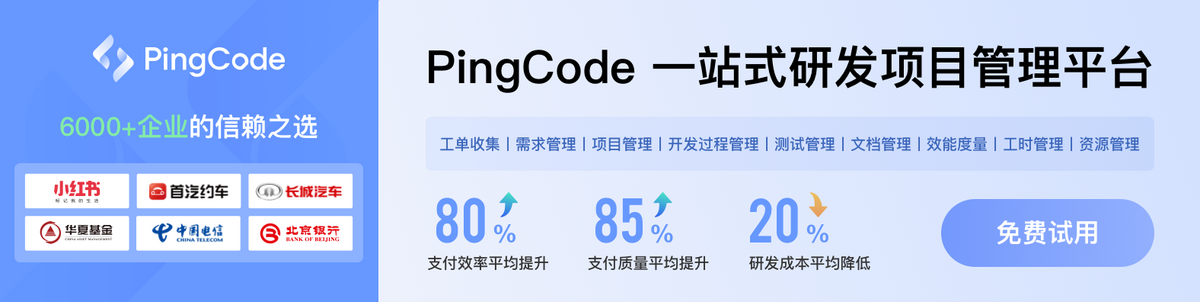