利用Python抓取网页信息主要通过以下几个步骤:选择合适的工具、发送请求、解析HTML、处理数据、保存结果。 其中,选择合适的工具是抓取网页信息的基础步骤,主要包括选择请求库和解析库。Python中常用的请求库有requests
,解析库有BeautifulSoup
和lxml
。我们可以通过requests
库发送HTTP请求获取网页内容,再通过BeautifulSoup
或lxml
库解析HTML,从中提取需要的信息。下面将详细介绍这几个步骤。
一、选择合适的工具
1、请求库:requests
requests
库是Python中最常用的HTTP库,它可以非常简便地发送HTTP请求并获取响应。使用requests
库可以轻松地进行各种HTTP操作,如GET、POST、PUT、DELETE等。
import requests
response = requests.get('https://example.com')
print(response.text)
2、解析库:BeautifulSoup和lxml
BeautifulSoup
和lxml
是Python中常用的HTML解析库,它们可以将复杂的HTML文档转换成便于操作的对象,方便我们提取其中的信息。
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title)
二、发送请求
通过requests
库发送HTTP请求,可以获取网页的内容。请求的类型有很多种,最常用的是GET请求和POST请求。
1、GET请求
GET请求用于从服务器获取数据。可以通过requests.get
方法发送GET请求。
response = requests.get('https://example.com')
print(response.status_code)
print(response.text)
2、POST请求
POST请求用于向服务器提交数据。可以通过requests.post
方法发送POST请求。
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://example.com', data=data)
print(response.status_code)
print(response.text)
三、解析HTML
获取到网页内容后,需要解析HTML文档,提取出我们需要的信息。可以使用BeautifulSoup
或lxml
库进行解析。
1、BeautifulSoup解析HTML
BeautifulSoup
可以将HTML文档转换成便于操作的对象,常用的操作包括查找节点、获取属性、提取文本等。
soup = BeautifulSoup(response.text, 'html.parser')
查找节点
title = soup.find('title')
print(title.text)
获取属性
link = soup.find('a')
print(link['href'])
提取文本
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.text)
2、lxml解析HTML
lxml
库功能强大,解析速度快,适用于处理复杂的HTML文档。可以使用lxml
库中的html
模块进行解析。
from lxml import html
tree = html.fromstring(response.text)
查找节点
title = tree.xpath('//title/text()')
print(title)
获取属性
links = tree.xpath('//a/@href')
print(links)
提取文本
paragraphs = tree.xpath('//p/text()')
for p in paragraphs:
print(p)
四、处理数据
解析出需要的信息后,可以进行进一步的数据处理,包括数据清洗、数据转换、数据存储等。
1、数据清洗
数据清洗是指对原始数据进行处理,使其符合要求。常见的数据清洗操作包括去除空格、去重、格式转换等。
cleaned_data = [p.strip() for p in paragraphs if p.strip()]
print(cleaned_data)
2、数据转换
数据转换是指将数据从一种形式转换为另一种形式。常见的数据转换操作包括类型转换、格式转换等。
import json
data_dict = {'title': title, 'links': links, 'paragraphs': cleaned_data}
json_data = json.dumps(data_dict, indent=4)
print(json_data)
五、保存结果
处理好的数据可以保存到文件、数据库等。常见的保存方式包括保存为文本文件、CSV文件、JSON文件等。
1、保存为文本文件
可以使用Python的内置文件操作函数将数据保存为文本文件。
with open('data.txt', 'w') as file:
for p in cleaned_data:
file.write(p + '\n')
2、保存为CSV文件
可以使用csv
库将数据保存为CSV文件。
import csv
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Title', 'Links', 'Paragraphs'])
writer.writerow([title, links, cleaned_data])
3、保存为JSON文件
可以使用json
库将数据保存为JSON文件。
with open('data.json', 'w') as file:
json.dump(data_dict, file, indent=4)
六、综合示例
下面是一个综合示例,通过上述步骤抓取网页信息并保存为JSON文件。
import requests
from bs4 import BeautifulSoup
import json
def fetch_webpage(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
return None
def parse_html(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.find('title').text
links = [a['href'] for a in soup.find_all('a', href=True)]
paragraphs = [p.text.strip() for p in soup.find_all('p') if p.text.strip()]
return {'title': title, 'links': links, 'paragraphs': paragraphs}
def save_to_json(data, filename):
with open(filename, 'w') as file:
json.dump(data, file, indent=4)
url = 'https://example.com'
html_content = fetch_webpage(url)
if html_content:
data = parse_html(html_content)
save_to_json(data, 'data.json')
print('Data saved to data.json')
else:
print('Failed to fetch webpage')
七、处理复杂网页
有时候,我们需要处理一些复杂的网页信息抓取任务,例如处理动态加载的内容、模拟用户行为等。此时,可以使用一些更强大的工具,如Selenium
、Scrapy
等。
1、处理动态加载内容
对于动态加载的内容,可以使用Selenium
库模拟浏览器行为,加载完整的网页内容。
from selenium import webdriver
设置浏览器驱动
driver = webdriver.Chrome()
访问网页
driver.get('https://example.com')
获取网页内容
html_content = driver.page_source
关闭浏览器
driver.quit()
解析HTML
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.title.text)
2、使用Scrapy进行大规模数据抓取
Scrapy
是一个强大的爬虫框架,适用于大规模数据抓取任务。使用Scrapy
可以方便地进行多线程抓取、数据清洗、数据存储等操作。
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
title = response.xpath('//title/text()').get()
links = response.xpath('//a/@href').getall()
paragraphs = response.xpath('//p/text()').getall()
paragraphs = [p.strip() for p in paragraphs if p.strip()]
yield {'title': title, 'links': links, 'paragraphs': paragraphs}
3、处理反爬机制
一些网站会采取反爬机制,阻止自动化抓取。常见的反爬机制包括IP封禁、验证码、JavaScript混淆等。可以通过以下几种方式应对反爬机制:
- 使用代理IP:通过使用代理IP,可以避免被服务器封禁。
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get('https://example.com', proxies=proxies)
- 模拟浏览器行为:通过设置请求头,模拟浏览器行为,避免被识别为爬虫。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get('https://example.com', headers=headers)
- 处理验证码:对于需要处理验证码的网站,可以使用OCR技术识别验证码,或者通过人工打码平台解决验证码问题。
from PIL import Image
import pytesseract
加载验证码图片
captcha_image = Image.open('captcha.png')
识别验证码
captcha_text = pytesseract.image_to_string(captcha_image)
print(captcha_text)
八、总结
通过以上步骤,我们可以使用Python方便地抓取网页信息,并对数据进行处理和保存。具体步骤包括选择合适的工具、发送请求、解析HTML、处理数据、保存结果等。在实际应用中,还需要根据具体情况,处理动态加载内容、应对反爬机制等。希望通过本文的介绍,能够帮助大家更好地利用Python进行网页信息抓取。
相关问答FAQs:
如何选择合适的库进行网页抓取?
在Python中,有多种库可用于网页抓取。常用的有Requests和BeautifulSoup。Requests库用于发送网络请求,获取网页内容,而BeautifulSoup则用于解析HTML代码,提取所需的信息。可以根据需要选择合适的库,甚至结合使用以达到最佳效果。
网页抓取的法律和道德问题是什么?
在进行网页抓取时,务必遵循网站的使用条款和法律规定。部分网站可能禁止抓取行为,因此在抓取数据前最好查看网站的robots.txt文件。此外,尊重网站的流量和服务器负担,避免频繁请求,以免对其造成影响。
如何处理网页抓取中的数据存储?
抓取到的数据可以存储在多种格式中,常见的有CSV、JSON和数据库。根据数据量和后续需求选择合适的存储方式。例如,较小的数据集可使用CSV文件,而大量数据则建议存储到数据库中,以便进行更复杂的查询和分析。
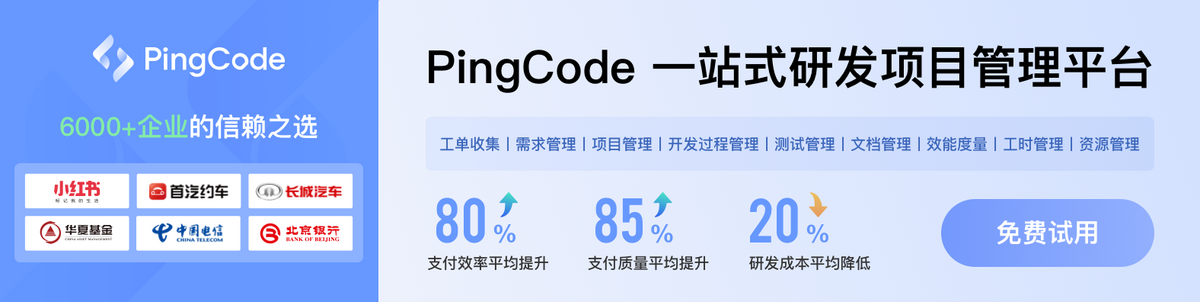