Python识别对象类型的方法包括使用type()
函数、isinstance()
函数、以及__class__
属性。 在本文中,我们将详细介绍这几种方法并探讨它们的应用场景和使用技巧。
一、type()
函数
type()
函数是Python内置的一个函数,可以用来返回对象的类型。其语法为:
type(object)
使用示例:
a = 5
print(type(a)) # <class 'int'>
b = "hello"
print(type(b)) # <class 'str'>
type()
函数的输出是对象的类型,可以通过它来判断某个对象是否属于某个特定类型,例如:
if type(a) is int:
print("a is an integer")
优点和局限性:
优点: type()
函数简单易用,适合在检查对象类型时使用。
局限性: 它不能处理继承关系,无法判断一个对象是否是某个类的子类。
二、isinstance()
函数
isinstance()
函数用于判断对象是否是已知的类型,或者是该类型的子类。其语法为:
isinstance(object, classinfo)
其中,classinfo
可以是一个类型或类型的元组。
使用示例:
class Animal:
pass
class Dog(Animal):
pass
d = Dog()
print(isinstance(d, Dog)) # True
print(isinstance(d, Animal)) # True
print(isinstance(d, int)) # False
优点和局限性:
优点: isinstance()
函数不仅可以检查对象的直接类型,还可以检查对象是否是某个类型的子类,适用于多态场景。
局限性: 需要明确指定类型或类型的元组,不够灵活。
三、__class__
属性
每个Python对象都有一个__class__
属性,可以通过它来获取对象的类型。其语法为:
object.__class__
使用示例:
a = 5
print(a.__class__) # <class 'int'>
b = "hello"
print(b.__class__) # <class 'str'>
优点和局限性:
优点: __class__
属性直观,可以直接访问对象的类型。
局限性: 不如isinstance()
函数灵活,无法处理继承关系。
四、实际应用场景
1. 类型检查
在某些场景下,我们需要对函数输入参数进行类型检查,以确保传入的参数符合预期。这时可以使用isinstance()
函数。例如:
def process_data(data):
if not isinstance(data, list):
raise TypeError("Input data must be a list")
# 处理数据的逻辑
2. 多态处理
在面向对象编程中,经常需要处理多态问题。可以使用isinstance()
函数来判断对象类型,并根据类型执行不同的操作。例如:
class Shape:
def draw(self):
pass
class Circle(Shape):
def draw(self):
print("Drawing a circle")
class Square(Shape):
def draw(self):
print("Drawing a square")
def draw_shape(shape):
if isinstance(shape, Shape):
shape.draw()
else:
print("Unknown shape")
circle = Circle()
square = Square()
draw_shape(circle) # Drawing a circle
draw_shape(square) # Drawing a square
3. 动态类型识别
在某些场景下,我们需要根据对象的类型动态执行不同的逻辑,可以通过type()
函数或__class__
属性来实现。例如:
def process_item(item):
item_type = type(item)
if item_type is int:
print("Processing an integer")
elif item_type is str:
print("Processing a string")
else:
print("Unknown item type")
process_item(10) # Processing an integer
process_item("hello") # Processing a string
五、总结
在Python中,识别对象类型的方法主要包括type()
函数、isinstance()
函数和__class__
属性。它们各有优缺点,适用于不同的应用场景。type()
函数简单易用,但不能处理继承关系;isinstance()
函数灵活,能处理多态问题;__class__
属性直观,但不如isinstance()
函数灵活。
在实际开发中,可以根据具体需求选择合适的方法进行对象类型的识别,以确保代码的正确性和健壮性。
相关问答FAQs:
如何在Python中判断一个对象是否是特定类型?
在Python中,可以使用内置函数isinstance()
来判断一个对象是否是某个特定类型。该函数接收两个参数,第一个是要检查的对象,第二个是类型或类型元组。如果对象是指定类型的实例,isinstance()
将返回True;否则返回False。示例代码如下:
x = 10
print(isinstance(x, int)) # 输出: True
Python中有哪些方法可以获取对象的类型信息?
Python提供了多种方法来获取对象的类型信息。最常用的方式是使用内置函数type()
,它会返回对象的类型。例如:
y = "Hello"
print(type(y)) # 输出: <class 'str'>
此外,__class__
属性也可以用来获取对象的类信息,例如:
print(y.__class__) # 输出: <class 'str'>
在Python中,如何处理不同类型的对象以实现多态性?
多态性是面向对象编程的一个重要特性。在Python中,可以通过方法重写和接口实现多态。通过定义相同名称的方法,不同的类可以实现不同的行为。例如:
class Animal:
def sound(self):
pass
class Dog(Animal):
def sound(self):
return "Woof!"
class Cat(Animal):
def sound(self):
return "Meow!"
def make_sound(animal):
print(animal.sound())
dog = Dog()
cat = Cat()
make_sound(dog) # 输出: Woof!
make_sound(cat) # 输出: Meow!
这样的设计允许你用相同的方式处理不同类型的对象,增强了代码的灵活性和可扩展性。
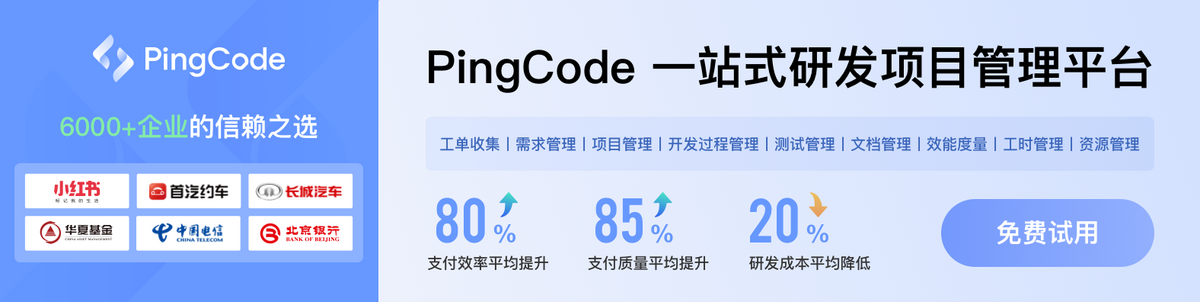