如何让Python显示多少行,可以通过使用内置函数、外部库、以及自定义函数等方式来实现。
使用内置函数、利用外部库、编写自定义函数、结合文件操作、统计字符串中的行数。这些方法可以帮助我们灵活地统计和显示Python代码或文件中的行数。
一、使用内置函数
Python内置函数可以非常方便地统计文件中的行数。我们可以使用open
函数打开文件并逐行读取,然后通过循环统计行数。
def count_lines(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return len(lines)
file_path = 'example.txt'
line_count = count_lines(file_path)
print(f'The file {file_path} has {line_count} lines.')
在这个例子中,open
函数用于打开文件,readlines
方法将文件的所有行读入一个列表中,然后使用len
函数计算列表的长度,即文件中的行数。
二、利用外部库
除了使用内置函数,我们还可以借助外部库来统计行数。一些外部库提供了更高级的功能,可以方便地处理大文件或特定格式的文件。例如,pandas
库可以非常方便地处理CSV文件。
import pandas as pd
def count_csv_lines(file_path):
df = pd.read_csv(file_path)
return len(df)
file_path = 'example.csv'
line_count = count_csv_lines(file_path)
print(f'The CSV file {file_path} has {line_count} lines.')
在这个例子中,pandas
库的read_csv
函数用于读取CSV文件,并将其转换为DataFrame对象,然后使用len
函数计算DataFrame对象的长度,即文件中的行数。
三、编写自定义函数
有时候我们需要更灵活的解决方案,例如统计字符串中的行数或者处理不同编码的文件。这时可以编写自定义函数来实现。
def count_string_lines(string):
lines = string.split('\n')
return len(lines)
text = """This is a sample text.
It has multiple lines.
Each line is separated by a newline character."""
line_count = count_string_lines(text)
print(f'The text has {line_count} lines.')
在这个例子中,自定义函数count_string_lines
使用字符串的split
方法将文本按换行符分割成多个行,然后使用len
函数计算行数。
四、结合文件操作
结合文件操作我们可以实现更复杂的行数统计功能,例如统计代码文件中的有效代码行数(排除空行和注释)。
def count_code_lines(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
code_lines = [line for line in lines if line.strip() and not line.strip().startswith('#')]
return len(code_lines)
file_path = 'example.py'
line_count = count_code_lines(file_path)
print(f'The Python file {file_path} has {line_count} code lines.')
在这个例子中,count_code_lines
函数读取文件内容后,通过列表推导式过滤掉空行和注释行,然后计算有效代码行数。
五、统计字符串中的行数
除了文件操作,我们还可以统计字符串中的行数,这在处理动态生成的文本时非常有用。
def count_lines_in_string(text):
lines = text.splitlines()
return len(lines)
text = """First line
Second line
Third line
"""
line_count = count_lines_in_string(text)
print(f'The text has {line_count} lines.')
在这个例子中,splitlines
方法用于将文本按行分割成列表,然后使用len
函数计算行数。
六、处理大文件
在处理大文件时,逐行读取并统计行数可以避免占用过多内存。以下是一个逐行读取大文件并统计行数的例子:
def count_large_file_lines(file_path):
line_count = 0
with open(file_path, 'r') as file:
for line in file:
line_count += 1
return line_count
file_path = 'large_file.txt'
line_count = count_large_file_lines(file_path)
print(f'The large file {file_path} has {line_count} lines.')
在这个例子中,count_large_file_lines
函数逐行读取文件内容,并在循环中统计行数。这种方法可以有效地处理大文件而不会占用大量内存。
七、多线程处理
在一些特殊场景下,我们可能需要更高效的行数统计方法,例如多线程处理。以下是一个使用多线程处理大文件并统计行数的例子:
import threading
class LineCounter(threading.Thread):
def __init__(self, file_path):
threading.Thread.__init__(self)
self.file_path = file_path
self.line_count = 0
def run(self):
with open(self.file_path, 'r') as file:
for line in file:
self.line_count += 1
file_path = 'large_file.txt'
thread = LineCounter(file_path)
thread.start()
thread.join()
print(f'The large file {file_path} has {thread.line_count} lines.')
在这个例子中,LineCounter
类继承自threading.Thread
,在run
方法中实现逐行读取文件并统计行数。我们可以创建LineCounter
对象并启动线程,然后等待线程完成统计任务。
八、处理不同编码的文件
在处理不同编码的文件时,我们需要指定文件的编码格式,否则可能会出现解码错误。以下是一个处理不同编码文件并统计行数的例子:
def count_encoded_file_lines(file_path, encoding):
with open(file_path, 'r', encoding=encoding) as file:
lines = file.readlines()
return len(lines)
file_path = 'example_utf8.txt'
encoding = 'utf-8'
line_count = count_encoded_file_lines(file_path, encoding)
print(f'The file {file_path} has {line_count} lines.')
在这个例子中,count_encoded_file_lines
函数使用open
函数时指定了文件的编码格式,确保能够正确读取文件内容并统计行数。
九、处理压缩文件
在处理压缩文件时,我们需要先解压文件内容,然后再统计行数。以下是一个处理压缩文件并统计行数的例子:
import gzip
def count_gzip_file_lines(file_path):
with gzip.open(file_path, 'rt') as file:
lines = file.readlines()
return len(lines)
file_path = 'example.gz'
line_count = count_gzip_file_lines(file_path)
print(f'The gzip file {file_path} has {line_count} lines.')
在这个例子中,count_gzip_file_lines
函数使用gzip.open
函数解压文件内容并读取文件行数。
十、处理特定格式的文件
在处理特定格式的文件时,我们可以使用相应的库来读取文件并统计行数。例如,处理Excel文件可以使用openpyxl
库:
import openpyxl
def count_excel_lines(file_path, sheet_name):
workbook = openpyxl.load_workbook(file_path)
sheet = workbook[sheet_name]
return sheet.max_row
file_path = 'example.xlsx'
sheet_name = 'Sheet1'
line_count = count_excel_lines(file_path, sheet_name)
print(f'The Excel file {file_path} has {line_count} lines in sheet {sheet_name}.')
在这个例子中,count_excel_lines
函数使用openpyxl
库读取Excel文件,并获取指定工作表中的最大行数。
十一、总结
通过以上多种方法,我们可以灵活地统计和显示Python代码或文件中的行数。使用内置函数、利用外部库、编写自定义函数、结合文件操作、统计字符串中的行数等方法可以帮助我们解决不同场景下的行数统计问题。在实际应用中,选择合适的方法可以提高代码的效率和可读性。
相关问答FAQs:
如何在Python中设置输出的行数限制?
在Python中,您可以使用pandas
库来控制输出的行数。通过设置pd.set_option('display.max_rows', n)
,您可以指定要显示的最大行数,其中n
是您希望显示的行数。例如,如果您希望显示前20行数据,可以使用pd.set_option('display.max_rows', 20)
。
Python中如何查看数据框的行数?
要查看数据框的行数,可以使用len(dataframe)
或dataframe.shape[0]
来获取行的数量。这两种方法都能有效地告诉您数据框中包含多少行。
在Python中如何处理输出过多行的问题?
当输出的行数过多时,可以使用head()
和tail()
方法来查看数据框的前几行或后几行。例如,dataframe.head(10)
将显示前10行数据,而dataframe.tail(10)
将显示最后10行。这样可以避免在控制台中输出过多数据,提高可读性。
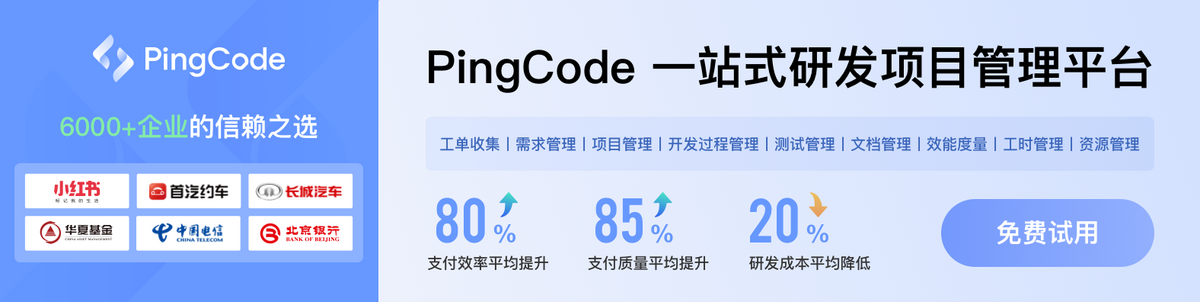