在Python中可以通过多种方法来计算两点之间的距离。使用欧几里得距离公式、使用scipy库、使用numpy库等都是常见的方法。下面将详细介绍其中一种方法,即欧几里得距离公式的使用。
欧几里得距离公式是计算两点之间距离最常用的方法。欧几里得距离公式如下:
[ d = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2} ]
其中,( (x_1, y_1) ) 和 ( (x_2, y_2) ) 是平面上两点的坐标。我们可以使用Python内置的数学函数来实现这个公式。
import math
def euclidean_distance(point1, point2):
return math.sqrt((point2[0] - point1[0]) <strong> 2 + (point2[1] - point1[1]) </strong> 2)
point1 = (1, 2)
point2 = (4, 6)
distance = euclidean_distance(point1, point2)
print("The Euclidean distance is:", distance)
一、使用欧几里得距离公式
1、定义函数
首先,我们可以定义一个函数来计算两点之间的欧几里得距离。这个函数接受两个参数,分别是两个点的坐标。我们可以使用Python的math库来计算平方根。
2、使用示例
import math
def euclidean_distance(point1, point2):
return math.sqrt((point2[0] - point1[0]) <strong> 2 + (point2[1] - point1[1]) </strong> 2)
point1 = (1, 2)
point2 = (4, 6)
distance = euclidean_distance(point1, point2)
print("The Euclidean distance is:", distance)
在这个示例中,point1
和point2
分别是两点的坐标,通过调用euclidean_distance
函数,我们可以计算出这两点之间的距离。这个示例可以很容易地扩展到三维空间或更高维度。
二、使用scipy库
1、安装scipy库
scipy是一个用于科学计算的Python库,其中包含了许多用于计算距离的函数。我们可以使用scipy库中的distance
模块来计算两点之间的距离。首先,我们需要安装scipy库。
pip install scipy
2、使用scipy计算距离
安装完成后,我们可以使用scipy库中的euclidean
函数来计算两点之间的距离。
from scipy.spatial import distance
point1 = (1, 2)
point2 = (4, 6)
dist = distance.euclidean(point1, point2)
print("The Euclidean distance is:", dist)
在这个示例中,我们导入了scipy库中的distance模块,并使用其中的euclidean
函数来计算两点之间的距离。这个函数接受两个参数,分别是两点的坐标,并返回两点之间的欧几里得距离。
三、使用numpy库
1、安装numpy库
numpy是一个用于科学计算的Python库,其中包含了许多用于数组和矩阵操作的函数。我们可以使用numpy库来计算两点之间的距离。首先,我们需要安装numpy库。
pip install numpy
2、使用numpy计算距离
安装完成后,我们可以使用numpy库中的函数来计算两点之间的距离。
import numpy as np
point1 = np.array((1, 2))
point2 = np.array((4, 6))
dist = np.linalg.norm(point1 - point2)
print("The Euclidean distance is:", dist)
在这个示例中,我们使用了numpy库中的array
函数来创建点的坐标,并使用linalg.norm
函数来计算两点之间的距离。这个函数接受一个向量,并返回该向量的范数。在这里,我们传入的是两点坐标的差值向量,从而计算出两点之间的欧几里得距离。
四、扩展到三维空间
1、欧几里得距离公式在三维空间中的应用
在三维空间中,欧几里得距离公式如下:
[ d = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2 + (z_2 – z_1)^2} ]
我们可以使用Python来实现这个公式。
import math
def euclidean_distance_3d(point1, point2):
return math.sqrt((point2[0] - point1[0]) <strong> 2 + (point2[1] - point1[1]) </strong> 2 + (point2[2] - point1[2]) 2)
point1 = (1, 2, 3)
point2 = (4, 6, 8)
distance = euclidean_distance_3d(point1, point2)
print("The Euclidean distance in 3D is:", distance)
在这个示例中,我们定义了一个新的函数euclidean_distance_3d
,它接受两个三维点的坐标,并返回它们之间的欧几里得距离。
2、使用scipy库计算三维空间的距离
我们也可以使用scipy库来计算三维空间中的距离。
from scipy.spatial import distance
point1 = (1, 2, 3)
point2 = (4, 6, 8)
dist = distance.euclidean(point1, point2)
print("The Euclidean distance in 3D is:", dist)
在这个示例中,我们同样使用scipy库中的euclidean
函数来计算三维空间中两点之间的距离。
3、使用numpy库计算三维空间的距离
同样地,我们也可以使用numpy库来计算三维空间中的距离。
import numpy as np
point1 = np.array((1, 2, 3))
point2 = np.array((4, 6, 8))
dist = np.linalg.norm(point1 - point2)
print("The Euclidean distance in 3D is:", dist)
在这个示例中,我们使用了numpy库中的array
函数来创建三维点的坐标,并使用linalg.norm
函数来计算两点之间的距离。
五、曼哈顿距离
1、曼哈顿距离公式
曼哈顿距离是另一种常用的距离度量方法,它是指在一个网格状路径上,两点之间的距离。曼哈顿距离公式如下:
[ d = |x_2 – x_1| + |y_2 – y_1| ]
我们可以使用Python来实现这个公式。
def manhattan_distance(point1, point2):
return abs(point2[0] - point1[0]) + abs(point2[1] - point1[1])
point1 = (1, 2)
point2 = (4, 6)
distance = manhattan_distance(point1, point2)
print("The Manhattan distance is:", distance)
在这个示例中,我们定义了一个函数manhattan_distance
,它接受两个点的坐标,并返回它们之间的曼哈顿距离。
2、使用scipy库计算曼哈顿距离
我们可以使用scipy库中的cityblock
函数来计算曼哈顿距离。
from scipy.spatial import distance
point1 = (1, 2)
point2 = (4, 6)
dist = distance.cityblock(point1, point2)
print("The Manhattan distance is:", dist)
在这个示例中,我们使用scipy库中的cityblock
函数来计算两点之间的曼哈顿距离。
3、使用numpy库计算曼哈顿距离
同样地,我们也可以使用numpy库来计算曼哈顿距离。
import numpy as np
point1 = np.array((1, 2))
point2 = np.array((4, 6))
dist = np.sum(np.abs(point1 - point2))
print("The Manhattan distance is:", dist)
在这个示例中,我们使用了numpy库中的array
函数来创建点的坐标,并使用sum
和abs
函数来计算两点之间的曼哈顿距离。
六、切比雪夫距离
1、切比雪夫距离公式
切比雪夫距离是另一种常用的距离度量方法,它是指在一个网格状路径上,两点之间的最大距离。切比雪夫距离公式如下:
[ d = \max(|x_2 – x_1|, |y_2 – y_1|) ]
我们可以使用Python来实现这个公式。
def chebyshev_distance(point1, point2):
return max(abs(point2[0] - point1[0]), abs(point2[1] - point1[1]))
point1 = (1, 2)
point2 = (4, 6)
distance = chebyshev_distance(point1, point2)
print("The Chebyshev distance is:", distance)
在这个示例中,我们定义了一个函数chebyshev_distance
,它接受两个点的坐标,并返回它们之间的切比雪夫距离。
2、使用scipy库计算切比雪夫距离
我们可以使用scipy库中的chebyshev
函数来计算切比雪夫距离。
from scipy.spatial import distance
point1 = (1, 2)
point2 = (4, 6)
dist = distance.chebyshev(point1, point2)
print("The Chebyshev distance is:", dist)
在这个示例中,我们使用scipy库中的chebyshev
函数来计算两点之间的切比雪夫距离。
3、使用numpy库计算切比雪夫距离
同样地,我们也可以使用numpy库来计算切比雪夫距离。
import numpy as np
point1 = np.array((1, 2))
point2 = np.array((4, 6))
dist = np.max(np.abs(point1 - point2))
print("The Chebyshev distance is:", dist)
在这个示例中,我们使用了numpy库中的array
函数来创建点的坐标,并使用max
和abs
函数来计算两点之间的切比雪夫距离。
七、结论
在Python中计算两点之间的距离有多种方法,包括使用欧几里得距离公式、使用scipy库、使用numpy库等。每种方法都有其优点和适用场景。欧几里得距离适用于计算直线距离,而曼哈顿距离和切比雪夫距离则适用于网格状路径上的距离计算。通过以上示例,我们可以根据不同的需求选择合适的方法来计算两点之间的距离。
相关问答FAQs:
如何在Python中计算两个点之间的距离?
在Python中,计算两个点之间的距离可以使用数学公式,例如欧几里得距离。给定两个点的坐标 (x1, y1) 和 (x2, y2),可以通过以下公式计算距离:
[ \text{distance} = \sqrt{(x2 – x1)^2 + (y2 – y1)^2} ]
可以通过导入 math
模块来使用 math.sqrt()
函数,或者使用 NumPy 库中的向量化操作来计算距离。
Python中有哪些库可以帮助我计算坐标距离?
Python有多个库能够简化坐标距离的计算。常用的包括:
- NumPy:提供了高效的数组和矩阵操作,使用
numpy.linalg.norm()
函数可以轻松计算多维空间中的距离。 - SciPy:提供了更多的科学计算功能,其中的
scipy.spatial.distance
模块包含多种距离计算方法,如曼哈顿距离和余弦相似度等。 - math:适合简单的计算,特别是当只需要计算两个点的欧几里得距离时。
在Python中如何处理三维坐标的距离计算?
对于三维坐标的距离计算,公式会稍作调整。给定点 A(x1, y1, z1) 和点 B(x2, y2, z2),可以使用以下公式:
[ \text{distance} = \sqrt{(x2 – x1)^2 + (y2 – y1)^2 + (z2 – z1)^2} ]
与二维情况一样,可以使用 math
或 numpy
库来实现这一计算,确保输入数据包含三维坐标信息。
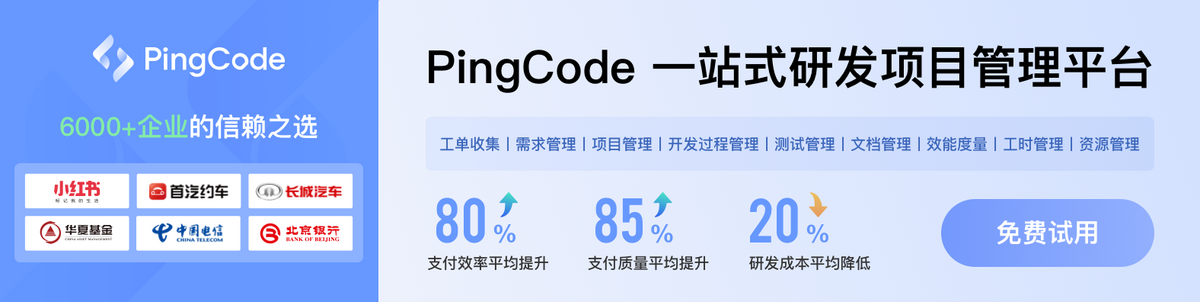