Python可以通过多种方式返回网页数据,例如使用requests库、urllib库、BeautifulSoup库等。本文将重点介绍如何使用requests库获取网页数据,并详细介绍如何使用BeautifulSoup库进行解析。
一、使用requests库获取网页数据
requests库是一个简洁、易用的HTTP库,可以轻松地发送HTTP请求。以下是使用requests库获取网页数据的步骤:
import requests
url = 'http://example.com'
response = requests.get(url)
print(response.text)
步骤解释:
- 导入requests库。
- 设置目标URL。
- 使用requests.get()方法发送HTTP GET请求。
- 使用response.text获取网页的HTML内容。
requests库有很多高级功能,例如设置请求头、处理Cookies、发送POST请求等。下面是一个更详细的示例:
import requests
url = 'http://example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
print(response.text)
二、使用BeautifulSoup解析网页数据
BeautifulSoup是一个用于解析HTML和XML文档的库,它能将复杂的HTML文档转换成一个简单的树形结构,更方便地提取数据。以下是使用BeautifulSoup库解析网页数据的步骤:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify())
步骤解释:
- 导入BeautifulSoup和requests库。
- 设置目标URL并发送HTTP GET请求。
- 使用BeautifulSoup解析HTML文档。
- 使用soup.prettify()方法格式化输出HTML内容。
三、实例:获取并解析具体网页数据
接下来,我们将通过一个具体的实例,获取并解析网页数据。例如,获取并解析一个新闻网站的标题和链接。
步骤1:获取网页HTML内容
import requests
url = 'https://news.ycombinator.com/'
response = requests.get(url)
html_content = response.text
步骤2:使用BeautifulSoup解析HTML内容
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
步骤3:提取新闻标题和链接
titles = soup.find_all('a', class_='storylink')
for title in titles:
print(title.text, title['href'])
四、处理动态网页数据
有时候网页内容是通过JavaScript动态加载的,requests库和BeautifulSoup库无法直接获取这些内容。对于这种情况,可以使用Selenium库来处理。Selenium是一个用于自动化Web浏览器的工具,可以模拟用户操作,获取动态加载的数据。
以下是使用Selenium获取动态网页数据的示例:
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
time.sleep(5) # 等待页面加载完成
html_content = driver.page_source
driver.quit()
步骤解释:
- 导入Selenium相关库。
- 设置目标URL并启动Web浏览器。
- 等待页面加载完成。
- 获取页面源代码并关闭浏览器。
五、处理分页数据
很多网站的数据是分页显示的,使用requests库和BeautifulSoup库可以逐页获取数据。以下是处理分页数据的示例:
import requests
from bs4 import BeautifulSoup
base_url = 'http://example.com/page='
page = 1
while True:
url = base_url + str(page)
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 提取数据
data = soup.find_all('div', class_='data-item')
if not data:
break # 如果没有数据,结束循环
for item in data:
print(item.text)
page += 1
步骤解释:
- 设置基础URL和初始页码。
- 循环发送HTTP GET请求,获取每一页的HTML内容。
- 使用BeautifulSoup解析HTML内容,提取数据。
- 如果没有数据,结束循环。
六、处理表单数据
有时候我们需要提交表单来获取网页数据,requests库也可以处理这种情况。以下是处理表单数据的示例:
import requests
url = 'http://example.com/form'
form_data = {
'username': 'your_username',
'password': 'your_password'
}
response = requests.post(url, data=form_data)
print(response.text)
步骤解释:
- 设置目标URL和表单数据。
- 使用requests.post()方法发送HTTP POST请求。
- 获取响应内容。
七、处理Cookies
requests库可以轻松地处理Cookies。以下是处理Cookies的示例:
import requests
url = 'http://example.com'
session = requests.Session()
response = session.get(url)
cookies = session.cookies.get_dict()
print(cookies)
使用Cookies发送请求
response = session.get(url, cookies=cookies)
print(response.text)
步骤解释:
- 创建一个Session对象。
- 发送HTTP GET请求并获取Cookies。
- 使用Cookies发送请求。
八、处理文件下载
requests库也可以处理文件下载。以下是处理文件下载的示例:
import requests
url = 'http://example.com/file.zip'
response = requests.get(url)
with open('file.zip', 'wb') as file:
file.write(response.content)
步骤解释:
- 设置目标URL。
- 发送HTTP GET请求并获取文件内容。
- 将文件内容写入本地文件。
九、处理异步请求
有时候我们需要处理异步请求,aiohttp库可以帮助我们实现这一点。以下是处理异步请求的示例:
import aiohttp
import asyncio
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
url = 'http://example.com'
html_content = asyncio.run(fetch(url))
print(html_content)
步骤解释:
- 导入aiohttp和asyncio库。
- 定义一个异步函数fetch(),发送HTTP GET请求并获取响应内容。
- 使用asyncio.run()运行异步函数。
十、总结
通过本文的介绍,我们详细了解了如何使用requests库获取网页数据,以及如何使用BeautifulSoup库解析HTML内容。此外,我们还介绍了处理动态网页数据、分页数据、表单数据、Cookies、文件下载和异步请求的方法。希望这些内容能帮助你更好地获取和解析网页数据。
相关问答FAQs:
如何使用Python从网页获取数据?
要从网页获取数据,您可以使用Python的库如requests
和BeautifulSoup
。首先,通过requests.get()
方法获取网页内容,然后利用BeautifulSoup
解析HTML结构,从中提取所需的数据。例如,您可以从特定的HTML标签中提取文本或链接。
使用Python进行网页抓取的最佳实践是什么?
在进行网页抓取时,遵循网站的robots.txt
文件,确保您的请求不会对服务器造成负担。此外,设置合理的请求间隔,避免频繁请求同一网页。使用异常处理来管理网络问题和解析错误,确保程序的稳定性和可靠性。
Python可以处理哪些类型的网页数据?
Python能够处理多种类型的网页数据,包括文本、图像、视频和表格等。通过使用不同的库,您可以轻松提取和处理这些数据。例如,使用Pandas
库可以将提取的表格数据转化为DataFrame格式,便于进一步分析和处理。
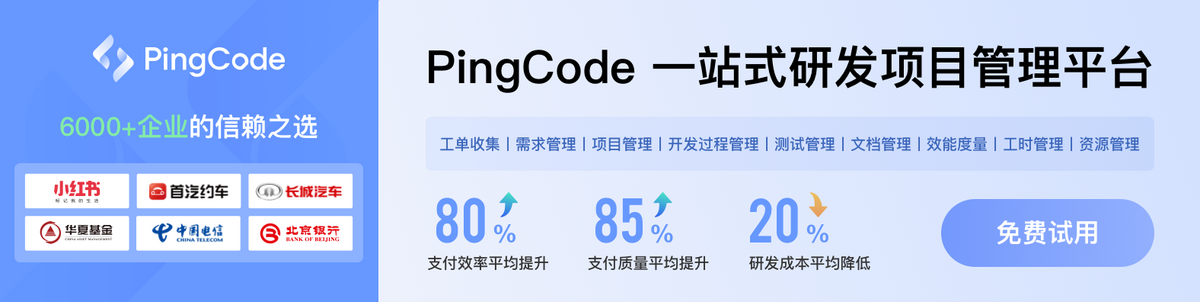