在Python中输入DOS命令可以通过os.system()
、subprocess.run()
、subprocess.Popen()
等方式实现。其中subprocess
模块是较为推荐的方法,因为它提供了更多的功能和更强的控制能力。 以下将详细介绍如何使用这些方法来输入DOS命令。
一、使用os.system()
1、基本用法
os.system()
是最简单的方式之一,它可以直接执行一个字符串形式的命令。虽然简单,但是它的功能有限,比如无法获取命令的输出。
import os
执行简单的DOS命令
os.system('dir')
2、局限性
os.system()
的局限性在于它无法捕获命令的输出,同时它的安全性较差,容易受到命令注入攻击。因此在需要捕获输出和确保安全的情况下,不建议使用这种方法。
二、使用subprocess.run()
1、基本用法
subprocess.run()
是Python 3.5引入的一个函数,用于替代os.system()
,它提供了更强的功能和更好的控制能力。
import subprocess
执行简单的DOS命令,并获取返回码
result = subprocess.run(['dir'], shell=True)
print(result.returncode)
2、捕获输出
通过设置capture_output=True
可以捕获命令的标准输出和标准错误。
import subprocess
执行命令并捕获输出
result = subprocess.run(['dir'], shell=True, capture_output=True, text=True)
print(result.stdout)
print(result.stderr)
3、处理错误
subprocess.run()
可以通过check=True
参数来自动抛出异常,从而简化错误处理。
import subprocess
try:
result = subprocess.run(['dir'], shell=True, check=True, capture_output=True, text=True)
print(result.stdout)
except subprocess.CalledProcessError as e:
print(f"Command failed with error: {e}")
三、使用subprocess.Popen()
1、基本用法
subprocess.Popen()
提供了更强的灵活性,可以在执行命令的同时进行更多的控制操作。
import subprocess
使用Popen执行命令
process = subprocess.Popen(['dir'], shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print(stdout)
print(stderr)
2、实时输出
通过subprocess.Popen()
可以实现命令的实时输出,这在一些长时间运行的命令中非常有用。
import subprocess
实时输出命令执行过程
process = subprocess.Popen(['ping', 'www.google.com', '-n', '5'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
while True:
output = process.stdout.readline()
if output == '' and process.poll() is not None:
break
if output:
print(output.strip())
3、输入输出重定向
subprocess.Popen()
允许将命令的输入输出重定向到文件或其他流中。
import subprocess
with open('output.txt', 'w') as f:
process = subprocess.Popen(['dir'], shell=True, stdout=f, stderr=subprocess.PIPE, text=True)
_, stderr = process.communicate()
四、推荐使用的方式
在大多数情况下,推荐使用subprocess.run()
,因为它提供了足够的功能和简单易用的接口。如果需要更多的控制或者实时处理命令输出,可以选择使用subprocess.Popen()
。
五、注意事项
1、Shell注入攻击
在使用shell=True
时要特别小心,避免使用用户输入直接构造命令字符串,以防止命令注入攻击。
import subprocess
可能存在安全风险的用法
user_input = "some_command"
subprocess.run(user_input, shell=True)
安全的用法
subprocess.run(['some_command'], shell=True)
2、字符编码
在处理命令输出时,需要注意字符编码问题。可以通过text=True
参数将输出自动解码为字符串形式。
import subprocess
result = subprocess.run(['dir'], shell=True, capture_output=True, text=True)
print(result.stdout)
3、跨平台兼容
在编写跨平台的Python代码时,尽量避免使用特定于某个操作系统的命令。可以使用Python标准库中的函数来替代,例如使用os.listdir()
代替dir
。
import os
使用Python标准库函数
files = os.listdir('.')
for file in files:
print(file)
六、示例代码
以下是一个完整的示例代码,展示了如何在Python中使用subprocess.run()
和subprocess.Popen()
来执行DOS命令,并处理输出和错误。
import subprocess
def run_command(command):
try:
result = subprocess.run(command, shell=True, check=True, capture_output=True, text=True)
print("Command succeeded with output:")
print(result.stdout)
except subprocess.CalledProcessError as e:
print(f"Command failed with error: {e}")
print(e.stderr)
def run_command_with_realtime_output(command):
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
while True:
output = process.stdout.readline()
if output == '' and process.poll() is not None:
break
if output:
print(output.strip())
stderr = process.stderr.read()
if stderr:
print("Command failed with error:")
print(stderr)
执行命令并捕获输出
run_command('dir')
实时输出命令执行过程
run_command_with_realtime_output('ping www.google.com -n 5')
总结
通过os.system()
、subprocess.run()
和subprocess.Popen()
等方法可以在Python中执行DOS命令。推荐使用subprocess.run()
来执行简单的命令,使用subprocess.Popen()
来进行更复杂的控制操作。在实际应用中,要特别注意安全性,避免使用不安全的字符串拼接方式构造命令,并处理好字符编码和跨平台兼容性问题。
相关问答FAQs:
如何在Python中执行DOS命令?
在Python中,可以使用os
模块或subprocess
模块来执行DOS命令。os.system()
方法可以直接执行命令,而subprocess.run()
提供了更强大的功能,如获取命令输出和错误处理。以下是一个简单的示例:
import os
os.system('dir') # 使用os模块执行DOS命令
import subprocess
result = subprocess.run(['dir'], shell=True, capture_output=True, text=True)
print(result.stdout) # 使用subprocess模块执行DOS命令并获取输出
在Python中执行DOS命令时如何处理错误?
使用subprocess
模块时,可以通过检查returncode
属性来判断命令是否成功执行。如果returncode
为0,则表示命令成功执行;如果为非零值,则表示出现了错误。例如:
result = subprocess.run(['your_command'], shell=True)
if result.returncode != 0:
print("命令执行失败:", result.stderr)
这种方式能够帮助开发者有效地捕获和处理错误信息。
是否可以在Python脚本中使用用户输入的DOS命令?
是的,可以使用input()
函数获取用户输入的命令,并通过subprocess
模块执行。为了安全起见,建议对用户输入进行验证和清理,以防止注入攻击。例如:
user_command = input("请输入要执行的DOS命令: ")
# 在这里添加验证和清理用户输入的逻辑
result = subprocess.run(user_command, shell=True)
确保用户输入是安全的,能够防止潜在的安全风险。
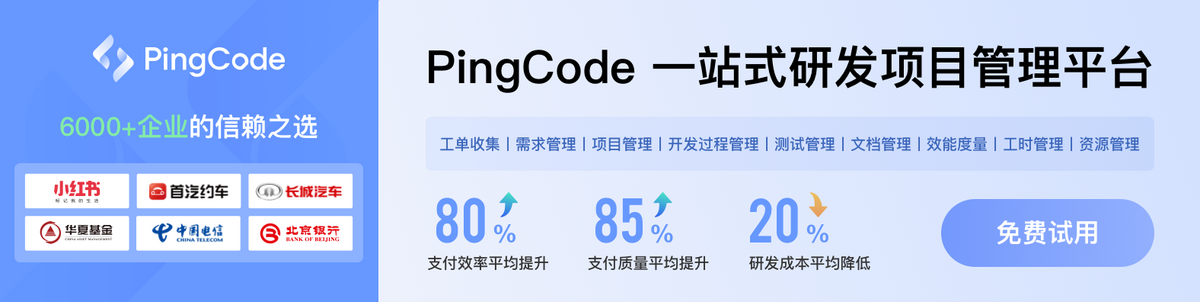