制作动态图的核心工具是Matplotlib和其动画子库FuncAnimation。要创建Python动态图,首先需要导入所需的库、定义绘图函数、设置动画参数、生成和保存动画。让我们详细讨论每个步骤。
一、导入所需的库
在Python中制作动态图的首选库是Matplotlib。我们首先需要安装并导入Matplotlib库,以及NumPy库来生成数据。
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
二、创建基本绘图
在创建动画之前,先创建一个静态图形。我们将绘制一个简单的正弦波图形。
fig, ax = plt.subplots()
x = np.linspace(0, 2 * np.pi, 100)
y = np.sin(x)
line, = ax.plot(x, y)
三、定义更新函数
更新函数在每个动画帧中被调用,用于更新图形内容。这个函数应该接受一个参数“帧编号”,并更新图形对象。
def update(frame):
y = np.sin(x + frame / 10.0)
line.set_ydata(y)
return line,
四、创建动画
使用FuncAnimation创建动画对象。该对象需要提供绘图的figure、更新函数、帧数和间隔时间等参数。
ani = animation.FuncAnimation(fig, update, frames=100, interval=50, blit=True)
plt.show()
五、保存动画
动画可以保存为GIF或MP4文件。需要安装额外的库如ImageMagick(保存为GIF)或FFmpeg(保存为MP4)。
ani.save('sine_wave.gif', writer='imagemagick')
六、实例:制作散点图动画
我们将进一步展示如何制作一个动态散点图。
1、导入库
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
2、生成数据
x = np.random.rand(100)
y = np.random.rand(100)
colors = np.random.rand(100)
sizes = 1000 * np.random.rand(100)
3、创建初始图
fig, ax = plt.subplots()
scatter = ax.scatter(x, y, c=colors, s=sizes, alpha=0.5)
4、定义更新函数
def update(frame):
x = np.random.rand(100)
y = np.random.rand(100)
colors = np.random.rand(100)
sizes = 1000 * np.random.rand(100)
scatter.set_offsets(np.c_[x, y])
scatter.set_sizes(sizes)
scatter.set_array(colors)
return scatter,
5、创建和保存动画
ani = animation.FuncAnimation(fig, update, frames=50, interval=200, blit=True)
ani.save('scatter.gif', writer='imagemagick')
plt.show()
七、实例:动态折线图
我们将展示如何制作一个动态折线图。
1、导入库
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
2、生成数据
x = np.arange(0, 2 * np.pi, 0.01)
y = np.sin(x)
3、创建初始图
fig, ax = plt.subplots()
line, = ax.plot(x, y)
4、定义更新函数
def update(frame):
y = np.sin(x + frame / 10.0)
line.set_ydata(y)
return line,
5、创建和保存动画
ani = animation.FuncAnimation(fig, update, frames=100, interval=50, blit=True)
ani.save('line.gif', writer='imagemagick')
plt.show()
八、实例:动态条形图
我们将展示如何制作一个动态条形图。
1、导入库
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
2、生成数据
x = np.arange(10)
y = np.random.rand(10)
3、创建初始图
fig, ax = plt.subplots()
bars = ax.bar(x, y)
4、定义更新函数
def update(frame):
y = np.random.rand(10)
for bar, height in zip(bars, y):
bar.set_height(height)
return bars,
5、创建和保存动画
ani = animation.FuncAnimation(fig, update, frames=50, interval=200, blit=True)
ani.save('bar.gif', writer='imagemagick')
plt.show()
九、实例:动态热图
动态热图是数据可视化的重要工具,尤其适用于展示二维数据的变化。
1、导入库
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
2、生成数据
data = np.random.rand(10, 10)
3、创建初始图
fig, ax = plt.subplots()
heatmap = ax.imshow(data, cmap='viridis')
4、定义更新函数
def update(frame):
data = np.random.rand(10, 10)
heatmap.set_data(data)
return heatmap,
5、创建和保存动画
ani = animation.FuncAnimation(fig, update, frames=50, interval=200, blit=True)
ani.save('heatmap.gif', writer='imagemagick')
plt.show()
十、实例:动态3D图
3D动画可以提供更丰富的视觉效果,尤其在展示复杂数据时。
1、导入库
import matplotlib.pyplot as plt
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.animation as animation
2、生成数据
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
x, y = np.meshgrid(x, y)
z = np.sin(np.sqrt(x<strong>2 + y</strong>2))
3、创建初始图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
surface = ax.plot_surface(x, y, z, cmap='viridis')
4、定义更新函数
def update(frame):
ax.clear()
z = np.sin(np.sqrt(x<strong>2 + y</strong>2) + frame / 10.0)
surface = ax.plot_surface(x, y, z, cmap='viridis')
return surface,
5、创建和保存动画
ani = animation.FuncAnimation(fig, update, frames=100, interval=50, blit=True)
ani.save('3d_surface.gif', writer='imagemagick')
plt.show()
十一、实例:动态饼图
动态饼图可以清晰展示数据在各个类别中的变化。
1、导入库
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
2、生成数据
data = np.random.rand(4)
labels = ['A', 'B', 'C', 'D']
3、创建初始图
fig, ax = plt.subplots()
wedges, texts, autotexts = ax.pie(data, autopct='%1.1f%%', startangle=140)
4、定义更新函数
def update(frame):
data = np.random.rand(4)
ax.clear()
wedges, texts, autotexts = ax.pie(data, autopct='%1.1f%%', startangle=140)
return wedges, texts, autotexts
5、创建和保存动画
ani = animation.FuncAnimation(fig, update, frames=50, interval=200, blit=True)
ani.save('pie_chart.gif', writer='imagemagick')
plt.show()
十二、最佳实践
- 优化性能:尽量避免在更新函数中创建新的图形对象,而是更新现有对象的属性。
- 使用blit参数:在FuncAnimation中使用blit=True可以提高动画性能,特别是在处理大量数据时。
- 选择合适的间隔时间:根据数据更新频率选择合适的间隔时间,以确保动画流畅。
- 调试更新函数:在创建动画之前,确保更新函数能正确更新图形内容。
- 保存动画:根据需求选择合适的格式保存动画,如GIF用于网页展示,MP4用于高质量存储。
总结
本文详细介绍了使用Matplotlib制作动态图的各个步骤,包括导入库、创建基本图形、定义更新函数、创建和保存动画。同时,通过多个实例展示了如何制作不同类型的动态图,如散点图、折线图、条形图、热图、3D图和饼图。通过这些实例和最佳实践,你可以轻松创建各种类型的动态图,提高数据可视化的效果。
相关问答FAQs:
如何使用Python制作动态图?
制作动态图的过程通常涉及几个步骤。首先,您需要选择适合的库,比如Matplotlib、Plotly或Seaborn。根据您的需求,Matplotlib可以通过FuncAnimation
类轻松创建动态效果,而Plotly则提供交互性强的图表。接下来,您需要准备数据,确保数据格式适合于动态图的展示。完成这些步骤后,您可以编写代码,通过循环或时间序列更新图形的内容,最终生成所需的动态图。
Python中的动态图与静态图有什么区别?
动态图与静态图主要在于数据展示的方式。静态图是在绘制时固定下来的,不会随着数据的变化而更新;而动态图则可以根据数据的变化实时更新图形,提供更直观的视觉效果。动态图常用于展示时间序列数据或需要实时更新的信息,可以帮助用户更好地理解数据变化的趋势和动态。
制作动态图时常见的错误有哪些?
在制作动态图时,有几种常见错误需要注意。首先,数据未更新或格式不正确,可能导致图形无法正确展示。其次,动画速度设置不当,可能使得动态图过快或过慢,影响观看体验。此外,内存管理不当可能导致程序崩溃,尤其是在处理大量数据时。确保使用适当的参数设置和优化代码,可以减少这些问题的发生。
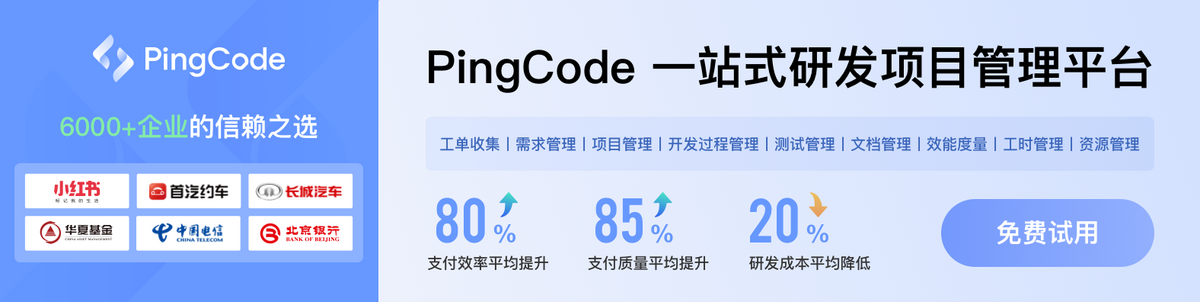