在Python中,实现文件复制文件有几种方法,包括使用内置模块shutil、os模块以及手动读取和写入文件。这些方法中,最常用和最简单的是使用shutil模块。
其中最常用的方法有:
- 使用shutil模块
- 使用os模块
- 手动读取和写入文件
使用shutil模块是最推荐的方法,因为它提供了一些高级操作来复制文件和文件夹,并且在处理大文件时性能较好。shutil模块包含了几个方法,比如shutil.copyfile、shutil.copy、shutil.copy2等,用于不同需求的文件复制。
下面我们详细介绍这三种方法:
一、使用shutil模块
1. 使用shutil.copyfile
shutil.copyfile
方法是最简单的方法之一,它只复制文件的内容,并且要求目标文件不能是一个目录。
import shutil
source_file = 'source.txt'
destination_file = 'destination.txt'
shutil.copyfile(source_file, destination_file)
2. 使用shutil.copy
shutil.copy
方法不仅复制文件内容,还会复制权限位。它适用于目标文件可能是目录的情况。
import shutil
source_file = 'source.txt'
destination_file = 'destination.txt'
shutil.copy(source_file, destination_file)
3. 使用shutil.copy2
shutil.copy2
方法在复制文件内容和权限位的同时,还复制元数据(如时间戳)。这在需要保留文件元数据的情况下非常有用。
import shutil
source_file = 'source.txt'
destination_file = 'destination.txt'
shutil.copy2(source_file, destination_file)
二、使用os模块
os模块提供了底层操作系统接口,可以用来实现文件的复制,不过需要手动处理读取和写入操作。通常不推荐这种方法,因为它比较繁琐且容易出错。
import os
source_file = 'source.txt'
destination_file = 'destination.txt'
with open(source_file, 'rb') as src, open(destination_file, 'wb') as dst:
dst.write(src.read())
三、手动读取和写入文件
这种方法同样不推荐,但在某些特定情况下可能会用到,比如需要对文件内容进行某些处理。
source_file = 'source.txt'
destination_file = 'destination.txt'
读取源文件内容
with open(source_file, 'r') as src:
data = src.read()
写入目标文件
with open(destination_file, 'w') as dst:
dst.write(data)
四、如何选择合适的方法
1. 使用shutil模块的优点
- 简单易用:shutil模块提供了高级的文件操作方法,使得文件复制变得非常简单。
- 功能丰富:shutil模块不仅能复制文件,还能复制文件夹、移动文件、删除文件等。
- 性能优越:shutil模块在处理大文件时性能较好,因为它使用了底层系统调用。
2. 使用os模块的场景
- 自定义操作:在文件复制过程中需要进行一些自定义的操作时,可以使用os模块。
- 跨平台支持:os模块提供了跨平台的操作系统接口,使得代码可以在不同操作系统上运行。
3. 手动读取和写入文件的场景
- 文件处理:在复制文件的过程中需要对文件内容进行处理时,可以使用手动读取和写入文件的方法。
- 细粒度控制:手动读取和写入文件的方法可以提供更细粒度的控制,比如逐行读取和写入。
五、性能对比
在处理大文件时,不同方法的性能可能会有所不同。通常来说,shutil模块的性能较好,因为它使用了底层系统调用。
import shutil
import os
import time
source_file = 'large_file.txt'
destination_file = 'destination_large.txt'
使用shutil.copyfile
start_time = time.time()
shutil.copyfile(source_file, destination_file)
end_time = time.time()
print(f"shutil.copyfile: {end_time - start_time} seconds")
使用os模块
start_time = time.time()
with open(source_file, 'rb') as src, open(destination_file, 'wb') as dst:
dst.write(src.read())
end_time = time.time()
print(f"os module: {end_time - start_time} seconds")
手动读取和写入文件
start_time = time.time()
with open(source_file, 'r') as src:
data = src.read()
with open(destination_file, 'w') as dst:
dst.write(data)
end_time = time.time()
print(f"manual read/write: {end_time - start_time} seconds")
通过以上代码可以对比不同方法的性能,从而选择适合自己需求的方法。
六、常见问题和解决方法
1. 权限问题
在复制文件时,可能会遇到权限问题。可以使用shutil.copy
或shutil.copy2
来复制权限位,或者在复制后手动修改目标文件的权限。
import os
import shutil
source_file = 'source.txt'
destination_file = 'destination.txt'
shutil.copy2(source_file, destination_file)
os.chmod(destination_file, 0o755) # 修改目标文件权限
2. 文件不存在
如果源文件不存在,可能会抛出FileNotFoundError异常。可以在复制文件前检查源文件是否存在。
import os
import shutil
source_file = 'source.txt'
destination_file = 'destination.txt'
if os.path.exists(source_file):
shutil.copyfile(source_file, destination_file)
else:
print(f"{source_file} does not exist")
3. 目标文件已存在
在复制文件时,目标文件可能已经存在。如果不希望覆盖目标文件,可以在复制前检查目标文件是否存在。
import os
import shutil
source_file = 'source.txt'
destination_file = 'destination.txt'
if not os.path.exists(destination_file):
shutil.copyfile(source_file, destination_file)
else:
print(f"{destination_file} already exists")
七、扩展功能
1. 复制文件夹
shutil模块还提供了复制文件夹的方法,比如shutil.copytree
。它可以递归地复制整个文件夹及其内容。
import shutil
source_dir = 'source_folder'
destination_dir = 'destination_folder'
shutil.copytree(source_dir, destination_dir)
2. 移动文件
shutil模块还提供了移动文件的方法,比如shutil.move
。它可以将文件从一个位置移动到另一个位置。
import shutil
source_file = 'source.txt'
destination_file = 'destination.txt'
shutil.move(source_file, destination_file)
3. 删除文件
shutil模块还提供了删除文件的方法,比如shutil.rmtree
。它可以递归地删除整个文件夹及其内容。
import shutil
dir_to_delete = 'folder_to_delete'
shutil.rmtree(dir_to_delete)
八、结论
在Python中,实现文件复制文件的方法有多种,其中最推荐的是使用shutil模块。shutil模块提供了简单易用的高级文件操作方法,适用于大多数文件复制需求。而os模块和手动读取写入文件的方法则适用于一些特定场景,比如需要自定义操作或对文件内容进行处理。在实际应用中,可以根据具体需求选择合适的方法,并注意处理常见问题,如权限问题、文件不存在和目标文件已存在等。通过合理使用这些方法,可以高效地实现文件复制及其他文件操作。
相关问答FAQs:
如何使用Python复制文件?
Python提供了多种方法来复制文件,其中最常用的是利用shutil
模块。通过shutil.copy()
函数可以轻松实现文件的复制。只需提供源文件路径和目标文件路径,即可完成复制操作。示例如下:
import shutil
shutil.copy('source_file.txt', 'destination_file.txt')
文件复制时,是否会保留文件的权限和元数据?
使用shutil.copy()
时,虽然会复制文件的内容,但它不会保留文件的权限和元数据。如果需要保留这些信息,可以使用shutil.copy2()
,这个函数会复制文件内容和元数据。
shutil.copy2('source_file.txt', 'destination_file.txt')
在复制大型文件时,有没有推荐的优化方法?
对于大型文件的复制,使用shutil.copyfileobj()
函数会更高效。此方法可以在内存中逐块读取和写入文件数据,避免一次性加载整个文件,适合处理大文件。可以通过设置缓冲区大小来优化性能:
with open('source_file.txt', 'rb') as source:
with open('destination_file.txt', 'wb') as destination:
shutil.copyfileobj(source, destination, length=1024*1024) # 每次复制1MB
这些方法能够帮助用户根据不同需求有效地实现文件复制。
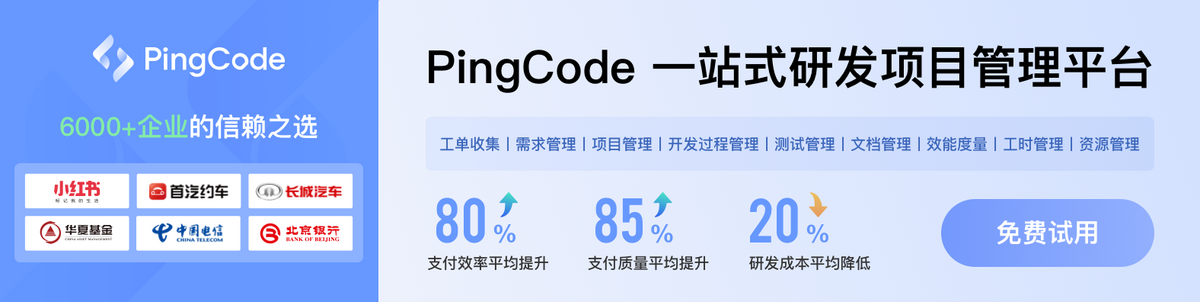